In C++, the `find` function from the `<algorithm>` library is used to search for a specific element within a range, returning an iterator to the first occurrence or the end iterator if not found.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = std::find(vec.begin(), vec.end(), 3);
if (it != vec.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found." << std::endl;
}
return 0;
}
What is the Find Function in C++?
The find function is a powerful tool provided by the C++ Standard Template Library (STL) that allows developers to efficiently search for the first occurrence of a given value in a range of elements. Understanding how to use the find function in C++ can significantly improve your ability to manipulate collections of data and perform searches in a variety of data structures.
Overview of C++ STL (Standard Template Library)
The STL is a collection of template classes and functions that provide general-purpose tools for data manipulation. The find function is a part of the `<algorithm>` header in the STL and offers a simple and efficient way to search through containers like arrays, vectors, lists, and more.
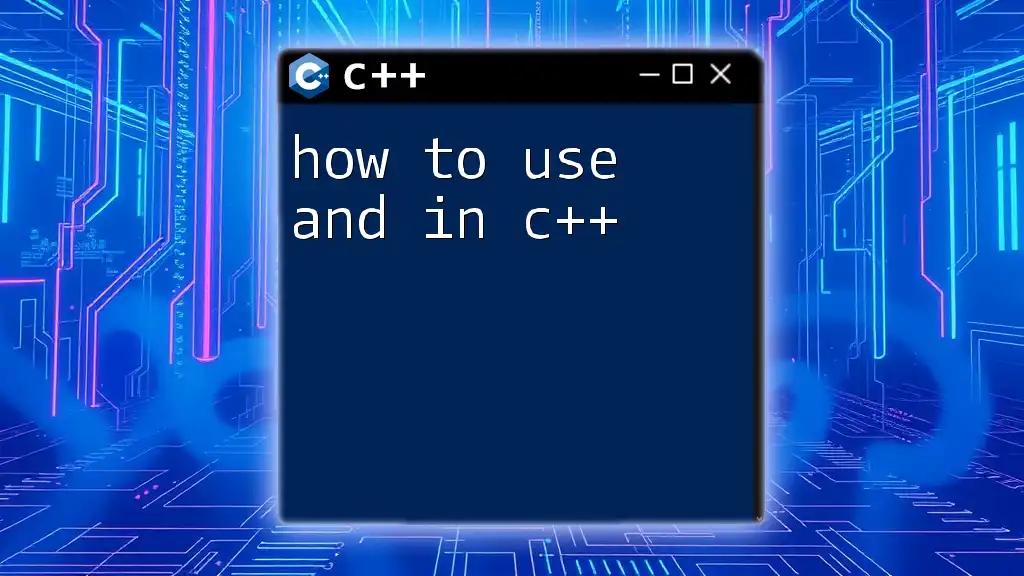
Understanding the Syntax of the Find Function
Basic Syntax of the Find Function
The basic syntax for the find function is as follows:
auto find(InputIterator first, InputIterator last, const T& val);
- InputIterator first: The beginning of the range to search.
- InputIterator last: The end of the range to search.
- const T& val: The value to search for.
The function returns an iterator pointing to the first occurrence of the value if found; otherwise, it returns the iterator equal to the end of the range.
Syntax Breakdown
Let’s examine this concept through an example:
#include <algorithm>
#include <vector>
std::vector<int> myVector = {1, 2, 3, 4, 5};
auto it = std::find(myVector.begin(), myVector.end(), 3);
In this snippet, we create a `vector` of integers and use `std::find()` to search for the value `3`. The function will return an iterator to the element if it exists or `myVector.end()` if it does not.
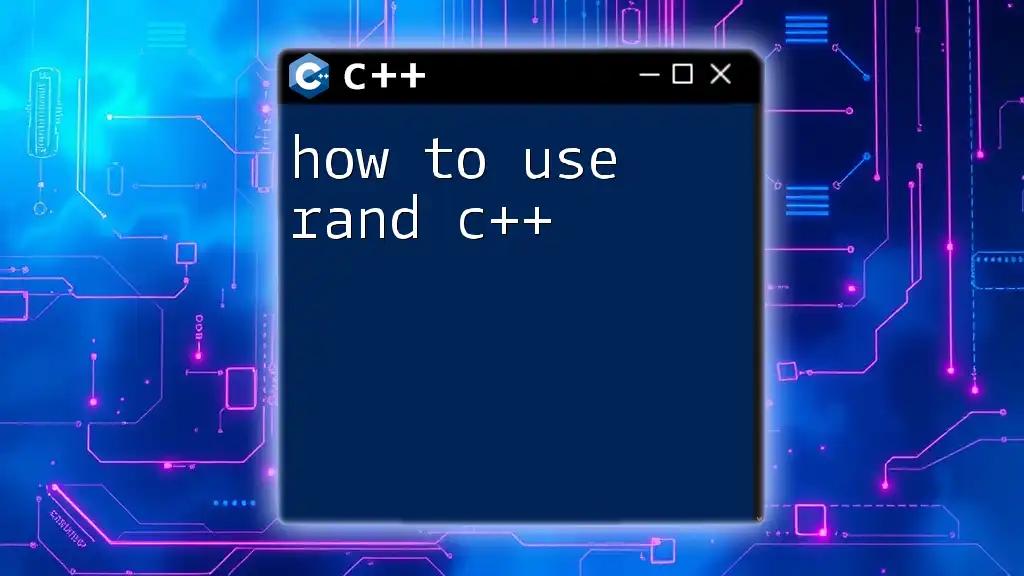
Step-by-Step Guide on Using Find in C++
Finding an Element in an Array
To demonstrate how to use find in C++, let's look at finding an element in a simple array:
#include <iostream>
#include <algorithm>
int arr[] = { 10, 20, 30, 40, 50 };
auto result = std::find(std::begin(arr), std::end(arr), 30);
if (result != std::end(arr)) {
std::cout << "Element found: " << *result << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
In this code, we search for the value `30` in the array. If found, the output will indicate that the element has been located. If not, it will indicate that the element is absent.
Finding an Element in a Vector
Vectors are dynamic arrays, and finding an element within a vector works similarly:
#include <iostream>
#include <vector>
#include <algorithm>
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = std::find(numbers.begin(), numbers.end(), 4);
if (it != numbers.end()) {
std::cout << "Found: " << *it << std::endl;
}
In this example, we are using `std::find()` to locate the integer `4`. Again, if this integer is found, it will be printed; if not, no output occurs.
Case-Sensitive and Case-Insensitive Search
When dealing with strings or characters, case sensitivity can be a consideration. The find function performs case-sensitive searches by default:
#include <iostream>
#include <string>
#include <algorithm>
std::string str = "Hello World!";
auto it = std::find(str.begin(), str.end(), 'H');
if (it != str.end()) {
std::cout << "Found: " << *it << std::endl;
}
Here, we're searching for the character 'H'. If the search were to include a lowercase 'h', the function would return not found because it’s case-sensitive.
To handle case-insensitive searches, one would typically need to implement a custom comparison, possibly through the use of `std::find_if()` with a lambda function that performs case normalization.
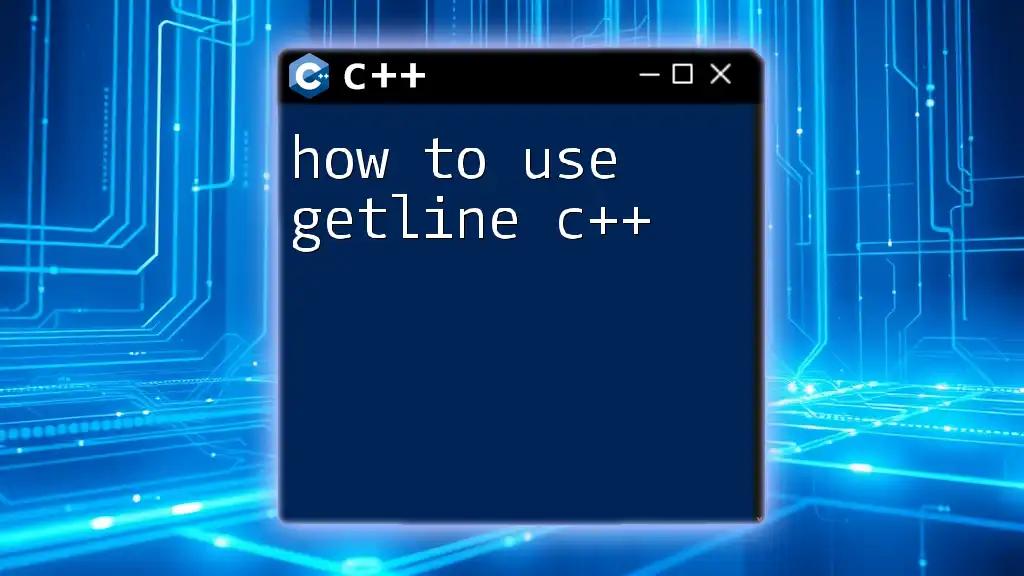
Advanced Usage of the Find Function
Custom Objects with Find Function
The find function can also be applied to collections of custom objects. Here’s how you can achieve this:
#include <iostream>
#include <vector>
#include <algorithm>
class Person {
public:
Person(std::string n) : name(n) {}
std::string name;
};
int main() {
std::vector<Person> people = { Person("Alice"), Person("Bob") };
auto it = std::find_if(people.begin(), people.end(),
[](const Person& p) { return p.name == "Alice"; });
if (it != people.end()) {
std::cout << "Found person: " << it->name << std::endl;
}
}
In this example, we utilize `std::find_if()`, allowing us to specify a custom condition for our search using a lambda function. This is especially useful when dealing with complex types.
Using Find in Multi-Dimensional Containers
When searching through multi-dimensional containers like a 2D vector, it may require a slightly altered approach:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<std::vector<int>> matrix = {{1, 2, 3}, {4, 5, 6}};
auto it = std::find_if(matrix[0].begin(), matrix[0].end(), [](int n) { return n == 2; });
if (it != matrix[0].end()) {
std::cout << "Found: " << *it << std::endl;
}
}
In the above code, we are looking for the number `2` in the first row of a 2D vector. Similarly, we could loop through the rows to search through all entries.
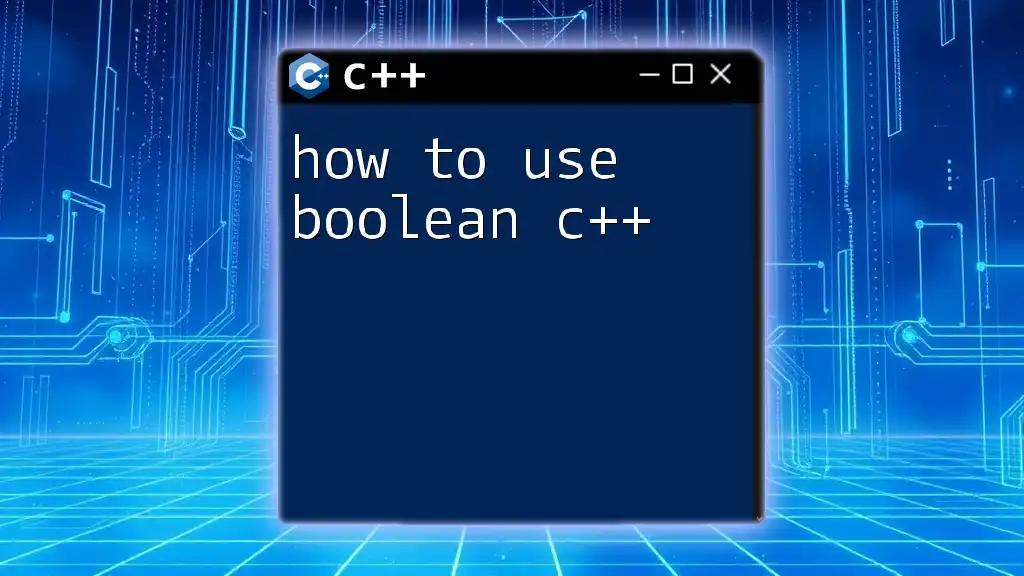
Best Practices for Using Find in C++
Performance Considerations
One significant consideration is the performance of the find function. The function has a time complexity of O(n), where n is the number of elements in the range. This means that as the size of your collection increases, the time it takes to find an element can become considerable. If you frequently need to search for items, consider alternative data structures like hash maps, which can provide average constant-time complexity for search operations.
Avoiding Common Pitfalls
When using the find function, there are a few common pitfalls to watch out for:
- Incorrect Range: Make sure the range specified does not go out of bounds. For example, trying to find an element in a vector while inadvertently referencing the end iterator can cause issues. Here’s an example of improper usage:
// Here’s an example of common pitfalls and how to avoid them
int incorrectUsage() {
const int arr[] = {1, 2, 3, 4};
auto found = std::find(arr, arr + 5, 3); // out-of-bounds issue
}
In this case, `arr + 5` goes beyond the array limit and can lead to undefined behavior.
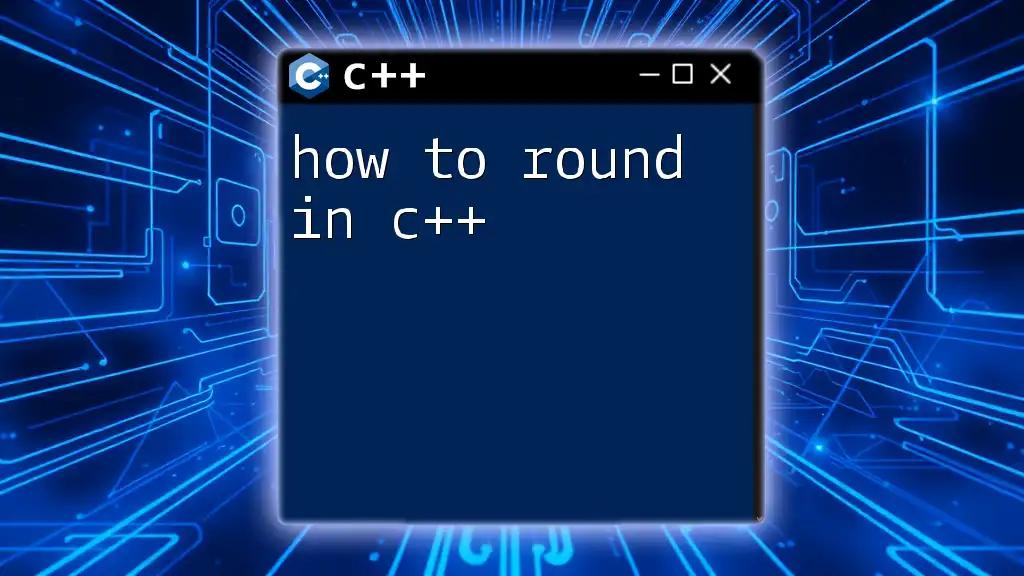
Conclusion
Recap of Using the Find Function
In summary, understanding how to use find in C++ is a fundamental skill for any programmer. By grasping this function's syntax and application, you gain the ability to search through a variety of data structures efficiently.
Further Learning Resources
For those interested in deepening their understanding of the C++ STL and the find function, there are many resources available, including books, online courses, and tutorials. Engaging with more complex examples and scenarios will further enhance your programming skills.
Call-to-Action
I encourage you to practice with the find function, experiment with different data structures, and explore advanced features. Consider taking part in workshops or courses provided by my company to expand your knowledge and skills in C++.