A Microsoft Visual C++ Runtime Error occurs when a program using the Microsoft C++ runtime library encounters an unexpected condition that disrupts the normal execution flow, typically resulting from issues like memory access violations or missing dependencies.
Here’s a simple code snippet demonstrating how to handle exceptions in C++:
#include <iostream>
int main() {
try {
// Code that may throw an exception
int* p = nullptr;
*p = 42; // This line will cause a runtime error
} catch (const std::exception& e) {
std::cerr << "Runtime error: " << e.what() << std::endl;
}
return 0;
}
Understanding Microsoft Visual C++ Runtime Errors
What is a Runtime Error?
A runtime error is an error that occurs during the execution of a program. Unlike compile-time errors, which prevent the program from compiling, runtime errors can occur only when the program is running. These errors can lead to unexpected behavior, such as crashes or incorrect output, often creating a frustrating experience for developers and users alike.
Common Causes: Runtime errors can arise from various sources, including:
- Logical errors: Flaws in the program's logic.
- Resource issues: Insufficient system resources, such as memory.
- Improper usage of language features: Misuse of pointers, arrays, or functions.
Types of Microsoft Visual C++ Runtime Errors
Microsoft Visual C++ runtime errors manifest through specific error codes that help diagnose the issue at hand. Some common error codes include:
- R6034: Indicates an application is trying to load the C runtime library incorrectly.
- R6025: Represents an attempt to use a pure virtual function, hinting at logic errors in the code.
Identifying the symptoms of these errors is essential, as they can vary from simple application crashes to unresponsive behavior, hindering user experience.
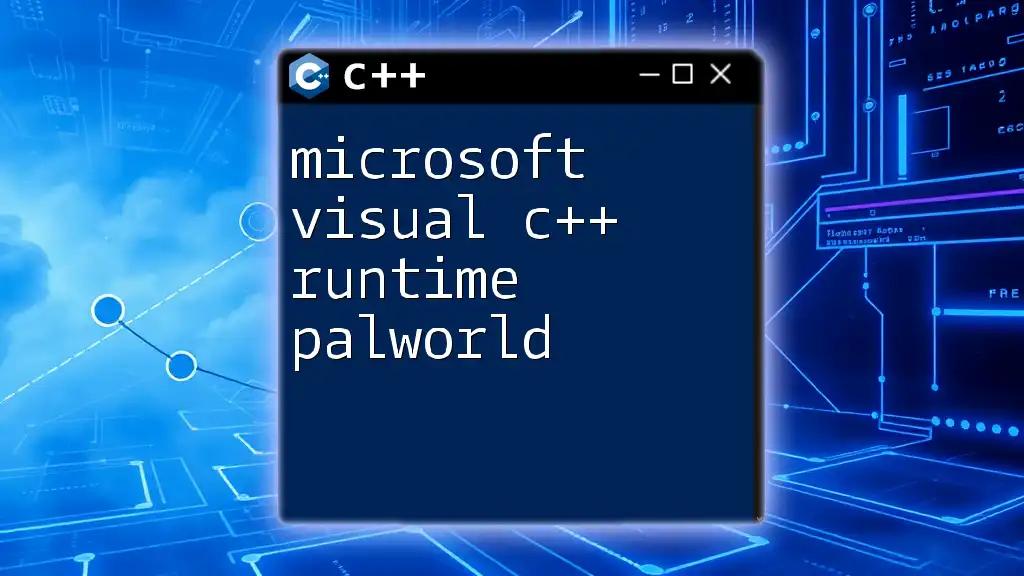
Causes of Microsoft Visual C++ Runtime Errors
Unresolved Dependencies
A prevalent cause of runtime errors is unresolved dependencies. This typically occurs when a required DLL (Dynamic Link Library) file is missing or not accessible. For instance, when deploying an application that relies on specific libraries, if any of these DLLs are not available on the target system, the application may throw runtime errors during execution.
This situation can lead to frustrating experiences for end-users, especially when they do not understand why the application fails to start.
Memory Issues
Memory-related issues significantly contribute to runtime errors. One of the most common problems is memory leaks, which occur when a program allocates memory but fails to release it back to the operating system. Over time, this can lead to insufficient memory resources for the application, ultimately resulting in a crash.
Example:
#include <iostream>
void memoryLeak() {
int* ptr = new int[10]; // Allocating memory
// Forgetting to delete the allocated memory leads to a leak.
}
int main() {
memoryLeak();
// The memory allocated above is not released.
return 0;
}
A related issue is dangling pointers, which happen when a pointer points to a memory location that has already been deallocated. Accessing such pointers can cause undefined behavior, leading to runtime errors.
Improper Pointers and References
The misuse of pointers and references is another common source of runtime errors. When developers attempt to dereference null or damaged pointers, the program can crash.
Code Example:
#include <iostream>
int main() {
int* ptr = nullptr; // A null pointer
std::cout << *ptr; // Attempting to dereference it causes a crash.
return 0;
}
In this example, attempting to dereference a null pointer clearly demonstrates a potential runtime error.
Environmental Issues
Runtime errors can also stem from environmental issues. Different versions of Visual C++ runtime libraries may clash with applications, particularly when an application is built on one version and executed on another. Additionally, outdated or incompatible systems may cause applications to behave unpredictably, leading to runtime errors.
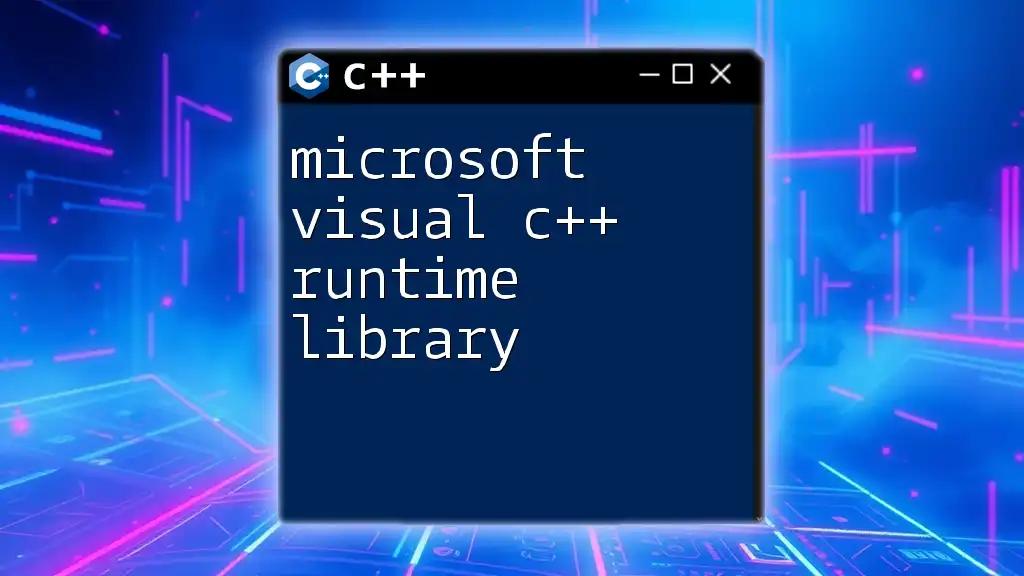
How to Fix Microsoft Visual C++ Runtime Errors
Troubleshooting Steps
When faced with runtime errors, it is crucial to follow a step-by-step troubleshooting approach. Start by examining error messages carefully to understand their origins. Employ debugging tools or IDE features to analyze the code and isolate segments likely responsible for generating the errors.
Reinstalling Visual C++ Redistributables
One effective fix is to reinstall Visual C++ Redistributables. These redistributables are essential for running applications built with Visual C++ as they provide the required libraries.
Instructions:
- Go to the official Microsoft website and locate the relevant Visual C++ Redistributable packages.
- Download the appropriate version (x86 or x64) based on your system architecture.
- Install the packages, and restart your computer to ensure everything is configured correctly.
Updating System Drivers
Outdated drivers can lead to conflicts and thus cause runtime errors in applications. Regularly updating system drivers ensures compatibility with modern applications.
How to Update:
- Use the Device Manager on Windows to check which drivers need updating.
- Alternatively, consider dedicated software tools that automate the process.
Checking for Windows Corruption
Windows systems may harbor corrupted files that could cause runtime errors. The System File Checker (SFC) is a built-in Windows tool that can help identify and repair these corrupted files.
Example Command: To run SFC, open a command prompt with administrative privileges and type:
sfc /scannow
This command scans system files and automatically repairs the corrupted ones, potentially resolving runtime errors.

Prevention of Runtime Errors
Best Coding Practices
Creating resilient applications starts with employing best coding practices. Adequate memory management is crucial; always ensure that every allocated memory is released. Implementing good error handling routines will also significantly decrease the chances of runtime errors.
Regular Maintenance
Regular maintenance of your software can go a long way in preventing runtime errors. Frequently check for available software updates, as staying current helps ensure that your applications are resilient against newly encountered issues.
Monitoring Systems: Utilize monitoring tools to track system performance and catch early symptoms of problems, allowing for preemptive actions before the issue escalates.
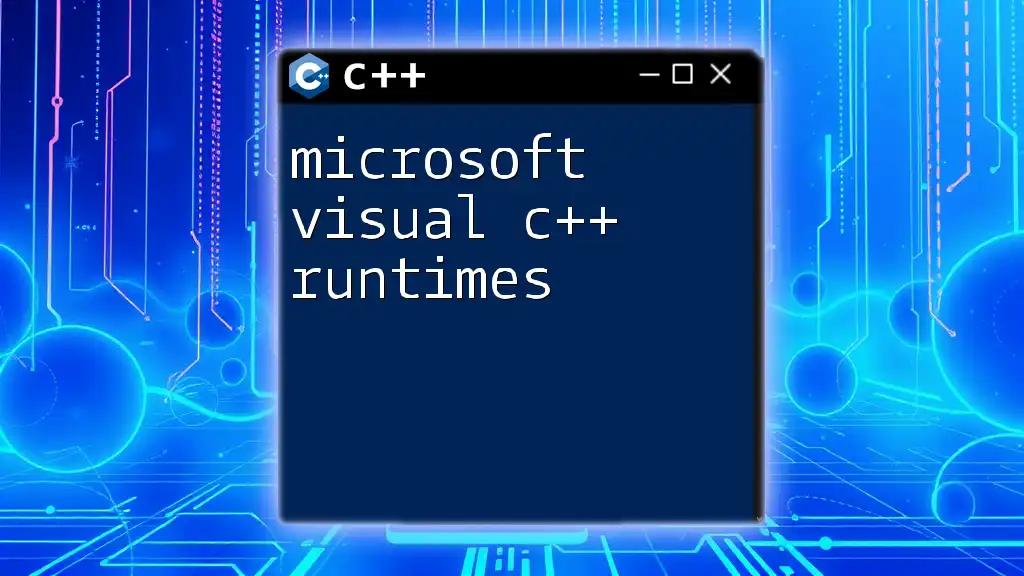
When to Seek Help
Consulting Online Communities
When unable to resolve runtime errors, consulting online communities can be invaluable. Platforms such as Stack Overflow or specialized tech forums provide a wealth of knowledge. Many users post solutions to similar problems, which can guide you toward fixing your specific issue.
Documentation and Resources
Always refer to the official Microsoft documentation for Visual C++. This will provide reliable insights into error codes and recommended practices. Additionally, consider investing time in reading specialized books or tutorials that delve deeper into C++ programming, enhancing your understanding and expertise.
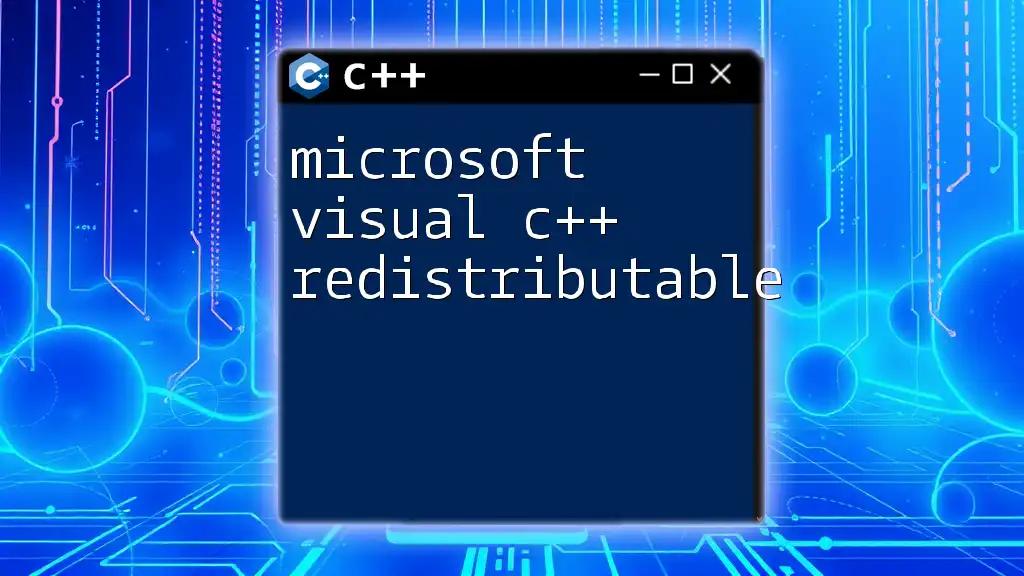
Conclusion
In summary, awareness of the Microsoft Visual C++ runtime error landscape allows developers to diagnose, address, and ultimately prevent these frustrating bugs. By understanding their causes, implementing sound coding practices, and utilizing community resources and official documentation, one can create robust and resilient applications. Remember, while runtime errors can be challenging, they are also valuable learning opportunities to improve your programming skills and application reliability.