The Visual C++ 2019 Redistributable is a package required to run applications developed with Visual C++ that dynamically link to the C++ Runtime Libraries.
Here's a code snippet to illustrate how to check if the "Visual C++ 2019 Redistributable" is installed:
#include <windows.h>
#include <iostream>
bool IsVC2019RedistributableInstalled() {
HKEY hKey;
if (RegOpenKeyEx(HKEY_LOCAL_MACHINE,
TEXT("SOFTWARE\\Microsoft\\VisualStudio\\14.0\\VC"),
0,
KEY_READ,
&hKey) == ERROR_SUCCESS) {
RegCloseKey(hKey);
return true;
}
return false;
}
int main() {
if (IsVC2019RedistributableInstalled()) {
std::cout << "Visual C++ 2019 Redistributable is installed." << std::endl;
} else {
std::cout << "Visual C++ 2019 Redistributable is not installed." << std::endl;
}
return 0;
}
What is Visual C++ Redistributable?
The Visual C++ Redistributable is a package of runtime components required to run applications developed using Visual C++. Redistribution of the libraries ensures that your system has the necessary files to execute a C++ application, regardless of whether Visual Studio is installed on the machine. This is crucial in maintaining software consistency and reducing dependency issues across various environments.
Key Features
The Visual C++ 2019 Redistributable comes equipped with several features:
-
Support for Various Versions of Windows: It can operate seamlessly on multiple Windows versions, which means that applications built with Visual C++ can reach a broader audience without compatibility problems.
-
Compatibility with Multiple Visual Studio Versions: Designed to work with different Visual Studio configurations, this redistributable allows developers to create applications across various Visual Studio releases without worrying about library mismatches.
-
Enhanced Application Performance: By including optimized libraries that applications can leverage, the redistributable improves execution speed and reliability.
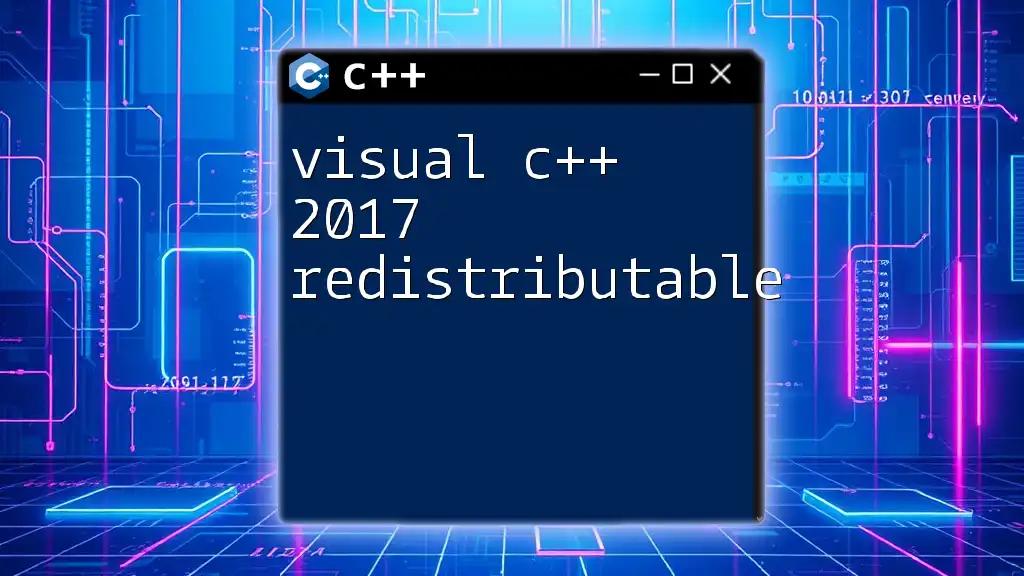
Installation of Visual C++ 2019 Redistributable
System Requirements
Before installation, make sure your system meets the minimum requirements:
- Operating Systems: Windows 10, Windows 8.1, Windows 8, Windows 7 SP1, and Windows Server 2008 R2 or later.
- Architecture: Choose between x86 (32-bit) and x64 (64-bit) based on your application's architecture.
Step-by-Step Installation Guide
Downloading the Redistributable: Navigate to the official Microsoft website to find the Visual C++ 2019 Redistributable download links. Depending on your application's architecture, select either the x86 or x64 version.
Installation Process: Once downloaded, run the installer and follow these steps:
- Accept the terms of the license agreement.
- Choose the installation location (default is usually suitable).
- Click on the install button and wait for the process to complete.
You may experience a progress bar indicating successful installation.
Common Installation Issues
During installation, you may encounter some error messages. Here are a few common issues and their solutions:
- Error 1603: A generic error indicating a failure during installation. Check for folder permissions or conflicts with existing installations.
- Missing Dependencies: Sometimes required components may not be installed. Ensure that your Windows framework is updated.
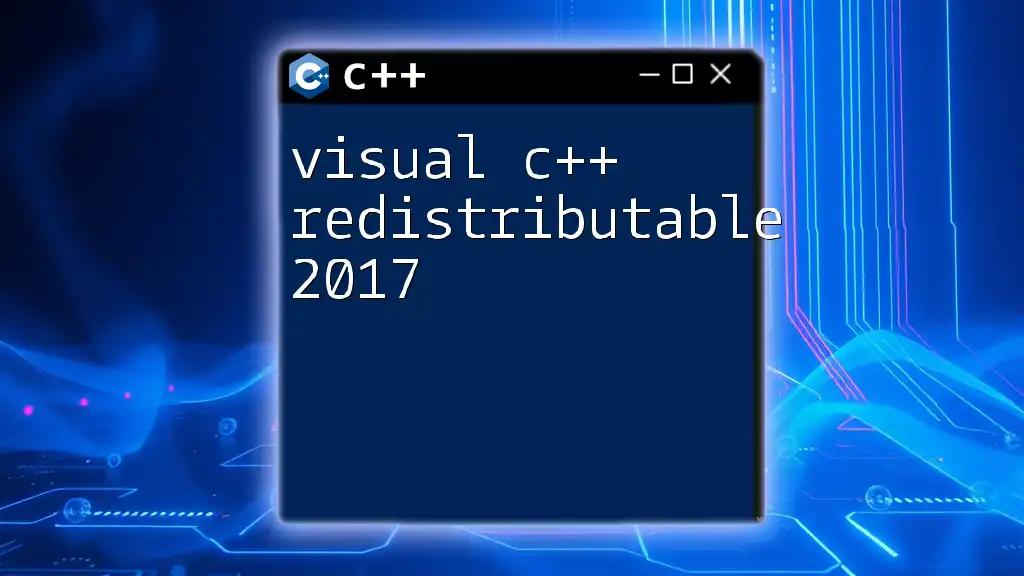
Understanding Visual C++ Redistributable Libraries
The Role of CRT, MFC, and ATL
The redistributable includes several critical components, notably:
-
C Runtime (CRT): This library is essential for managing memory, strings, and other tasks in C++. Without it, many applications would not function correctly as they rely heavily on these runtime functions.
-
Microsoft Foundation Classes (MFC): MFC is a powerful library that simplifies the development of Windows applications. It provides classes for handling Windows features effectively, which can significantly reduce development time and complexity. For instance, you can create a simple window with MFC in just a few lines of code.
-
Active Template Library (ATL): Useful for developing COM (Component Object Model) components, ATL provides a lightweight framework that allows for rapid application development.
How These Libraries Affect Performance
Optimizing application performance is essential, and using these libraries can significantly impact this. By leveraging the built-in functions provided by the redistributable, developers can decrease the overall application size while improving execution speed.
For example, MFC provides efficient drawing operations that can replace complex coding methods, while ATL allows for streamlined communication between COM components, enhancing responsiveness.
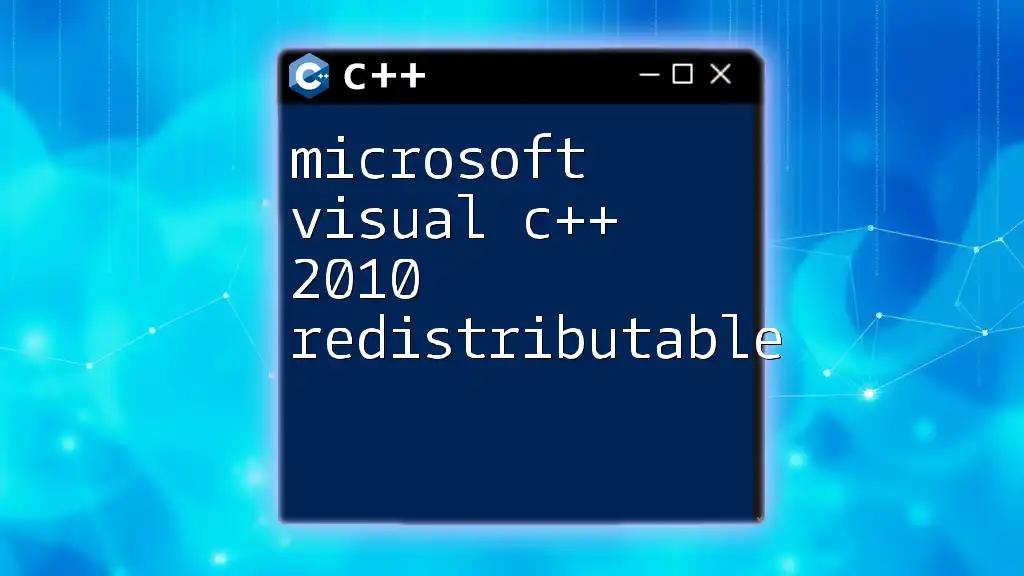
How to Verify the Installation
Checking the Installed Version
To verify that you have successfully installed the Visual C++ 2019 Redistributable, follow these steps:
- Go to the Control Panel.
- Click on "Programs and Features."
- Look for "Microsoft Visual C++ 2019 Redistributable" in the list. The version number will indicate which version you have installed.
Alternatively, you can check via the command prompt by executing the following command:
wmic product get name, version
This command will list all installed applications along with their version numbers, allowing you to confirm the installation.
Uninstalling Visual C++ 2019 Redistributable
If you need to uninstall the redistributable for any reason, follow these steps:
- Open Control Panel.
- Click on "Programs and Features."
- Find "Microsoft Visual C++ 2019 Redistributable" and select it.
- Click on "Uninstall."
Be cautious when uninstalling, as removing this package may impact applications that depend on it.
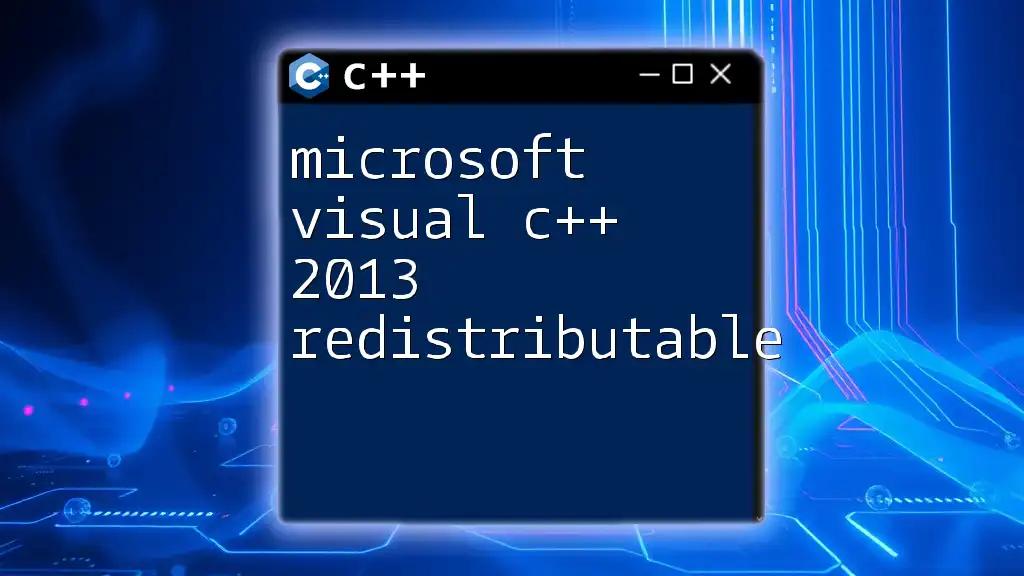
Practical Examples
Application Development Scenarios
To illustrate the role of the Visual C++ 2019 Redistributable in development, consider a simple C++ application:
#include <iostream>
int main() {
std::cout << "Hello, Visual C++ 2019 Redistributable!" << std::endl;
return 0;
}
This small program demonstrates how you can compile and execute a basic C++ application. When you run the application on a system without the redistributable installed, it will fail to execute, showcasing the necessity of having it present in the environment.
Debugging Common Issues
While developing with C++, you might encounter compile-time errors due to missing libraries that the redistributable provides. For example, you may see errors related to CRT functions when trying to compile code that requires this library.
#include <cstdlib>
int main() {
int* arr = (int*) malloc(10 * sizeof(int)); // Crashes without CRT!
return 0;
}
This basic code snippet highlights how crucial it is to have the C Runtime Library (CRT) installed; otherwise, memory management functions won’t operate correctly, leading to runtime crashes.

Best Practices
Keeping Your Redistributables Updated
Software evolves, and so do its dependencies. To ensure seamless operation and security, it's vital to keep your redistributable updated. Regularly check for updates from Microsoft's official site.
Choosing the Right Version for Your Application
When deploying applications, carefully assess which version of the redistributable is necessary. Applications built with different versions of Visual Studio may require specific Redistributable versions to function correctly. Consider packaging the appropriate version along with your application for user convenience.
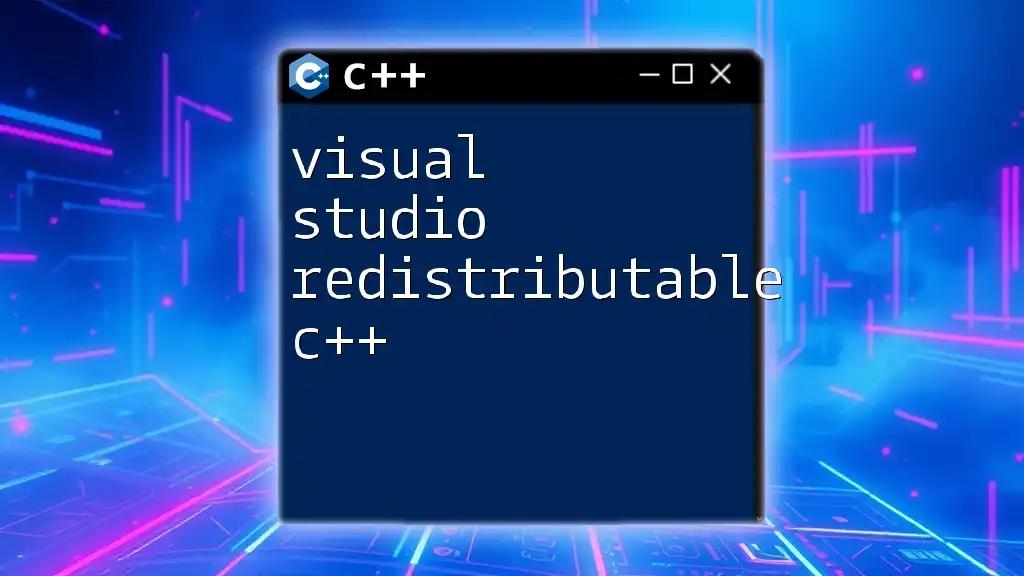
Conclusion
The Visual C++ 2019 Redistributable is a fundamental component in the ecosystem of C++ application development. By understanding its purpose, installation process, and best practices, developers can ensure smoother application deployments and a better experience for end users. Whether you are developing simple applications or complex software, the redistributable plays a vital role in ensuring that your applications run smoothly and efficiently.
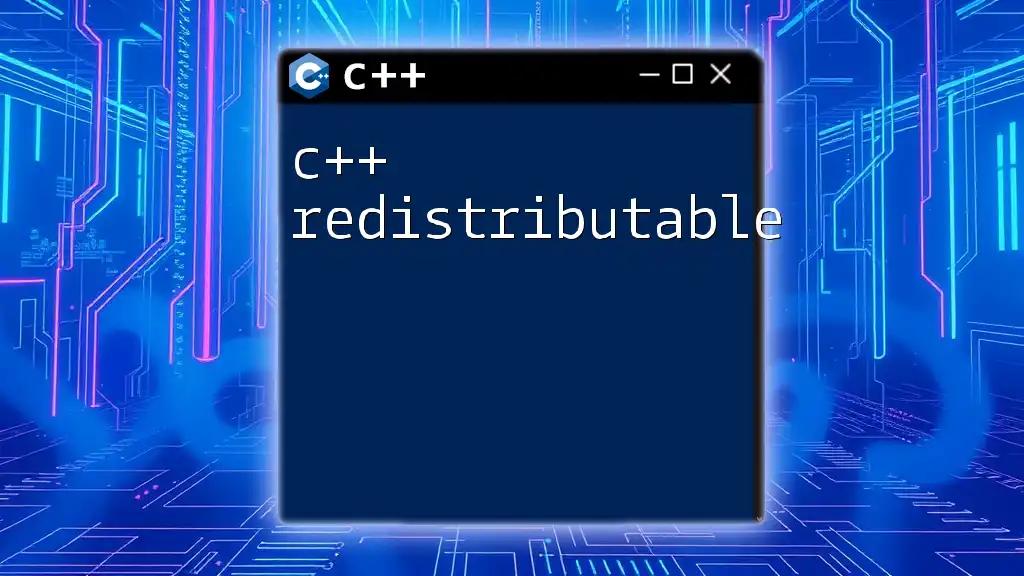
Additional Resources
For further reading and resources, refer to the official Microsoft documentation on the Visual C++ Redistributable. Engaging with community forums, such as Stack Overflow, can also provide insights and troubleshooting assistance from experienced developers.
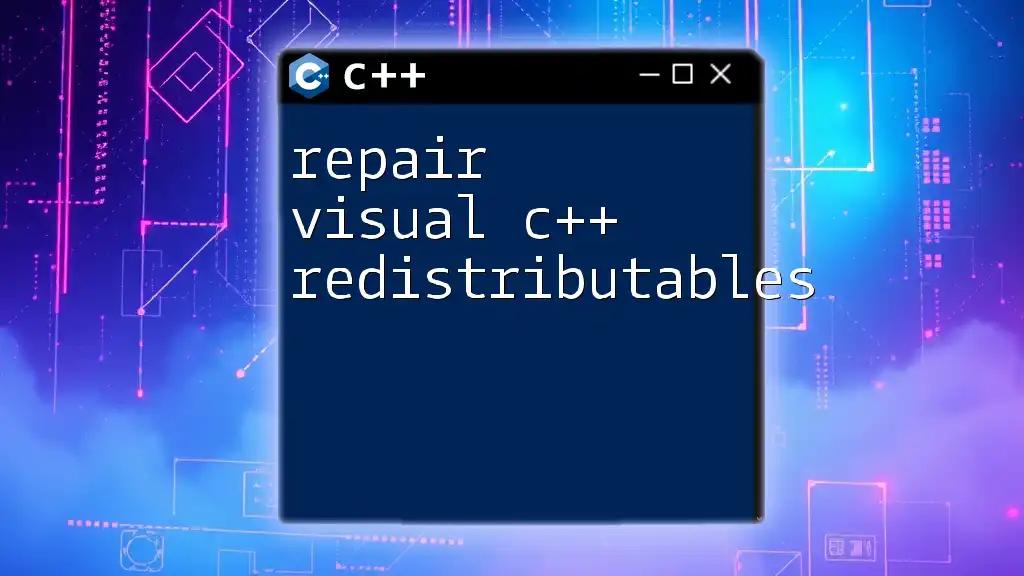
Call to Action
If you haven't already done so, be sure to download the Visual C++ 2019 Redistributable. If you have any questions or feedback, please share your thoughts in the comments section; we are here to help you continue your learning journey in C++ development.