The Microsoft Visual C++ Redistributable 2019 is a runtime package that installs the necessary components required to run applications developed with Visual C++ 2019.
Here's a code snippet to check if the redistributable is installed on your system:
#include <iostream>
#include <windows.h>
int main() {
HKEY hKey;
if (RegOpenKeyEx(HKEY_LOCAL_MACHINE,
TEXT("SOFTWARE\\Microsoft\\VisualStudio\\14.0\\VC\\Runtimes\\x64"),
0, KEY_READ, &hKey) == ERROR_SUCCESS) {
std::cout << "Microsoft Visual C++ Redistributable 2019 is installed." << std::endl;
RegCloseKey(hKey);
} else {
std::cout << "Microsoft Visual C++ Redistributable 2019 is not installed." << std::endl;
}
return 0;
}
Understanding Microsoft Visual C++ 2019
What is Microsoft Visual C++?
Microsoft Visual C++ (MSVC) is a crucial component of the Microsoft development suite. It's designed to help developers create Windows applications with an easy-to-navigate interface and powerful libraries. Offering a range of features, from advanced debugging tools to comprehensive libraries, MSVC streamlines the development process.
Overview of Microsoft Visual C++ 2019
The Microsoft Visual C++ 2019 version comes with several new features, enhancing both performance and usability:
- C++20 Support: Enhancements to modern C++ features make it easier to write efficient code.
- Improved Compiler: Faster compilation times and better error messages improve the developer experience.
- Enhanced Libraries: Updated standard libraries provide better performance and more functionalities.
- Compatibility: This version maintains compatibility with a wide variety of Windows operating systems, including Windows 7 through 10.
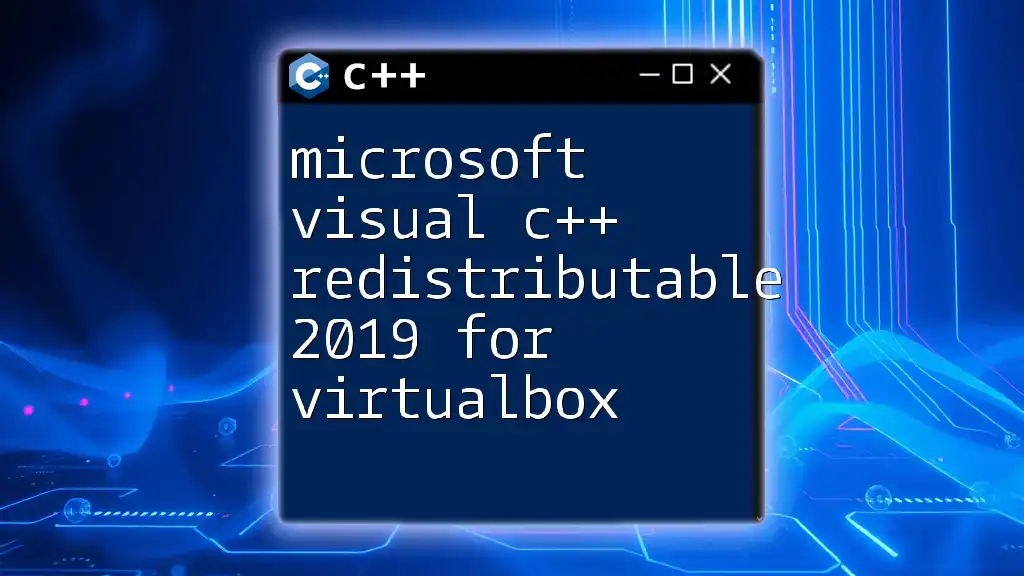
Microsoft Visual C++ 2019 Redistributable Package
What is the Redistributable Package?
The Microsoft Visual C++ Redistributable Package is essential for applications that are developed using Visual C++. When developers compile applications, the code often relies on external libraries included in the redistributable. This package ensures that end-users have the necessary components required for successful execution.
Components of the Microsoft Visual C++ 2019 Redistributable
The redistributable package consists of vital components that many applications depend upon:
- Run-time Libraries: These include essential C and C++ runtime libraries that various applications depend on for execution.
- Debugging and Profiling Tools: While typically not visible to the end-user, these tools enhance application performance and debugging capabilities.
- DLL Files: Dynamic Link Libraries that are necessary for running applications developed in Visual C++.
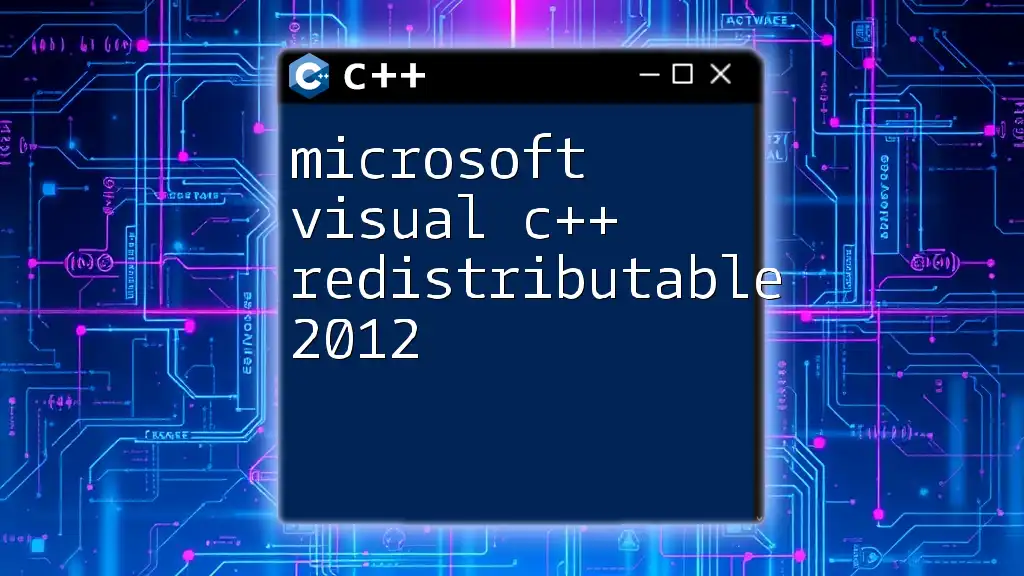
Downloading Microsoft Visual C++ 2019 Redistributable
How to Find the Right Version
It’s essential to choose the correct version: x64 (64-bit) or x86 (32-bit).
- Use x64 if your application is designed for 64-bit Windows.
- Opt for x86 for applications targeting 32-bit systems.
Though 64-bit systems can support both, ensuring you install the correct version prevents potential application errors.
Direct Download Links
You can obtain the Microsoft Visual C++ 2019 Redistributable Package directly from Microsoft's official website. The download links are separated by architecture:
- Microsoft Visual C++ 2019 Redistributable Package x64: Download from [Microsoft](https://support.microsoft.com/en-us/help/2977003/the-latest-supported-visual-c-downloads)
- Microsoft Visual C++ 2019 Redistributable Package x86: Download from the same link.
Follow these steps for seamless installation:
- Navigate to the download link.
- Select the appropriate version (x64 or x86).
- Click the download button and follow the on-screen instructions to complete the process.
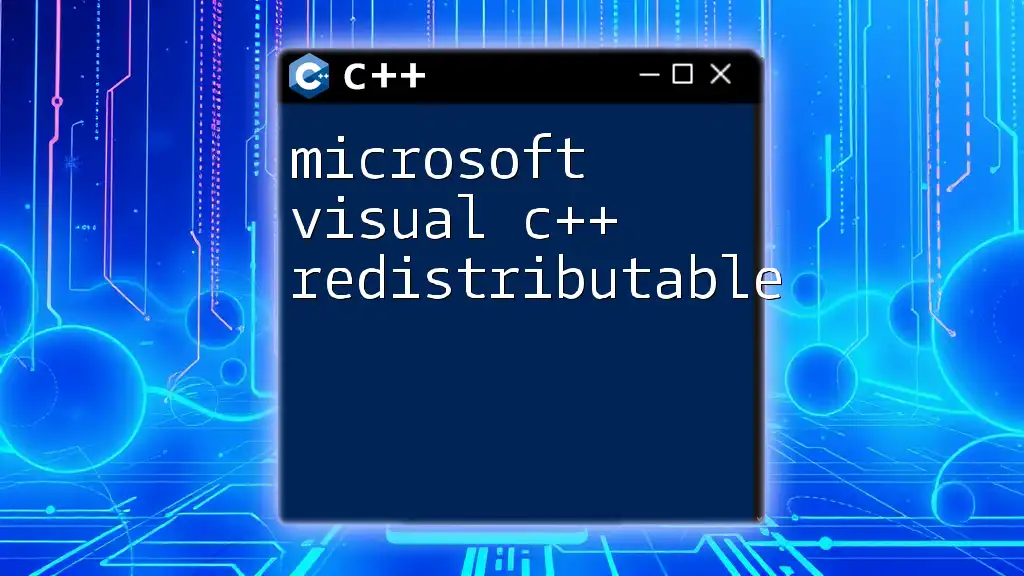
Installing Microsoft Visual C++ 2019 Redistributable
Installation Steps on Windows
Installing the redistributable is straightforward:
- Run the Installer: Locate the downloaded file and double-click to run it.
- Agree to the Terms: Follow the prompts to accept the Microsoft license terms.
- Choose Install Location: You can choose the default location or change it as per your requirements.
- Complete Installation: Click the "Install" button and wait for the process to finish.
Verifying Installation Success
After installation, verifying successful installation is crucial. To check if the redistributable is correctly installed:
- Open the Control Panel and navigate to Programs and Features.
- Search for Microsoft Visual C++ 2019 Redistributable in the list.
- The presence of the package implies successful installation.
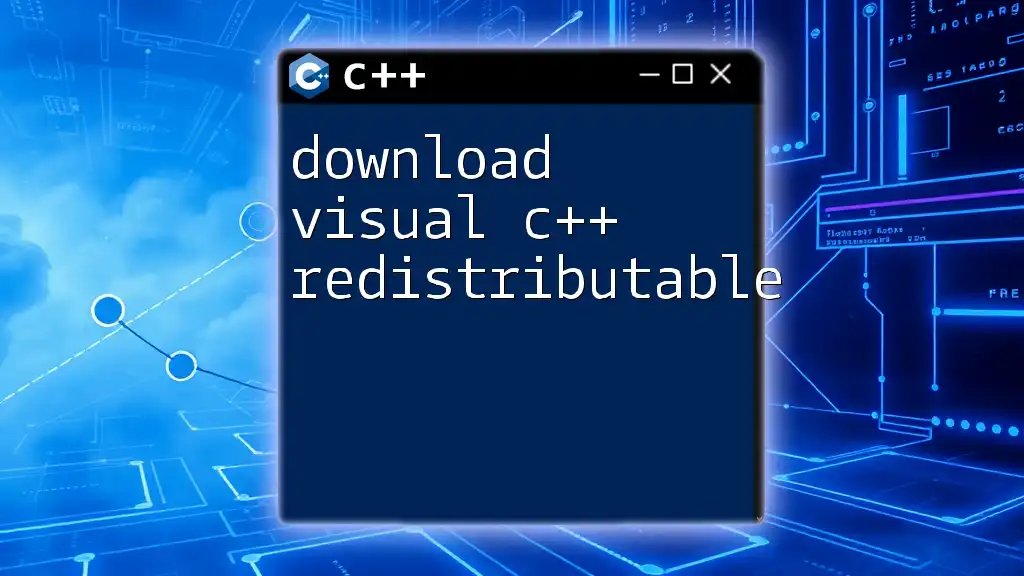
Programming with Visual C++ 2019
Setting Up Your Development Environment
To begin programming with Visual C++ 2019 effectively:
- Install Visual Studio: Ensure you have the appropriate version of Visual Studio that supports C++ development.
- Project Settings: Go to project properties and ensure the correct version of the redistributable is targeted in the configuration.
Sample Code Snippet
Here is a simple code snippet that utilizes standard output. This example demonstrates a console application that requires the runtime libraries provided by the redistributable:
#include <iostream>
int main() {
std::cout << "Hello, Microsoft Visual C++ 2019!" << std::endl;
return 0;
}
This simple program illustrates the essential functionality provided by the Visual C++ runtime. When executed, it will display a greeting message in the console.
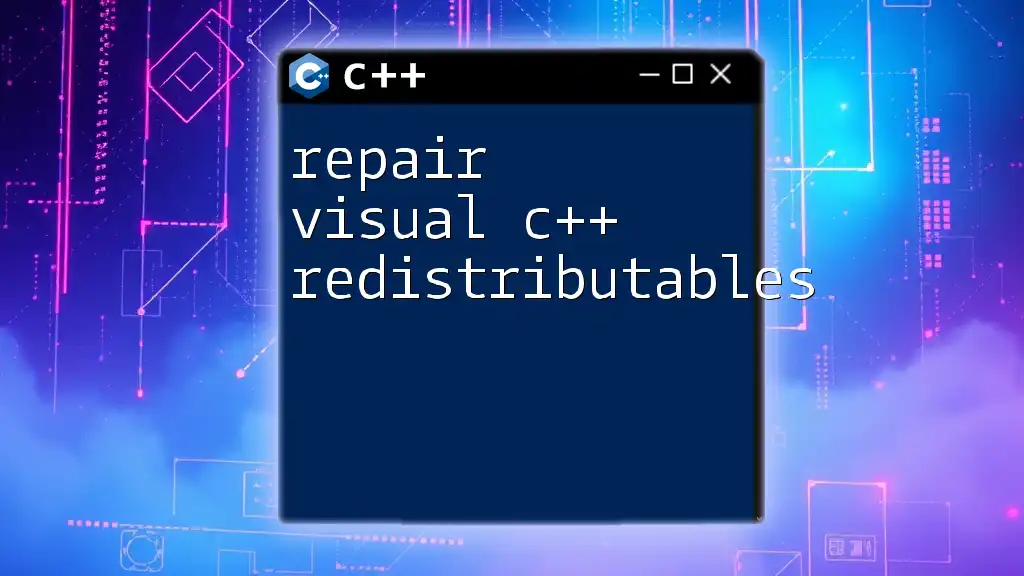
Common Issues with Microsoft Redistributables
Typical Errors Encountered
While working with the Microsoft Visual C++ redistributable, you might encounter some common issues:
- Missing DLL Errors: Indicate that the necessary redistributable is not installed.
- Compatibility Issues: Some applications may have specific version requirements, leading to errors if the wrong version is installed.
Best Practices for Developers
To mitigate problems associated with redistributables:
- Update Regularly: Keep redistributable packages updated to ensure compatibility and security.
- Follow Proper Packaging Guidelines: Include the necessary redistributables within your application installer, ensuring end-users have all required components.
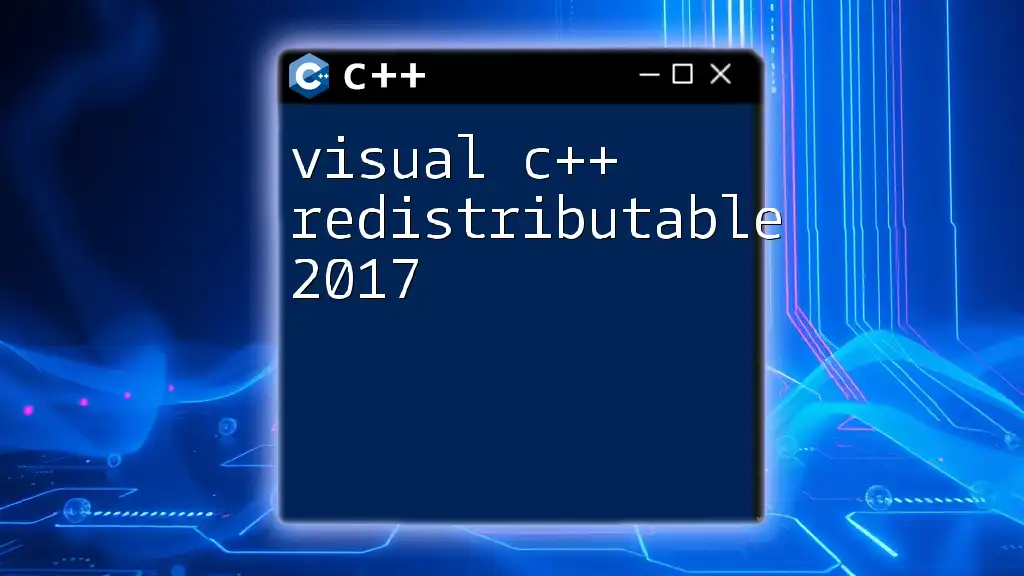
Benefits of Using Microsoft Visual C++ 2019 Redistributable
Performance Optimization
Using the Microsoft Visual C++ 2019 Redistributable can lead to significant performance enhancements. The updated run-time libraries are optimized for speed, reducing execution time and improving application responsiveness.
Enhanced Security
Regular updates to the redistributable packages help address vulnerabilities, improving the overall security of both applications and systems. This is especially critical in today’s landscape, where security is a paramount concern.
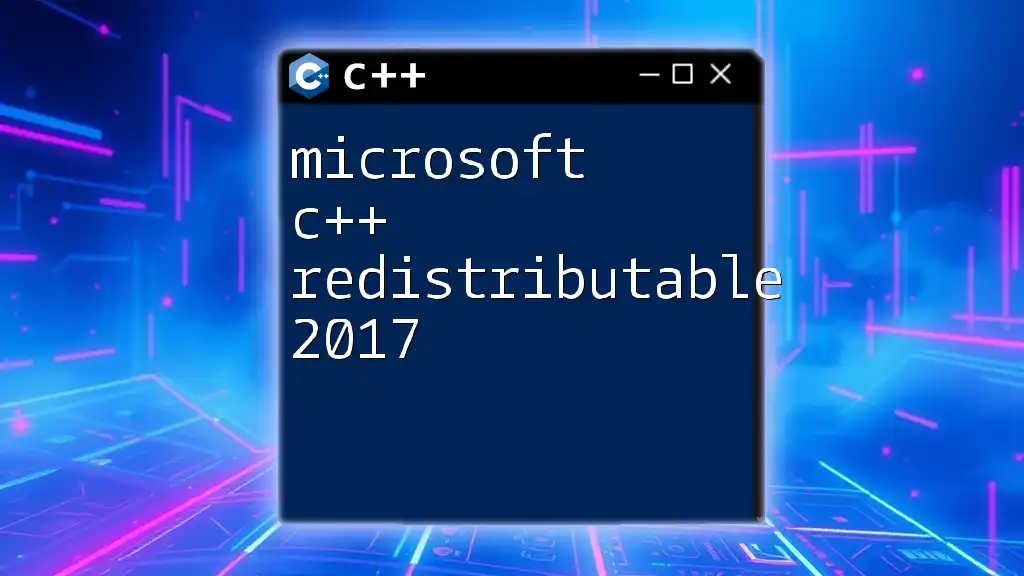
Conclusion
The Microsoft Visual C++ Redistributable 2019 is an essential tool for developers aiming to ensure their applications run seamlessly on user machines. Its proper installation and usage not only enhance application performance but also ensure compatibility across various Windows environments. For those looking to improve their C++ development skills, leveraging resources and tutorials on Visual C++ can be incredibly beneficial.
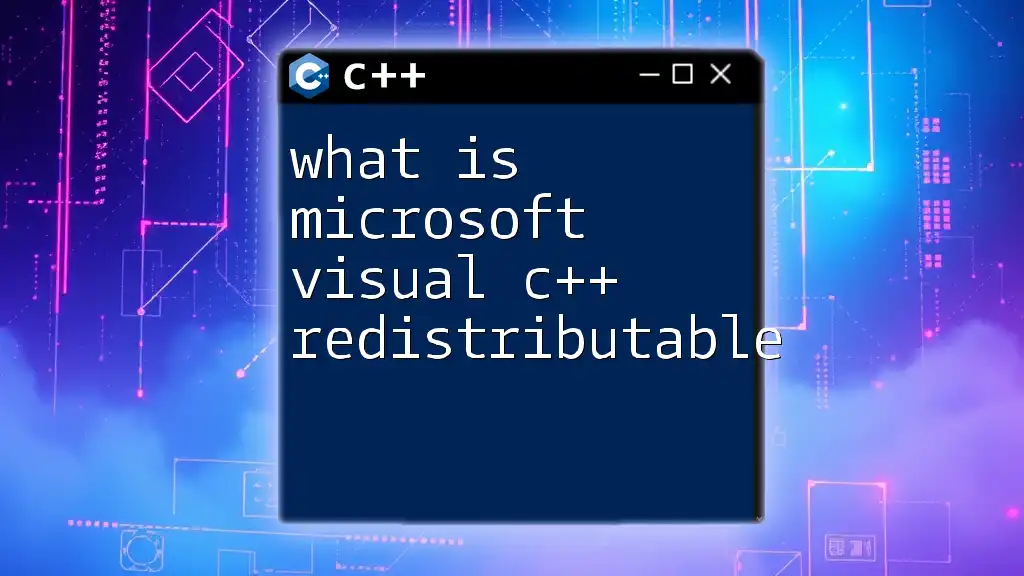
FAQ Section
What is the difference between x64 and x86?
The difference lies primarily in architecture. x64 is tailored for 64-bit systems, offering enhanced performance and memory capabilities, while x86 is meant for 32-bit systems, which limit the amount of memory an application can utilize.
How often should I update my redistributable packages?
Regular updates are encouraged, especially when significant enhancements or security patches are released. Keeping your redistributables up to date helps maintain application stability and security.
Can I redistribute the Redistributable package with my application?
Yes, you can include the redistributable package in your application installers. However, ensure that you comply with Microsoft’s licensing agreements regarding redistribution.
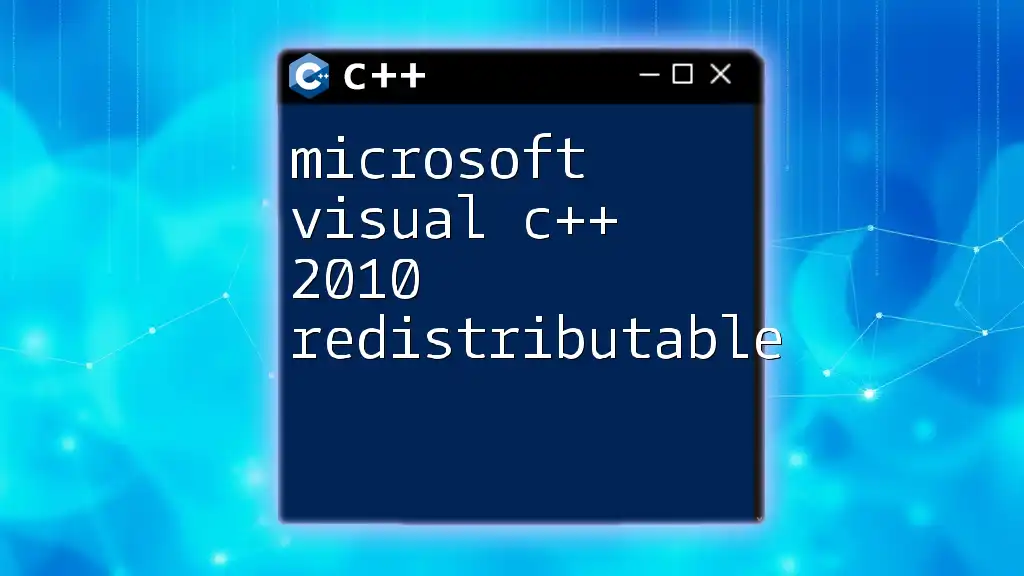
Additional Resources
For further exploration of Microsoft Visual C++ and its redistributable packages, consider visiting the official [Microsoft documentation](https://docs.microsoft.com/en-us/cpp/) as well as online platforms offering C++ tutorials and courses for in-depth learning opportunities.