The Microsoft Visual C++ 2013 Redistributable is a package that installs runtime components required to run C++ applications built with Visual Studio 2013.
Here's a simple code snippet demonstrating how to check for the installation of a specific Visual C++ Redistributable in a C++ program:
#include <iostream>
#include <windows.h>
int main() {
HKEY hKey;
if (RegOpenKeyEx(HKEY_LOCAL_MACHINE,
L"SOFTWARE\\Microsoft\\VisualStudio\\12.0\\VC\\Runtimes\\x86",
0,
KEY_READ,
&hKey) == ERROR_SUCCESS) {
std::cout << "Microsoft Visual C++ 2013 Redistributable is installed." << std::endl;
RegCloseKey(hKey);
} else {
std::cout << "Microsoft Visual C++ 2013 Redistributable is not installed." << std::endl;
}
return 0;
}
What is Microsoft Visual C++ 2013 Redistributable?
The Microsoft Visual C++ 2013 Redistributable is a runtime package that enables applications developed using Microsoft Visual Studio 2013 to run on a target machine. This package contains important C++ library files that applications depend on for execution.
Benefits
One of the primary benefits of using redistributable packages is that they simplify the deployment of C++ applications. By bundling all the necessary components together, developers ensure that users can run their software without requiring additional installations. The use of redistributables also guarantees a consistent runtime environment across different machines, which helps avoid compatibility issues.
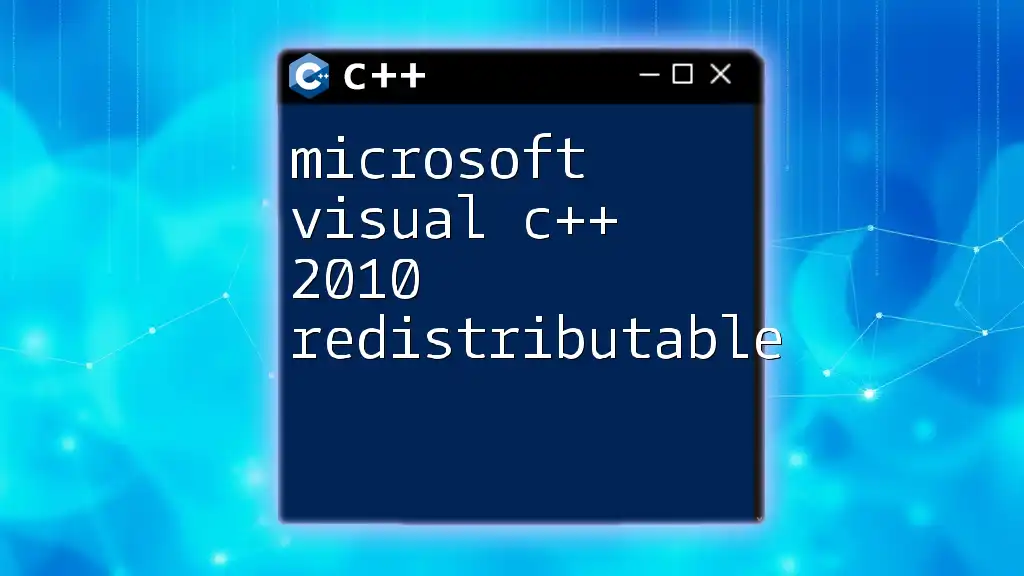
Why Do You Need the Microsoft Visual C++ 2013 Redistributable?
Common Scenarios
Applications that are developed using Visual Studio 2013 will often require the Visual C++ 2013 Redistributable to operate correctly. This includes software that directly relies on C++ libraries, as well as third-party applications that depend on those libraries being installed on the system. Without the required redistributable, users may encounter roadblocks that prevent successful application launches.
Issues Without Redistributables
Failing to install the Microsoft Visual C++ 2013 Redistributable may lead to a variety of problems. Users might see error messages indicating missing DLL files or incomplete components. In severe cases, applications may completely fail to start, leading to frustration and diminished user experience.
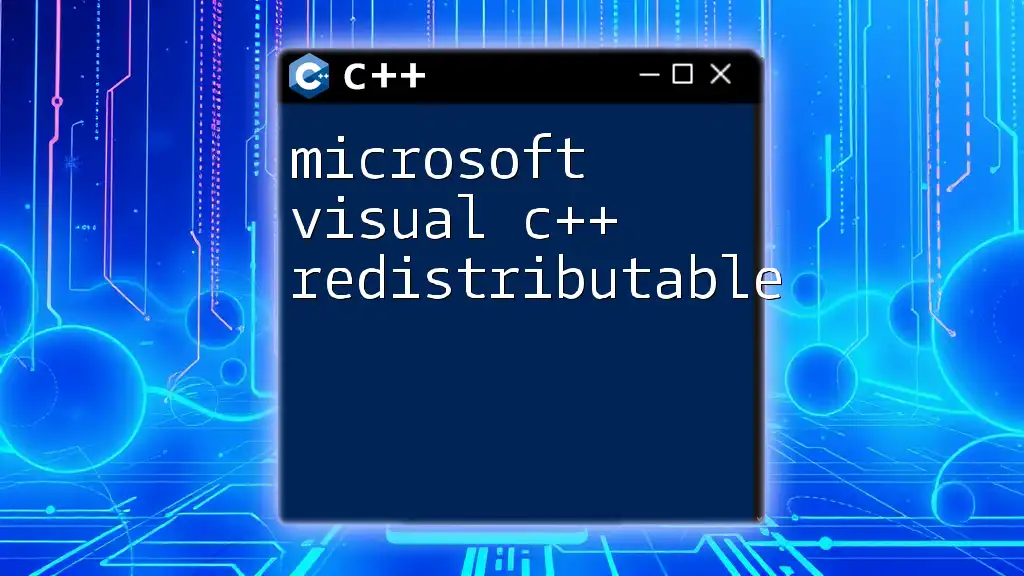
Key Features of Microsoft Visual C++ 2013 Redistributable
Versions Available
The Microsoft Visual C++ 2013 Redistributable comes in both x86 (32-bit) and x64 (64-bit) versions. This allows developers to target both 32-bit and 64-bit systems, which is particularly important for ensuring compatibility with a wider audience.
Compatibility
This redistributable is compatible with various versions of Windows, particularly Windows 7, Windows 8, Windows 8.1, and Windows 10. Furthermore, it offers backward compatibility with older redistributables, which means it can work alongside earlier versions without conflict.
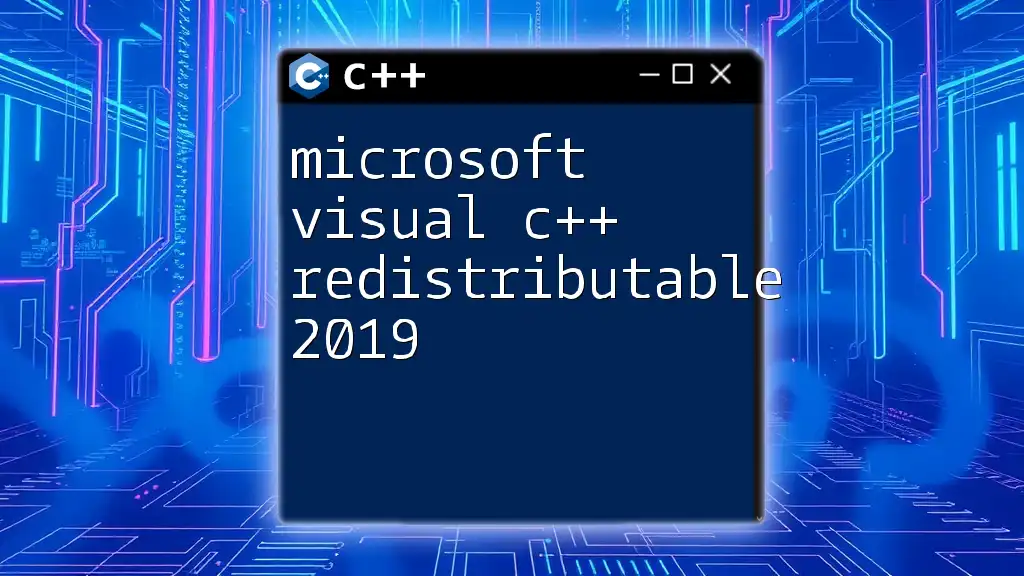
How to Install Microsoft Visual C++ 2013 Redistributable
Downloading the Package
To download the Microsoft Visual C++ 2013 Redistributable, navigate to the official Microsoft website. Once there, look for the section dedicated to downloads and locate the specific redistributable package.
Installation Process
For Windows Users
The installation process is straightforward. After downloading the package, double-click the installer file and follow the on-screen prompts. Accept the EULA (End User License Agreement) to continue, and the setup will proceed to install the necessary components.
Silent Installation for Advanced Users
For users comfortable with the command line, you can perform a silent installation using the following command:
vc_redist.x64.exe /install /quiet /norestart
This command allows you to install the redistributable without any user interaction, ideal for deploying via scripts or in enterprise environments.
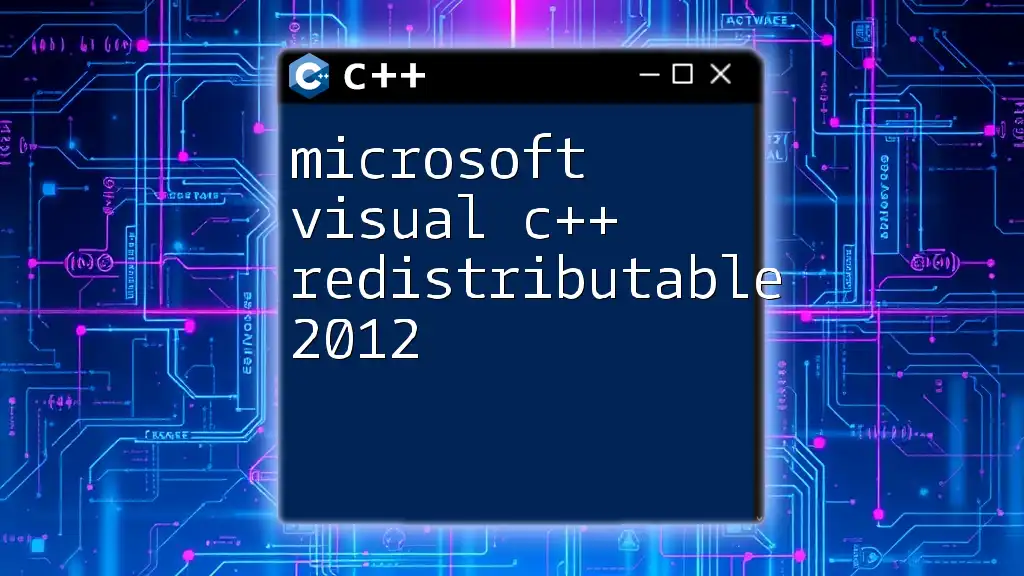
Troubleshooting Common Issues
Installation Errors
Users may encounter errors during installation, such as error code 0x80070005, which typically indicates a permissions issue. Ensure that you have the necessary administrative privileges to install software on the machine.
Runtime Errors
Regarding runtime errors, one common issue is applications failing to launch due to missing shared DLLs. Familiarize yourself with common error messages and guidelines on diagnosing and resolving these issues.
Reinstallation Tips
If problems persist, uninstall the redistributable from the Control Panel and reinstall it. Make sure to remove all versions that may conflict, ensuring a clean slate for the installation.
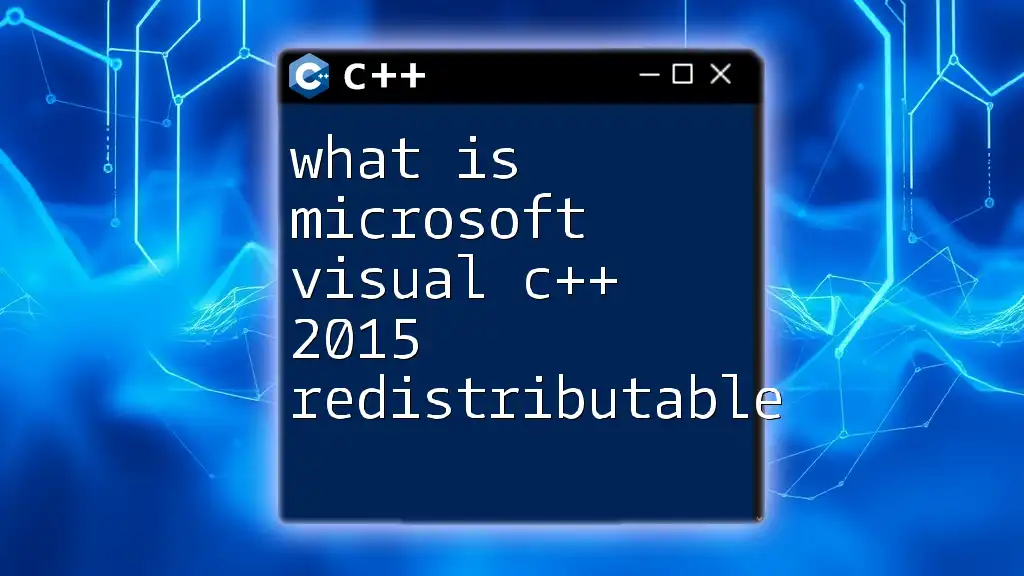
Best Practices When Working with Microsoft Visual C++ 2013 Redistributable
Version Management
It’s crucial to keep track of the various redistributable versions you have installed on your system. Regular updates and management can prevent compatibility issues when running applications. Consider maintaining a log of installations to streamline troubleshooting.
Testing and Verification
Always test the installation of the Microsoft Visual C++ 2013 Redistributable after completion. You can verify success by running applications that depend on it. If the software runs without error, it’s likely that the installation was successful.
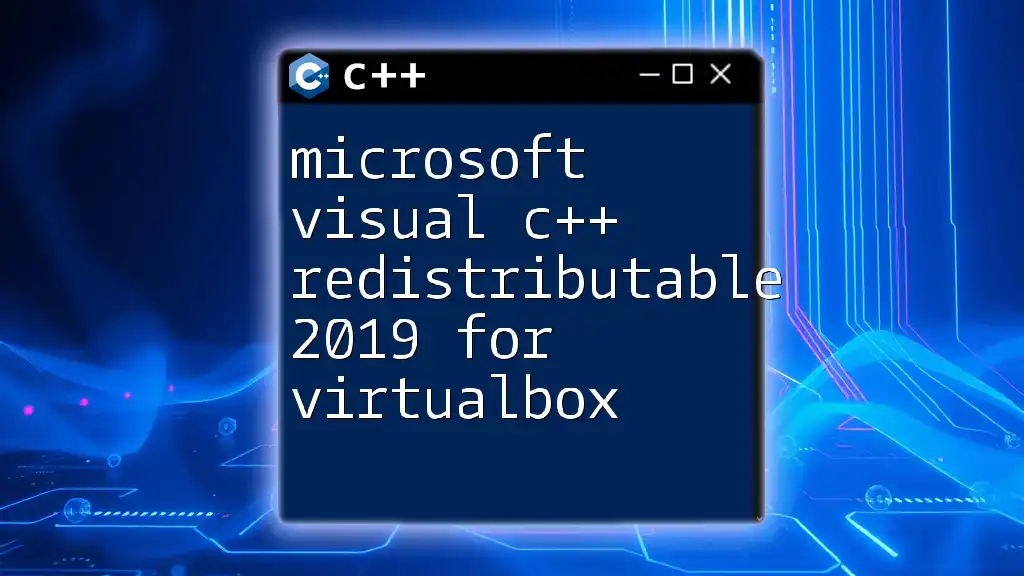
Examples of Applications Using Microsoft Visual C++ 2013 Redistributable
Many popular applications require the Microsoft Visual C++ 2013 Redistributable to function properly. For instance, game development engines, design software, and various utilities rely on specific runtime libraries for their operation. By understanding the software that utilizes these libraries, developers can better prepare for ensuring compatibility across installations.
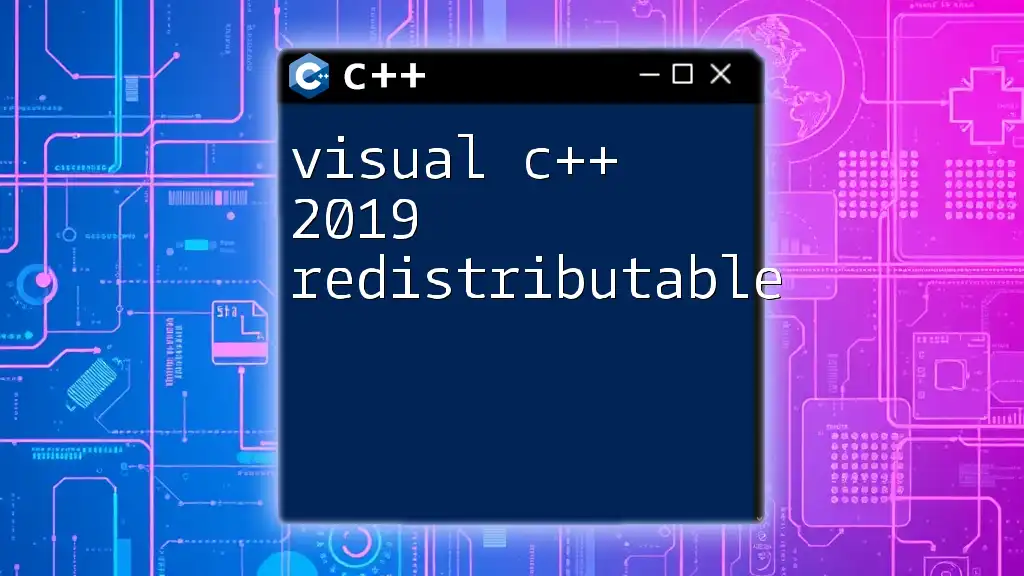
Conclusion
In summary, the Microsoft Visual C++ 2013 Redistributable is an essential component for executing many applications built with Visual Studio 2013. Understanding its purpose, how to install it, and common practices associated with its use will greatly enhance your ability to manage and deploy C++ applications effectively.
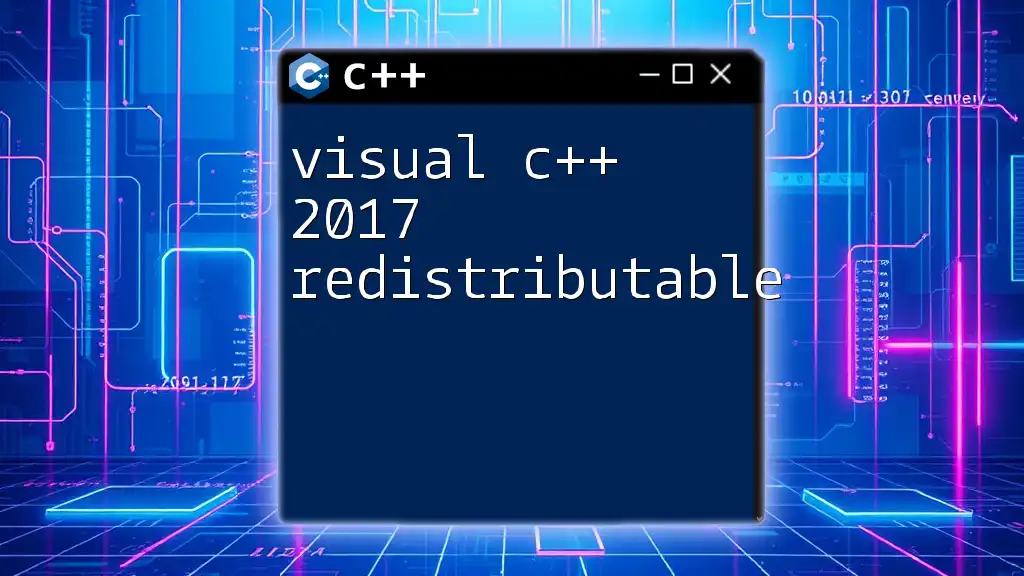
Additional Resources
For further reading and a deeper dive into installation and troubleshooting, consider exploring the official Microsoft documentation. Community forums like Stack Overflow and the Microsoft Tech Community are also excellent resources for seeking help and sharing knowledge with fellow developers.
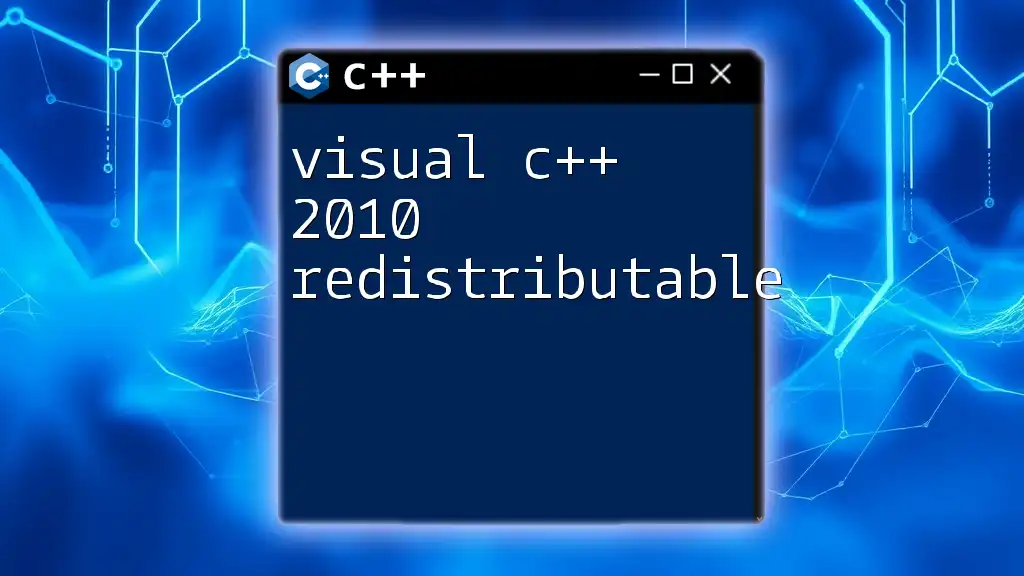
Call to Action
We encourage you to share your experiences and insights regarding the Microsoft Visual C++ 2013 Redistributable. If you have tips or techniques related to its use, please join the conversation! Follow us for more valuable C++ tips and guides!