To debug C++, you can use the built-in debugger in your IDE or employ tools like GDB to step through your code, inspect variables, and trace the flow of execution by compiling with debugging symbols.
Here’s an example of how to use GDB to compile and debug a simple C++ program:
// simple.cpp
#include <iostream>
int main() {
int a = 5, b = 0;
std::cout << "Result: " << a / b << std::endl; // This will cause a runtime error
return 0;
}
To compile with debugging symbols and run it in GDB:
g++ -g simple.cpp -o simple
gdb ./simple
Understanding Basic Debugging Terminology
What is Debugging?
Debugging is the systematic process of identifying, isolating, and fixing problems or "bugs" in a software program. These issues can manifest in various forms, such as unexpected behavior, crashes, or incorrect output. The ultimate goal of debugging is to ensure that the program runs smoothly and produces the desired results. Understanding debugging is essential for every C++ programmer, as it enhances the overall quality and reliability of their code.
Types of Bugs in C++
In C++, bugs can generally be categorized into three types:
1. Syntax Errors: These occur when the code does not conform to the syntax rules of the C++ language, preventing it from compiling. Common examples include misspelled keywords and missing punctuation.
2. Runtime Errors: These are issues that arise while the program is running, often leading to crashes or undefined behavior. For instance, trying to access memory that is not allocated can cause a runtime error.
3. Logic Errors: These bugs occur when the code compiles and runs without crashing, but produces incorrect results. A typical example is an incorrect formula in calculations.
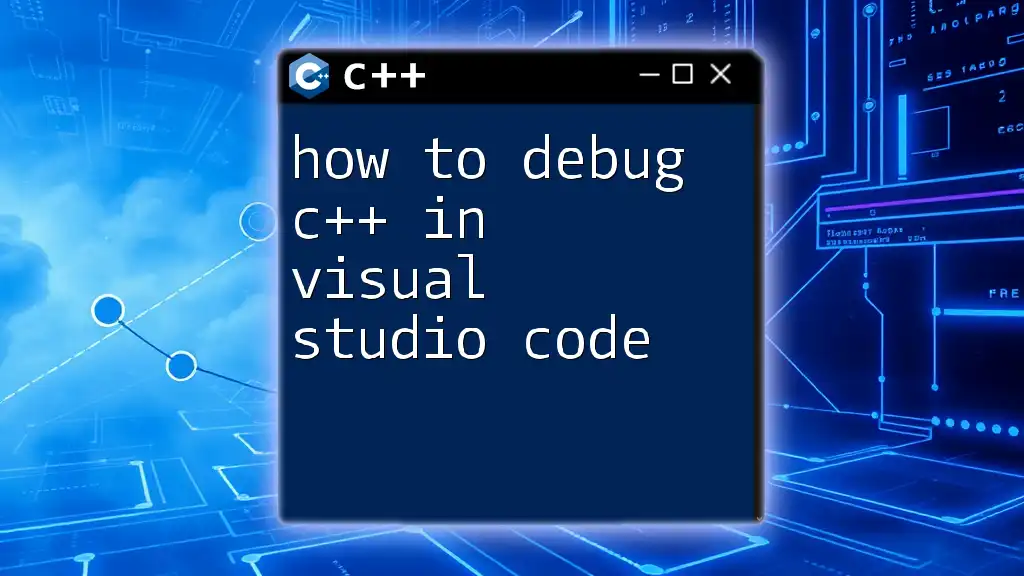
Setting Up Your Debugging Environment
Choosing a C++ IDE/Debugger
The choice of an Integrated Development Environment (IDE) or debugger is crucial for effective debugging. Some popular IDEs include:
- Visual Studio: Offers a powerful debugging interface with advanced features.
- CLion: Provides excellent support for CMake projects and includes robust refactoring tools.
- Code::Blocks: A lightweight IDE that works well for beginner programmers.
Each IDE comes with built-in debugging features, making it essential to select one that fits your development style and project requirements.
Configuring Debugger Settings
To fully utilize the debugging capabilities of your chosen IDE, it’s essential to configure its settings properly. Optimization settings can significantly influence the speed and efficiency of the debugger. For example, ensure that you compile your code with debug symbols enabled, which allows more informative outputs during debugging.
Moreover, setting up breakpoints and watchpoints is crucial for an effective debugging experience. Breakpoints allow you to halt program execution at specific lines, enabling you to inspect variable states.
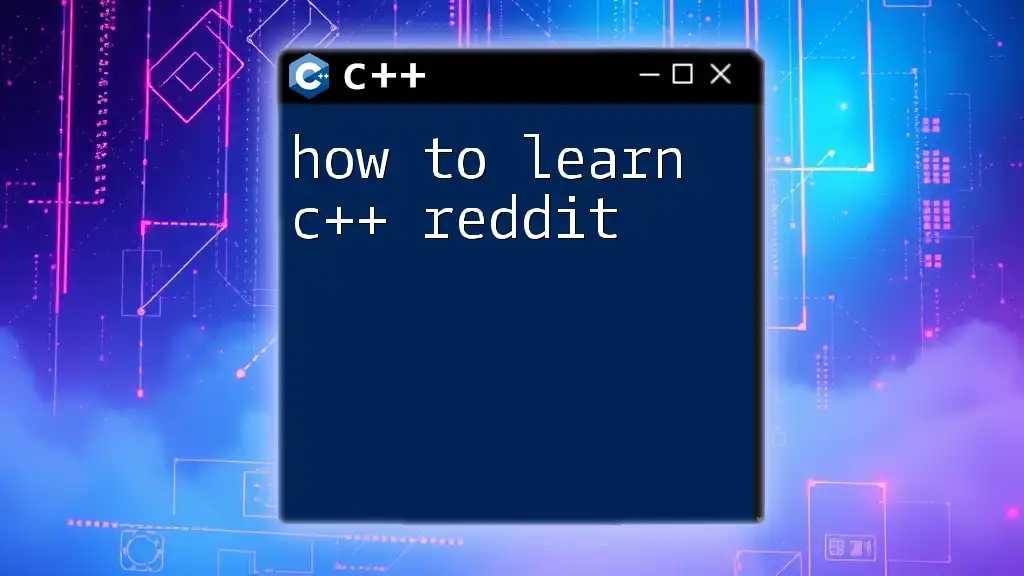
Common Debugging Techniques
Using Print Statements
One of the simplest yet effective debugging techniques involves inserting print statements into your code. This technique helps to verify that certain parts of your code are being executed as intended and allows you to check the values of variables at different points in time.
Code Example: Using `std::cout` to Trace Variables
#include <iostream>
int main() {
int x = 5;
int y = 10;
std::cout << "Before calculation, x: " << x << ", y: " << y << std::endl;
int sum = x + y;
std::cout << "Sum: " << sum << std::endl;
return 0;
}
In this code snippet, the use of `std::cout` before and after the calculation allows you to trace the variables' values effectively.
Utilizing Breakpoints
Setting breakpoints is a powerful debugging technique. They allow you to pause program execution at designated points, giving you a chance to inspect the state of your application and the values contained in variables. Most IDEs feature a straightforward method for setting breakpoints, typically by clicking alongside the line number.
When a breakpoint is hit, you can control the execution flow by stepping over, into, or out of functions. This practice makes locating logic errors simpler by providing insight into the control flow of your program.
Inspecting Variables
While debugging, inspecting variable values is crucial for understanding how your program is behaving in real-time. You can usually hover over the variable's name in the IDE, or use a dedicated variable watch window to monitor the values continuously.
Watch expressions allow you to evaluate specific variables of interest during execution, enabling you to diagnose how variable values change over time, which can be vital in pinpointing the source of complex bugs.
Call Stack Inspection
A call stack is a data structure that tracks active subroutines (function calls) in your program. By inspecting the call stack, you can gain insights into the order in which functions are called, revealing how you arrived at your current point in the program.
This information becomes invaluable when diagnosing how a particular state was reached, especially if many functions interconnect. It can help you identify incorrect function calls or unexpected returns that lead to bugs.
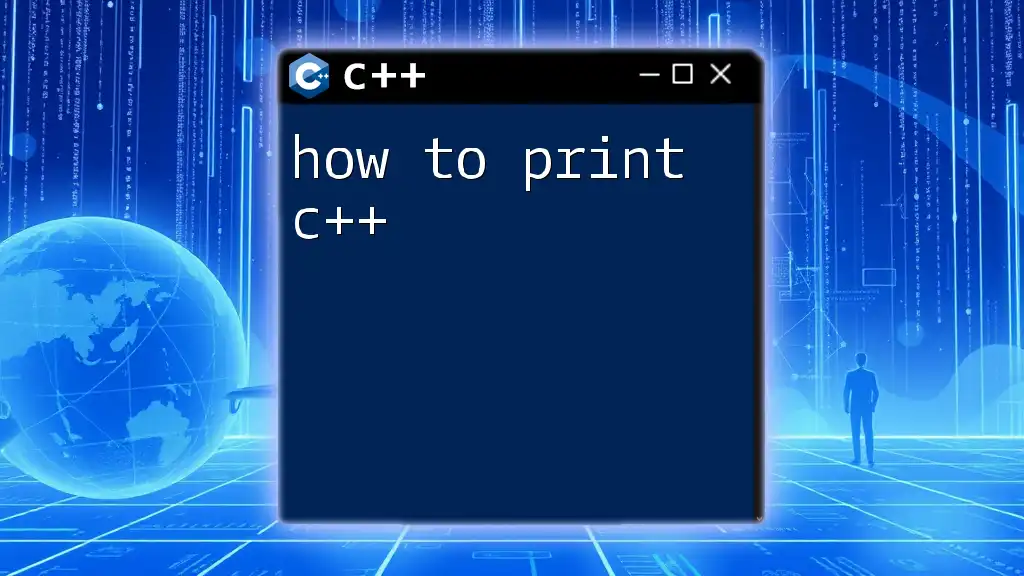
Advanced Debugging Techniques
Memory Leak Detection
One of the most notorious pitfalls in C++ programming is memory management, where memory leaks can lead to significant performance issues. A memory leak occurs when allocated memory is not properly released after use.
Common Causes of Memory Leaks in C++:
- Failing to delete dynamically allocated memory.
- Not using modern C++ practices such as smart pointers.
To help detect memory leaks, tools like Valgrind or AddressSanitizer can be used. These tools perform comprehensive checks and report any instances of memory usage that do not conform to best practices.
Code Example:
#include <cstdlib>
int main() {
int* arr = new int[10]; // Memory allocated
// Forgetting to delete leads to memory leak
// delete[] arr; // Uncommenting this would prevent the leak
return 0;
}
In the example, memory allocated for `arr` is never released, leading to a leak that tools would flag during analysis.
Using Static Analysis Tools
Static code analysis helps identify potential issues by examining code without executing it. This practice can catch errors before runtime, saving time in the debugging process.
Popular Tools:
- CPPcheck: A static analysis tool that detects bugs and undefined behavior.
- Clang-Tidy: Offers refactoring options alongside analysis.
Integrating these tools into your workflow can help maintain code quality and prevent issues before they escalate into complex bugs.
Automated Testing and Debugging
Incorporating automated testing is a forward-thinking approach to debugging. Unit tests can help ensure that individual components behave as expected, serving both as documentation and a safety net for future modifications.
Testing Frameworks:
- Google Test: A widely-used framework for testing C++ code.
- Catch2: Offers a user-friendly interface with powerful features.
Code Example of a Simple Unit Test:
#include <gtest/gtest.h>
int add(int a, int b) {
return a + b;
}
TEST(AddTest, HandlesPositiveInput) {
EXPECT_EQ(add(1, 2), 3);
}
In this example, a unit test verifies that the `add` function returns the correct sum, providing a level of assurance as you develop.
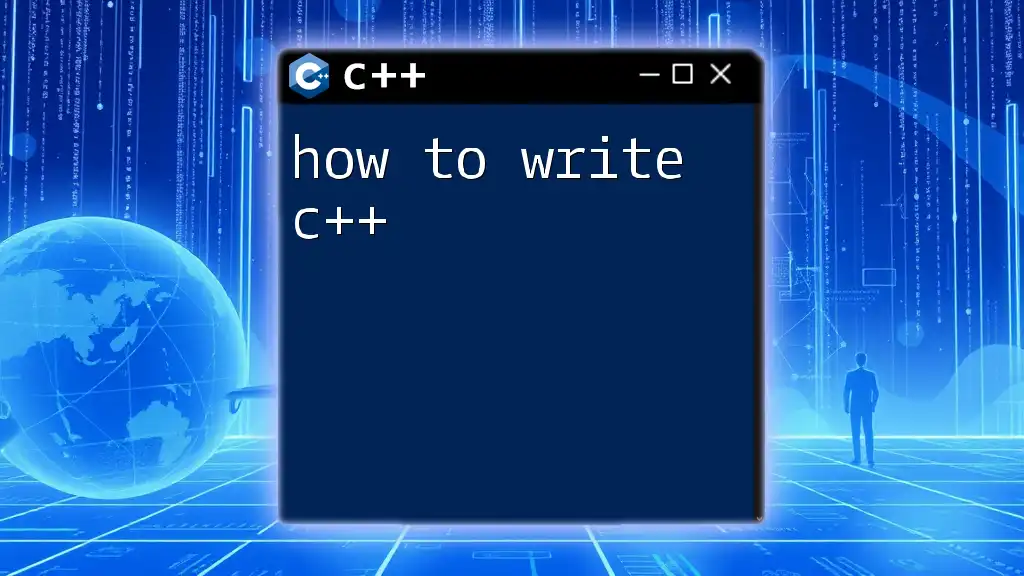
Debugging Best Practices
Write Clear and Concise Code
Clear and functional code is typically easier to debug. Following best practices in coding, such as naming conventions and readability, can significantly enhance the debugging process. Refactoring code to avoid complexity is a process worth investing in.
Document Your Code
Proper documentation is one of the most effective tools you have for debugging. Adding informative comments in your code not only helps you but also aids others in understanding the logic and flow when they encounter issues.
Maintaining a log of changes and errors encountered can help you notice patterns and improve your debugging skills progressively.
Learn from Bugs
Every bug encountered is an opportunity to learn. By identifying patterns in the bugs that arise within your code, you can adapt your coding practices to mitigate their recurrence. Take notes on how different bugs arose and the steps taken to resolve them; this record will serve as a valuable resource in the future.
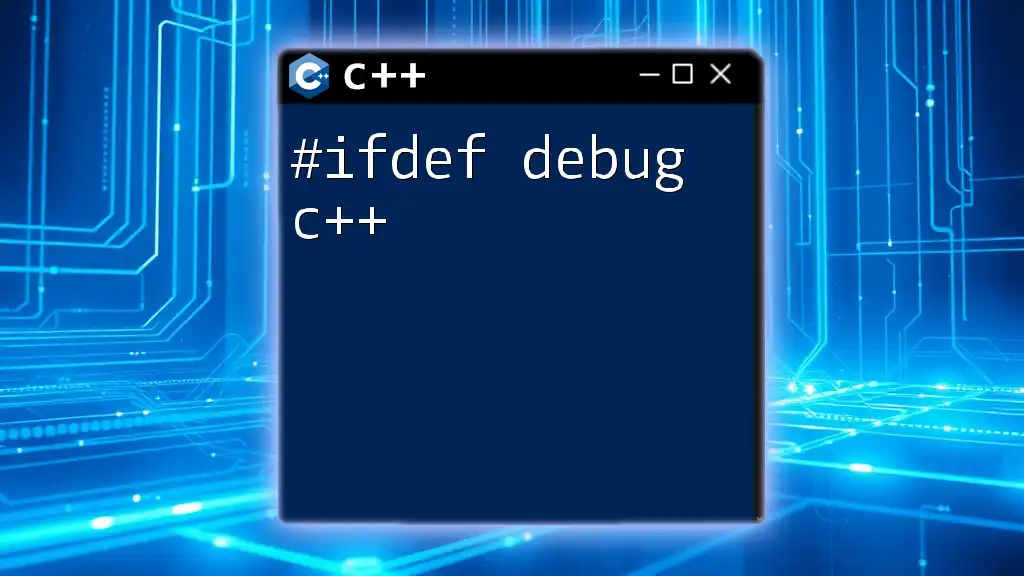
Conclusion
Debugging C++ is an essential skill that every developer must master. By applying the techniques discussed in this guide, such as using print statements, breakpoints, and memory leak detection tools, you can enhance your debugging prowess. With practice and attention to best practices, you will inevitably improve the quality of your code while creating more robust applications. Remember that learning is a continuous journey—embrace each bug, and let it lead you towards becoming a better C++ programmer.
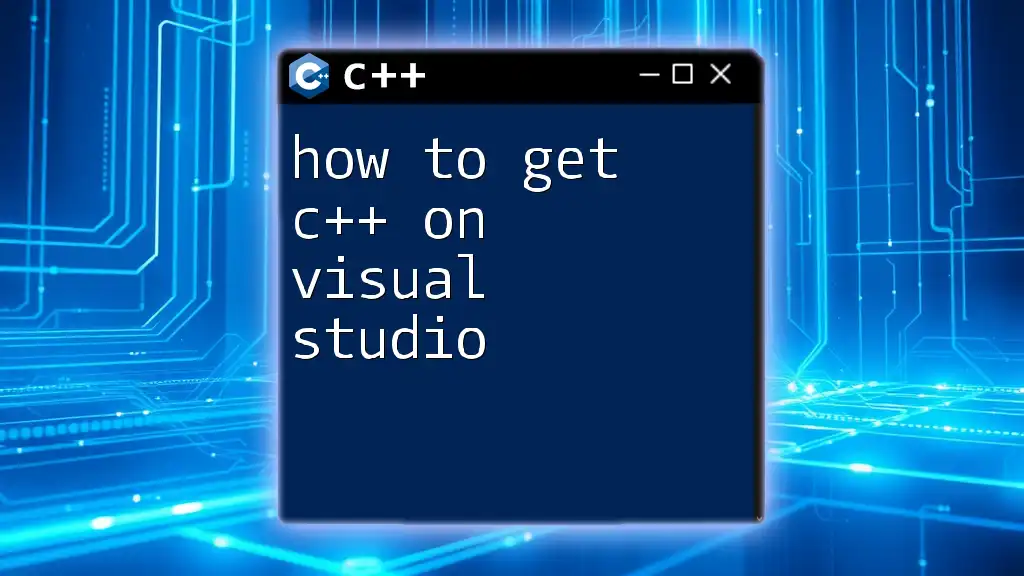
Additional Resources
Consider exploring recommended books on C++ debugging and online tutorials for in-depth learning, as well as engaging with community forums and support groups for shared knowledge and insights.