In C++, you can multiply two numbers using the `*` operator, as shown in the following code snippet:
#include <iostream>
using namespace std;
int main() {
int num1 = 5, num2 = 10;
int product = num1 * num2;
cout << "The product is: " << product << endl;
return 0;
}
Basics of C++ Multiplication
What is Multiplication in C++?
Multiplication in C++ is a fundamental arithmetic operation that allows you to combine two or more numbers to produce a product. In programming terms, it can be expressed using the multiplication operator, which is denoted by the asterisk symbol (*). This simple operator serves as a powerful tool in performing calculations within various applications, from basic computations to complex algorithms.
Data Types Suited for Multiplication
C++ supports several data types that can be utilized for multiplication, each with its own characteristics:
- Integer Types: This includes `int`, `long`, and `short`. They are useful for counting whole numbers. Multiplying integers can lead to overflow if the resulting product exceeds the maximum supported value.
- Floating-Point Types: This includes `float` and `double`. They are particularly useful for dealing with decimals and real numbers. Floating-point multiplication can introduce precision issues due to the way these types handle numerical data.
Here is an example code snippet demonstrating how different types interact during multiplication:
int a = 5;
float b = 2.5;
float result = a * b; // result would be 12.5
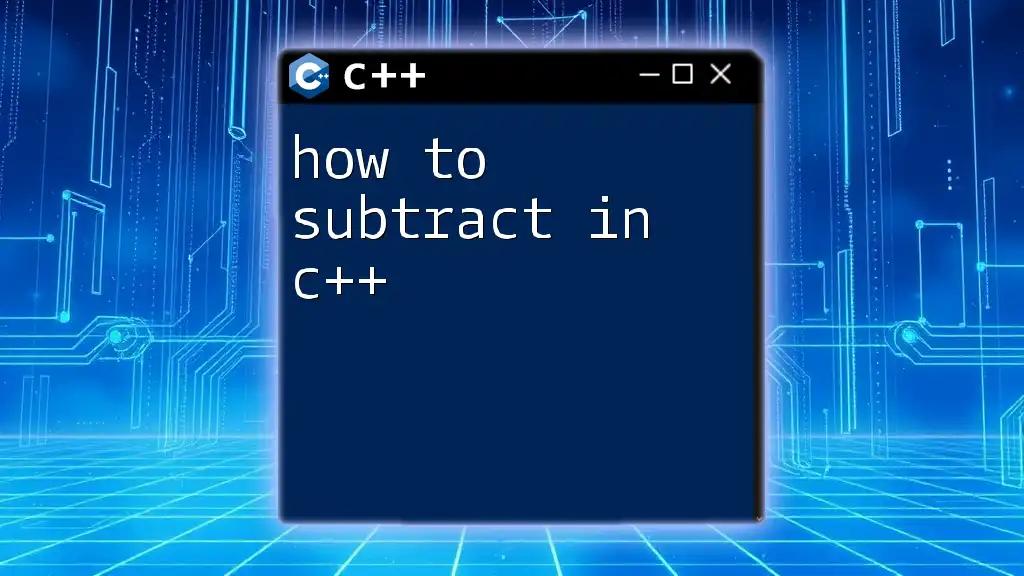
Performing Multiplication in C++
Using the Multiplication Operator
The multiplication operator in C++ is straightforward to use. You can perform multiplication between two values directly using the `*` operator. Below is an example code that illustrates simple multiplication:
#include <iostream>
using namespace std;
int main() {
int x = 10;
int y = 20;
int product = x * y;
cout << "The product of " << x << " and " << y << " is: " << product << endl;
return 0;
}
When this code runs, it calculates the product of `x` and `y`, displaying the result as The product of 10 and 20 is: 200.
Multiplication with Variables
Multiplication becomes even more dynamic when using variables. By allowing user input, you can compute products based on real-time data. Here's an example illustrating this concept:
#include <iostream>
using namespace std;
int main() {
int num1, num2;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
cout << "The product is: " << num1 * num2 << endl;
return 0;
}
In this program, users can enter any two integers, and the program will display the corresponding product. This feature fosters interactivity and broadens the use cases for multiplication in C++.
Multiplying in Loops
Using multiplication within loops can significantly expand your calculations, especially when dealing with a series of numbers. For example, you may want to compute the product of all numbers in an array. Here is how you can do it:
#include <iostream>
using namespace std;
int main() {
int numbers[] = {1, 2, 3, 4, 5};
int product = 1;
for (int i = 0; i < 5; i++) {
product *= numbers[i];
}
cout << "The product of the array is: " << product << endl;
return 0;
}
This code multiplies every element in the `numbers` array, resulting in the output The product of the array is: 120. This technique is highly valuable in algorithm design and data analysis processes.

Advanced Considerations in C++ Multiplication
Overflow in Integer Multiplication
One important factor to consider when performing multiplication in C++ is the potential for integer overflow. When the result of multiplying two integers exceeds the maximum value that can be stored in that data type, it can lead to incorrect calculations. Here's an illustration of this risk:
#include <iostream>
using namespace std;
int main() {
int largeNumber1 = 100000;
int largeNumber2 = 100000;
int product = largeNumber1 * largeNumber2; // Potential overflow
cout << "The product is: " << product << endl; // May give incorrect results
return 0;
}
In this scenario, the product exceeds the limit and may yield a seemingly nonsensical result. It stresses the need for developers to choose appropriate data types to handle large numbers.
Precision in Floating-Point Multiplication
When dealing with floating-point numbers, another consideration is the precision issues that may arise due to the way these numbers are stored in computer memory. The representation of decimals can lead to unexpected results. Here's an example:
#include <iostream>
using namespace std;
int main() {
double a = 0.1;
double b = 0.2;
double result = a * b;
cout << "The result of 0.1 * 0.2 is: " << result << endl; // Precision issues may occur
return 0;
}
The output may not match your mathematical expectation due to how floating-point arithmetic is handled internally, highlighting the significance of being cautious with operations involving decimals.

Conclusion
Understanding how to multiply in C++ is crucial for programming as it forms a thousand different applications across software development. Mastering the multiplication operator, while being aware of data types and potential pitfalls, empowers you to perform effective calculations in your programs.
Explore different multiplication scenarios, and consider the complexity they may introduce. Happy coding!