To write a simple C++ program, start by including the necessary headers, define the `main` function, and use `cout` to output text to the console. Here's a basic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++
What You Need to Start Coding
Before diving into how to write C++, it's essential to set up your development environment.
Development Environment You need a suitable environment to begin coding. You can choose between an Integrated Development Environment (IDE) or a simple text editor. Here are some recommended IDEs:
- Visual Studio: Rich in features, great for Windows users.
- Code::Blocks: Lightweight and cross-platform.
- CLion: A powerful IDE for C++ development by JetBrains that supports modern C++ standards.
Installing a Compiler A compiler translates your C++ code into executable programs. Popular compilers include GCC (GNU Compiler Collection) and Clang. Here’s how to install GCC on different platforms:
- Windows: Install MinGW or use WSL for a Linux-like experience.
- MacOS: Install Xcode Command Line Tools using `xcode-select --install`.
- Linux: Use your package manager (e.g., `sudo apt install build-essential` for Debian-based systems).
Writing Your First C++ Program
To understand how to write C++, you should start with the basic structure of a C++ program.
Basic Structure of a C++ Program Every C++ program begins with the main function, which serves as the entry point. Here's the syntax:
#include <iostream> // Include header file for input and output
using namespace std; // Use the standard namespace
int main() { // Main function
// Code goes here
return 0; // End of the program
}
Hello World Example Let's write your first program—a simple "Hello, World!" application. This classic example demonstrates basic output functionality in C++.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!"; // Print to console
return 0;
}
In this example:
- `#include <iostream>` imports the input-output stream library.
- `cout << "Hello, World!";` prints the text to the console.
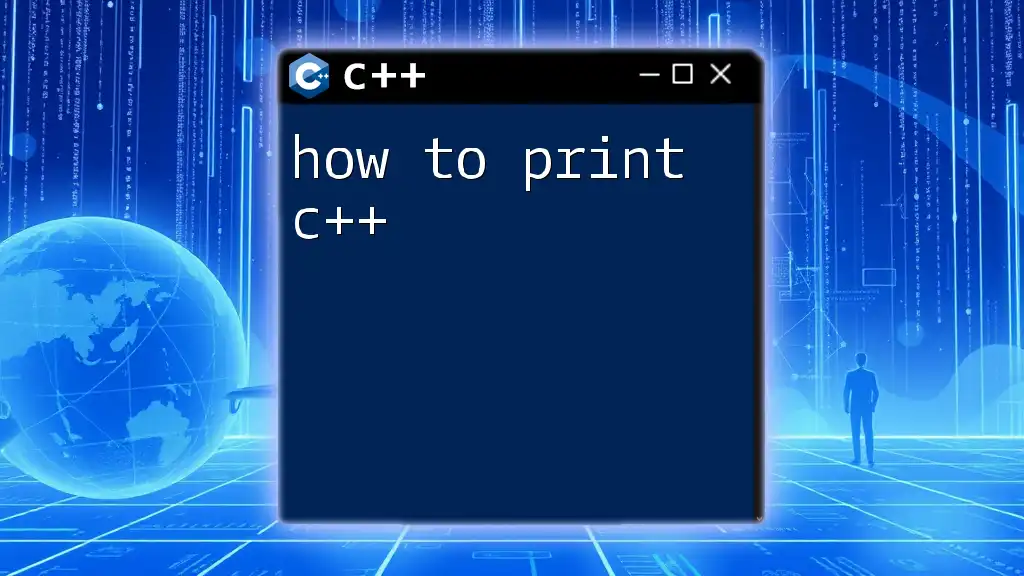
Core Concepts of C++
Data Types and Variables
Understanding data types is crucial when learning how to write C++.
Fundamental Data Types C++ has several basic data types:
- int: For integers.
- float: For single-precision floating-point numbers.
- double: For double-precision floating-point numbers.
- char: For single characters.
Declaring Variables Variables must be declared before use. Here’s how to declare different variables:
int age = 30; // Integer variable
float salary = 50000.50; // Floating-point variable
char grade = 'A'; // Character variable
Operators
Operators allow you to perform calculations and comparisons. Here’s a brief overview of important operator types.
Arithmetic Operators These include `+`, `-`, `*`, `/`, and `%`. For example:
int sum = a + b; // Addition
int product = a * b; // Multiplication
Comparison Operators These operators let you compare values, such as `==`, `!=`, `>`, `<`, `>=`, and `<=`.
Logical Operators Logical operators include `&&` (AND), `||` (OR), and `!` (NOT) which can help control flow in conditional statements.
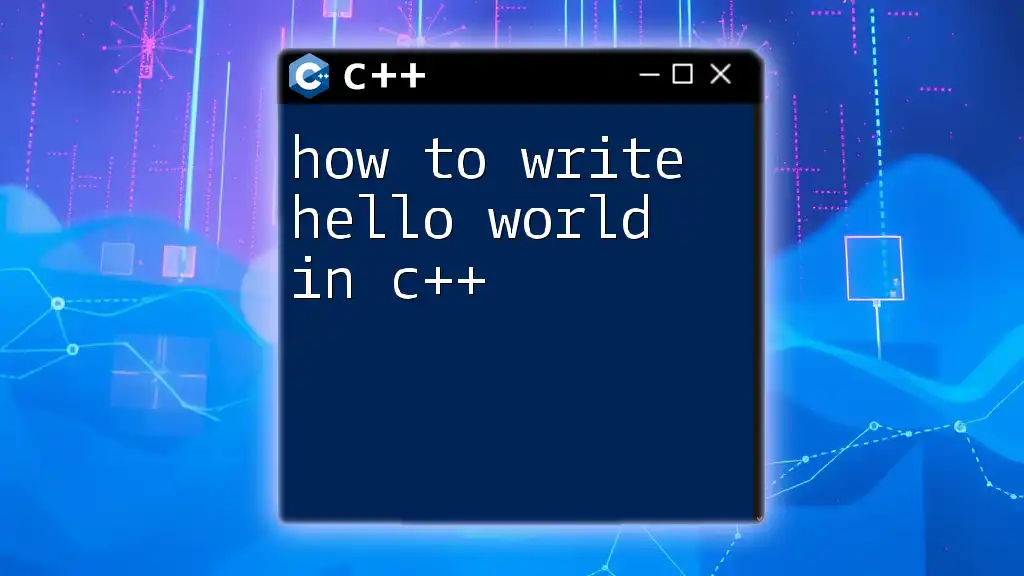
Control Structures
Conditional Statements
Conditional statements control the flow of execution in your application.
If and Else Statements Here's the basic syntax:
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
For instance:
int age = 20;
if (age >= 18) {
cout << "You are an adult.";
} else {
cout << "You are a minor.";
}
Switch Case Statement Switch statements are helpful for multiple conditions based on the value of a variable:
switch (grade) {
case 'A':
cout << "Excellent!";
break;
case 'B':
cout << "Good job!";
break;
default:
cout << "Keep trying!";
}
This structure helps reduce complexity when dealing with many conditions.
Loops
Loops are used for repeating a block of code.
For Loops Ideal for a set number of iterations:
for (int i = 0; i < 10; i++) {
cout << i << " ";
}
While Loops A while loop continues as long as the condition remains true:
int i = 0;
while (i < 10) {
cout << i << " ";
i++;
}
Do-While Loops A do-while loop executes the block at least once:
int i = 0;
do {
cout << i << " ";
i++;
} while (i < 10);
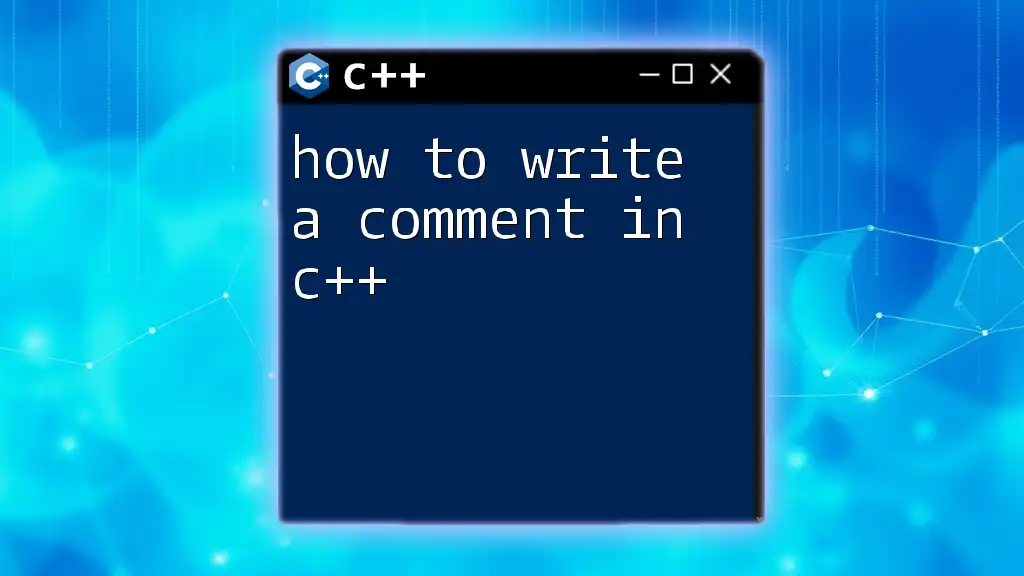
Functions in C++
Understanding Functions
Functions are essential in C++ for organizing code into reusable blocks.
What is a Function? A function is a block of code that performs a specific task. It can take inputs and return outputs.
Function Syntax Here is a simple function that adds two integers:
int add(int a, int b) {
return a + b; // Return the sum
}
Function Overloading
C++ allows functions to have the same name but different parameters. This is known as function overloading.
Examples of Function Overloading
void print(int i) {
cout << "Integer: " << i;
}
void print(double d) {
cout << "Double: " << d;
}
You can call `print(5);` to output the integer version or `print(5.5);` for the double version.
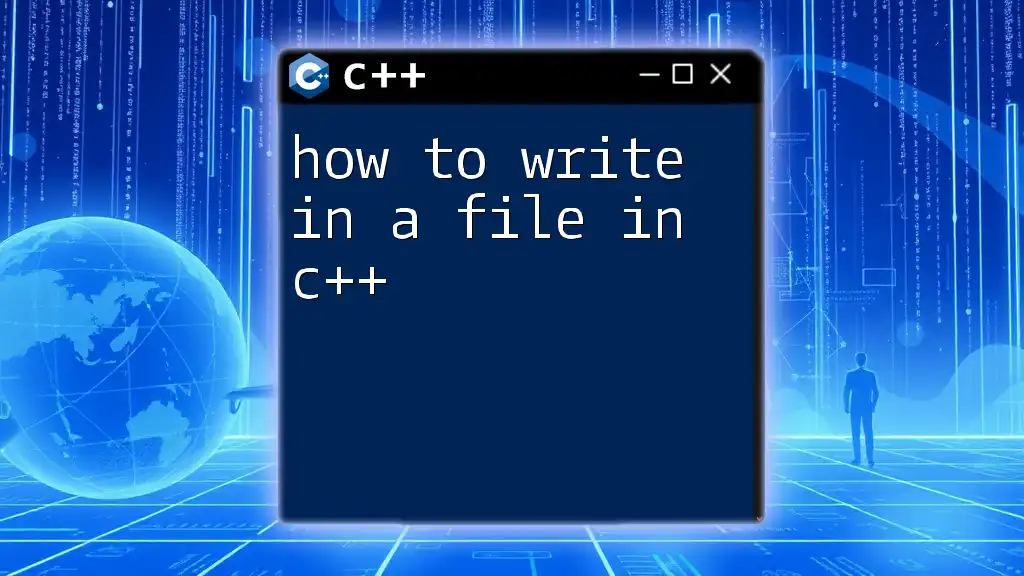
Object-Oriented Programming in C++
Introduction to OOP
Object-oriented programming (OOP) is a programming paradigm based on objects that contain data and methods.
Basic Principles of OOP OOP is built around the following concepts:
- Encapsulation: Wrapping data and methods into a single unit.
- Inheritance: Deriving new classes from existing ones.
- Polymorphism: Allowing functions to use entities of different types.
Creating Classes and Objects
To create a class in C++:
class Car {
public:
string brand; // attribute
void honk() { // method
cout << "Beep!";
}
};
You can create an object from this class:
Car myCar;
myCar.brand = "Toyota";
myCar.honk();
Inheritance and Polymorphism
Understanding Inheritance Inheritance allows a class to inherit attributes and methods from another class:
class Vehicle {
public:
void start() {
cout << "Vehicle started";
}
};
class Car : public Vehicle {
};
Polymorphism Polymorphism enables a single function to behave differently based on the object type:
class Animal {
public:
virtual void sound() {
cout << "Animal sound";
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark!";
}
};
This allows you to call `sound()` without knowing the exact type of the object at compile time.
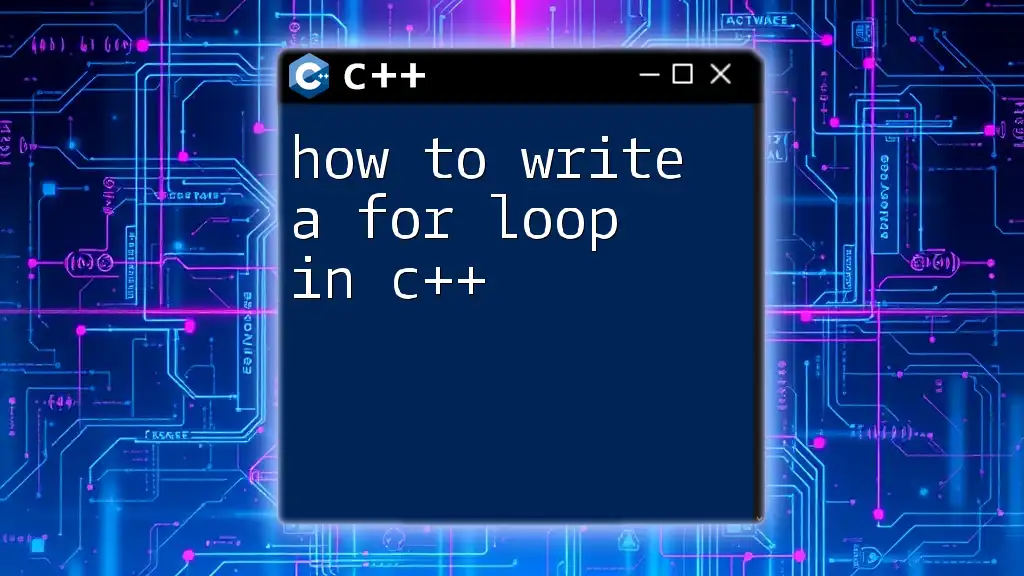
Conclusion
In this guide on how to write C++, we covered essential concepts, from setting up your environment to core programming principles. Practice is crucial—the more code you write, the more adept you'll become at C++.
As you move forward, explore additional resources, such as books, online courses, and C++ communities. Remember, hands-on coding and building projects are the best ways to solidify your understanding and skills in C++. Best of luck on your journey to mastering C++!