In C++, the `getline` function is used to read an entire line from an input stream into a string variable, effectively capturing all characters until a newline is encountered.
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter a line of text: ";
std::getline(std::cin, line);
std::cout << "You entered: " << line << std::endl;
return 0;
}
Understanding getline
What is getline?
The `getline` function in C++ is designed to read an entire line of text from an input stream into a string variable. Unlike other input functions like `cin`, which terminate their reading at whitespace (like spaces and tabs), `getline` captures all characters until a newline character is encountered. This makes it valuable for capturing full sentences or phrases that may contain spaces.
Syntax of getline
The fundamental syntax of `getline` is straightforward:
getline(input_stream, string_variable);
- input_stream: This indicates where the input will be read from, such as the console (`std::cin`) or a file input stream.
- string_variable: This variable will store the line of text read from the input stream.
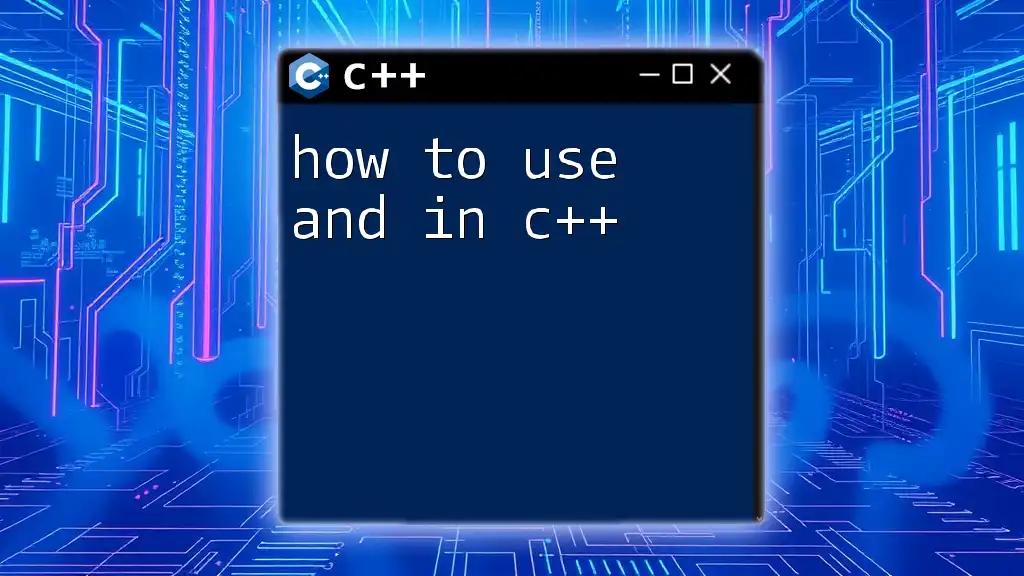
The Purpose of getline
Reading Entire Lines
The main advantage of using `getline` is its capability to read an entire line of input, including spaces. For example, if a user enters "Hello World!", `getline` will capture exactly that, while `cin` would stop reading at the first space, returning only "Hello".
Common Use Cases
- Interactive Console Applications: When you need to take user input that may contain spaces, `getline` is the function of choice.
- File I/O: When reading data from files line by line, `getline` conveniently handles each line individually.
- Multi-word String Inputs: In scenarios where users may input multi-word strings (like names or addresses), `getline` will ensure that the entire entry is captured.
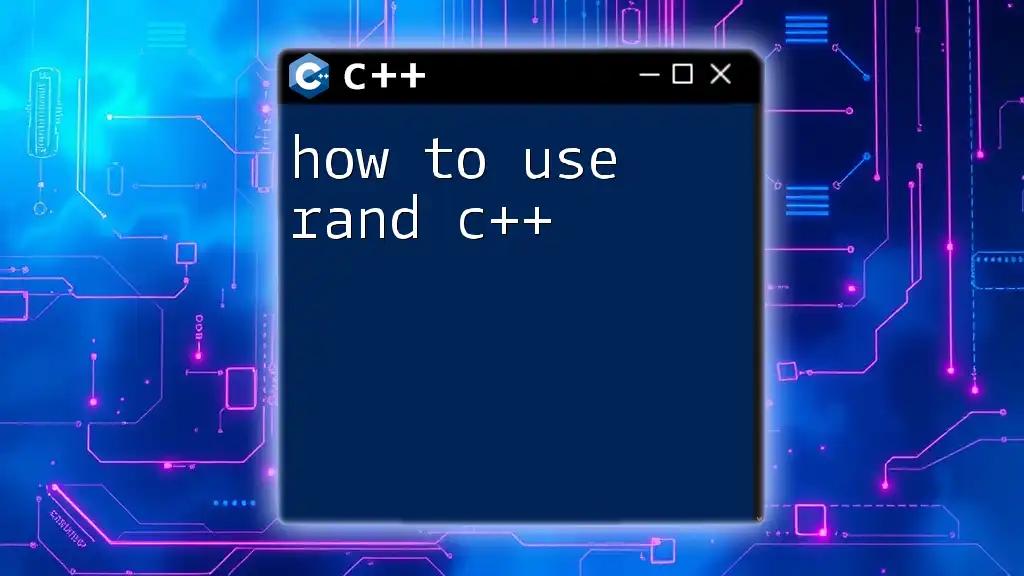
Implementing getline in Your Code
Using getline with cin
Example 1: Basic Usage
Here is a simple example of using `getline` with `std::cin`:
#include <iostream>
#include <string>
int main() {
std::string myString;
std::cout << "Enter a line of text: ";
std::getline(std::cin, myString);
std::cout << "You entered: " << myString << std::endl;
return 0;
}
In this code:
- The program prompts the user for a line of text.
- The `getline` function captures the entire line input by the user and stores it in the `myString` variable.
- Finally, it outputs the captured input, demonstrating how effectively `getline` handles the input.
Using getline with File Streams
Example 2: Reading from a File
If you want to read text from a file, `getline` can be used as shown in the following example:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt");
std::string line;
if (inputFile.is_open()) {
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
} else {
std::cout << "Unable to open file";
}
return 0;
}
In this example:
- The code opens a file named "example.txt".
- The program uses a while loop to read lines from the file until the end (EOF) is reached.
- Each line is output to the console.
- If the file cannot be opened, a relevant error message is printed.
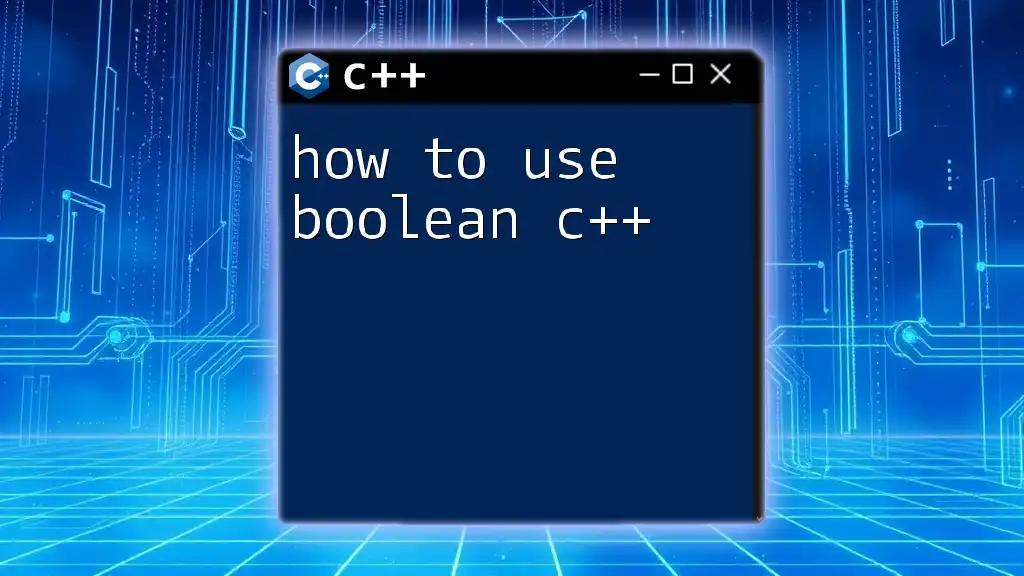
Advanced Usage of getline
Specifying Delimiters
Although `getline` by default uses the newline character as a delimiter, you can also specify a custom delimiter. This is useful for parsing specific formats. For example:
std::getline(std::cin, myString, ':'); // Reads until a colon is encountered
This approach allows you to use a different character to denote the end of the input, making `getline` versatile for various parsing needs.
Handling Special Cases
Empty Lines
When the user provides an empty line as input, `getline` will simply store an empty string in the variable. This enables developers to handle inputs conditionally based on whether something was entered.
Input Validation
After using `getline`, you may need to conduct validation checks on the user input. For instance, you could check if the string is empty or ensure that certain conditions are met (like not exceeding a specific length).
Combining getline with Other Functions
Another powerful feature of `getline` is its ability to work seamlessly with other string manipulation functions. Consider the following example:
std::string name;
std::cout << "Enter your full name: ";
std::getline(std::cin, name);
std::string firstName = name.substr(0, name.find(' '));
std::cout << "First Name: " << firstName << std::endl;
In this snippet:
- The program captures an entire name from the user.
- It then extracts the first name using the `substr` method and `find` to locate the first space.
- This demonstrates how you can leverage `getline` to simplify input handling and string processing in C++.
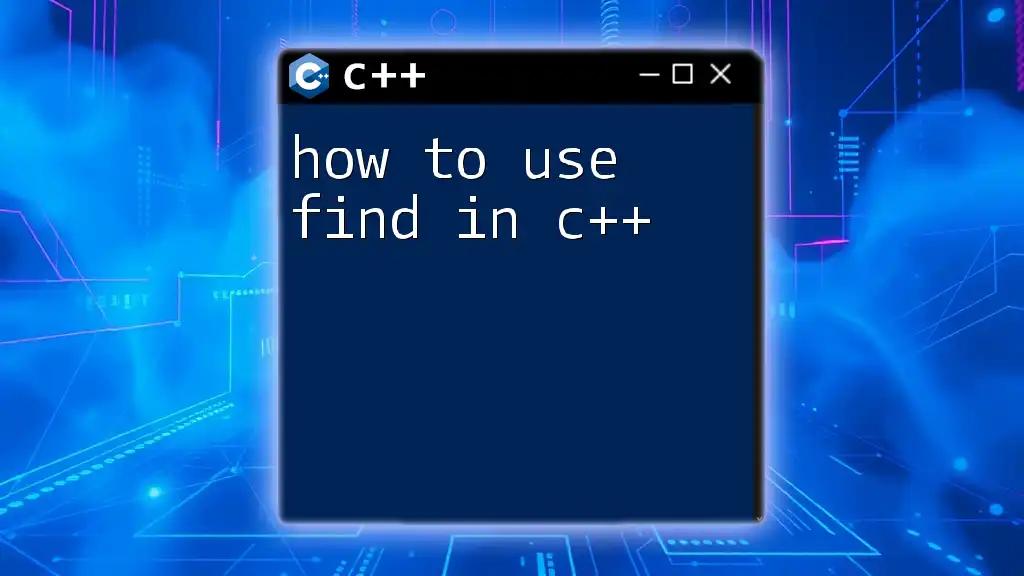
Common Mistakes and Troubleshooting
Forgetting to Include Headers
Always remember to include the necessary headers: `<string>` for the string class and `<iostream>` or `<fstream>` for input and output. Neglecting to do so will lead to compilation errors.
Mixing getline with cin
A common pitfall is mixing `getline` with `cin` without understanding how they interact. For instance, if you use `cin` to read an integer followed by `getline`, the newline character left in the input buffer can cause `getline` to read an empty string. To avoid this, ensure you consume remaining newline characters before calling `getline`, like this:
int myInt;
std::cin >> myInt;
std::cin.ignore(); // This clears the newline left in the buffer
std::string myString;
std::getline(std::cin, myString);

Conclusion
By utilizing `getline`, you can effectively manage user input, read entire lines, and customize input handling in your C++ applications. This function enhances your program's ability to handle various input scenarios gracefully.
Experiment with different use cases of `getline` in your projects and notice how it simplifies managing strings in C++. This function is an essential part of your C++ toolkit, making it easier to create interactive and user-friendly applications.