Microsoft Visual C++ Runtimes are a collection of libraries required to run applications developed with Microsoft Visual C++, ensuring that C++ programs execute correctly on a Windows system.
Here’s a sample code snippet that demonstrates how to include the necessary headers in a simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What are Microsoft Visual C++ Runtimes?
Microsoft Visual C++ Runtimes are essential libraries that support the execution of applications developed using Microsoft’s Visual C++ development environment. These runtimes provide functions for performing various tasks essential to application operation, such as memory management, process control, and input/output operations. Understanding these runtimes is crucial for both developers and end-users, as missing or outdated runtime libraries can lead to application errors.
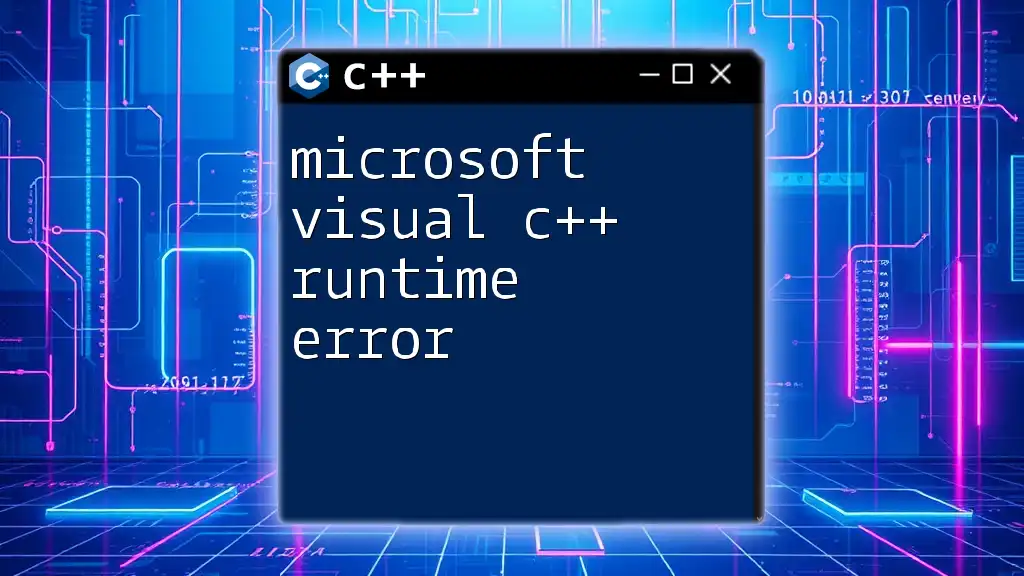
Understanding C++ Runtimes
Definition of C++ Runtimes
C++ runtimes refer to collections of libraries and components that provide the necessary runtime functions in the Visual C++ environment. These libraries enable developers to utilize a suite of common functions without having to implement them from scratch, thus facilitating efficient software development.
Why are Runtimes Necessary?
Runtime libraries are indispensable in executing compiled C++ applications. They handle essential services such as error handling, math computations, and standard input/output operations. Without these runtimes, applications may not function correctly or may even fail to run altogether, leading to frustrating experiences for users.
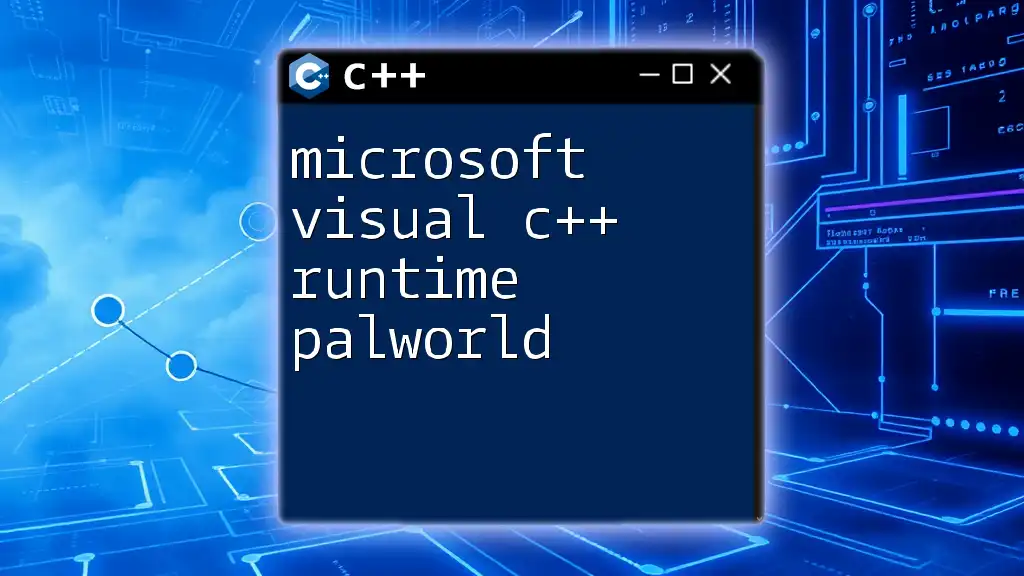
Components of Microsoft Visual C++ Runtimes
Core Runtime Libraries
The Microsoft Visual C++ Runtimes consist of several core libraries, each serving distinct purposes in application development.
-
C Runtime Library (CRT): This library includes fundamental functions for performing tasks like input/output operations, string manipulation, and mathematical computations. It is essential for any C++ application that performs basic operations.
-
Microsoft Foundation Classes (MFC): MFC provides a powerful set of classes for developing Windows applications. It streamlines the process of interacting with Windows APIs, making it easier to create graphical user interfaces and handle events.
-
Active Template Library (ATL): ATL simplifies the development of Component Object Model (COM) objects. It allows for efficient creation of reusable software components that can be integrated into various applications.
Different Versions of Visual C++ Runtimes
Microsoft Visual C++ Runtimes have evolved through numerous versions, corresponding to different releases of the Visual Studio IDE. Each version includes updates and enhancements that may not be backward compatible. Applications often depend on specific runtime versions, which means developers must ensure users have the correct runtimes installed. For example, a software application built with Visual Studio 2013 may require the Visual C++ Redistributable Packages for 2013, while applications made with Visual Studio 2019 will depend on a different set.
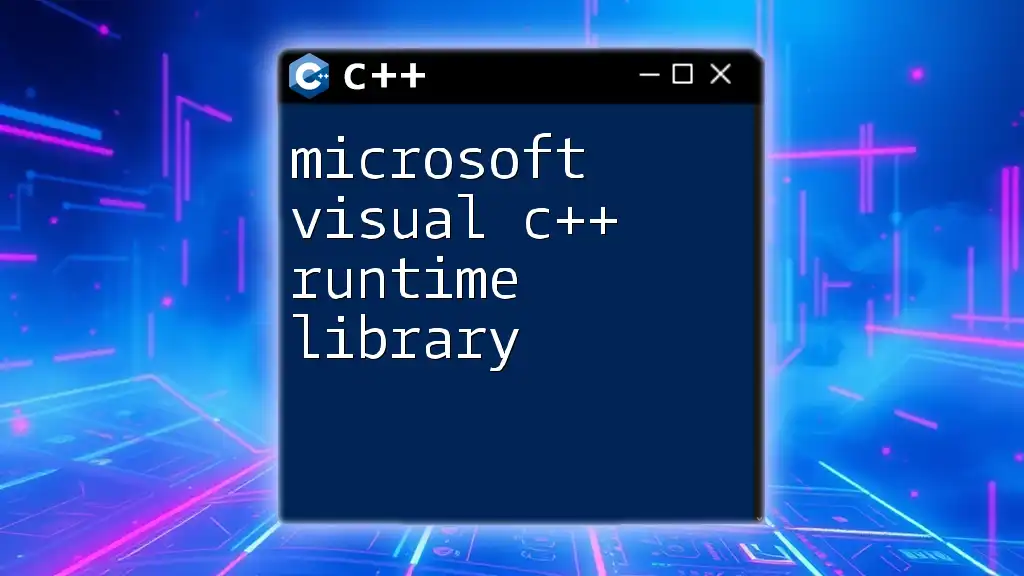
Installing Microsoft Visual C++ Runtimes
Where to Download Visual C++ Runtimes
The recommended source for downloading Microsoft Visual C++ Runtimes is the official Microsoft website. Here, users can find Redistributable Packages for various VC++ versions, which include all necessary libraries and components.
Installation Process
-
Downloading the Installer: Navigate to Microsoft's official site and locate the desired version of the Visual C++ Redistributable Package. Click on the download link.
-
Running the Installation Wizard: After downloading, run the installer. Simply follow the prompts of the installation wizard, which will guide you through the process.
-
Verifying Successful Installation: Once the installation completes, you can verify it by checking the "Programs and Features" section in the Windows Control Panel. Look for the installed version of the Visual C++ Redistributable Package.
Handling Installation Errors
Occasionally, users may encounter errors during installation. Common issues include corrupted downloads or conflicting older versions. In such cases, it's advisable to uninstall any previous versions of Visual C++ runtimes before attempting a new installation. Always ensure that your operating system is updated to avoid compatibility problems.
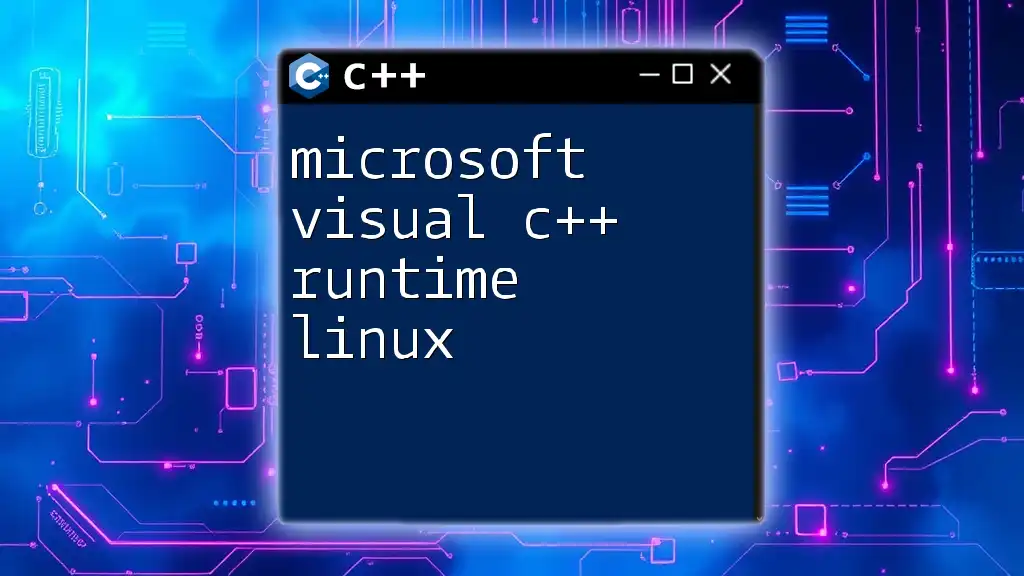
Using Microsoft Visual C++ Runtimes
Integrating Runtimes in Your C++ Projects
Integrating Microsoft Visual C++ Runtimes into your projects in Visual Studio is straightforward. When creating or configuring your C++ project, linking against the necessary runtime libraries is crucial for optimizing application performance. To do this:
- Open your project in Visual Studio, navigate to the project properties, and select the linker settings to ensure the CRT and any other libraries required (like MFC or ATL) are included.
Here is a simple example of a C++ program utilizing the standard C++ runtime functions:
#include <iostream>
int main() {
std::cout << "Hello, World!";
return 0;
}
Examples of Common Functions and Usage
Microsoft Visual C++ Runtimes offer a variety of functions that are essential for development. For instance, the CRT provides mathematical functions that can handle various data types.
Consider the following code snippet that demonstrates error handling using runtime libraries:
#include <cmath>
#include <iostream>
#include <cerrno>
int main() {
double result = sqrt(-1); // Generates domain error
if (errno == EDOM) {
std::cerr << "Error: Domain error!" << std::endl;
}
return 0;
}
In this example, we attempt to calculate the square root of a negative number, which will trigger a domain error. The program utilizes `errno` to check and handle the error gracefully.
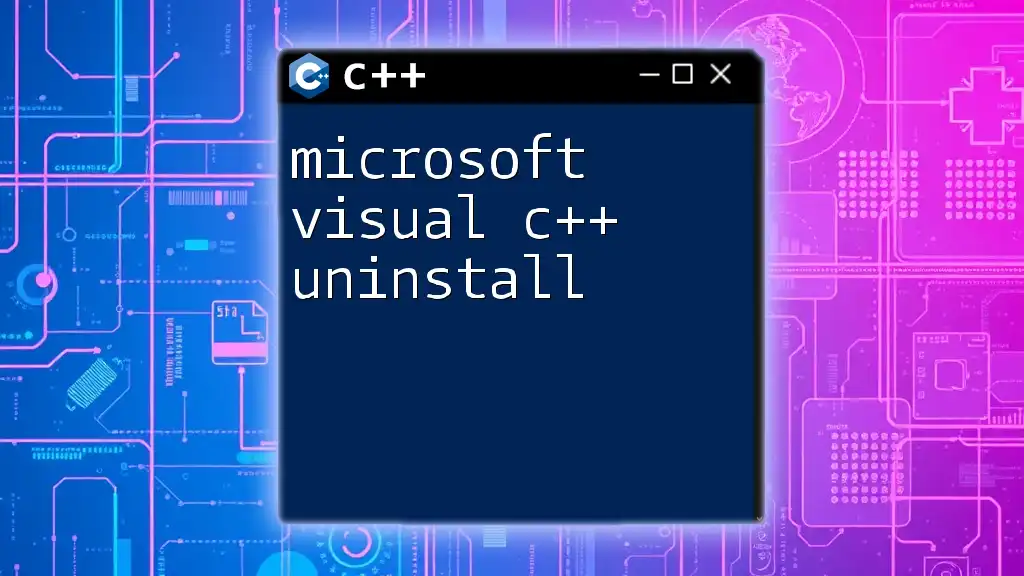
Troubleshooting Common Issues
Missing Runtime Errors
One of the most common pain points for users is encountering "missing runtime" errors, such as "MSVCR100.dll was not found." These errors occur when a required runtime library is missing from the system. To resolve this, users should download and install the appropriate Visual C++ Redistributable Package corresponding to the application they wish to run.
Updating Runtimes
Keeping runtimes updated is vital for maintaining application stability. Users can set Windows to automatically update Visual C++ runtimes through Windows Update. Alternatively, you can manually check for updates via the Microsoft website.
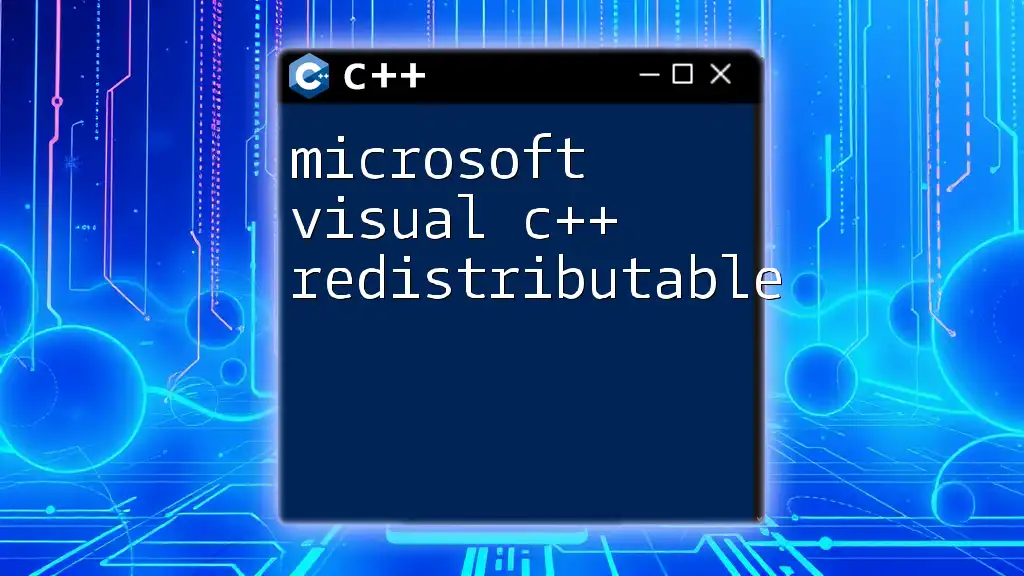
Advanced Topics
Custom Builds with Runtimes
When developing applications, developers can choose between static and dynamic linking of runtime libraries. Statically linked libraries become part of the application, making deployment simpler but potentially increasing file size. Conversely, dynamically linked libraries decrease application size but require the correct versions of the libraries to be present on the target machine. Weighing these trade-offs allows developers to optimize their applications based on the intended distribution and usage.
Cross-Platform Considerations
With the rise of cross-platform development frameworks, understanding how Microsoft Visual C++ Runtimes behave across different operating systems is essential. For instance, while the runtimes greatly simplify Windows development, portability issues can arise when deploying applications on non-Windows platforms. Developers should understand the differences in runtime behavior and ensure compatibility when targeting multiple platforms.
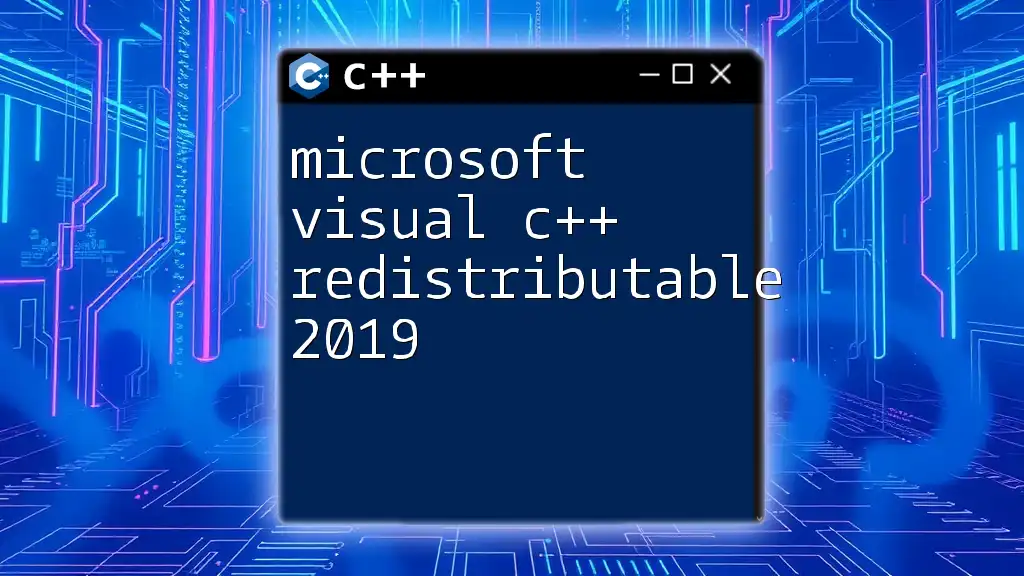
Conclusion
In summary, Microsoft Visual C++ Runtimes play a crucial role in the execution of C++ applications, offering vital functions that simplify the development process and enhance application performance. Understanding the significance of these runtimes, installing them correctly, and utilizing their capabilities within your projects will lead to more efficient and effective software development.
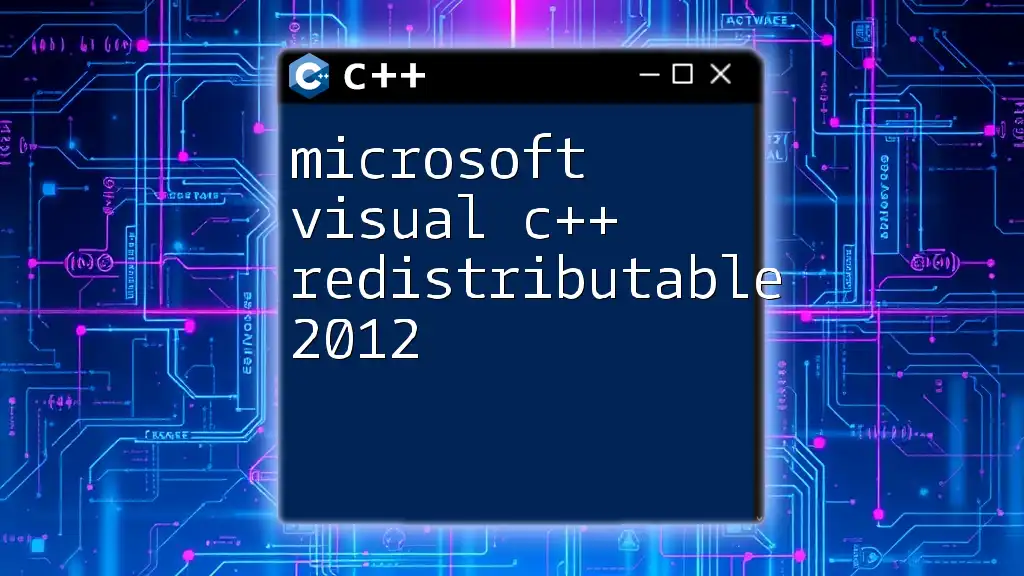
Additional Resources
For further learning, explore the official Microsoft documentation on Visual C++ and its runtimes. Additionally, numerous books and online tutorials are available that delve deeper into C++ programming concepts and techniques, aiding in the mastery of Microsoft Visual C++ Runtimes.
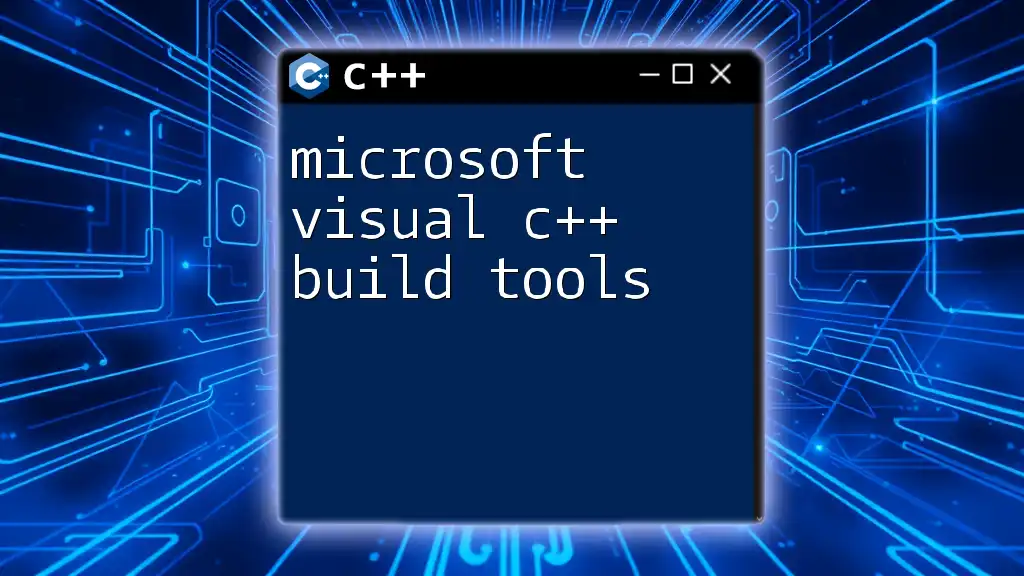
Call to Action
Have you encountered any challenges or successes with Microsoft Visual C++ Runtimes? We invite you to share your experiences, insights, and questions. Also, consider exploring our company services designed to help you master C++ commands quickly and efficiently. Whether you are an aspiring developer or a seasoned programmer, we're here to support your learning journey.