Microsoft Visual C++ Build Tools is a set of tools designed for compiling and building C++ applications, enabling developers to create robust software without needing the complete Visual Studio IDE.
Here's a simple example of a C++ "Hello, World!" program using Microsoft Visual C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Build Tools
What are Build Tools?
In software development, build tools are essential for automating the process of converting source code files into executable programs. They encompass tools that manage the tasks of compiling code, linking it together, and packaging the output. The automation provided by build tools streamlines development workflows and minimizes the chance of human error.
Types of Build Tools
Understanding the different types of build tools can help you choose the right tool for your needs:
-
Compilers: These convert source code written in high-level programming languages into machine code. Microsoft Visual C++ Build Tools includes the `cl` compiler specifically designed for C++.
-
Linkers: After compilation, linkers combine object files and resolve dependencies, resulting in a final executable. This step is crucial when working with multiple source files or external libraries.
-
Dependency Managers: These tools manage external libraries that your code relies on, ensuring easy access and version control.
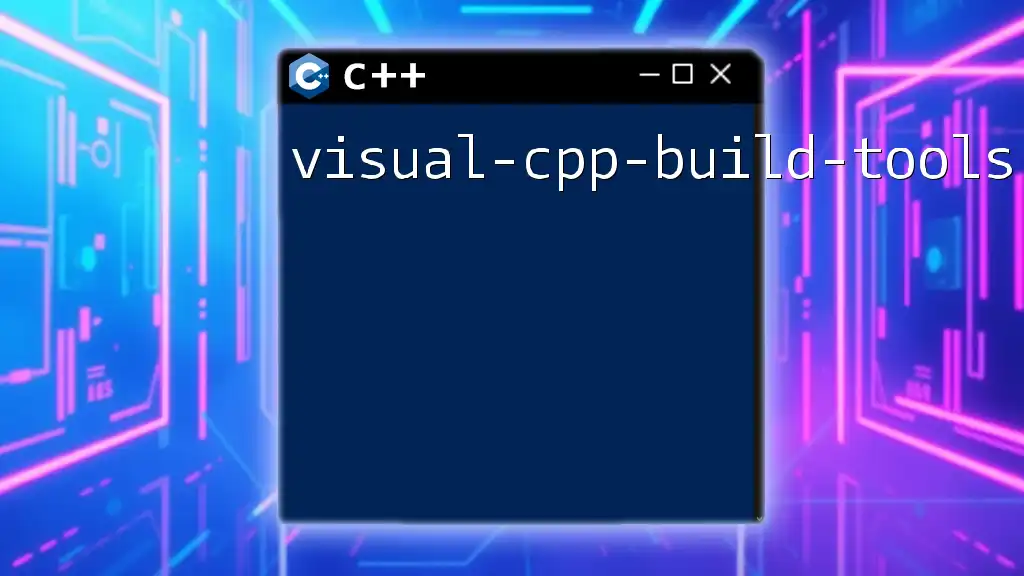
Microsoft Visual C++ Build Tools
Features of Visual C++ Build Tools
Integrated Development Environment (IDE) Support
One of the standout features of Microsoft Visual C++ Build Tools is its seamless integration with the Visual Studio IDE. This integration provides a user-friendly interface, offering developers a robust environment to write, compile, and debug code efficiently.
Compiler and Linker
The Microsoft C++ compiler offers robust functionality and supports various language features, allowing developers to leverage modern C++ capabilities. Here's a simple example demonstrating how to compile a basic C++ program using the Visual C++ compiler:
// Example: HelloWorld.cpp
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this file using Microsoft Visual C++ Build Tools, you would run the following command in the command prompt:
cl HelloWorld.cpp
This command invokes the compiler, compiles the `HelloWorld.cpp` file, and if no errors are present, generates an output executable named `HelloWorld.exe`.
Support for C++ Standards
Microsoft Visual C++ Build Tools supports multiple C++ standards, including C++11, C++14, C++17, and C++20. This comprehensive support allows developers to utilize the latest features and improvements in C++ directly in their projects, enhancing code quality and performance.
Setting Up Microsoft Visual C++ Build Tools
Installation of Visual C++ Build Tools
Installing the Microsoft Visual C++ Build Tools is a straightforward process. Here’s how you can do it:
-
Download the Installer: Head over to the official Microsoft website to download the Visual Studio installer.
-
Choose the C++ Development Workload: During installation, select the ‘Desktop development with C++’ workload, which includes all necessary components for C++ development.
Configuration
After installation, it’s important to configure the tools for your specific project needs. One essential step includes setting environment variables to allow command-line access to the tools. You can add the installation path of Visual Studio to your system’s PATH variable, making it easier to open the command prompt and use the `cl` command without navigating to the installation directory.
Usage of Visual C++ Build Tools
Compiling C++ Programs
Once set up, you can efficiently compile your C++ projects. The compilation process can include various flags to customize your build. Below are examples:
-
Optimization Flags: Using optimization flags can enhance the performance of your code:
cl /O2 HelloWorld.cpp
-
Debugging Flags: For debugging purposes, the `/Zi` flag generates debugging information:
cl /Zi HelloWorld.cpp
By compiling with the appropriate flags, you can tailor the output based on your specific performance or debugging needs.
Linking with Libraries
When your project depends on external libraries, understanding both static and dynamic linking is crucial. Static linking incorporates library code into the executable, whereas dynamic linking retains the library as a separate file. Here’s how you can include a library in your project.
Assuming you have a library named `MyLibrary.lib`, you can link it during compilation like this:
cl HelloWorld.cpp MyLibrary.lib
This command links `MyLibrary.lib` with your program, allowing you to utilize the functions defined within the library.
Common Issues and Troubleshooting
Compilation Errors
Despite the powerful features of Visual C++ Build Tools, you might encounter compilation errors. Common issues include missing semicolons, undeclared variables, and type mismatches. Reviewing the error messages provided in the command line can often help pinpoint the exact issue.
Runtime Errors
Runtime errors can pose a challenge and may include segmentation faults or incorrect output. To troubleshoot these, take advantage of the integrated debugging tools within Visual Studio. You can set breakpoints, step through code, and inspect variables, which assists in identifying where things go wrong during execution.
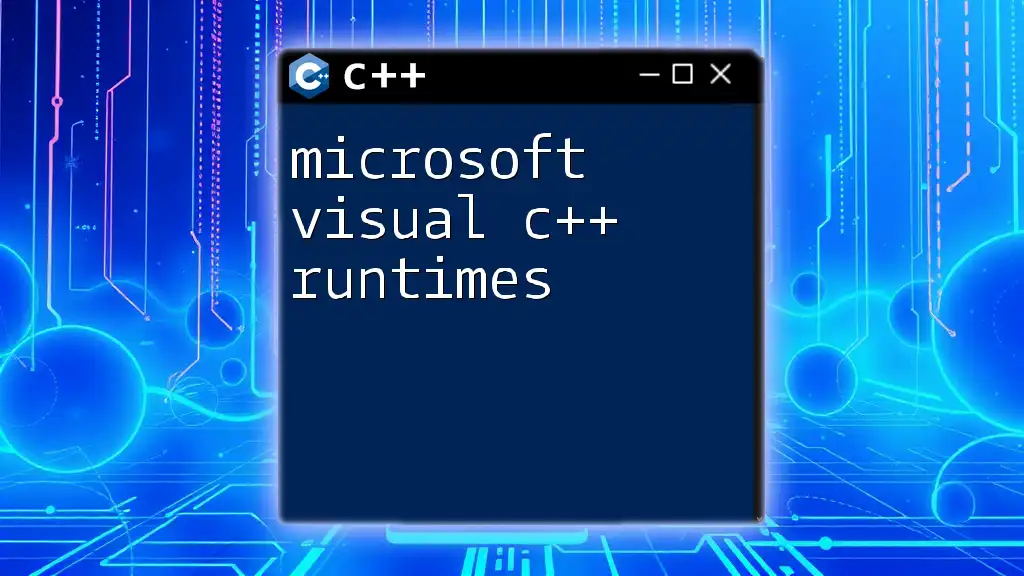
Alternatives to Visual C++ Build Tools
Other Build Tools for C++
Although Microsoft Visual C++ Build Tools are highly effective, other options may suit specific needs, such as:
- GCC (GNU Compiler Collection): A widely-used open-source compiler offering robust support for C++.
- Clang: Known for its performance and user-friendly diagnostics, Clang is another compelling alternative.
- CMake: A cross-platform build system generator, ideal for handling complex build processes.
Choosing the Right Build Tool
When choosing a build tool, consider factors like your project's scope, team preferences, and required features. For example, Microsoft Visual C++ Build Tools may be ideal for Windows-centric projects, while GCC or Clang could be more appropriate for cross-platform development.
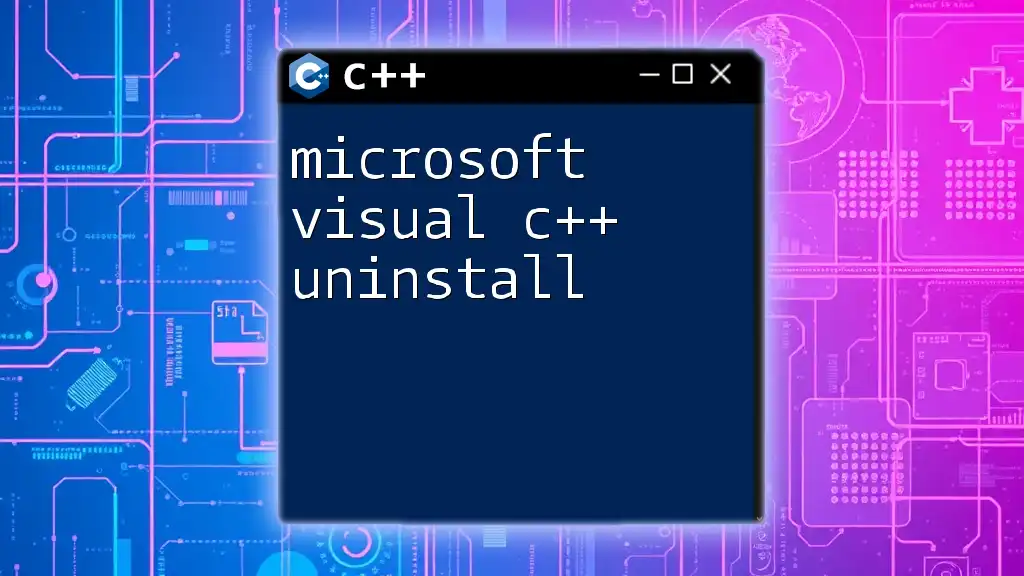
Conclusion
In summary, Microsoft Visual C++ Build Tools serve as a powerful asset for C++ development, providing essential features that streamline the build process while enabling efficient programming. Understanding how to leverage these tools will significantly enhance your experience and productivity as a C++ developer.
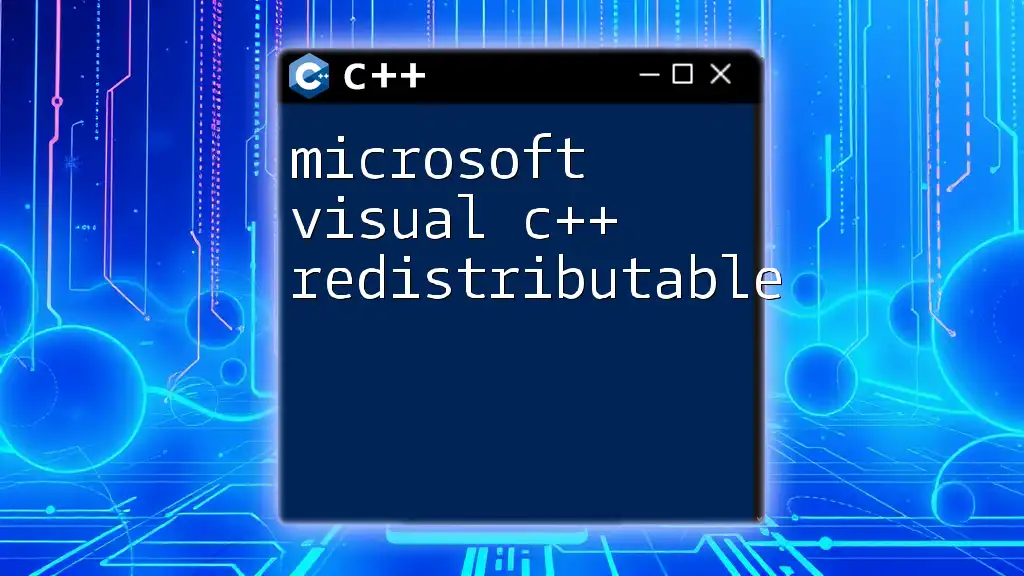
Call to Action
To dive deeper into the world of Microsoft Visual C++ Build Tools, explore our upcoming tutorials and workshops tailored to help you master C++ commands and build processes effectively. Stay ahead in your programming journey!
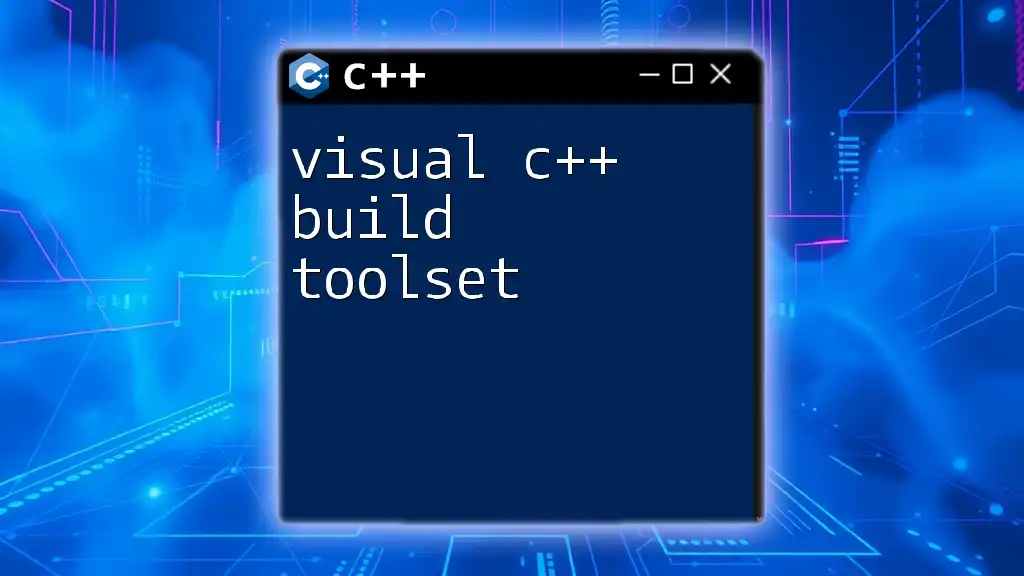
Additional Resources
For further learning, check out reputable books, online courses, and forums focused on C++ development. Additionally, familiarize yourself with the official Microsoft documentation for Visual C++ Build Tools to unlock the full potential of these tools in your programming endeavors.