To use C++ in Visual Studio, create a new project, select "Console Application," and write your code in the provided main file.
Here's a simple "Hello, World!" example in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Visual Studio for C++
Downloading Visual Studio
To begin your journey on how to use C++ in Visual Studio, the first step is downloading the software. Visual Studio is available on its official website, where you can find various versions, including Community, Professional, and Enterprise. For beginners, the Community version is highly recommended. It’s free and provides all the essential features needed for developing C++ applications.
Installing C++ Workloads
During the installation process, you will be prompted to select workloads. Make sure to choose the Desktop development with C++ workload. This selection ensures that you have all tools necessary for creating standard C++ applications. Additionally, if you are interested in C++ and .NET applications, consider including the C++/CLI support workload.
During installation, visualize the process and ensure that you select the right options to maximize your development environment.

Creating a New C++ Project
Starting a New Project
Once Visual Studio is installed, it’s time to create your first C++ project. To do this, navigate to:
File -> New -> Project
A dialog will pop up allowing you to select a template. When prompted, opt for the Console App under the C++ templates. This template is ideal for writing simple C++ code and understanding the basics of the language.
Configuring Project Properties
After creating your project, you’ll want to configure the project properties. This configuration is crucial as it will define how your application is built. Right-click on your project in the Solution Explorer and select Properties. Here, you will find critical settings such as the Platform and the Configuration Type. Understanding these properties is essential as they affect the compilation process and overall performance of your application.

Writing Your First C++ Program
Basic Structure of a C++ Program
Understanding the basic structure of a C++ program is fundamental. Every C++ program begins with headers, where you include essential libraries, followed by the `main()` function, which serves as the entry point of the program. Here’s a simple example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, `#include <iostream>` includes the input-output stream library, allowing us to use `cout` for console output. This program will simply print "Hello, World!" to the console, demonstrating the basics of C++ syntax.
Adding Code to Your Project
To add code to your project, create a new .cpp file by navigating to:
File -> Add -> New Item -> C++ File
You can then copy and paste the provided example into your new file. Understanding the syntax and how it executes will be your stepping stone into more complex programming tasks. Watch for common errors, such as missing semicolons or mismatched braces, which can easily hinder your compilation process.

Compiling and Running Your C++ Program
Building the Project
After you’ve added your code, the next step is to compile or "build" your project. This process translates your C++ code into machine-readable instructions. To build your project, go to:
Build -> Build Solution
Ensure that there are no errors in the Error List window that might prevent the build from succeeding.
Running the Application
Once built successfully, it’s time to run your application. You can either debug your application or run it without debugging. To run without debugging, navigate to:
Debug -> Start Without Debugging
This command executes your application, allowing you to see the output of your code.

Debugging Your C++ Code
Understanding the Debugger
Debugging is a crucial aspect of programming, and Visual Studio's debugger offers powerful features. Breakpoints allow you to pause execution at a specific line of code, enabling you to inspect variable values and control flow. To set a breakpoint, click in the margin next to the line number. This step is essential when you need to trace through your code to identify logic errors.
Common Debugging Techniques
Once you’ve set breakpoints, you can utilize step-through debugging by pressing the F10 key. This functionality allows you to execute code line-by-line, giving you the opportunity to closely monitor how your variables change during execution. Additionally, you can use watch variables to keep track of specific data states throughout the debugging process.

Working with Libraries and Includes
Standard Library
C++ comes with the Standard Library, which provides a collection of classes and functions for common tasks, including handling data structures and algorithms. To include these libraries in your code, use the `#include` directive:
#include <vector>
#include <string>
Utilizing the Standard Library can save time and effort and enhance your programming efficiency.
Third-Party Libraries
If you wish to expand your capabilities further, consider integrating third-party libraries like Boost or SDL. The integration process typically involves downloading the library and linking it to your project. Follow the specific instructions provided with the library for setup. This process often includes modifying project properties to include library directories and linking against needed binaries, further expanding your development toolkit.

Conclusion
Mastering how to use C++ in Visual Studio is essential for developers looking to build powerful applications. By following the steps outlined in this guide, you can set up your environment, write your first program, debug your code, and leverage various libraries effectively.
As you continue learning, explore additional resources such as online tutorials and documentation to deepen your understanding of C++ concepts, enabling you to tackle more complex projects in no time. Start building and experimenting today, and take your coding skills to the next level!
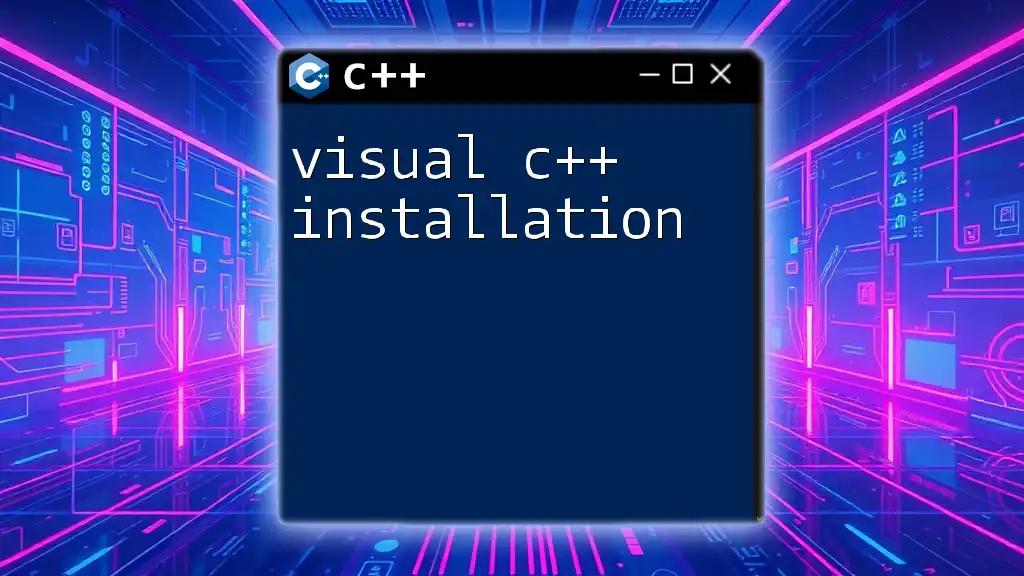
Additional Resources
For further learning, check out the official [C++ documentation](https://en.cppreference.com/w/), and explore recommended books and online courses tailored for aspiring C++ developers. Your journey in C++ programming is just beginning—embrace the challenge and enjoy the process!