In C++, you can subtract two numbers using the subtraction operator `-` within a simple expression or within a function as shown in the following code snippet:
#include <iostream>
using namespace std;
int main() {
int a = 10;
int b = 5;
int result = a - b;
cout << "The result of subtraction is: " << result << endl;
return 0;
}
Understanding the Basics of Subtraction in C++
Defining Subtraction
Subtraction is a fundamental arithmetic operation that represents the process of determining the difference between two numbers. In the context of programming, understanding how to perform subtraction is crucial as it often serves as a building block for more complex calculations and algorithms.
Subtraction Syntax in C++
In C++, subtraction is expressed using the `-` operator. This operator can be employed in simple arithmetic, as well as integrated within more complex expressions alongside other mathematical operations.
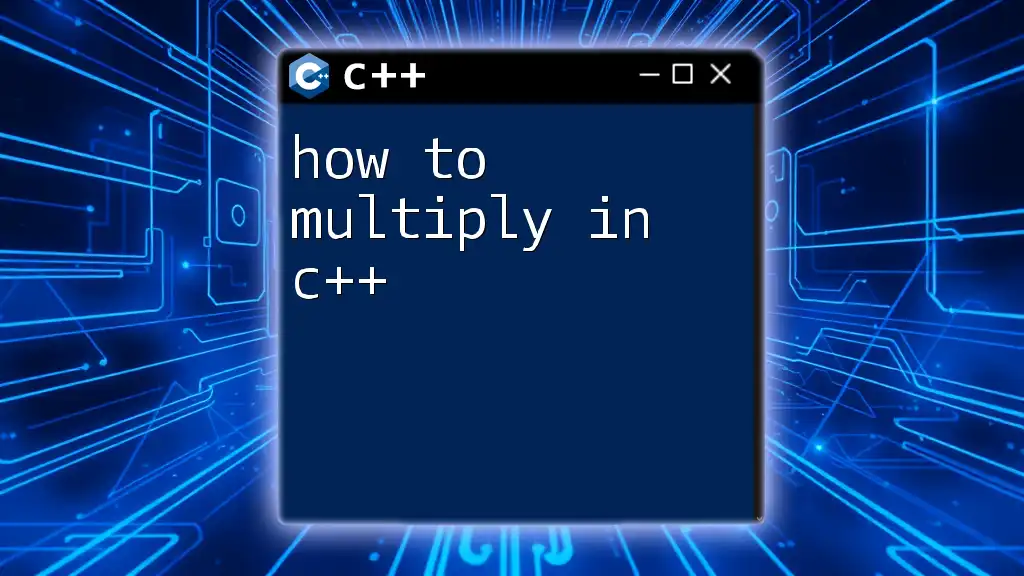
The Subtraction Operator: `-`
What is the Subtraction Operator?
The subtraction operator `-` is a binary operator that takes two operands and returns the result of the first operand minus the second operand. Below is a simple illustration of its use:
int a = 10;
int b = 5;
int result = a - b; // result will be 5
In this example, `a` is reduced by `b`, and the difference (5) is stored in the variable `result`.
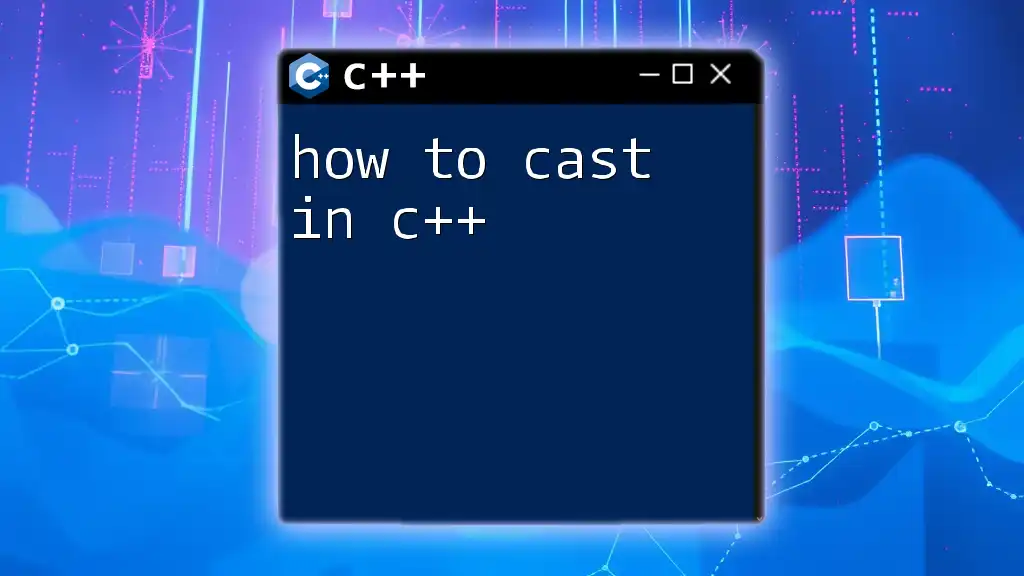
Subtracting Variables
Subtracting Two Variables
When working with variables, subtraction allows us to manipulate data dynamically. For instance, consider the following code:
float x = 7.5;
float y = 2.3;
float difference = x - y; // difference will be 5.2
Here, the variables `x` and `y` are subtracted, and the result, `5.2`, is stored in `difference`. This showcases how even with floating-point numbers, C++ handles subtraction effectively.
Subtracting Multiple Variables
Subtraction isn't limited to just two variables—it's possible to subtract multiple variables in a single expression. For example:
int a = 20;
int b = 5;
int c = 3;
int total = a - b - c; // total will be 12
The variable `total` will hold the result of the subtraction, which is `12` in this case. Understanding this allows programmers to handle complex calculations efficiently.
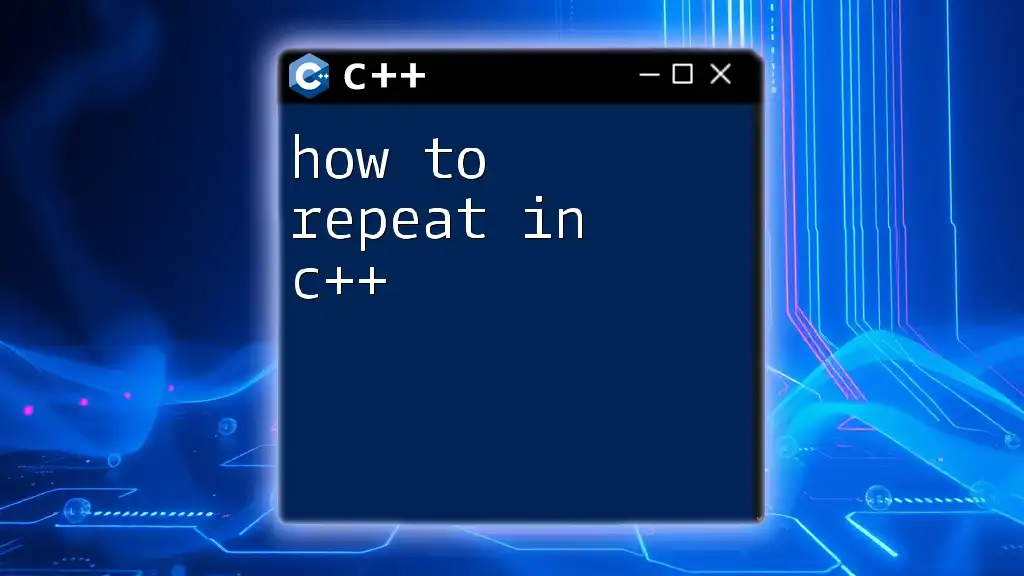
Subtraction with Input from Users
Getting User Input for Subtraction
C++ allows for user interaction through command line inputs. You can obtain two numbers from the user and perform subtraction as shown below:
int num1, num2;
std::cout << "Enter two numbers: ";
std::cin >> num1 >> num2;
int result = num1 - num2;
std::cout << "The result is: " << result << std::endl;
This snippet prompts the user to enter two numbers, performs the subtraction, and outputs the result. It emphasizes the dynamic nature of programming where inputs can change based on user interaction.
Handling Invalid Input
When accepting user input, it’s essential to consider input validation to prevent errors or unexpected behavior. A basic way to handle this is by checking whether the input is of the expected type, using a simple validation mechanism:
if (std::cin.fail()) {
std::cout << "Invalid input. Please enter numeric values." << std::endl;
std::cin.clear(); // clear the error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // discard input
}
This code checks if the inputs are valid integers. If not, it prompts the user to provide correct input.
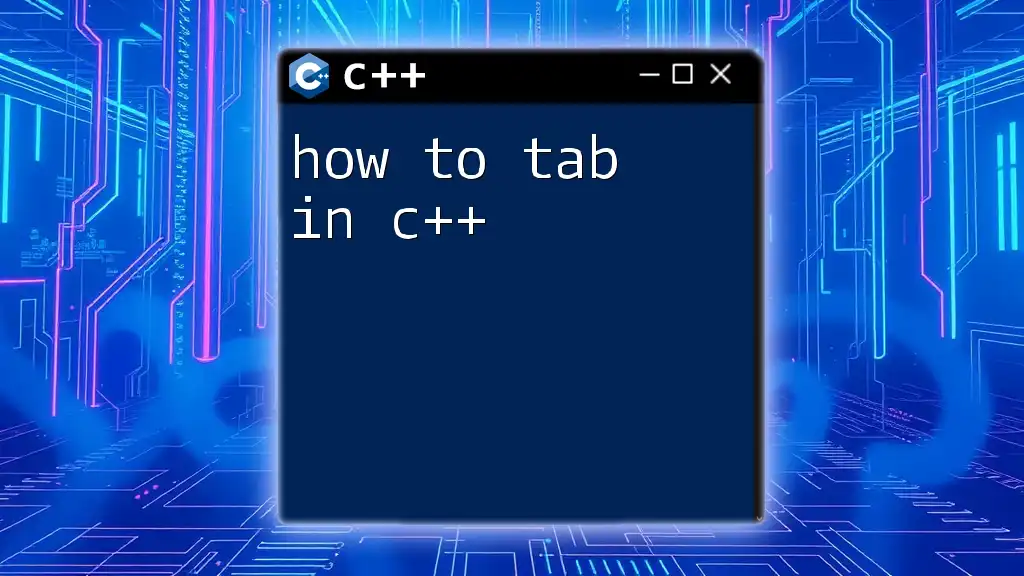
Real-Life Applications of Subtraction
Use Cases in Programming
Subtraction is commonly encountered in various programming applications. For instance, in budgeting software, there may be a need to find the remaining balance after expenses. Additionally, subtraction is critical in handling time calculations, such as determining the difference between deadlines.
Subtraction in Mathematical Operations
Subtraction can also play a vital role in more complex math-related software, like calculators. Developers can implement a simple command-line calculator that performs subtraction as follows:
char operation;
int num1, num2;
std::cout << "Enter two numbers: ";
std::cin >> num1 >> num2;
std::cout << "Choose operation (+, -, *, /): ";
std::cin >> operation;
if (operation == '-') {
std::cout << "The result is: " << (num1 - num2) << std::endl;
}
This code snippet allows users to perform subtraction interactively, demonstrating a practical application of the `-` operator.
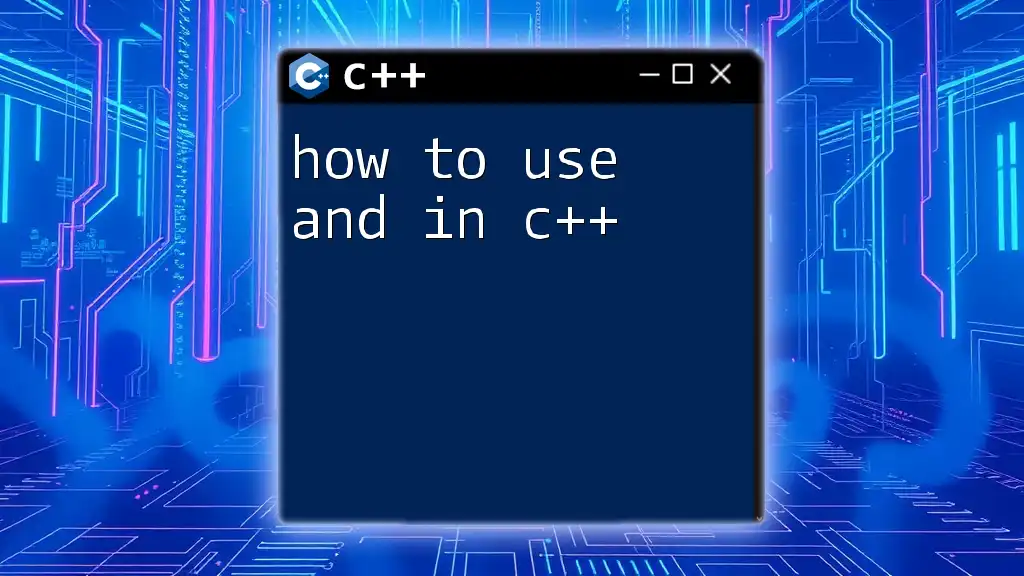
Advanced Subtraction Techniques
Overloading the Subtraction Operator
For more complex data structures, such as classes, C++ allows operator overloading. This enables you to define custom subtraction logic for user-defined types. For example:
class Point {
public:
int x, y;
Point(int x, int y) : x(x), y(y) {}
Point operator-(const Point& p) {
return Point(x - p.x, y - p.y);
}
};
In this example, the subtraction operator is overloaded to handle `Point` objects, allowing for subtraction of their respective coordinates, thus providing a more intuitive interface.
Subtraction with Different Data Types
It's also important to recognize how C++ handles subtraction across different data types, such as integers, floats, and doubles. With implicit type conversion, the compiler tries to interpret one type as another as needed. However, care must be taken to avoid unexpected results, particularly involving rounding errors with floating-point numbers.
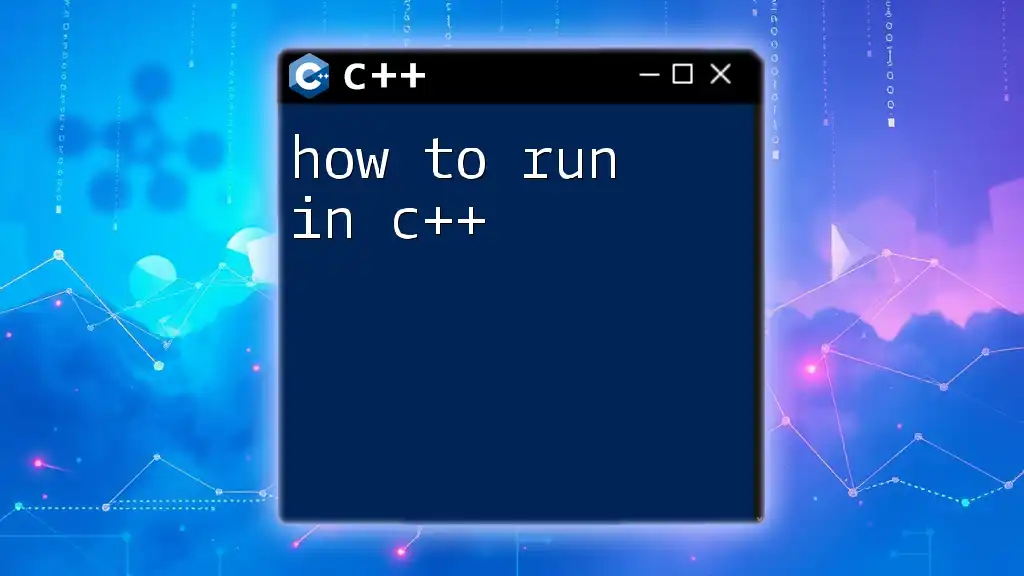
Common Errors and Troubleshooting
Compilation Errors
Subtraction operations can lead to compilation errors if the operands are incompatible. For example, trying to subtract a string from an integer would generate an error. Always ensure that both operands are of compatible types to avoid such issues.
Logical Errors
Logical errors may occur if the intended outcome of the subtraction is not produced due to miscalculations or incorrect logic. For example:
int a = 10;
int b = 20;
int c = a - b; // c will be -10, which may not be the expected behavior if intended to always be positive
Identifying and resolving these issues often requires careful tracing of variable values and logic flow.
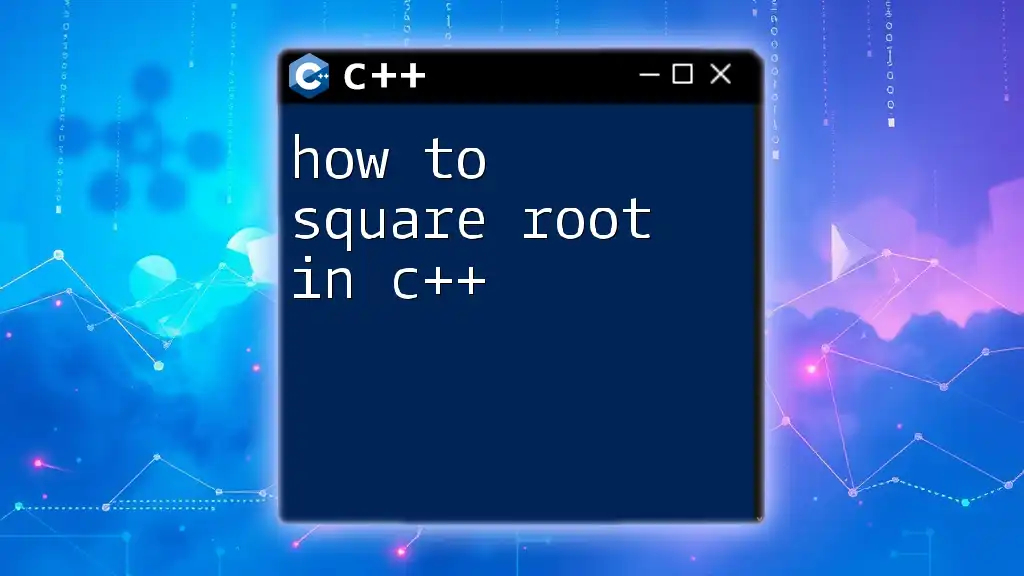
Conclusion
Subtraction in C++ is a fundamental operation that not only serves as a basic arithmetic function but also plays a significant role in various coding scenarios, from algorithms to user interaction. By mastering how to subtract in C++, programmers gain the ability to implement more complex functionalities and solve a wider variety of problems.
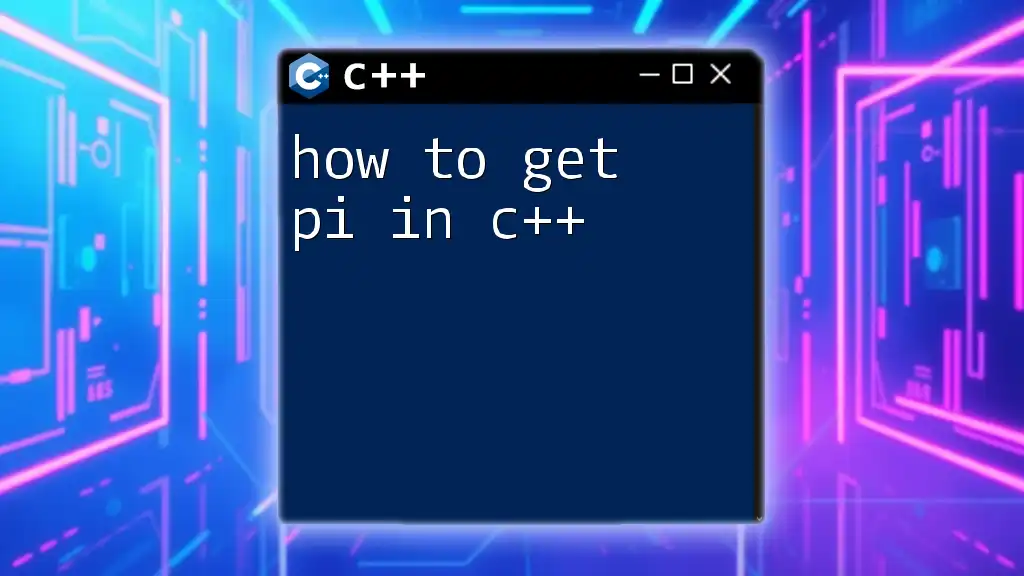
Additional Resources
For further exploration of subtraction and other C++ operations, consider consulting online resources such as official documentation, coding courses on platforms like Codecademy, and coding challenge websites to enhance your practical skills.