In C++, you can round a floating-point number to the nearest integer using the `round()` function from the `<cmath>` library. Here's a simple example:
#include <iostream>
#include <cmath>
int main() {
double num = 4.6;
std::cout << "Rounded value: " << round(num) << std::endl; // Outputs: 5
return 0;
}
Understanding Rounding in C++
Rounding is a common operation in programming, particularly in situations where we need to work with decimal numbers or perform calculations that require precision. In C++, rounding serves an essential role in ensuring that numbers are presented in an accurate and readable way.
The rounding process involves converting a floating-point number to the nearest whole number, which can be crucial for various applications—from statistical analysis to financial calculations.

The Round Function in C++
What is the Round Function?
At the heart of rounding in C++ is the round function, which can be found in the `<cmath>` header. The function's prototype is:
double round(double x);
This function takes a single argument, `x` (the number you wish to round), and returns the nearest integer as a double. Its behavior adheres to specific rules, especially when dealing with midpoint values (i.e., values that are exactly halfway between two integers).
How the Round Function Works
The round function applies a straightforward strategy to determine the nearest integer:
- If the decimal portion of `x` is less than 0.5, the value is rounded down.
- If the decimal portion is 0.5 or greater, the value is rounded up.
This means that for Midpoint values, the function rounds away from zero, which is an essential detail to consider in various applications.

Using the Round Function
Basic Usage of the Round Function
Implementing the round function in your C++ program is straightforward. Here’s a simple example demonstrating how to use it:
#include <iostream>
#include <cmath> // Required for round function
int main() {
double value = 3.6;
double roundedValue = round(value);
std::cout << "Rounded value: " << roundedValue << std::endl; // Outputs 4
return 0;
}
In this example, the number 3.6 is rounded to 4, illustrating how the round function operates.
Rounding Negative Numbers
It’s crucial to note that the round function also works with negative numbers. For instance:
#include <iostream>
#include <cmath>
int main() {
double value = -2.5;
double roundedValue = round(value);
std::cout << "Rounded value: " << roundedValue << std::endl; // Outputs -3
return 0;
}
In this scenario, -2.5 rounds to -3, which emphasizes that the round function rounds away from zero, further clarifying how it treats midpoints.

Alternative Rounding Methods
While the round function is effective, C++ provides several other rounding methods. Understanding these alternatives will enrich your rounding toolkit.
The Floor Function
The `floor()` function is another option found in the `<cmath>` header. This function simply rounds a number down to the nearest whole number:
#include <iostream>
#include <cmath>
int main() {
double value = 2.9;
std::cout << "Floor value: " << floor(value) << std::endl; // Outputs 2
return 0;
}
Here, the number 2.9 gets rounded down to 2.
The Ceil Function
In contrast, the `ceil()` function rounds numbers up to the nearest whole number. For instance:
#include <iostream>
#include <cmath>
int main() {
double value = 2.1;
std::cout << "Ceil value: " << ceil(value) << std::endl; // Outputs 3
return 0;
}
In this example, 2.1 rounds up to 3.
The Trunc Function
The `trunc()` function truncates the decimal portion of a number, effectively rounding it toward zero:
#include <iostream>
#include <cmath>
int main() {
double value = 5.7;
std::cout << "Truncate value: " << trunc(value) << std::endl; // Outputs 5
return 0;
}
Here, 5.7 is simply truncated to 5.
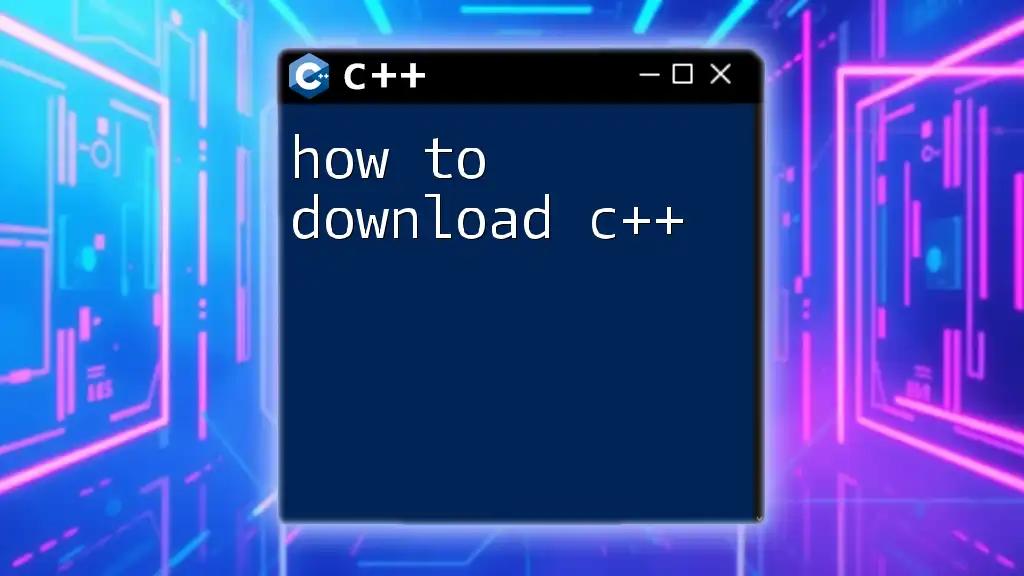
Use Cases for Rounding in C++
Real-Life Applications
Rounding operations are vital in various real-life applications:
-
Business Scenarios: In financial programming, rounding can help present monetary values in a user-friendly way (e.g., displaying a total price as $19.99 rather than $19.985).
-
Scientific Computations: When analyzing data, rounding can help in reporting results more neatly, such as presenting a mean value of 4.7 rather than 4.66666667.
-
Gaming Applications: For games, scores are often represented as whole numbers; thus, rounding scores can ensure a clear presentation.
Performance Considerations
When learning how to round in C++, it's important to use rounding functions judiciously. You should consider when and why to apply them to avoid inaccuracies especially in data-sensitive computations.
Best practices include:
- Performing rounding operations only when necessary, especially in high-performance applications.
- Utilizing appropriate rounding functions depending on your needs—whether you require rounding down, up, or truncation.
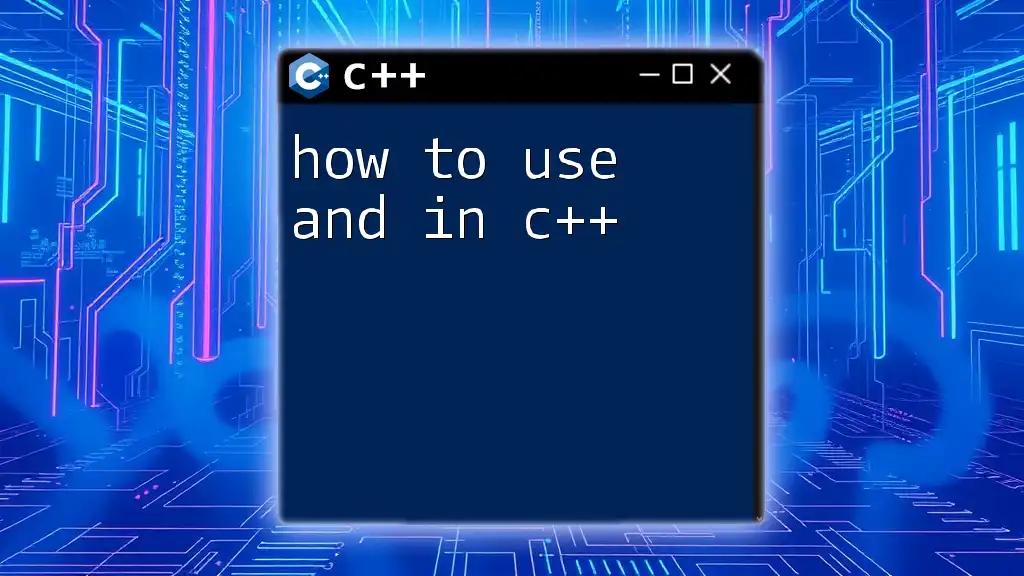
Conclusion
In summary, mastering how to round in C++ is an essential skill that can greatly enhance your programming capabilities. The round function, along with alternatives like floor, ceil, and trunc, provides a robust framework for handling numerical data. Experimenting with these functions can help solidify your understanding and improve your application of rounding in various scenarios.
Delve deeper into these functions, practice implementing them in your own projects, and share your experiences or questions in the comments section!