To run a C++ file in the terminal, you need to compile it using a compiler like `g++` and then execute the resulting binary file; for example, if your C++ file is named `example.cpp`, you can do this with the following commands:
g++ example.cpp -o example && ./example
Understanding the Basics of C++
What is C++?
C++ is a powerful, high-performance programming language widely used for a variety of applications. It offers essential features like object-oriented programming, low-level memory manipulation, and efficient performance, making it ideal for system/software development, game development, and more.
The Role of the Terminal
The terminal (or command line interface) is a text-based interface that allows users to interact with their operating system. Understanding how to use the terminal is crucial for programmers, as it provides a way to execute commands, compile code, and manage files efficiently. Different operating systems have slightly different terminal applications—Windows uses Command Prompt or PowerShell, while Linux and macOS have Terminal or iTerm2.
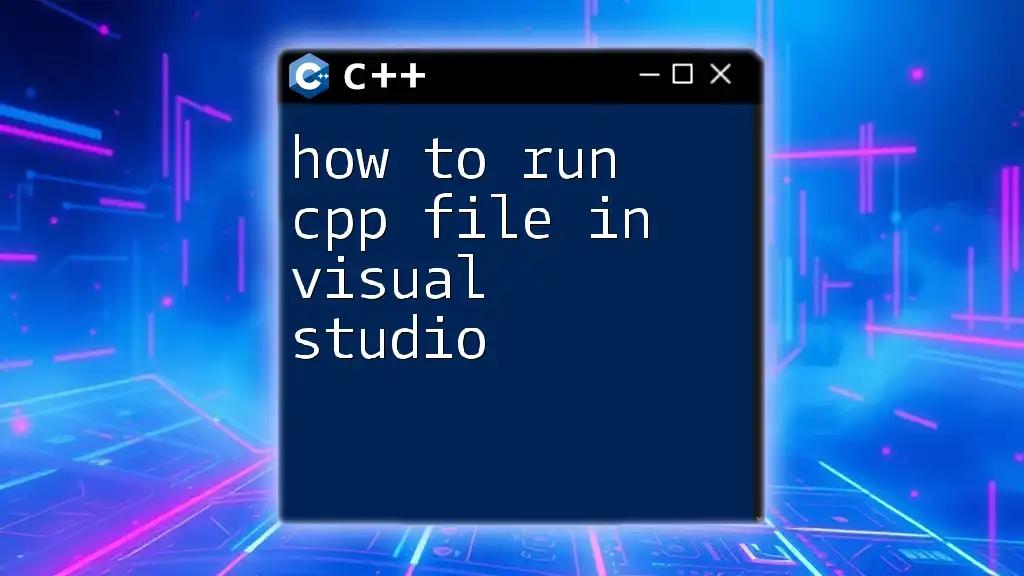
Setting Up Your Environment
Installing a C++ Compiler
Before you can learn how to run a C++ file in terminal, you need to install a C++ compiler, as it translates your high-level C++ code into machine code that the computer can execute. Common compilers include:
- GCC (GNU Compiler Collection): Popular on Linux.
- Clang: Often used in macOS development.
- MSVC (Microsoft Visual C++): Typically used for Windows applications.
Step-by-Step Guide on Installation
- For Windows: You can download MinGW, which includes the GCC compiler. Installation instructions are readily available on the MinGW website. Alternatively, you can install Microsoft Visual Studio, which comes with MSVC.
- For Linux: Most distributions have GCC pre-installed. You can install it via your package manager. For example, using Ubuntu:
sudo apt-get install g++
- For macOS: You can install GCC through Homebrew using:
brew install gcc
Choosing a Text Editor
Choosing the right text editor will enhance your coding experience. Popular choices include:
- Visual Studio Code: A free, powerful editor with plenty of extensions.
- Vim: A command-line editor known for its efficiency.
- Nano: A simple editor, ideal for terminal use.
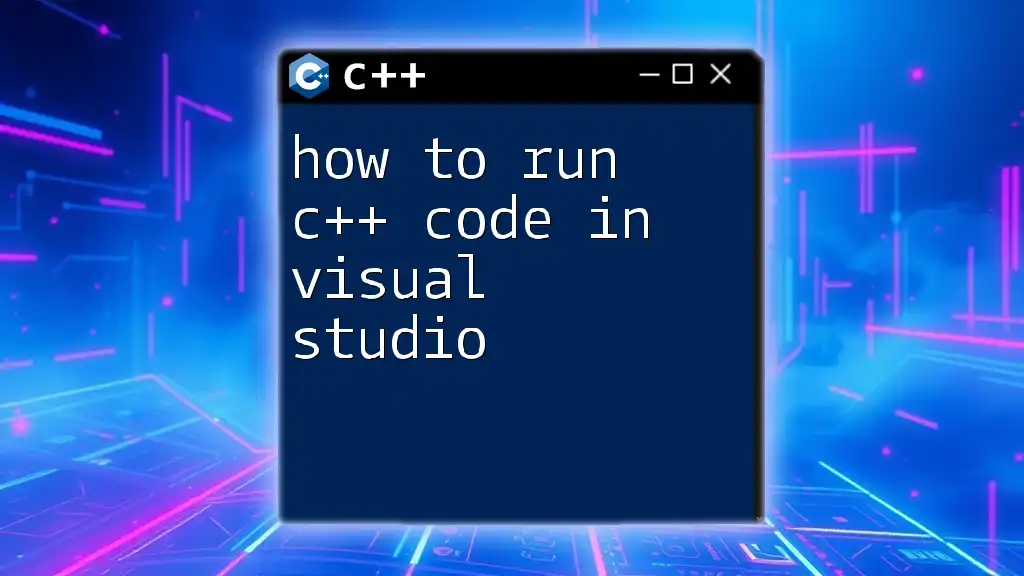
Writing Your First C++ Program
Creating a Simple C++ File
Now that you have set up your environment, the next step is to create your first C++ program. Open your text editor and write the following code for a simple “Hello, World!” program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Saving Your C++ File
Save the above code in a file with a .cpp extension, like `HelloWorld.cpp`. Choosing a meaningful name makes it easier to identify your files later on.
![How to Delete Char in Terminal C++ [Quick Guide]](/images/posts/h/how-to-delete-char-in-terminal-cpp.webp)
Compiling Your C++ Program in Terminal
What is Compilation?
Compilation is the process of translating your high-level C++ code into machine code. This is a crucial step before running any C++ program, as the terminal only understands machine code.
Compiling the C++ File
To compile your C++ file, open your terminal and navigate to the directory where your file is saved using the `cd` command. Then, execute the following command for GCC:
g++ -o HelloWorld HelloWorld.cpp
Here, `g++` is the compiler, `-o HelloWorld` specifies the output filename, and `HelloWorld.cpp` is the input file. The terminal will produce no output if the compilation is successful.
Understanding Compiler Flags
Compiler flags modify how the compiler operates. For instance, `-Wall` can be added to enable all compiler’s warning messages, which helps in identifying potential issues in your code:
g++ -Wall -o HelloWorld HelloWorld.cpp
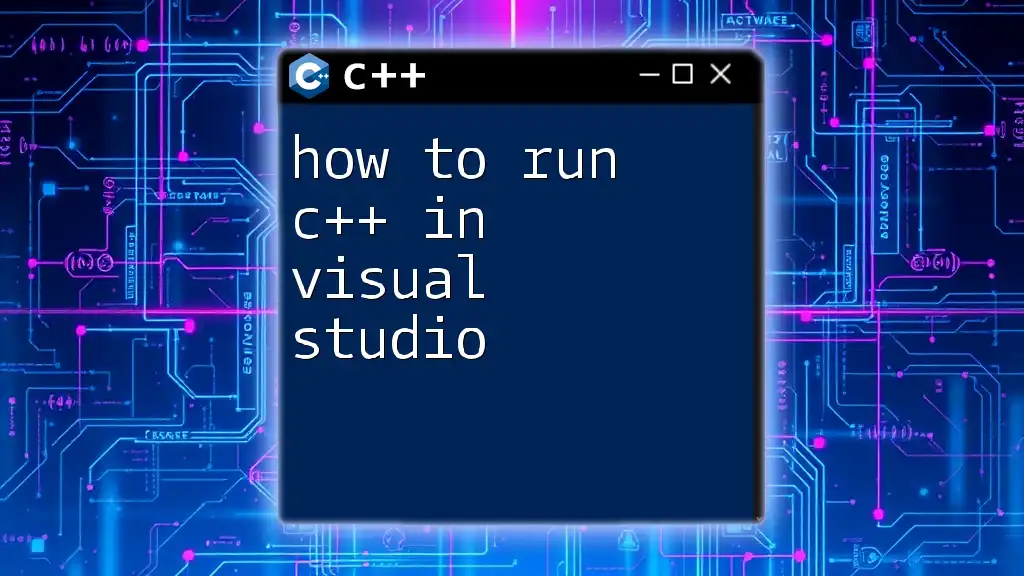
Running Your Compiled C++ Program
How to Execute a C++ Program in Terminal
Once your C++ file is successfully compiled, it generates an executable file. To run your program, simply type the following command in the terminal:
./HelloWorld
You should see the output:
Hello, World!
Common Errors and Fixes
While working within the terminal, you may encounter errors during compilation or execution. Common compilation errors include syntax mistakes or missing files. If your program doesn’t run, check the error messages provided by the compiler, as they are usually quite descriptive. For runtime errors, ensure that your code logic is correct.
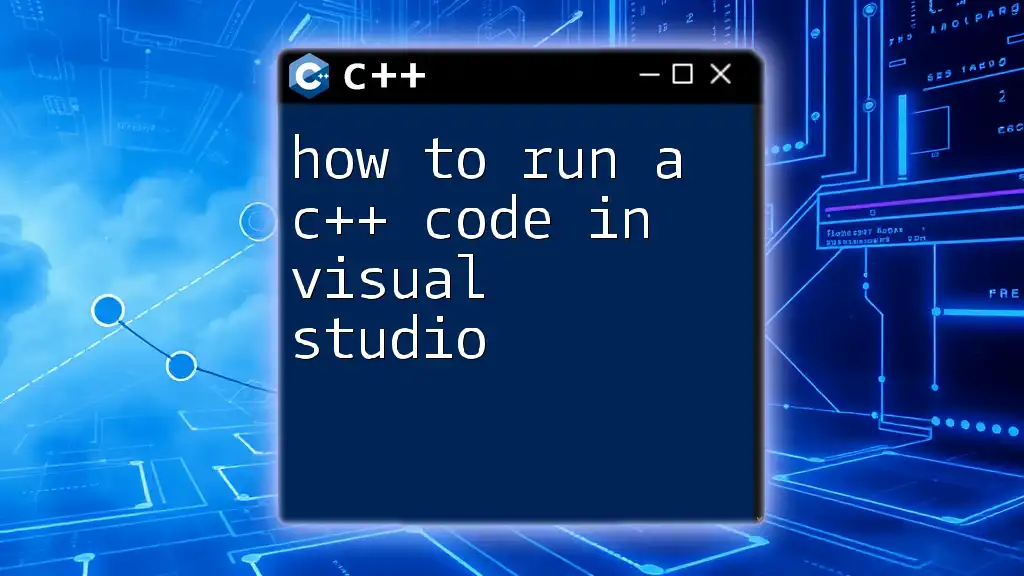
Advanced Compilation Techniques
Using Makefiles
As your projects become more complex, consider using Makefiles for easier management of your build process. Makefiles help automate the compilation of multiple source files. An example of a basic Makefile could look like this:
all: HelloWorld
HelloWorld: HelloWorld.cpp
g++ -o HelloWorld HelloWorld.cpp
clean:
rm HelloWorld
In this Makefile, running `make` will compile your program, and `make clean` will remove the output file.
Compiling Multiple Source Files
If you have multiple C++ files in a project, you can compile them all together. Suppose you have `main.cpp` and `utils.cpp`, you can compile them using:
g++ -o MyProgram main.cpp utils.cpp
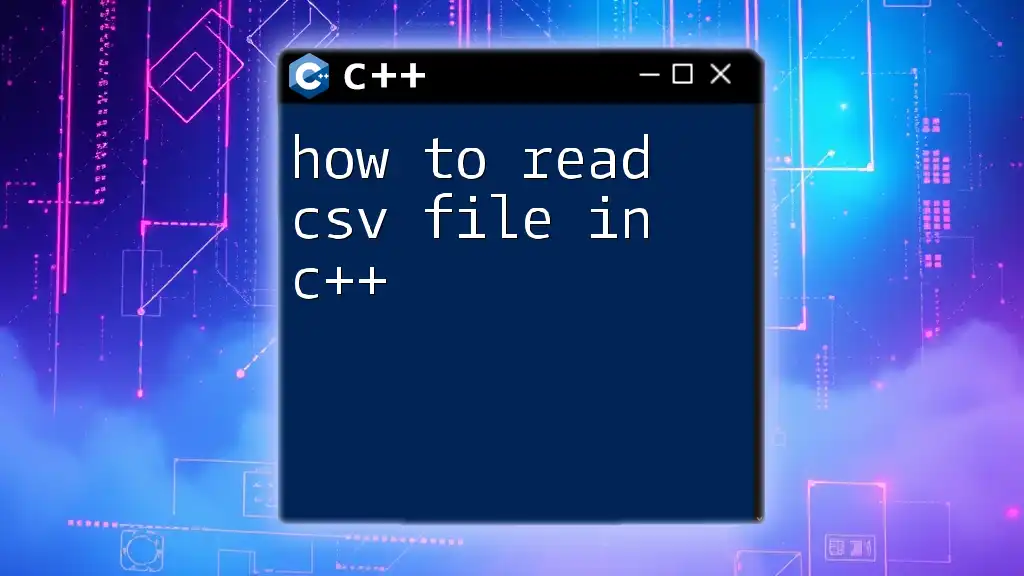
Additional Tips for Running C++ Programs in Terminal
Debugging Your C++ Code
Debugging is essential for identifying issues in your program. Tools like `gdb` (GNU Debugger) allow you to step through your code line-by-line to find logical errors. To start debugging:
gdb ./HelloWorld
Inside gdb, you can run your program, set breakpoints, and inspect variables.
Integrating with Version Control
Using version control systems like Git can greatly enhance your development process. It allows you to track changes, collaborate with others, and revert to previous versions of your code. Initialize a Git repository in your project folder using:
git init
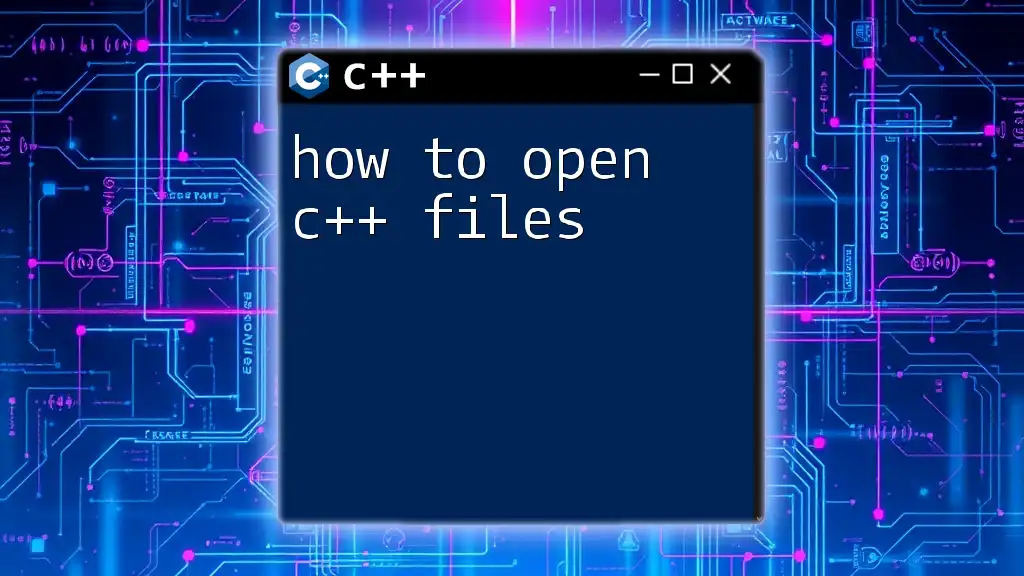
Conclusion
Now you’re equipped with the fundamental knowledge on how to run a C++ file in terminal. Practice compiling and executing various C++ programs to gain more confidence. As you advance, explore more complex programs and projects. Don’t hesitate to experiment and put your skills to the test!
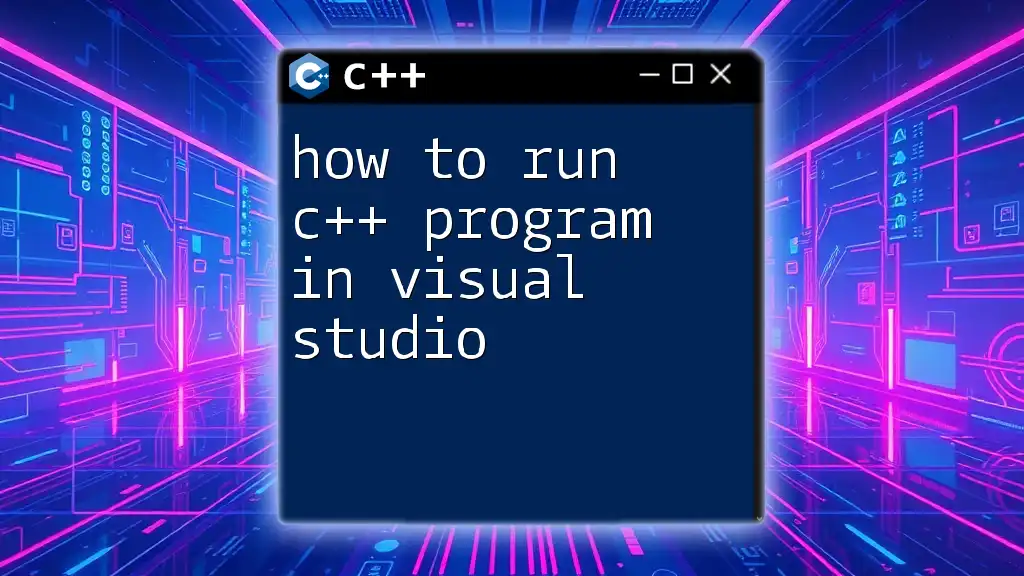
FAQs
How do I run a CPP file in terminal on Windows?
On Windows using MinGW, navigate to the directory in Command Prompt and use the same commands as you would in Linux:
g++ -o HelloWorld HelloWorld.cpp
./HelloWorld
What is the command to run a C++ program in Linux?
The command remains consistent across platforms. After compiling, just type:
./HelloWorld
Can I run C++ code directly in the terminal without compiling?
While some online IDEs allow for direct code execution, C++ typically always requires compilation to run the code locally. However, tools like `clang` allow for REPL-like environments for some quick tests if you want to avoid manual compilation.
By following this guide, you have laid the foundation for not just writing but also executing your C++ programs efficiently from the terminal!