In C++, comments can be added using either single-line comments with `//` or multi-line comments enclosed within `/* */` to improve code readability without affecting execution.
Here’s a code snippet demonstrating both types of comments:
#include <iostream>
// This is a single-line comment explaining the following function
void greet() {
std::cout << "Hello, World!" << std::endl; /* This is a multi-line comment explaining the print statement */
}
int main() {
greet();
return 0;
}
Types of Comments in C++
Single-Line Comments
Single-line comments are a simple and effective way to annotate your code. These comments start with `//` and continue until the end of the line. They are useful for brief explanations or notes.
Example:
// This function adds two integers
int add(int a, int b) {
return a + b; // Returning the sum
}
In this example, the comment helps clarify the purpose of the function and the action of returning the sum. Use single-line comments judiciously for quick notes that don’t require extensive explanation.
Multi-Line Comments
In contrast, multi-line comments are used when you need to write longer annotations. They begin with `/` and end with `/`, allowing you to span multiple lines without breaking the comment.
Example:
/*
This function multiplies two integers
and returns the product.
*/
int multiply(int a, int b) {
return a * b;
}
This style can be particularly helpful for documenting complex logic or providing detailed descriptions that would overwhelm a single line.
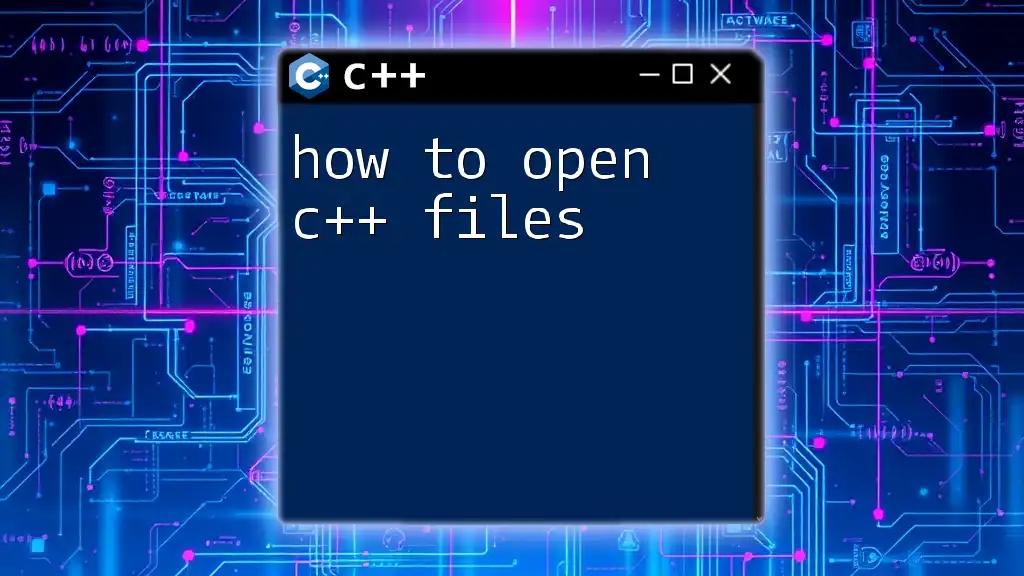
Best Practices for Commenting in C++
Clarity and Conciseness
When commenting, it’s vital to keep the commentary clear and concise. Avoid overly complex language that might confuse readers; instead, opt for straightforward terminology. For instance, explain the purpose of a function without getting lost in technical jargon that might not be universally understood.
Avoiding Redundancy
One common pitfall is the tendency to add comments that simply restate what the code does. This not only clutters the code but can also mislead developers into thinking they need to read every comment as part of the coding logic.
Example of Redundancy:
// This is an int variable
int number; // Avoid this type of comment
Instead of repeating what’s already evident from the code, focus on the why of your code. Explain decisions and challenges that lead to particular implementations rather than trivial details already clear from the context.
Using Comments to Explain “Why”
To maximize the value of comments, emphasize the reason behind the code rather than just the functionality. This practice enriches the understanding and serves as valuable guidance for those who will work with the code later.
Example:
// Using bubble sort here for demonstration purposes
// Although it is not the most efficient for large datasets
void bubbleSort(vector<int>& arr) {
// Sorting logic...
}
Here, the comments address the specific choice of algorithm, providing context that enhances understanding for future developers.

How to Comment C++ Code Effectively
Utilizing Documentation Comments
Documentation comments are particularly useful for generating automatic documentation. These comments often follow specific formatting rules, which allow tools like Doxygen to compile them into comprehensive documentation automatically.
Example:
/**
* @brief Adds two integers.
* @param a First integer
* @param b Second integer
* @return Sum of a and b
*/
int add(int a, int b) {
return a + b;
}
The structured format not only helps provide clarity but also fosters reusable documentation practices across different projects, greatly enhancing your development process.
Grouping FAQs as Comments
In larger projects, it’s beneficial to include FAQs as comments. This can preemptively address common queries that teammates may have, promoting better collaboration and understanding.
Example:
/*
FAQ:
Q: What is the limit of the integer size?
A: Depending on the compiler, it can be 32 or 64 bits.
*/
Such comments can act as a quick reference, helping to maintain transparency and ensuring that everyone is on the same page concerning crucial information.
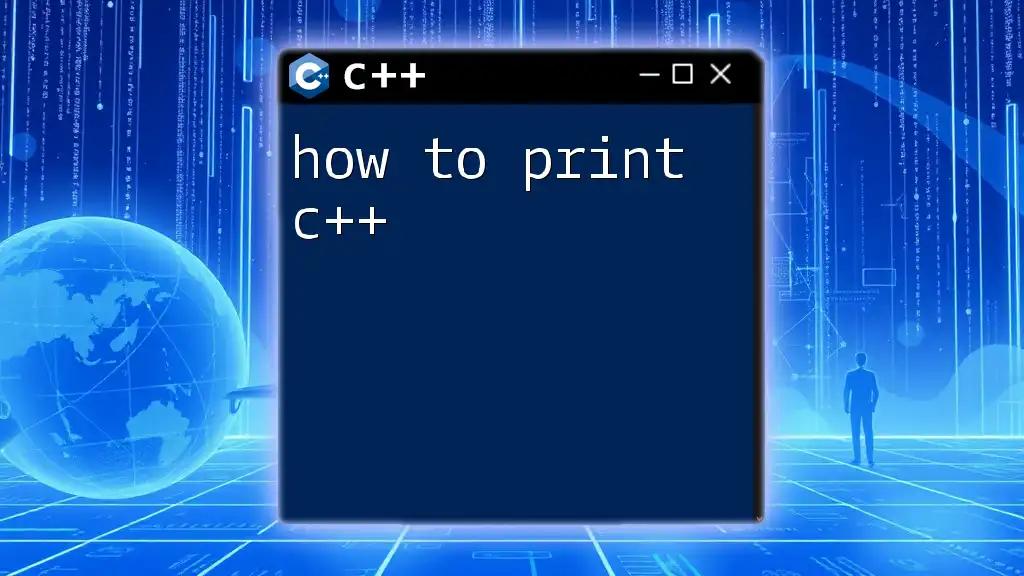
Commenting in the Context of Collaborative Work
Importance of Comments in Team Settings
In collaborative environments, comments become essential communication tools. Effective comments serve to bridge the understanding gaps between different team members, allowing for smoother transitions when someone new starts working on existing code.
Real-life projects often involve multiple developers contributing to the same codebase. Without adequate commenting, misunderstandings can lead to costly mistakes or rework. Clear and insightful comments reassure your teammates and facilitate better collaboration.

Tools for Comment Management
Using Version Control Systems
Version control systems (VCS) such as Git offer a powerful way to manage comment history alongside your code. Comments in a VCS can help track why changes were made over time. Properly documented commit messages accompany each version change and enable developers to look back at changes within the context they were made.
IDE Features
Leveraging the built-in features of Integrated Development Environments (IDEs) can greatly simplify the process of commenting. Many IDEs provide keyboard shortcuts for adding comments and navigating through comments easily. Tools like Visual Studio, JetBrains CLion, and Eclipse often come with functionalities that can streamline your commenting practice.
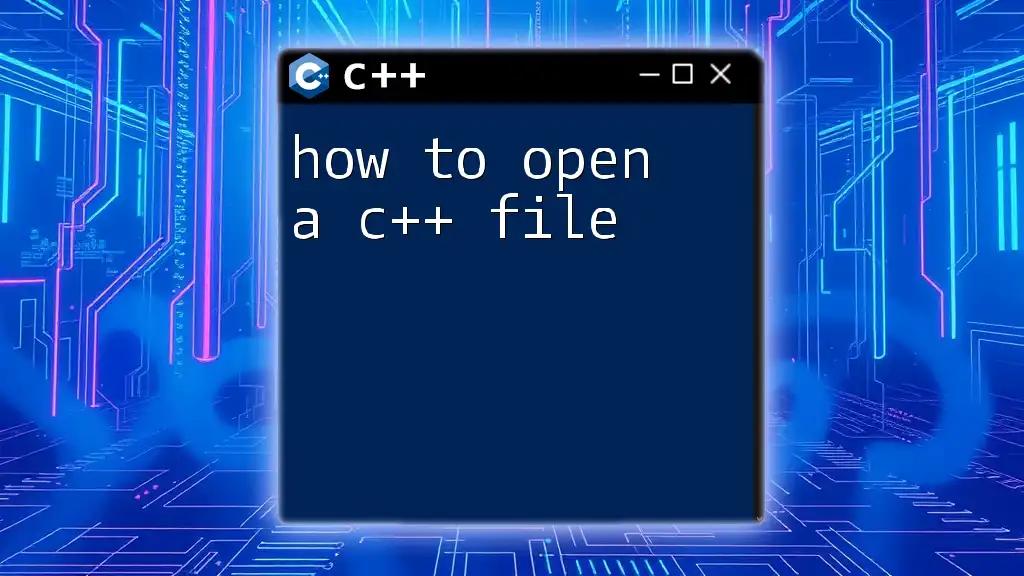
Conclusion: The Art of Commenting C++
Commenting is an art that blends technical skill with effective communication. A well-commented codebase leads not only to improved readability but also to better collaboration in team settings. Mastering how to comment C++ will enhance your programming skills and make your codebase a valuable asset for everyone involved.
Encouraging oneself to frequently practice and adapt one's commenting style to suit different projects can facilitate continuous improvement. As you refine your commenting technique, consider sharing your experiences and best practices with others, fostering a community of eager learners and developers.