To use C++ in Visual Studio, simply create a new project, select the C++ application template, and write your code in the provided editor before compiling and running it.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Visual Studio
What is Visual Studio?
Visual Studio is a powerful Integrated Development Environment (IDE) widely used for developing applications across multiple programming languages, including C++. It provides a comprehensive suite of tools for writing, testing, and debugging code, making it an ideal choice for both beginners and experienced developers.
Installing Visual Studio
System Requirements Before installing Visual Studio, ensure your system meets the minimum hardware and software requirements. A modern processor (Intel or AMD) and at least 2 GB of RAM (4 GB or more is recommended) will help development speed, alongside sufficient disk space for installation.
Download and Installation Steps You can download Visual Studio from the official Microsoft website. Once there, you'll see different editions available, including Community, Professional, and Enterprise. The Community edition is free for individual developers and small teams.
- Click on the Download button for the Community edition.
- Once downloaded, run the installer.
- During installation, you’ll be prompted to select workloads. Make sure to select the "Desktop development with C++" workload. This includes everything you need for C++ development, including compilers and libraries.
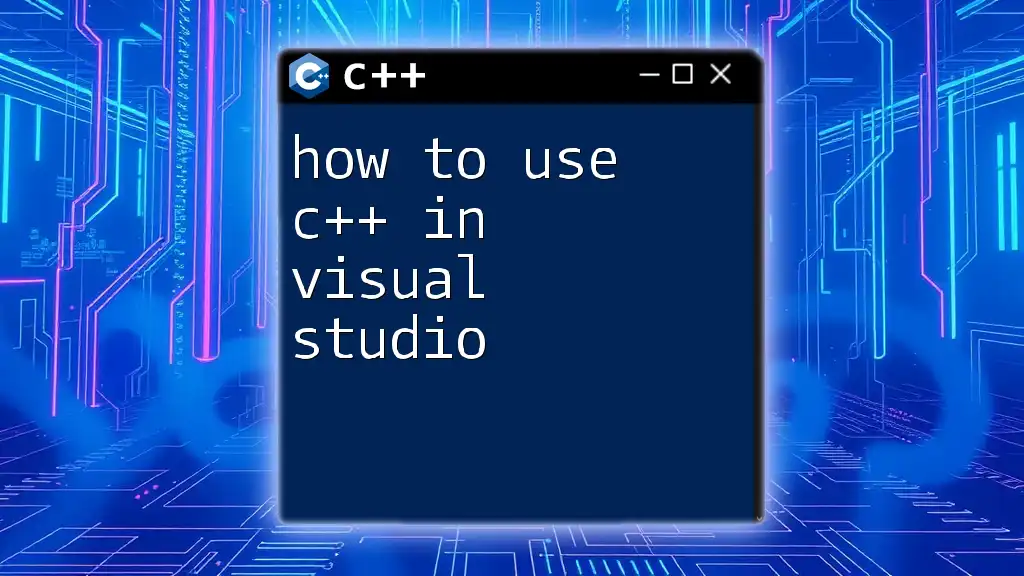
Setting Up a C++ Project
Creating a New Project
Launching Visual Studio Once Visual Studio is installed, launch it to access the start menu.
Creating a New C++ Project
- Select "Create a new project."
- In the project type dropdown, filter by C++.
- Choose the Console Application template if you're starting with a simple command-line application.
Configuring Project Settings
Understanding Project Properties Every project has properties that determine how it is built, linked, and run. Familiarize yourself with these settings to customize your project based on your needs.
Setting up Debugging Options To ensure smooth debugging, navigate to the project properties:
- Right-click on the project in Solution Explorer.
- Select Properties.
- In the Debugging section, you can set various options like command arguments, working directories, and specific environments.
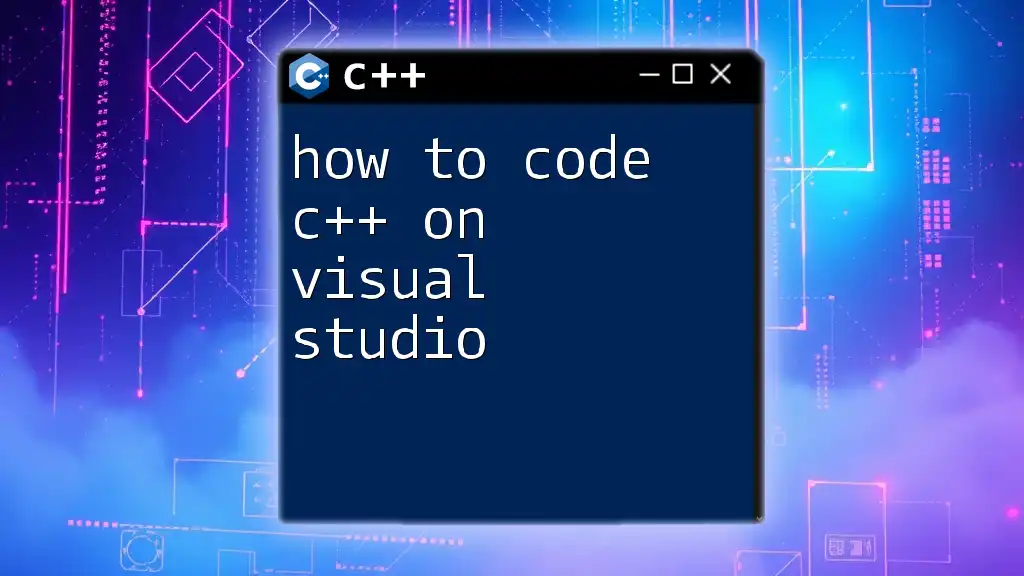
Writing Your First C++ Program
The Basics of C++ Syntax
C++ syntax is structured but straightforward. At its most basic, a C++ program consists of:
- Headers: Use `#include` to include libraries.
- Functions: The entry point is usually `int main()`.
- Output Statements: Use `std::cout` for displaying text.
Here’s a simple example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code snippet, `#include <iostream>` allows us to use input-output streams, while `std::cout` is used for outputting text to the console.
Compiling and Running Your Program
Building Your Project To compile your code:
- Navigate to the Build menu at the top.
- Select Build Solution (or simply press Ctrl + Shift + B).
Running Your Program Once your program compiles without errors, you can run it in various ways. The easiest method is to click the Start button or press F5. This action will run your program in debug mode, enabling you to step through your code if needed.
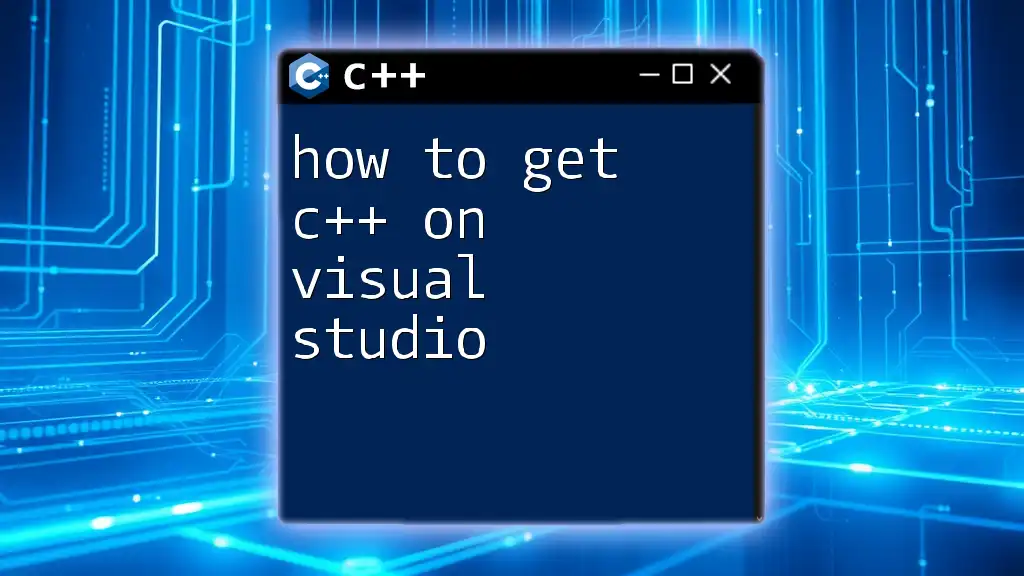
Debugging Techniques
Setting Breakpoints
Debugging is crucial for identifying and rectifying errors in your code. Breakpoints allow you to pause execution at a specific line of code, letting you inspect variable values and program state. Simply click on the left margin next to the line number where you wish to place a breakpoint.
Using the Debugger Tools
Debugger Windows When you hit a breakpoint and enter debug mode, several windows will be available:
- Locals: Displays local variables and their current values.
- Watch: Allows you to add variables you want to monitor during execution.
- Call Stack: Shows the active function calls.
Stepping Through Your Code Utilize the stepping features to control the flow of execution:
- Step Into (F11): Step into a function to debug it line by line.
- Step Over (F10): Execute the line of code without stepping into functions.
- Step Out (Shift + F11): Completes the execution of the current function and returns to the calling function.
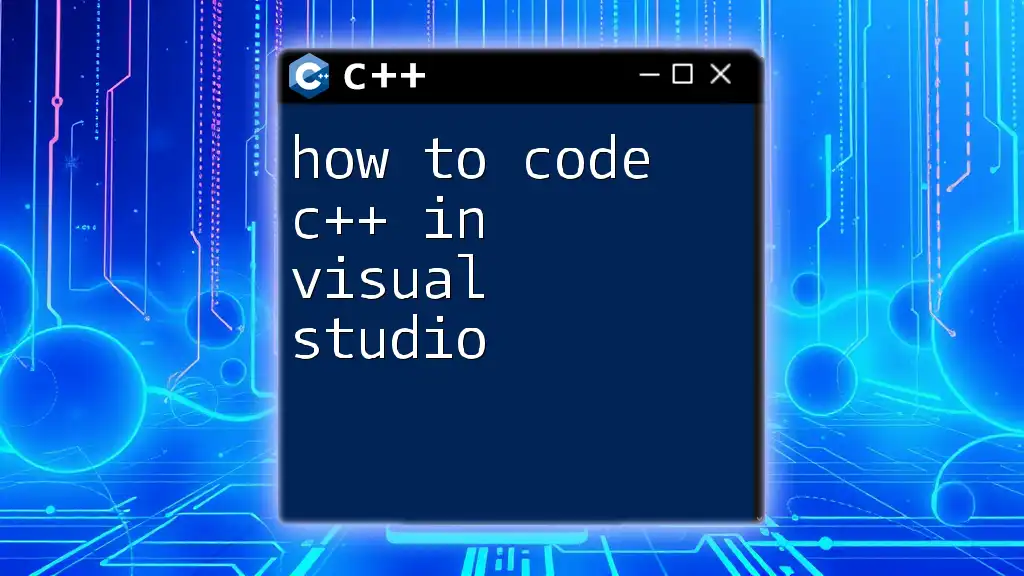
Enhancing Your C++ Skills
Using Libraries and Frameworks
C++ benefits from a rich ecosystem of libraries. Popular libraries like the Standard Template Library (STL) and Boost offer enhanced functionality and faster development times.
To integrate libraries into Visual Studio:
- Go to the project properties.
- Under C/C++ > General, add the path to your library under Additional Include Directories.
- For linking, under Linker > Input, add the library names to Additional Dependencies.
Building a Simple Application
One practical way to apply your C++ knowledge is by building a simple Calculator application. Below is a basic example of how this could look:
#include <iostream>
int main() {
// Simple Calculator example
float num1, num2;
char operation;
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter operation (+, -, *, /): ";
std::cin >> operation;
std::cout << "Enter second number: ";
std::cin >> num2;
switch (operation) {
case '+':
std::cout << (num1 + num2) << std::endl;
break;
case '-':
std::cout << (num1 - num2) << std::endl;
break;
case '*':
std::cout << (num1 * num2) << std::endl;
break;
case '/':
if (num2 != 0)
std::cout << (num1 / num2) << std::endl;
else
std::cout << "Error! Division by zero." << std::endl;
break;
default:
std::cout << "Invalid operation!" << std::endl;
}
return 0;
}
This example covers basic operations and includes error handling for division by zero. Expanding on this simple program could involve adding more operations, user input validation, or even a graphical user interface.
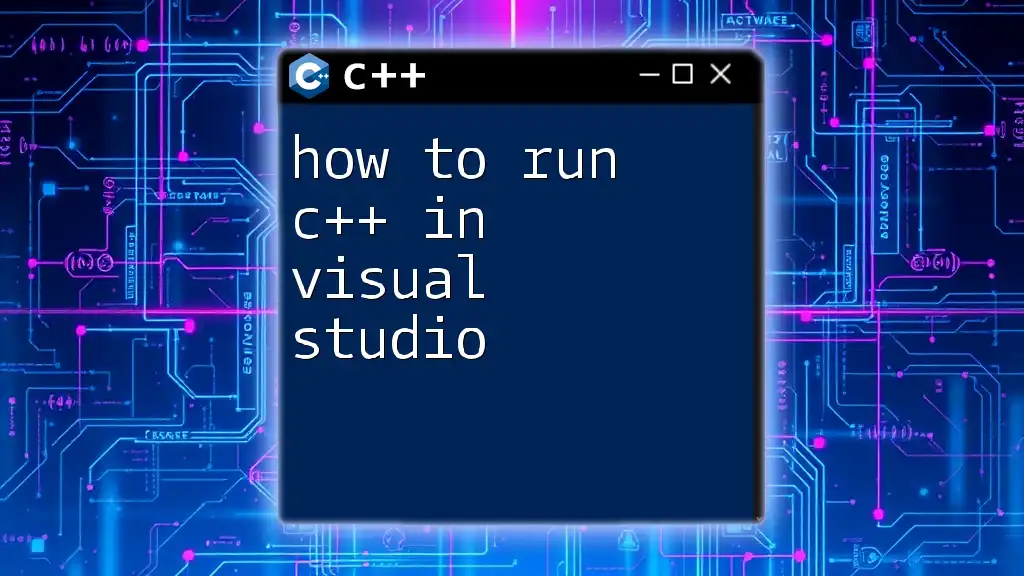
Conclusion
Mastering how to use C++ on Visual Studio is an invaluable skill that can enhance your programming capabilities. Through understanding the IDE, setting up projects, writing and debugging code, and utilizing external libraries, you can significantly improve your proficiency in C++. Practice regularly and explore additional resources to deepen your knowledge and skills in C++ programming.
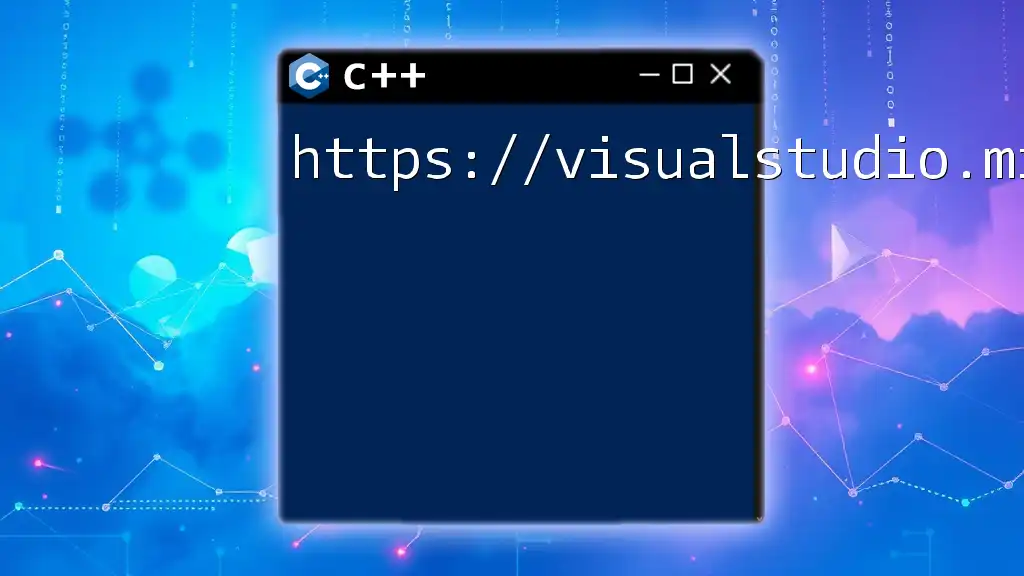
Additional Resources
To further your journey in learning C++, consider reviewing the official C++ documentation or Microsoft’s Visual Studio guides. Additionally, online courses and textbooks can provide more structured learning paths and deeper insights into advanced concepts.
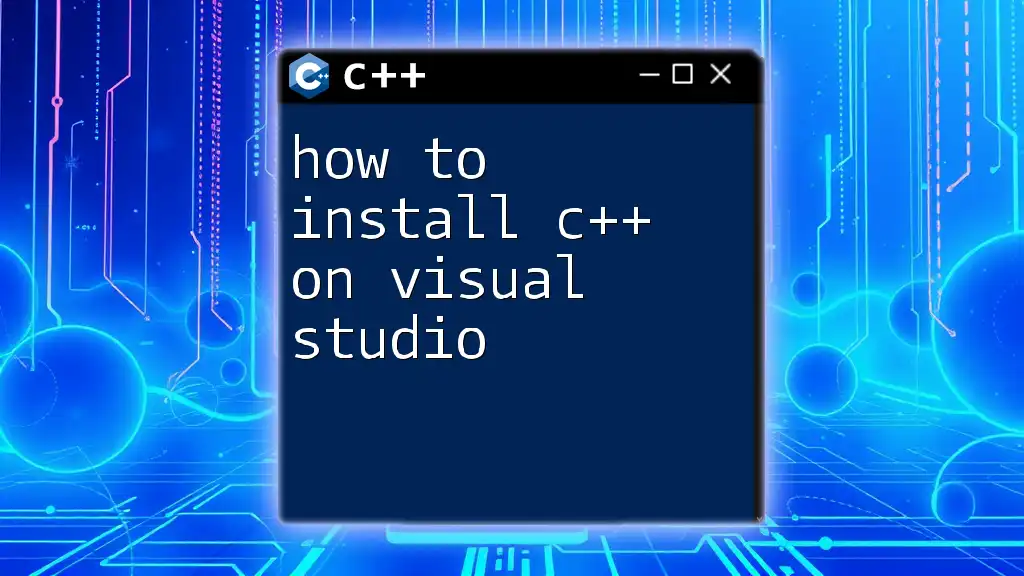
FAQs
Common Issues When Using C++ on Visual Studio
Troubleshooting compilation errors often involves checking for syntax mistakes or missing libraries. Use the output window for detailed error messages.
Where to Learn More About C++
Further recommendations for both online and offline resources can provide valuable learning experiences, from video tutorials to coding boot camps. Engage in C++ programming communities as they can offer great support and guidance.