In C++, casting is the process of converting one data type into another using specific operators such as `static_cast`, `dynamic_cast`, `const_cast`, or `reinterpret_cast`.
Here's an example of how to use `static_cast` to convert a `double` to an `int`:
#include <iostream>
int main() {
double num = 5.7;
int castedNum = static_cast<int>(num); // Cast double to int
std::cout << "Casted number: " << castedNum << std::endl; // Output: Casted number: 5
return 0;
}
Understanding Data Types in C++
Before diving into the intricacies of casting in C++, it's essential to understand the different data types that the language provides. C++ offers a variety of built-in data types, including integers, floats, doubles, and characters, as well as user-defined types such as structs and classes.
Type safety is crucial in C++, as it prevents errors that can arise from incompatible data type operations. Ensuring that variables hold the correct type is a fundamental aspect of writing robust C++ programs.
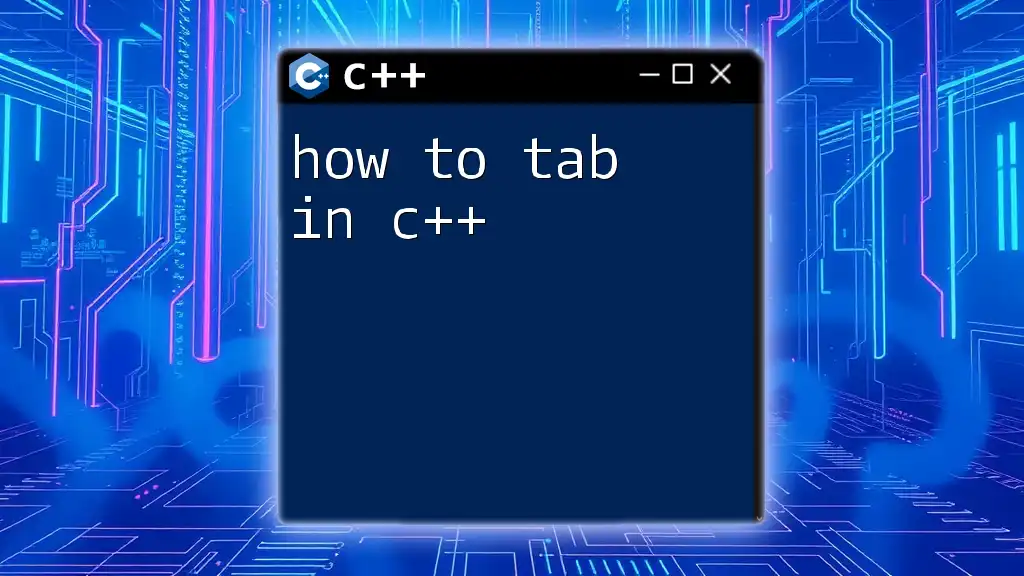
Types of Casting in C++
Implicit Casting
Implicit casting, also known as automatic conversion, occurs when the compiler automatically converts one data type to another without requiring any additional code. This typically happens when converting from a smaller data type to a larger one, where data loss is not a concern.
For example, if you assign an integer value to a double variable, the compiler handles the conversion seamlessly.
int intValue = 10;
double doubleValue = intValue; // Implicit casting
In this snippet, `intValue` is converted to a `double` automatically, ensuring precision is maintained.
Explicit Casting
Explicit casting requires the developer to specify the desired conversion, enabling greater control over the process. This is essential in scenarios where the conversion might lead to data loss or when dealing with incompatible types.
A common method for explicit casting is `static_cast`. This operator is used for conversions where the types are related, such as upcasting or downcasting in class hierarchies.
double doubleValue = 9.89;
int intValue = static_cast<int>(doubleValue); // Explicit casting with static_cast
In this example, using `static_cast` explicitly converts the `double` value to an `int`, truncating the decimal part. It's crucial to choose explicit casting when there's a risk of losing relevant data.
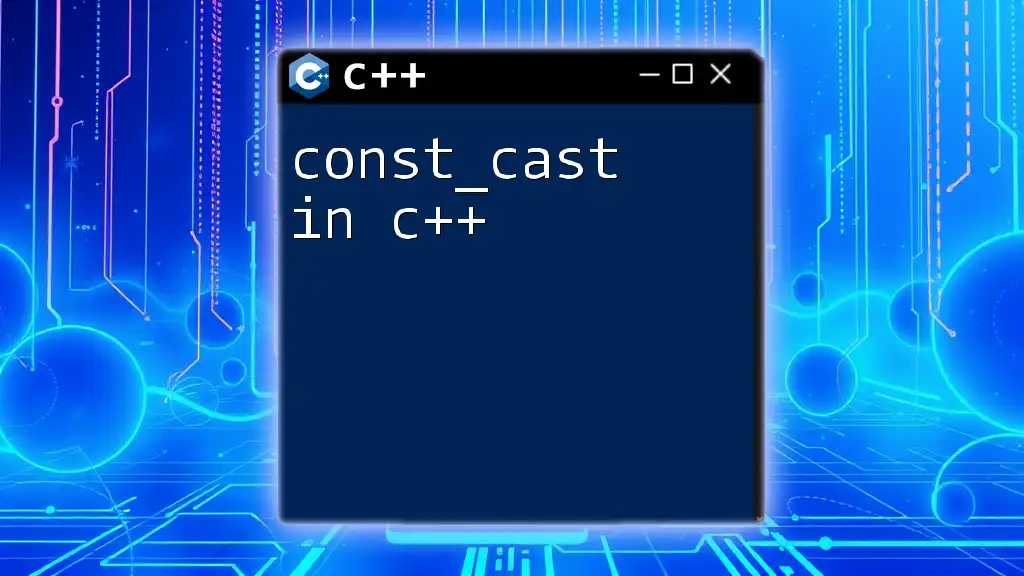
The Different Cast Operators
static_cast
The `static_cast` operator is versatile and commonly used for safe type conversions. It can convert between different numeric types, arrays, references, and pointers in a class hierarchy, provided that the conversion is logically valid.
For instance, if you have a base class pointer and want to cast it to a derived class, `static_cast` can facilitate this.
class Base {};
class Derived : public Base {};
Base* basePtr = new Derived();
Derived* derivedPtr = static_cast<Derived*>(basePtr); // Safely cast to Derived
When to use `static_cast`: Use `static_cast` when you need to perform a conversion that is guaranteed to be valid by the existing hierarchy of types.
dynamic_cast
`dynamic_cast` is designed to ensure safe downcasting, especially when polymorphism is involved. It is particularly useful when working with base class pointers or references pointing to derived class objects. Using `dynamic_cast` allows you to safely verify the type at runtime.
class Base {
virtual void foo() {}
};
class Derived : public Base {};
Base* basePtr = new Derived();
Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Safe downcast
if (derivedPtr) {
// Successfully downcasted, derivedPtr can be used
} else {
// Downcasting failed
}
In the example above, `dynamic_cast` checks if the `basePtr` truly points to an instance of `Derived`. If the cast fails, `derivedPtr` will be `nullptr`, making it essential to handle such failures gracefully.
const_cast
The `const_cast` operator allows you to add or remove the `const` qualifier from a variable. This is particularly useful in scenarios where a function prototypes point to const data but you need to modify it.
const int constantValue = 20;
int* modifiableValue = const_cast<int*>(&constantValue);
While the above code will allow you to theoretically modify `constantValue` through `modifiableValue`, be cautious: altering a `const` variable can lead to undefined behavior if the original variable is actually `const`.
reinterpret_cast
The `reinterpret_cast` operator facilitates low-level casts, allowing you to convert any pointer type to any other pointer type. It is useful in situations where you need to handle memory directly.
int* intPtr = new int(5);
char* charPtr = reinterpret_cast<char*>(intPtr); // Pointer type change
Important: Exercise caution when using `reinterpret_cast`, as it bypasses type safety and can lead to dangerous manipulation if not handled properly.
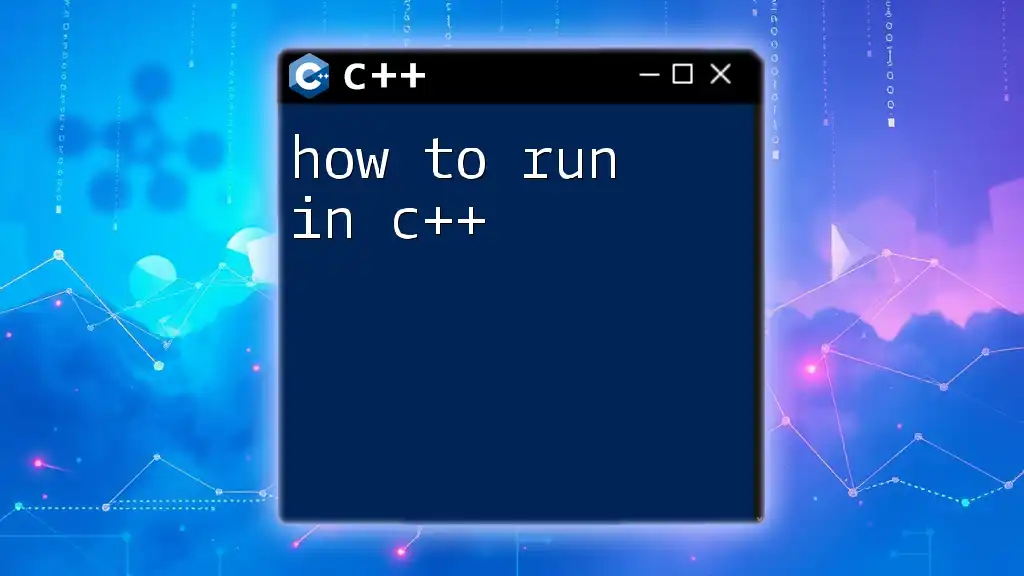
Best Practices and Performance Considerations
Maintaining type safety enhances the readability and maintainability of your code. Avoid unnecessary casts, and only use them when required. This practice not only prevents bugs but also improves performance.
When considering performance, prefer `static_cast` and `dynamic_cast` for safer type conversions. Avoid heavy reliance on `reinterpret_cast` unless absolutely necessary, as it can create complex code that is difficult to debug.
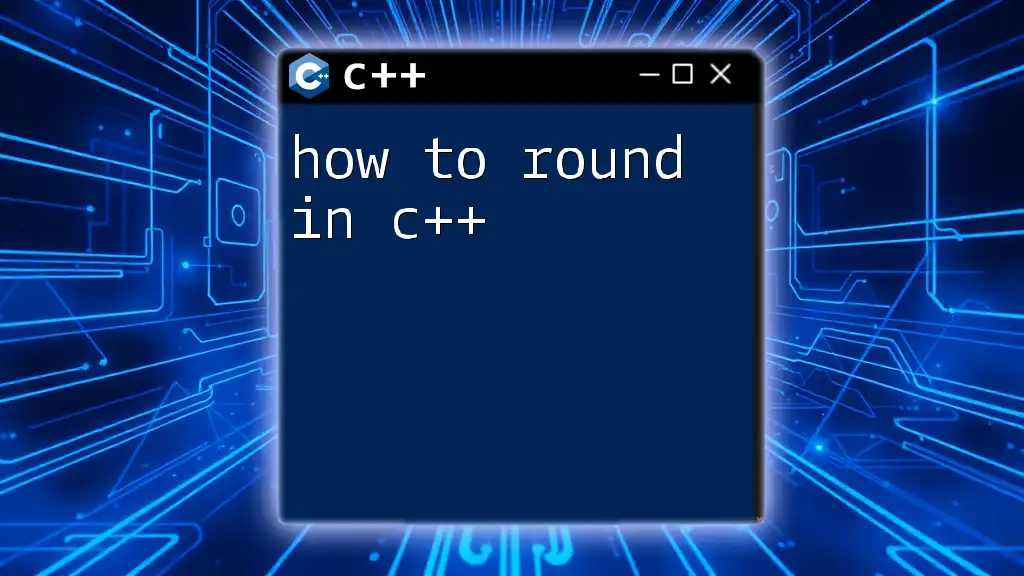
Debugging Casting Errors in C++
Casting errors can be subtle and challenging to identify. Common mistakes include failing to check the validity of a dynamic cast and using the wrong cast operator for the situation.
To effectively debug, you can employ tools such as Valgrind or address sanitizers, which help in detecting invalid memory accesses and other issues. Always include error handling for dynamic casts to manage failures safely and provide informative messages for better diagnostics.
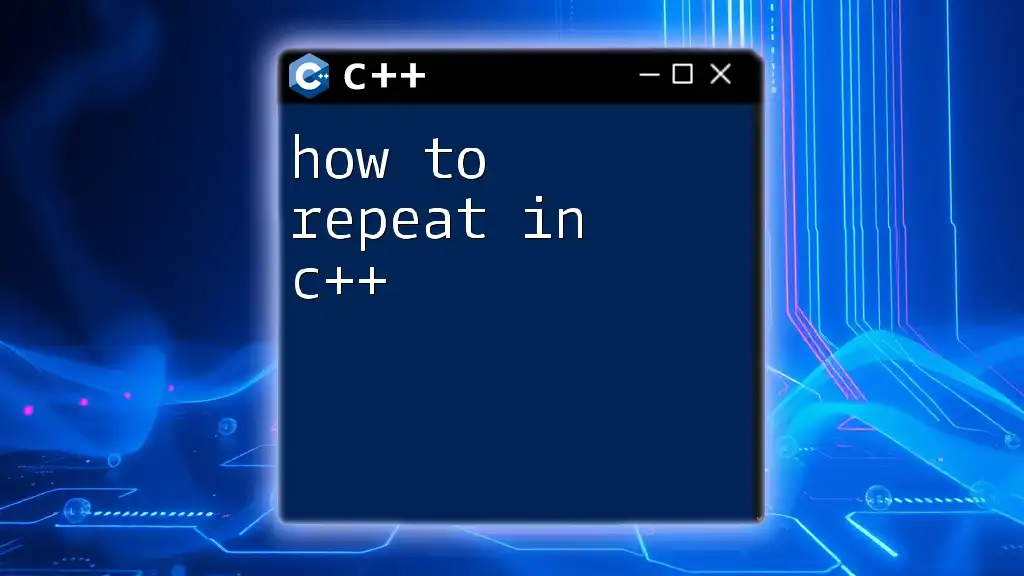
Conclusion
Mastering how to cast in C++ is essential for developers, enabling them to write robust and maintainable code. From understanding the various casting operators to recognizing when to use each correctly, the knowledge gained will make a significant difference in approaching C++ programming tasks. To solidify your understanding, practice the concepts outlined, and explore further resources dedicated to C++ casting.