The Microsoft Visual C++ Runtime Library provides essential functions and routines that are needed to run applications developed with Microsoft Visual C++, ensuring that software can execute properly on Windows systems.
Here's a simple code snippet demonstrating how to include a standard library and create a basic console application in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is the Microsoft Visual C++ Runtime Library?
Definition and Purpose
The Microsoft Visual C++ Runtime Library is a collection of pre-written code that helps C++ applications run efficiently on Windows environments. This library provides essential routines for memory management, file handling, and string manipulation, among other functionalities. Essentially, it allows developers to leverage existing code, reducing the time and effort needed to implement common features in their applications.
Components of the Microsoft C++ Runtime Library
The runtime library consists of multiple components, primarily encapsulated in Dynamic-Link Libraries (DLLs). These libraries are crucial for executing applications developed with Microsoft Visual C++. Key libraries include:
- `MSVCRT.dll`: The Microsoft C Runtime Library.
- `MSVCP140.dll`: The Microsoft C++ Standard Library (for Visual Studio 2015 and later).
Each of these DLLs plays a role in providing specific functionalities required by C++ applications, ensuring they can execute various tasks seamlessly. Understanding the function of each DLL is important for troubleshooting and optimizing applications.

Why is the Microsoft Visual C++ Runtime Library Important?
Runtime Errors and Their Handling
One of the critical reasons for understanding the Microsoft Visual C++ Runtime Library is its role in error handling. Missing the necessary DLL files can lead to runtime errors, causing applications to crash or behave unpredictably. For example, attempting to run a C++ application compiled with Visual Studio can result in errors like:
- "The program can't start because MSVCP140.dll is missing from your computer."
This library acts as a fallback mechanism to manage these runtime errors, allowing developers to focus on core application logic rather than low-level error handling.
Performance Optimization
The performance of C++ applications greatly benefits from the runtime library. It provides intelligent memory management and resource allocation functions that allow applications to run efficiently. By utilizing optimized routines provided by the Microsoft Visual C++ Runtime Library, applications can perform complex tasks quickly and reduce the likelihood of memory leaks.
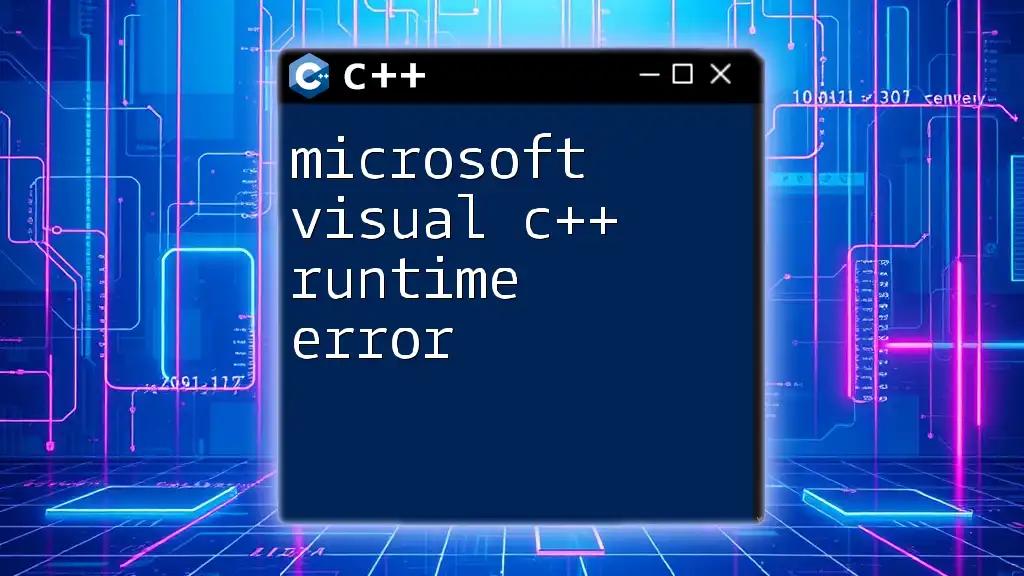
How to Install the Microsoft Visual C++ Runtime Library
Methods of Installation
To use the Microsoft Visual C++ Runtime Library, you must ensure it's installed correctly on your machine, which can be done through multiple ways:
Installing via Visual Studio
When installing Visual Studio, you can choose to include the runtime library. Simply:
- Launch the Visual Studio installation program.
- On the installation options screen, check the box for "Desktop development with C++".
- The Microsoft Visual C++ Runtime Library will be installed along with the development environment.
Standalone installer
If you prefer to install the library independently, download the appropriate version from the Microsoft website. Once downloaded:
- Run the installer.
- Follow the on-screen instructions to complete the installation.
Verifying the Installation
After installation, it's essential to confirm that the Microsoft C++ Runtime Library is correctly installed. You can do this by:
- Opening the command prompt.
- Running the command to check for the presence of specific DLLs. This can also help you determine the version installed:
dir C:\Windows\System32\MSVCP*.dll
You should see the installed versions listed. If any required DLL is missing, you may need to reinstall the library.
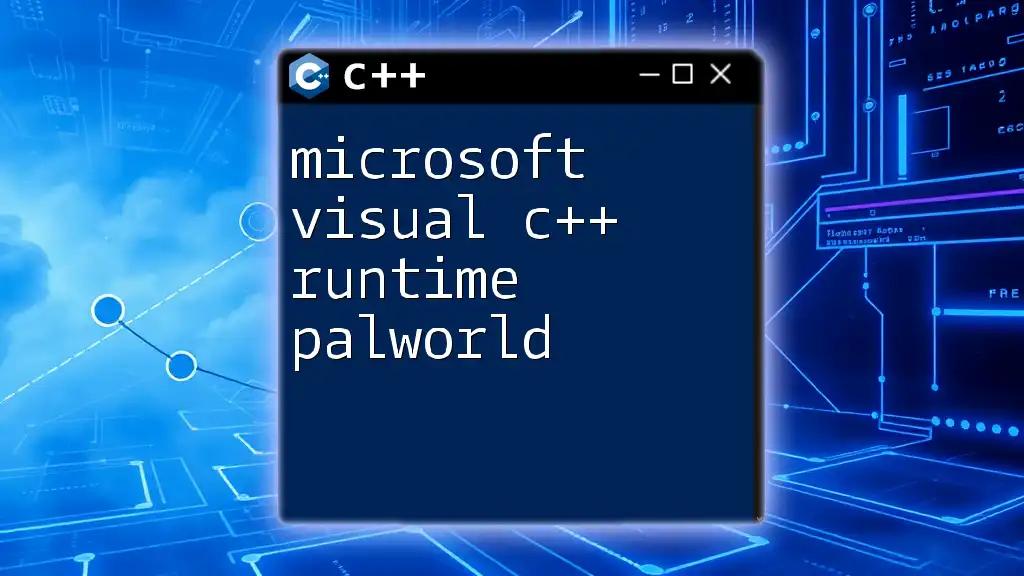
Common Issues with the Microsoft Visual C++ Runtime Library
Missing or Corrupted DLL Files
One of the most prevalent issues developers may face is the missing or corrupted DLL files associated with the Microsoft Visual C++ Runtime Library. Such problems can stem from unintentional file deletion, software updates, or system corruption.
Solutions include:
- Repairing: Use the installation program to repair the Visual C++ Redistributable.
- Reinstalling: Completely uninstall and reinstall the library.
Compatibility Issues
Compatibility is another challenge developers may encounter. Applications built on different versions of the runtime library might not work seamlessly together, especially if they rely on specific versions of the DLLs. Mixing static and dynamic linking can exacerbate compatibility issues, leading to unexpected behavior.
Understanding the specific version your application requires is crucial to maintaining compatibility across different environments.
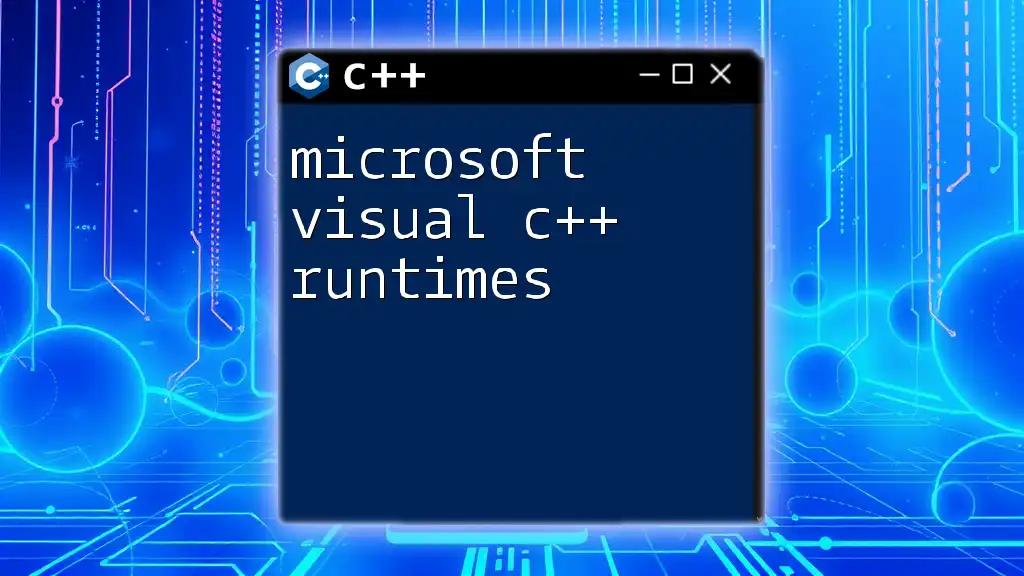
Best Practices for Using the Microsoft Visual C++ Runtime Library
Linking Strategies
When linking libraries, developers should consciously choose between static and dynamic linking.
-
Static Linking: The code in the library becomes part of the executable, reducing dependency on external DLLs. However, this may increase the executable file size.
-
Dynamic Linking: The executable relies on external DLLs. While this saves space, any missing or mismatched DLL on the target machine may lead to runtime errors.
Consider the needs of your application before deciding on a linking strategy.
Handling Dependencies
Incorporating third-party libraries may lead to complications if they depend on specific versions of the Microsoft C++ Runtime Library. Understanding and managing these dependencies is critical to ensuring a smooth deployment.
A useful tool here is the Dependency Walker, which identifies all required DLLs and their dependencies. This tool aids in troubleshooting missing library issues before deploying the application.
Code Examples
When utilizing the Microsoft Visual C++ Runtime Library, you can write simple C++ code to engage its functionality. Here’s a basic example demonstrating how the library simplifies string handling and output to the console:
#include <iostream>
#include <string>
using namespace std;
int main() {
string greeting = "Hello, Microsoft C++ Runtime!";
cout << greeting << endl;
return 0;
}
This simple program leverages the runtime library for standard I/O operations and string manipulation, showcasing the ease of use it provides to developers.

Conclusion
The Microsoft Visual C++ Runtime Library is a vital component for C++ developers, offering necessary functionalities that ensure applications run smoothly and efficiently. Understanding its role, installation procedures, common issues, and best practices is essential for developers who wish to optimize their applications. By utilizing resources like this, developers can enhance their skills in C++ programming and application development.