In C++, a shallow copy duplicates the immediate values of an object, sharing memory for complex data types, while a deep copy creates a completely independent clone, including all dynamically allocated memory.
Here's a simple example demonstrating both shallow and deep copy in C++:
#include <iostream>
#include <cstring>
class MyClass {
public:
int value;
char* data;
MyClass(int v, const char* d) : value(v) {
data = new char[strlen(d) + 1];
strcpy(data, d);
}
// Shallow copy constructor
MyClass(const MyClass& other) : value(other.value), data(other.data) {}
// Deep copy constructor
MyClass deepCopy(const MyClass& other) {
MyClass obj(other.value);
obj.data = new char[strlen(other.data) + 1];
strcpy(obj.data, other.data);
return obj;
}
~MyClass() {
delete[] data;
}
};
int main() {
MyClass original(42, "Hello");
MyClass shallowCopy = original; // Shallow copy
MyClass deepCopy = original.deepCopy(original); // Deep copy
std::cout << "Original: " << original.data << "\n";
std::cout << "Shallow Copy: " << shallowCopy.data << "\n";
std::cout << "Deep Copy: " << deepCopy.data << "\n";
// Modify original's data
strcpy(original.data, "World");
std::cout << "\nAfter modification:\n";
std::cout << "Original: " << original.data << "\n";
std::cout << "Shallow Copy: " << shallowCopy.data << "\n"; // Affected
std::cout << "Deep Copy: " << deepCopy.data << "\n"; // Not affected
return 0;
}
Understanding Shallow Copy
Definition of Shallow Copy
A shallow copy refers to copying an object in such a way that only the outermost layer of the structure is duplicated while the references to nested objects are shared. This means that if the original object and the copied object modify shared references, both will reflect the change since they point to the same memory location.
How Shallow Copy Works in C++
In C++, a shallow copy can be performed using the default copy constructor provided by the compiler. When the copy constructor is utilized, it copies all member variables of the object, including pointers and references, which results in both the original and copied objects sharing the same memory space for dynamically allocated members.
Memory Layout During Shallow Copy Operations
When a shallow copy is made:
- The memory addresses of pointers are copied, not the actual data.
- If one object alters the data pointed to by a shared pointer, the other object sees this change because they refer to the same memory.
Example of Shallow Copy in C++
Consider the following code snippet demonstrating a shallow copy:
class Shallow {
public:
int* data;
Shallow(int value) {
data = new int(value);
}
// Shallow Copy Constructor
Shallow(const Shallow& other) {
data = other.data; // Pointing to the same memory location
}
~Shallow() {
delete data; // Will crash if both original and copy are deleted
}
};
In this example, the constructor creates a new integer and allocates memory for it. The shallow copy constructor merely copies the pointer, meaning both instances (original and copy) will point to the same integer. When one instance is destructed, it will `delete` the memory, and the second instance will be left with a dangling pointer, leading to undefined behavior.
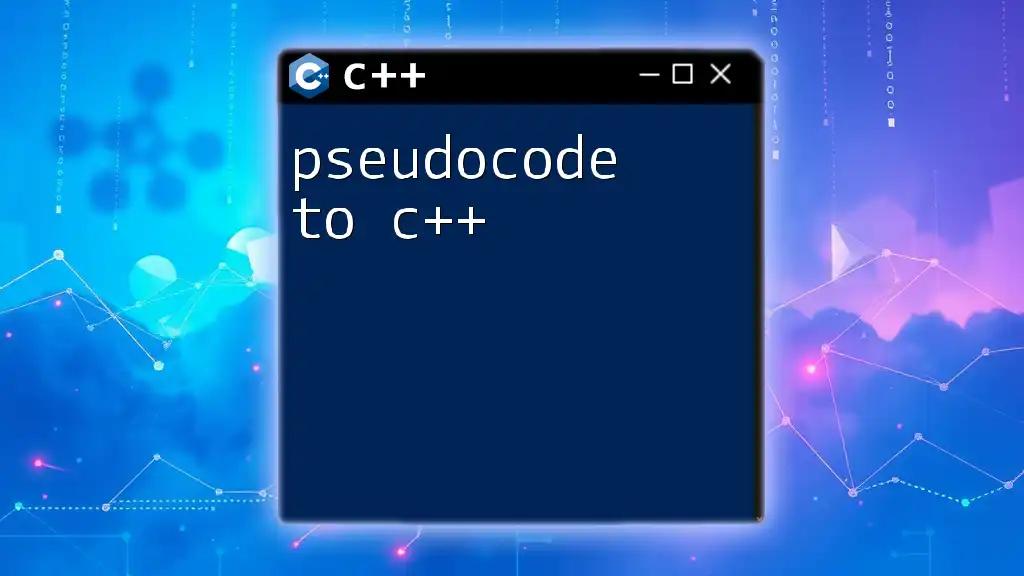
Understanding Deep Copy
Definition of Deep Copy
A deep copy, on the other hand, creates a new object and recursively copies all objects found within the original. This means that any references to dynamically allocated memory within the object are also duplicated, ensuring that the original and copied objects operate independently of one another.
How Deep Copy Works in C++
Deep copies require defining a custom copy constructor that allocates new memory for pointers and copies the actual data being pointed to rather than just the pointers themselves. This allows independent manipulation of the data in each instance.
Memory Layout During Deep Copy Operations
With a deep copy:
- New memory is allocated for each dynamically allocated member.
- Each object has its own copy of the data, avoiding the pitfalls of shared references.
Example of Deep Copy in C++
Here’s how a deep copy can be implemented:
class Deep {
public:
int* data;
Deep(int value) {
data = new int(value);
}
// Deep Copy Constructor
Deep(const Deep& other) {
data = new int(*other.data); // Allocating new memory for the copy
}
~Deep() {
delete data; // Safe to delete, as each has its own data
}
};
In this example, the deep copy constructor allocates new memory and copies the value pointed to by the original data. This ensures that each instance maintains its own separate copy of the integer.
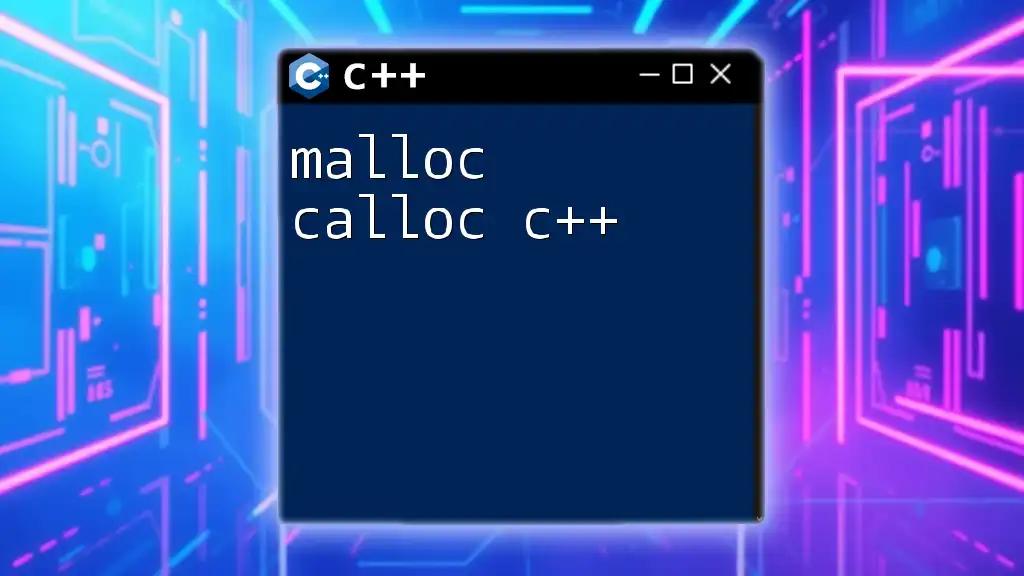
Comparing Shallow Copy and Deep Copy
Key Differences
- Memory Management: Shallow copies share memory addresses for references, while deep copies allocate separate memory for each instance.
- Performance Considerations: Shallow copying is often faster due to less memory allocation overhead; however, deep copying is safer when independent object states are required.
- Use Cases: Use shallow copy when objects hold immutable data or simple types, and opt for deep copy when working with complex objects that modify shared data.
Visual Representation
To better understand the differences, imagine a box (the object) with a single key (pointer) inside it. A shallow copy of the box would mean making another identical box with another key pointing to the original box’s contents. A deep copy, in contrast, would create a completely new box with its own key to a new set of contents.
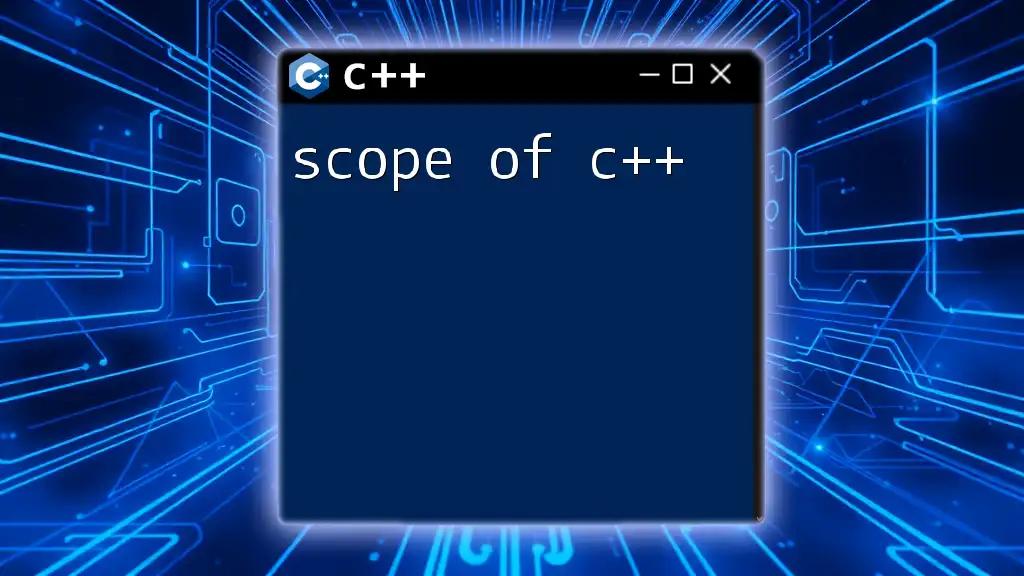
Potential Pitfalls of Each Method
Issues with Shallow Copy
While shallow copying is efficient, it can lead to:
- Double Deletion: If both objects are destroyed, they’ll attempt to delete the same memory, causing runtime errors or crashes.
- Dangling Pointers: If one object is destructed and the other tries to access the shared memory, it can lead to undefined behavior.
Issues with Deep Copy
Although deep copying avoids some pitfalls of shallow copying, it isn't without its drawbacks:
- Performance Overhead: Deep copying can be slower due to additional memory allocation and data copying processes.
- Complexity in Memory Management: Managing multiple copies requires careful consideration to avoid memory leaks, particularly if the objects contain more complex nested structures.

Best Practices for Copying in C++
When to Use Shallow Copy
Utilize shallow copy in simple applications where:
- Objects hold immutable data, such as basic types or constant data.
- Memory efficiency is paramount and there’s no risk of dangling pointers.
When to Use Deep Copy
Deep copying is essential when:
- Objects are mutable, and changes to one instance should not affect another.
- Complex data structures require independent management.
Avoiding premature optimization or unnecessary complexity is crucial. Always assess the specific requirements of your application before deciding on a copying method.

Conclusion
Understanding the concepts of shallow copy and deep copy in C++ is fundamental for effective memory management and data integrity in programming. Recognizing when to use each type of copy can greatly enhance your code's reliability and performance. Always assess your specific use cases to make informed decisions regarding memory handling in your applications.

Frequently Asked Questions
-
What is the default copy behavior in C++? The default copy behavior in C++ performs a shallow copy of an object.
-
Can I customize copy behavior in my classes? Yes, custom copy constructors and assignment operators can be implemented to manage copying behavior explicitly.
-
How does the Rule of Three relate to shallow and deep copying? The Rule of Three states that if you define a destructor, copy constructor, or copy assignment operator, you should define all three to manage resources correctly.