The Visual Studio Redistributable for C++ is a package that installs the necessary runtime components required to run applications developed with C++, enabling seamless execution of programs on different machines.
Here’s a simple code snippet demonstrating a basic C++ program that you can compile and run using Visual Studio:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Visual Studio C++ Redistributable
What Are Redistributable Packages?
Visual Studio Redistributable C++ packages contain the necessary runtime components for running C++ applications developed with Visual Studio. These components include dynamic link libraries (DLLs) that your application depends on at runtime. When you build a C++ application, it often relies on certain functionalities provided by the Visual C++ libraries, which need to be present on the target machine where the application is executed. Without these redistributable packages, users may encounter errors indicating missing DLL files when they try to run your application.
Types of Visual Studio C++ Redistributable
Different versions of Visual Studio have their own redistributable packages, which are designed to be backward-compatible to ensure that applications built with older versions of Visual Studio can still run on machines that have newer redistributable versions installed.
The following are some of the key versions of Visual Studio C++ Redistributable:
- Visual Studio 2010
- Visual Studio 2012
- Visual Studio 2013
- Visual Studio 2015-2019
- Visual Studio 2022
It’s crucial to ensure compatibility between the redistributable version and the application you are targeting. For example, an application built with Visual Studio 2015 should ideally be run with the Visual Studio 2015 Redistributable, although the 2015–2019 package may provide compatibility in most cases.
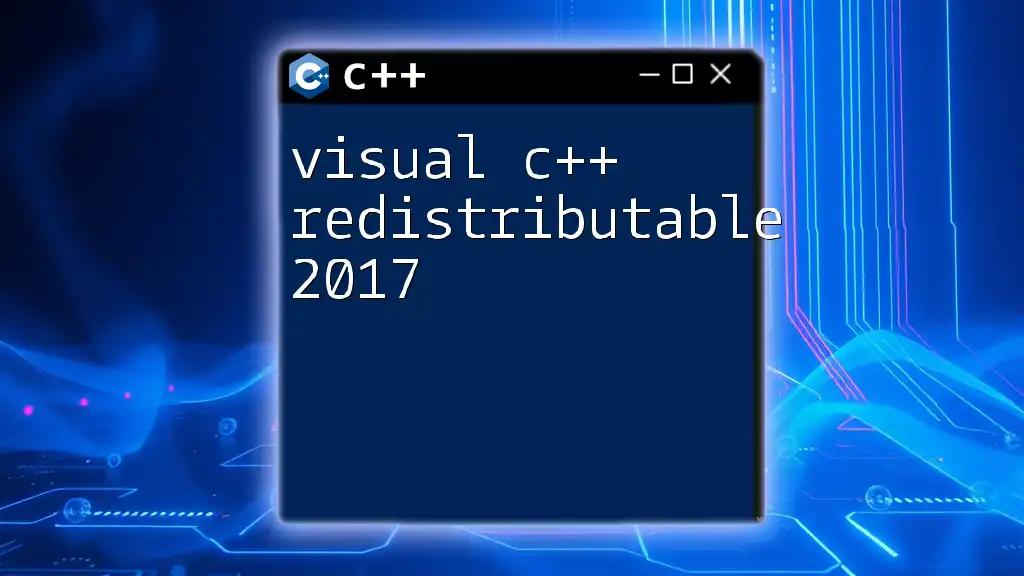
How Visual Studio Redistributable Works
Installation Process
To run a C++ application on a user's machine, the appropriate Visual Studio Redistributable must be installed. Here’s how to do it:
-
Downloading the Redistributable Package
- You can download the latest Visual Studio Redistributable from the official [Microsoft website](https://support.microsoft.com/en-us/help/2977003/the-latest-supported-visual-c-downloads).
-
Running the Installer
- Once downloaded, simply run the installer and follow the prompts to complete the installation.
You can also install the redistributable via the command line. Here’s an example code snippet for installing the redistributable using command prompt:
vcredist_x64.exe /install /quiet /norestart
This command runs the installer quietly, meaning it doesn’t display prompts, and it prevents the machine from restarting automatically after installation.
Deployment of C++ Applications
When preparing C++ applications for distribution, it's essential to include redistributable dependencies. Visual Studio offers various build configurations, which help you set up your application for release. Here’s an example of how to bundle redistributables:
- In Visual Studio, navigate to Project Properties.
- Go to Configuration Properties → General, and set Configuration Type to Application (.exe).
- Ensure that your project is set to Release mode for production deployment.
- In the Linker → Input section, ensure that the necessary libraries are included.
After these settings, you can package your application with the required redistributables. If you're using a deployment tool, ensure it can detect and package the necessary components.
// Example code to bundle redistributables using an installer
void BundleRedistributables() {
// Your bundling logic here
CopyFile("path_to_vc_redist.x64.exe", "destination_path");
}

Troubleshooting Common Issues
Common Errors Related to Redistributables
Many users encounter issues such as missing .dll files when running applications that require Visual Studio Redistributables. These errors often manifest as pop-up messages indicating that specific DLLs (like `msvcp140.dll`) are missing.
To resolve these issues:
- Download and install the correct redistributable version that matches your application's build version.
- If users still face issues after installation, recommend them to repair the installation via Control Panel.
Checking Installed Redistributables
To verify which redistributable packages are installed, users can either check through the Control Panel or use the command prompt:
- Control Panel Method: Navigate to Control Panel → Programs → Programs and Features. Look for entries like "Microsoft Visual C++ 2015 Redistributable (x64)".
- Command Prompt Method: Use the command below to list installed packages in the command prompt:
wmic product get name | findstr "Visual C++"
This command provides a clear view of all installed Visual C++ Redistributable packages.
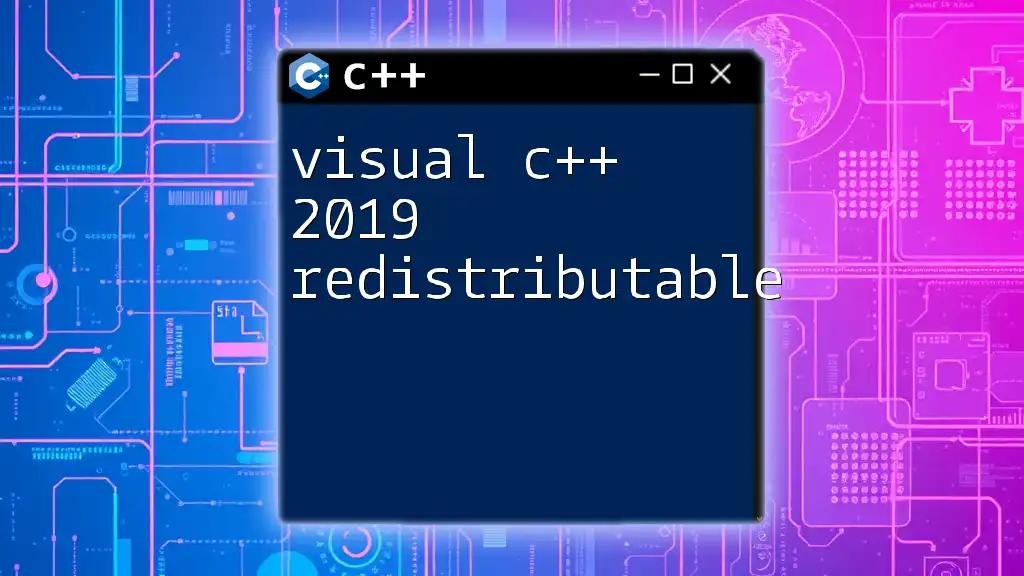
Best Practices for Using Visual Studio C++ Redistributables
Version Management
Managing different versions of Visual Studio Redistributables can be challenging. Developers should keep the redistributable packages updated to avoid compatibility issues. Regularly check the official Microsoft website for the latest versions and release notes to understand compatibility.
Optimization Strategies
To optimize your application's size and reduce dependency on redistributable packages, consider the following techniques:
-
Static Linking vs Dynamic Linking: Static linking incorporates the required libraries directly into your application, eliminating the need for separate redistributable packages. However, this increases the application size.
-
Selective Usage of Libraries: Only include the necessary libraries your application depends on. This minimizes bloat and enhances performance.
By following these strategies, developers can create more efficient deployments and streamline application installations for end users.
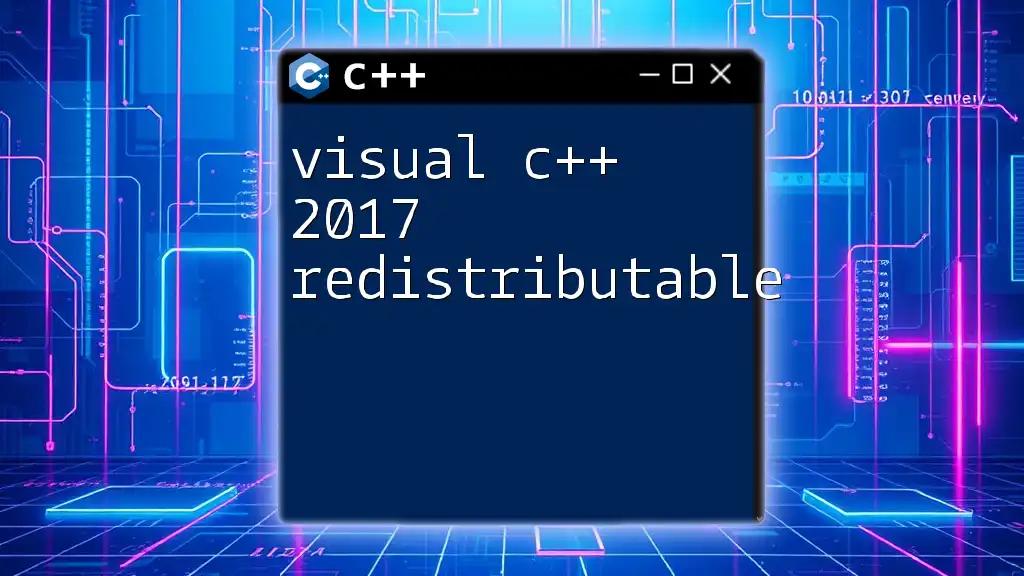
Conclusion
Visual Studio Redistributable C++ packages are integral for deploying C++ applications seamlessly. They ensure that end users have the necessary runtime components to run applications developed in Visual Studio efficiently. By understanding their importance, installation processes, common issues, and best practices, developers can leverage these redistributables to create robust and user-friendly applications.

Additional Resources
For further information, developers can check the official [Microsoft documentation](https://docs.microsoft.com/en-us/cpp/build/redistributable-package?view=msvc-160) for an in-depth understanding of Visual Studio C++ Redistributables. Additionally, exploring relevant books and online courses can provide more insights into effective C++ development and deployment techniques.