In C++, a boolean is a data type that can hold two values, `true` or `false`, and is commonly used in conditional statements and control flow.
Here's a simple example demonstrating the use of a boolean in an if statement:
#include <iostream>
int main() {
bool isSunny = true;
if (isSunny) {
std::cout << "It's a sunny day!" << std::endl;
} else {
std::cout << "It's not sunny today." << std::endl;
}
return 0;
}
What is a Boolean Value?
A Boolean value is a data type that can hold one of two possible values: true or false. It forms the backbone of decision-making in programming; conditional statements, loops, and logical operations all rely heavily on Booleans to determine the flow of execution. Understanding how to use Boolean values effectively in C++ is crucial for writing efficient and logical code.
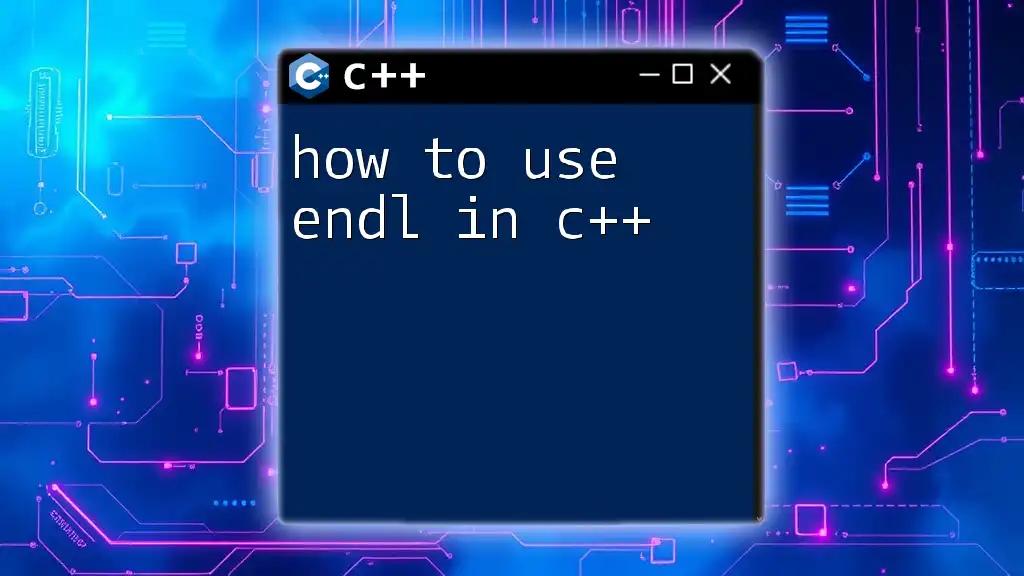
Understanding `bool` Data Type in C++
In C++, the `bool` data type is specifically designed to handle Boolean values. A `bool` variable can only represent two states:
- `true`
- `false`
This makes `bool` particularly useful for controlling the logic of the program, ensuring that code executes only under specific conditions.
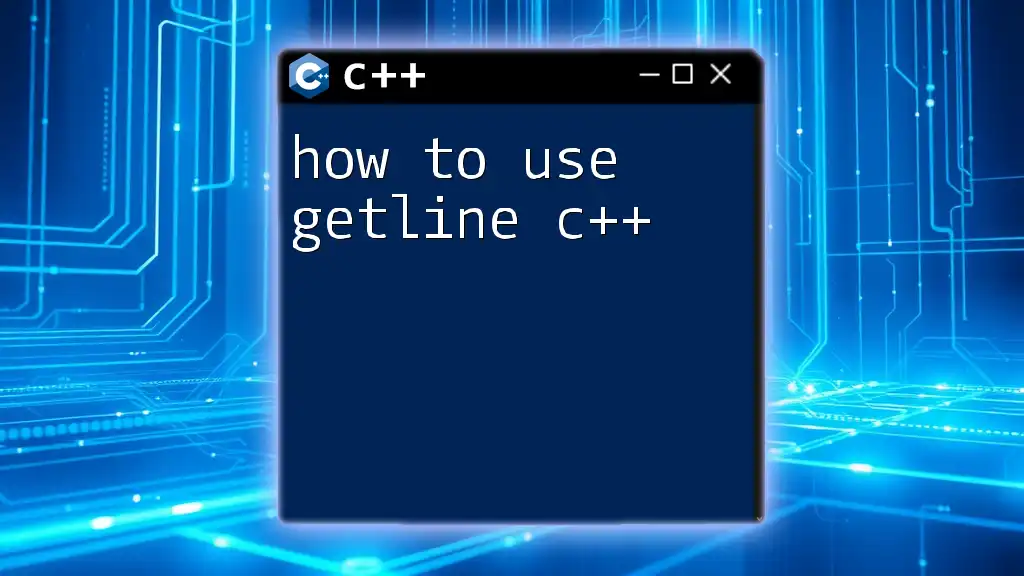
How to Declare and Use Boolean Variables in C++
Declaring a Boolean Variable
Declaring a Boolean variable is straightforward. Use the `bool` keyword followed by your variable name:
bool isActive = true;
This line of code initializes a Boolean variable `isActive` to `true`. Initializing your variables is essential to avoid undefined behavior in your programs.
Assigning Values to Boolean Variables
You can also assign values to a Boolean variable after declaring it. Here’s how it looks:
bool isRaining;
isRaining = false;
In this code, the Boolean variable `isRaining` is declared without an initial value and is later assigned `false`. This flexibility lets you change the state of a variable as your program runs.
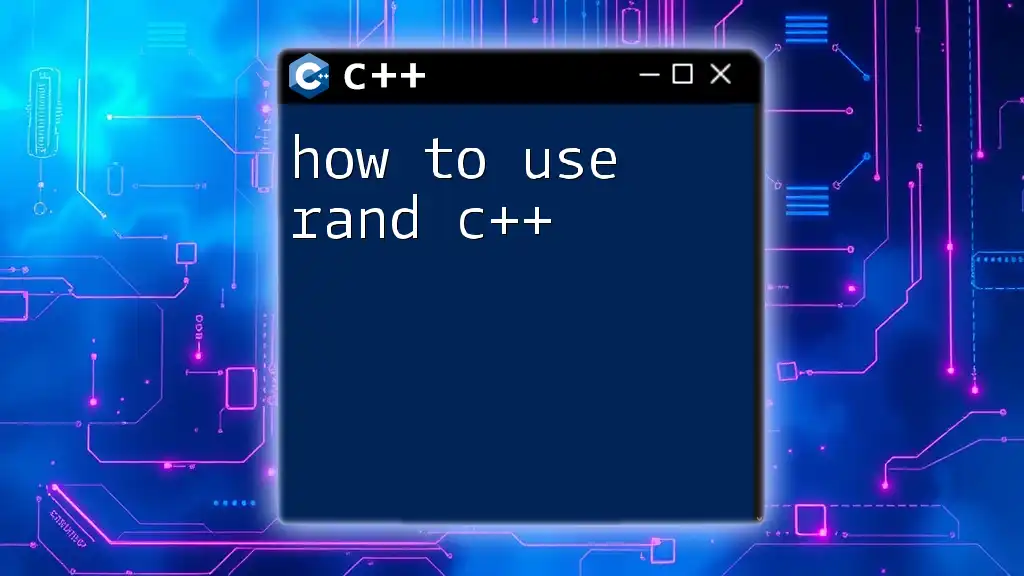
Using Boolean in Conditional Statements
Using Booleans in `if` Statements
The `if` statement is one of the most common uses of Boolean values. Here’s a basic structure:
if (isActive) {
// Execute code if isActive is true
}
In this example, the code within the braces will execute only if `isActive` is `true`. It is vital to put your conditions within parentheses for proper evaluation.
Using Booleans in `while` and `for` Loops
Boolean values can also control loops. For example, a `while` loop will continue executing as long as the condition is `true`:
while (isRaining) {
// Perform action while it's raining
}
This loop will continue until `isRaining` becomes `false`. Using Booleans in control structures like this allows for dynamic execution of code based on runtime conditions.
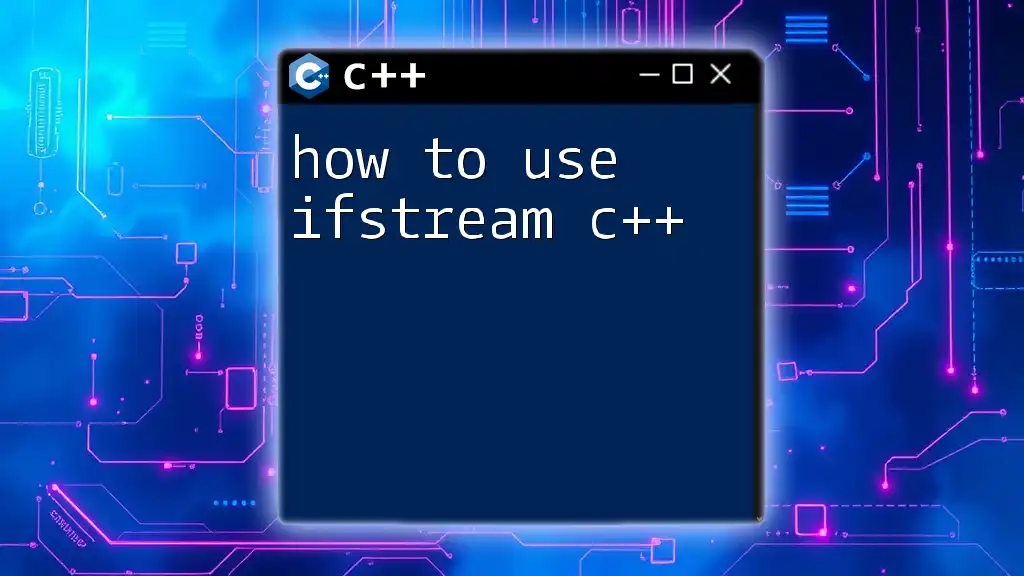
Logical Operators with Boolean in C++
Overview of Logical Operators
C++ provides several logical operators that work with Boolean values:
- AND (`&&`): Returns `true` if both operands are `true`
- OR (`||`): Returns `true` if at least one operand is `true`
- NOT (`!`): Reverses the Boolean value
Using Logical Operators
AND Operator Example
The AND operator can be used like this:
bool isSunny = false;
if (isActive && isSunny) {
// Code executes only if both are true
}
The block will execute only if both `isActive` and `isSunny` are `true`.
OR Operator Example
The OR operator looks like this:
if (isActive || isSunny) {
// Code executes if at least one is true
}
This means that the code block will run if either `isActive` or `isSunny` is `true`.
NOT Operator Example
Using the NOT operator can simplify logic:
if (!isRaining) {
// Code executes if it's not raining
}
In this case, the condition evaluates to `true` if `isRaining` is `false`.
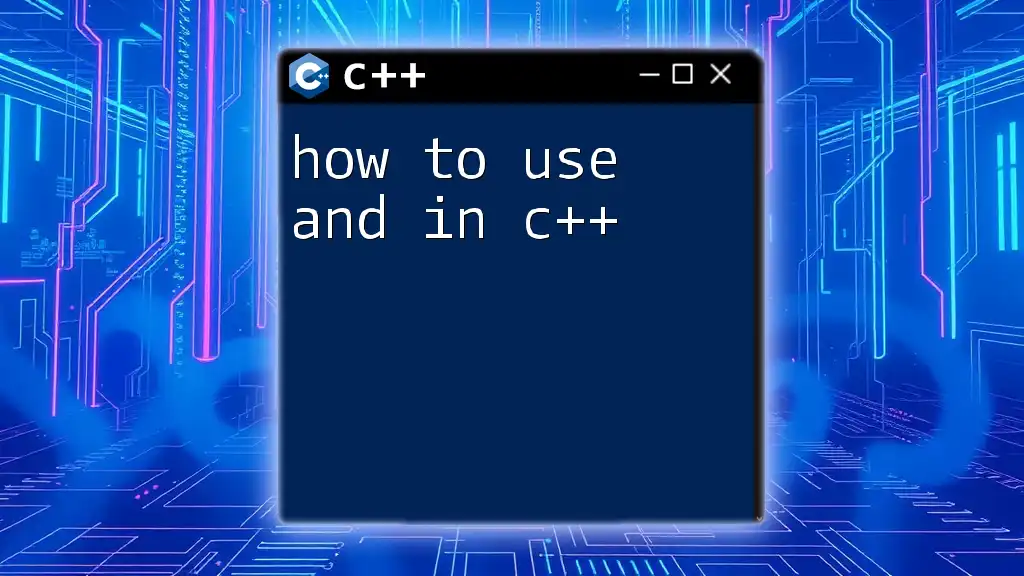
Boolean Expressions and Their Evaluation
Constructing Boolean Expressions
Creating Boolean expressions allows for complex conditions. For example:
bool canDrive = (age >= 16) && (hasLicense);
Here, the expression evaluates whether `age` is 16 or older and whether `hasLicense` is true. Proper structuring of Boolean expressions can streamline your decision-making processes in C++.
Short-Circuit Evaluation
C++ utilizes short-circuit evaluation in logical operations. This means that if the result can be determined from the first condition, the second condition is not evaluated. Such behavior can improve performance, especially in computationally expensive conditions:
if (isReady && !isFinished) {
// Code executes based on short-circuit evaluation
}
In this example, if `isReady` is `false`, `!isFinished` is never checked, which can save computation time.
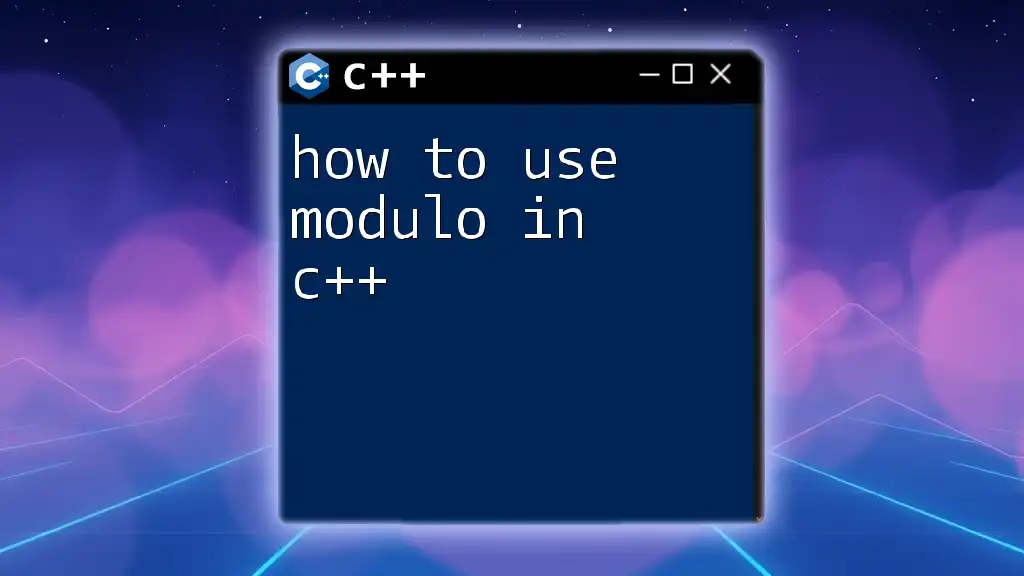
Common Mistakes with Booleans in C++
Misunderstanding Truthy and Falsy Values
A common pitfall is misinterpreting values as Boolean. In C++, any non-zero value is considered true, and zero is false.
bool isValid = (5); // This is true in C++
Here, `isValid` ends up being `true`. Understanding these nuances is essential in working with Boolean logic.
Confusion Between `==` and `=` Operators
New programmers often confuse the assignment operator (`=`) with the equality operator (`==`). The following code represents an error:
bool status;
if (status = true) { // Incorrect usage (assignment)
}
In this case, the condition always evaluates to `true`, as `status` is assigned `true` rather than being checked. Always remember to use `==` for comparisons.
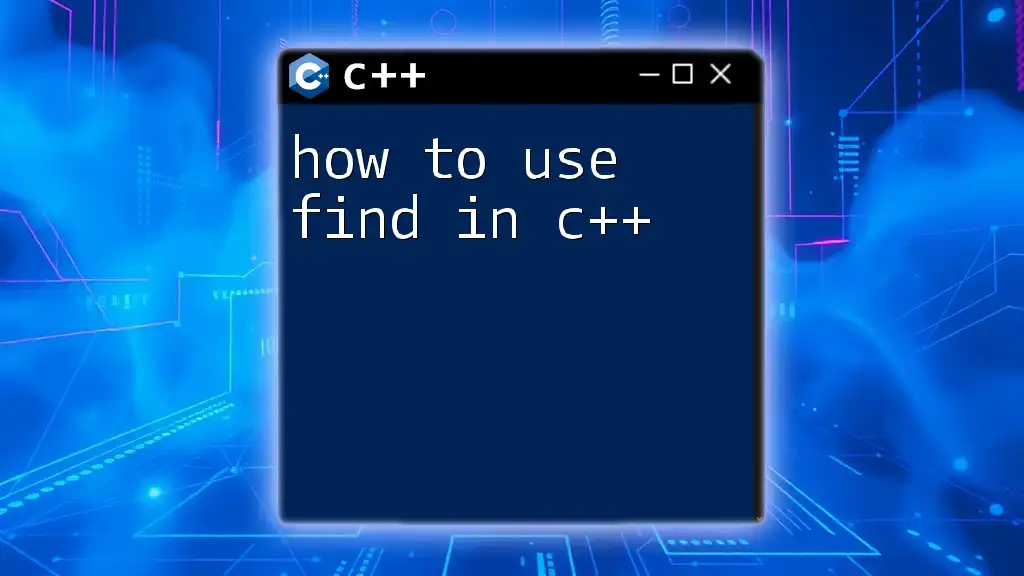
Best Practices for Using Boolean in C++
Keep Boolean Expressions Simple
Simplicity is key when working with Boolean expressions. Clear and concise conditions enhance the readability of your code, allowing for easier debugging and maintenance.
Use Descriptive Names for Boolean Variables
Naming conventions play a crucial role in code clarity. Use descriptive names for your Boolean variables. For example, `isSunny` is far more informative than just `x`. Follow good practices to ensure that your code remains understandable.
Avoid Negative Logic When Possible
Using positive logic is generally easier to read and understand:
if (isActive) { // Positive is clearer
// Do something
}
Instead of using negative logic:
if (!isInactive) { // Less clear
// Do something
}
Positive conditions foster better readability and intuitiveness in your program’s logic.
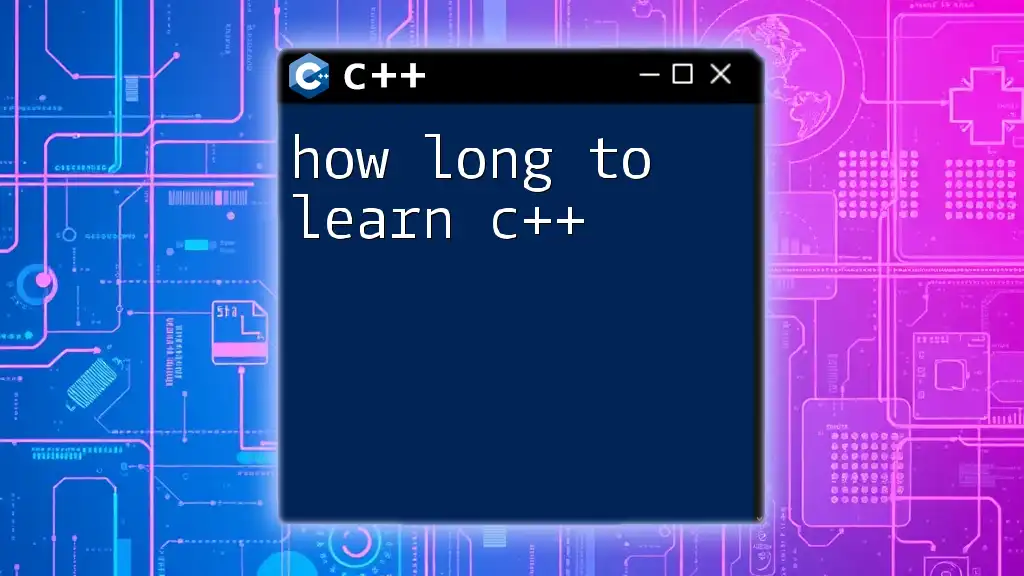
Conclusion
Understanding how to use Boolean values in C++ is pivotal for creating effective control flow in your programs. By implementing conditional statements and logical operators, you can make informed decisions based on your program’s state. Practice constructing Boolean expressions and maintaining clarity in your code with descriptive naming and straightforward logic. The more you incorporate these principles into your coding practice, the more efficient and robust your programs will become.
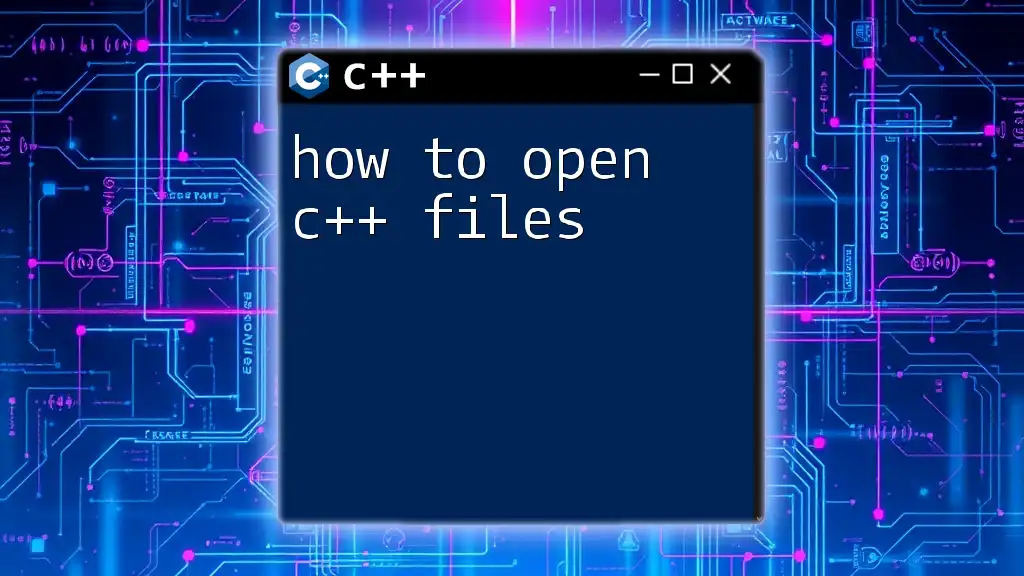
Additional Resources
To further enhance your knowledge on the subject, consider exploring additional reading materials, tutorials, and exercises focused on Boolean logic and conditions in C++. Engaging with professional C++ communities can also provide valuable insights and support.