In C++, the `&&` operator is used as a logical AND to combine two boolean expressions, returning `true` only if both expressions are true.
Here's a code snippet illustrating its use:
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = false;
if (a && b) {
cout << "Both are true." << endl;
} else {
cout << "At least one is false." << endl;
}
return 0;
}
What is the `&&` Operator?
Definition of Logical AND
The `&&` operator is a fundamental logical operator in C++. It evaluates two boolean expressions and returns `true` only if both expressions evaluate to `true`. Understanding this operator is crucial for controlling the flow of your program based on multiple conditions.
Syntax of the `&&` Operator
The basic syntax of the logical AND operator is as follows:
expression1 && expression2
In this structure, `expression1` and `expression2` can be any valid boolean expressions, including comparisons, function calls that return a boolean, or other logical operators.
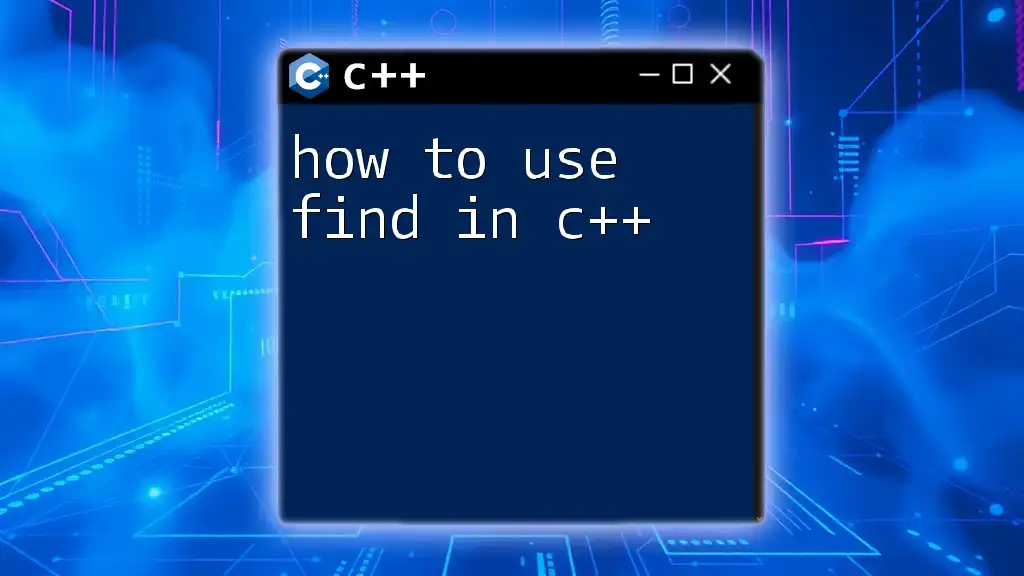
Behavior of the `&&` Operator
Short-Circuit Evaluation
One of the most notable features of the `&&` operator is its short-circuit evaluation behavior. This means that if the first expression evaluates to `false`, the second expression is not evaluated at all because the result of the entire expression can’t possibly be `true`.
Consider the following example:
bool func() {
// some code
return true;
}
if (false && func()) {
// func() is never called
}
In this case, since the first expression is `false`, the function `func()` is never executed, which could be crucial in terms of performance or side effects.
Truth Table for Logical AND
Understanding how the `&&` operator works fundamentally revolves around its truth table, which can be summarized as follows:
- `true && true` = `true`
- `true && false` = `false`
- `false && true` = `false`
- `false && false` = `false`
This truth table provides a clear view of how `&&` evaluates logical conditions, reinforcing the need for both operands to be `true` for the whole expression to yield `true`.
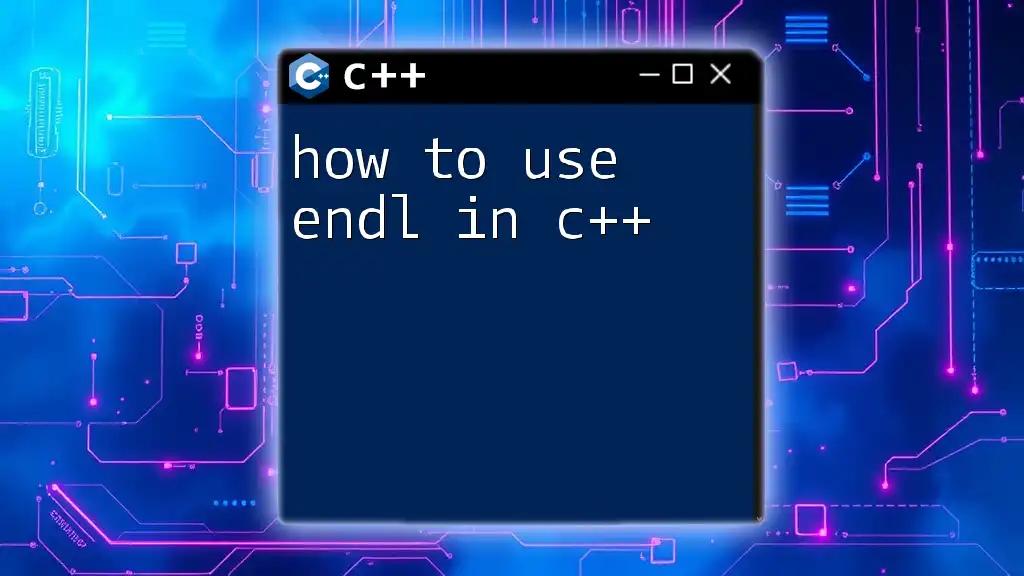
Using the `&&` Operator in Conditions
Conditional Statements
The `&&` operator is often used in `if` statements to evaluate multiple conditions simultaneously. Here’s a simple example:
int x = 10;
int y = 20;
if (x > 5 && y > 15) {
// both conditions are true
// execute some code
}
In this code, the block inside the `if` statement will run because both conditions, `x > 5` and `y > 15`, evaluate to `true`. Thus, using the `&&` operator effectively can streamline your conditional logic.
Combining Multiple Conditions
You can combine several conditions using `&&`, enabling a finely-tuned control flow based on multiple criteria. For instance:
if (x > 5 && y < 25 && x + y == 30) {
// multiple conditions evaluated
// execute code when all conditions are met
}
In this example, the code block will execute only if all three conditions evaluate to `true`.
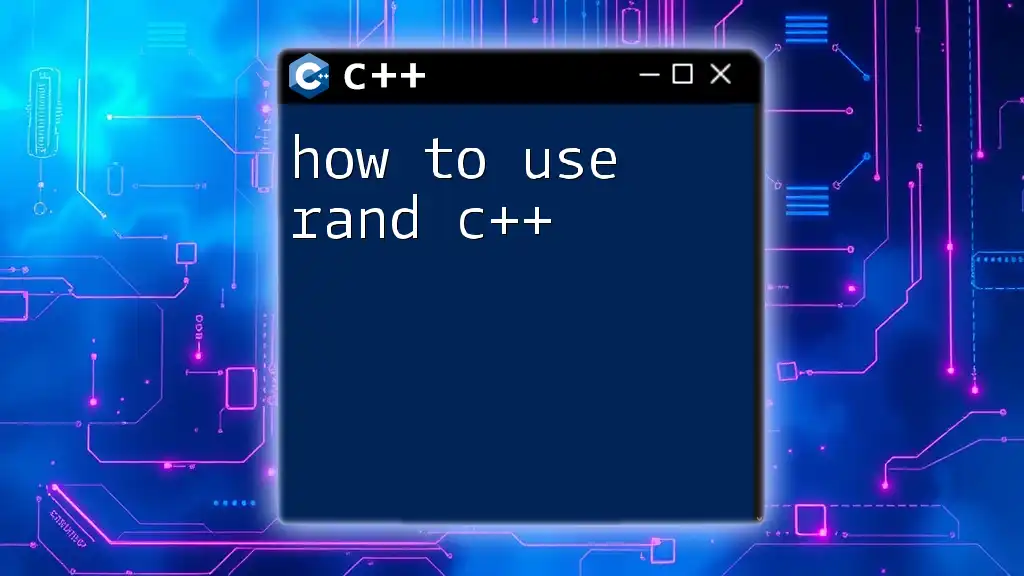
Applications of the `&&` Operator
In Loops
The `&&` operator can also be very useful within loop conditions, allowing for more complex and effective iteration control. Here’s an example using a `while` loop:
int i = 0;
while (i < 10 && i != 5) {
// Loop will break when i == 5
i++;
}
This loop will continue to execute as long as `i` is less than 10 and not equal to 5. Once `i` reaches 5, the loop will terminate, demonstrating the practical uses of the `&&` operator in controlling loop execution.
In Function Calls
Additionally, the `&&` operator can be directly applied in function arguments, allowing for cleaner code. Consider the following example:
void checkConditions(bool a, bool b) {
if (a && b) {
// Perform action
}
}
checkConditions(true, false); // Will not perform action
In this case, the action inside the function will only occur if both `a` and `b` are `true`. This illustrates how `&&` can be used to control behavior based on the combined values of multiple arguments.
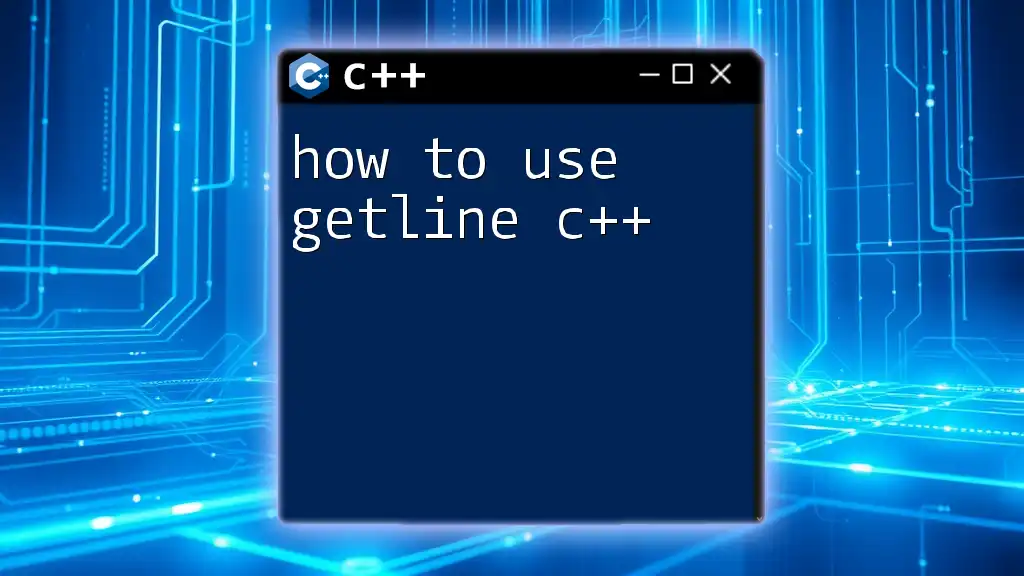
Common Pitfalls and Best Practices
Logical Confusion
When using the `&&` operator, it’s essential to avoid logical confusion. A common mistake is to use the assignment operator (`=`) instead of the equality operator (`==`). For example:
if (x = 10 && y > 5) {
// Mistake: should be x == 10
}
In this scenario, `x` is assigned the value of `10`, which will always evaluate as `true`, leading to unintended behavior. Always ensure that you’re using the `==` operator when you intend to compare values.
Readability and Maintainability
A best practice for writing clear C++ code is to enhance readability. Using parentheses can improve clarity when combining conditions. For instance:
if ((a && b) || (c && d)) {
// clearer grouping of conditions
}
This explicit grouping helps prevent logical errors and enhances maintainability, allowing yourself or others to quickly grasp the intended logic of the condition.
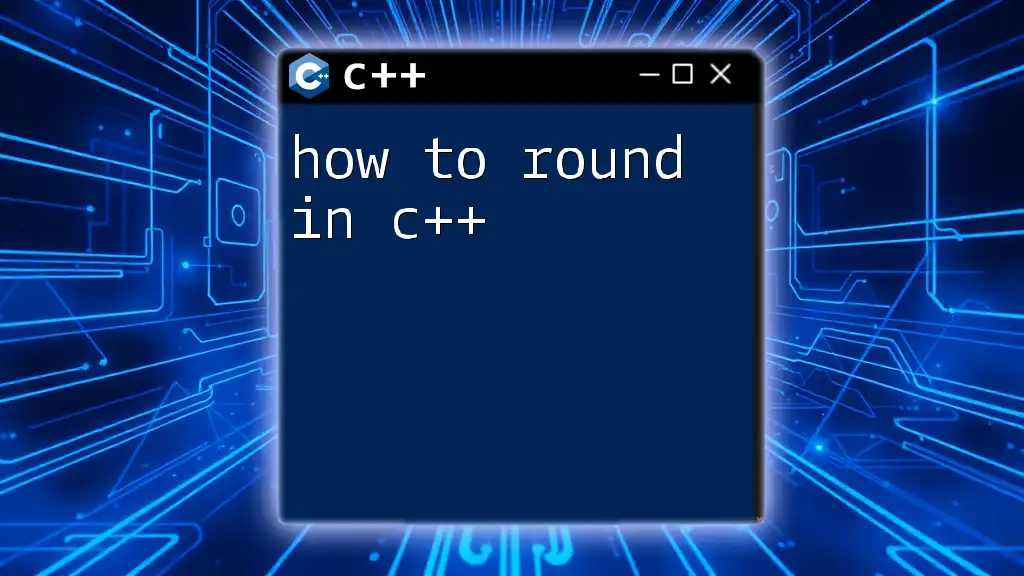
Conclusion
By now, you should have a comprehensive grasp of how to use `&&` in C++. Understanding the logical AND operator enables you to write more complex and effective conditional logic in your programs. I encourage you to practice using `&&` in various scenarios to reinforce your skills and deepen your understanding.
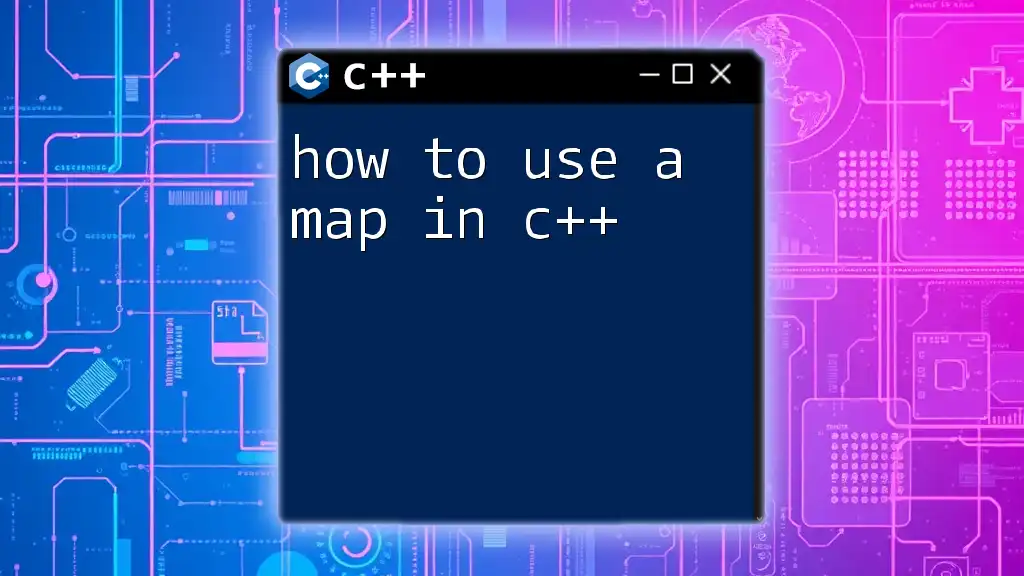
Further Resources
For those looking to expand their knowledge further, consider exploring recommended books, online tutorials, and forums dedicated to C++ programming. Engaging with the community can provide valuable insights and answers to your questions as you continue your journey in mastering C++.