Microsoft Visual C++ 14.0 is a development environment that provides tools for creating C++ applications, supporting C++11 features and enabling efficient code compilation.
Here's a simple code snippet demonstrating a basic "Hello World" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Microsoft Visual C++ 14.0
System Requirements
Before installing Microsoft Visual C++ 14.0, it is essential to ensure that your system meets the necessary specifications. This version of Visual C++ generally requires:
- Processor: 1.8 GHz or faster (x86 or x64 processor)
- RAM: At least 2 GB for basic installation; 4 GB or more is recommended
- Disk Space: Approximately 10 GB of free space
- Operating System: Windows 7 SP1 or later (Windows 8.1 and 10 support is optimal)
Installation Guides
To begin using Microsoft Visual C++ 14.0, you need to download and install the Visual Studio Community Edition, which offers a free version suitable for individual developers and small teams.
- Download Visual Studio: Visit the official Visual Studio website to download the installer.
- Launch the Installer: Once downloaded, run the installer and select the Desktop development with C++ workload to configure the IDE for C++ programming.
- Configuration Options: During the installation process, you can customize the installation by selecting additional components such as MFC, Windows SDK, and .NET framework support if needed.
Using the Integrated Development Environment (IDE)
Navigating through Visual Studio is straightforward, thanks to its well-organized interface.
The Solution Explorer will provide you with a tree view of your projects, while the Properties Window allows you to configure various settings.
Creating Your First Project
To create your first C++ project in Microsoft Visual C++ 14.0, follow these steps:
- Open Visual Studio and select File > New > Project.
- Choose C++ from the language options.
- Select a console application template.
- Give your project a meaningful name and specify the location to save it.
- Click Create.
By following these steps, you will set up a basic C++ project ready for coding.
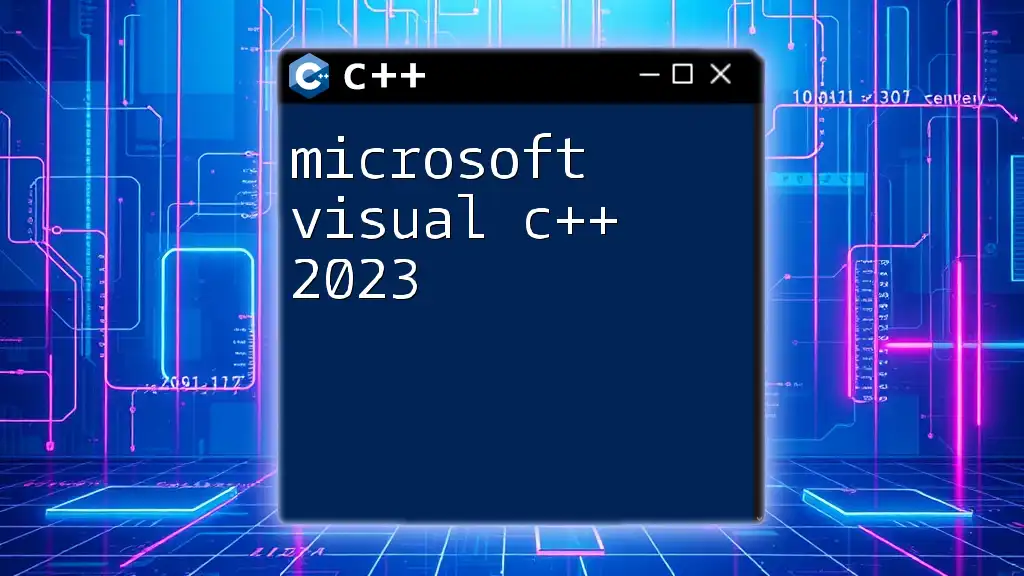
C++ Programming Fundamentals in Visual C++ 14.0
Writing Your First C++ Program
Let’s write a simple "Hello World" program to familiarize ourselves with C++ coding in Visual C++ 14.0.
Here’s your first C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code:
- `#include <iostream>` allows access to input/output streams.
- `std::cout` is used to print text to the console.
- The `main` function is the entry point of the program.
Debugging and Compiling
Understanding the build process is crucial for developing software efficiently.
The build process compiles your source code into executable format. When you click the Build button, Visual C++ 14.0 will compile your code and alert you to any errors or warnings.
Using the Debugger
Debugging is an essential skill in programming. Here’s how to use the debugging tools in Visual C++:
- To set a breakpoint, click on the left margin next to the line number in your code editor.
- Run your program in debug mode by pressing F5. The execution will pause at the breakpoints you set.
- Utilize step-through debugging with F10 (Step Over) and F11 (Step Into) to trace through your code and understand its flow better.
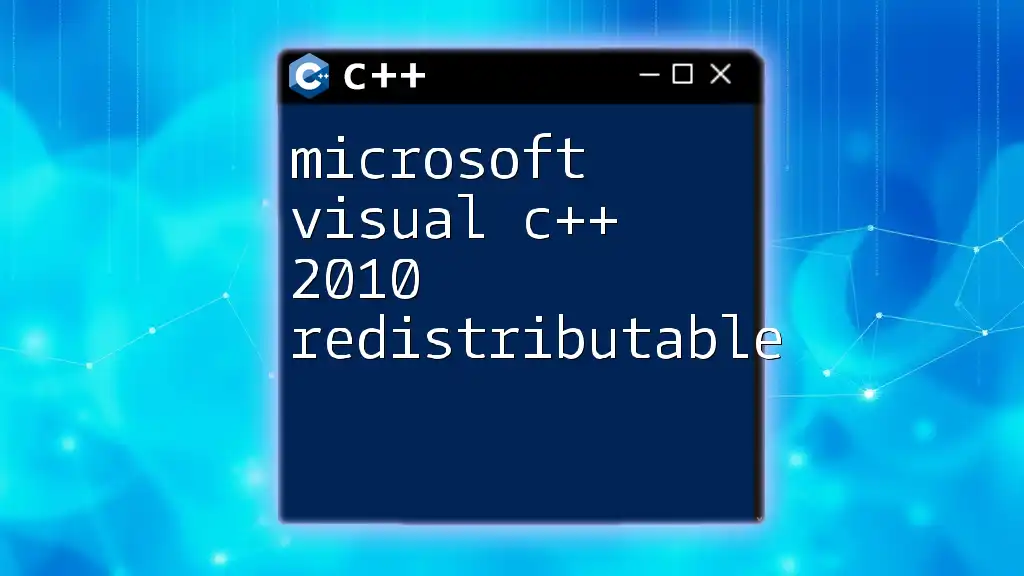
Advanced Features of Visual C++ 14.0
Standard Template Library (STL) Enhancements
Microsoft Visual C++ 14.0 offers extensive support for the Standard Template Library (STL), enabling easier data structure management and algorithms.
Code Example
Here’s an example demonstrating how to use a vector and sort it:
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 3, 5, 2, 4};
std::sort(numbers.begin(), numbers.end());
return 0;
}
In this example, we declare a vector of integers and utilize `std::sort` to sort the numbers in ascending order.
Multithreading Support
Multithreading is vital for improving the performance of applications, allowing them to execute multiple operations simultaneously.
Using the Thread Library
Here’s how to create and manage threads in Microsoft Visual C++ 14.0:
#include <iostream>
#include <thread>
void printMessage() {
std::cout << "Hello from a thread!" << std::endl;
}
int main() {
std::thread t(printMessage);
t.join(); // Wait for the thread to finish
return 0;
}
This snippet demonstrates how to create a thread that runs the `printMessage` function, showcasing how simple it is to implement multithreading.
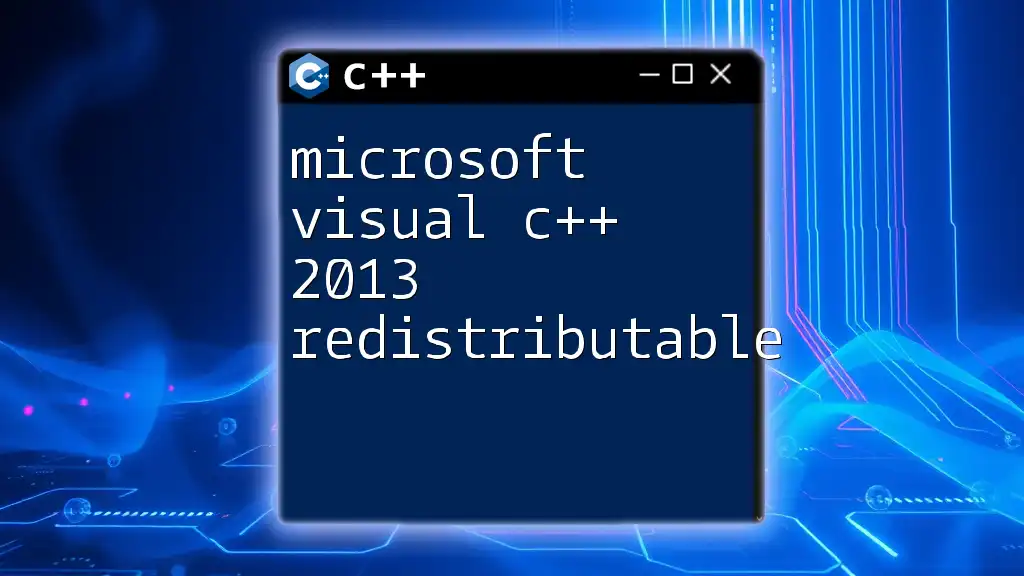
Best Practices for C++ Development in Visual C++ 14.0
Code Organization and Maintenance
Structuring your codebase efficiently will make a significant difference in long-term project maintainability.
- Project Structure: Utilize folders logically to categorize source files, headers, and resources. This will help in keeping your project organized.
- Documentation: Always document your code adequately, specifying the purpose of functions, classes, and crucial logic. This practice facilitates easier maintenance down the line.
Performance Optimization Tips
Optimizing your code enhances its efficiency and speed. Microsoft Visual C++ 14.0 offers various compiler optimization options you can leverage.
- Compiler Optimizations: Access optimization settings in your project properties. Select between Debug and Release configurations based on your development phase.
- Memory Management: Properly manage memory in C++ using smart pointers (like `std::unique_ptr` and `std::shared_ptr`) to enhance resource management and minimize memory leaks.
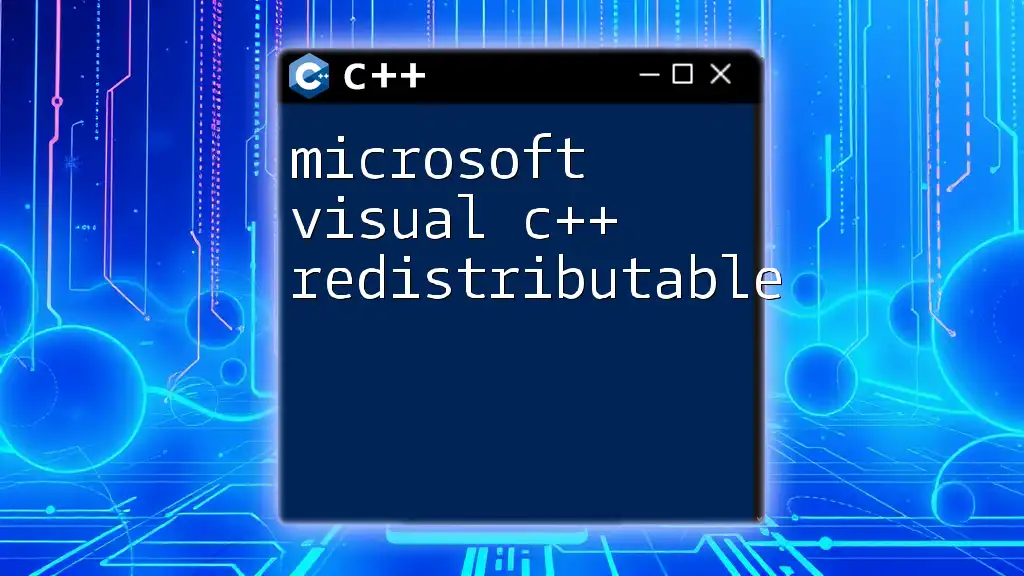
Common Issues and Troubleshooting
Debugging Toolset
While using Microsoft Visual C++ 14.0, developers may encounter various common errors and warnings.
Some frequent issues include:
- Compilation Errors: Syntax errors, unresolved references, or type mismatches.
- Linker Errors: Missing libraries or unresolved external symbols.
Community Resources
If you face challenges while working, don't hesitate to seek resources and community support. The Microsoft documentation provides extensive guidance, and platforms like Stack Overflow host vibrant communities willing to help.
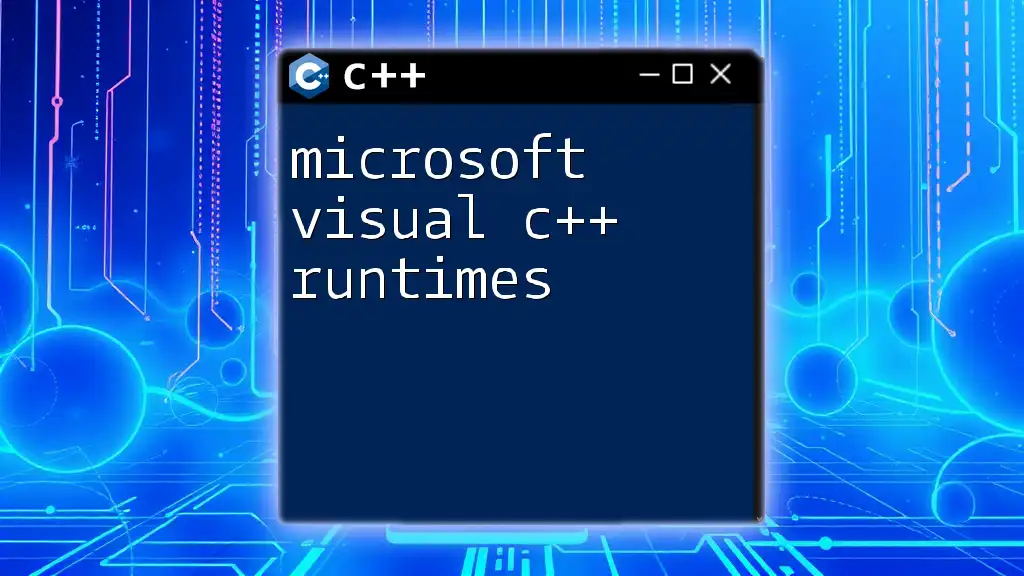
Conclusion
Mastering Microsoft Visual C++ 14.0 opens doors to countless opportunities in software development. This comprehensive guide highlights essential features, coding best practices, and troubleshooting techniques to enhance your C++ programming journey. Embrace the challenges, practice regularly, and experiment boldly to solidify your learning!
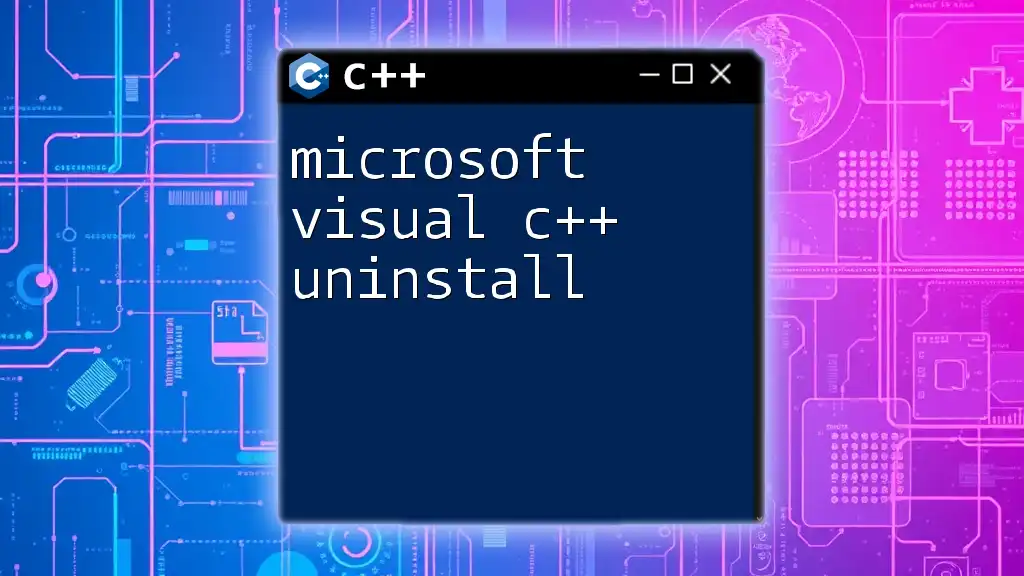
Additional Resources
To further enrich your understanding and skills, consider exploring recommended books on C++ programming to deepen your insights, as well as enrolling in online courses tailored for C++ enthusiasts. Engage with online forums to network with other developers and share knowledge, enhancing both your learning and professional development in Microsoft Visual C++ 14.0.