The Visual C++ runtime provides a set of libraries and services that allow C++ applications to execute and utilize system resources effectively, ensuring proper memory management and functionality across different Windows platforms.
#include <iostream>
int main() {
std::cout << "Hello, Visual C++ Runtime!" << std::endl;
return 0;
}
Understanding the Visual C++ Runtime
What are Runtime Libraries?
Runtime libraries are essential collections of code that a program uses while it executes. They provide the functions and processes necessary to support various programming tasks and operations. These libraries enable developers to write programs without needing to reinvent the wheel for common functionalities, allowing them to focus on the unique aspects of their applications.
Components of Visual C++ Runtime
The Visual C++ Runtime consists of several key components:
- C Runtime Library (CRT): This library handles the C standard library functions, crucial for operations like memory management, string manipulation, and mathematical computations.
- Standard C++ Library (STL): The STL provides a set of common classes and functions, such as containers, algorithms, and iterators, that enable developers to write efficient and robust C++ code.
- Microsoft C++ Standard Libraries: These libraries extend functionality beyond the STL, providing additional tools and classes tailored for Visual C++ development.
Each of these components is vital for creating applications that run effectively and efficiently on Windows systems.
Description of Each Component with Code Snippets
C Runtime Library
The CRT is indispensable for managing low-level tasks in C and C++. Here is a simple example of using the CRT to perform basic input/output operations:
#include <stdio.h>
int main() {
printf("Hello, Visual C++ Runtime!\n");
return 0;
}
Standard C++ Library
The STL allows for advanced operations, such as leveraging data structures and algorithms. For instance, here’s how you can use a `vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
The above example shows how easy it is to store and iterate through a dynamic array of integers using the STL.
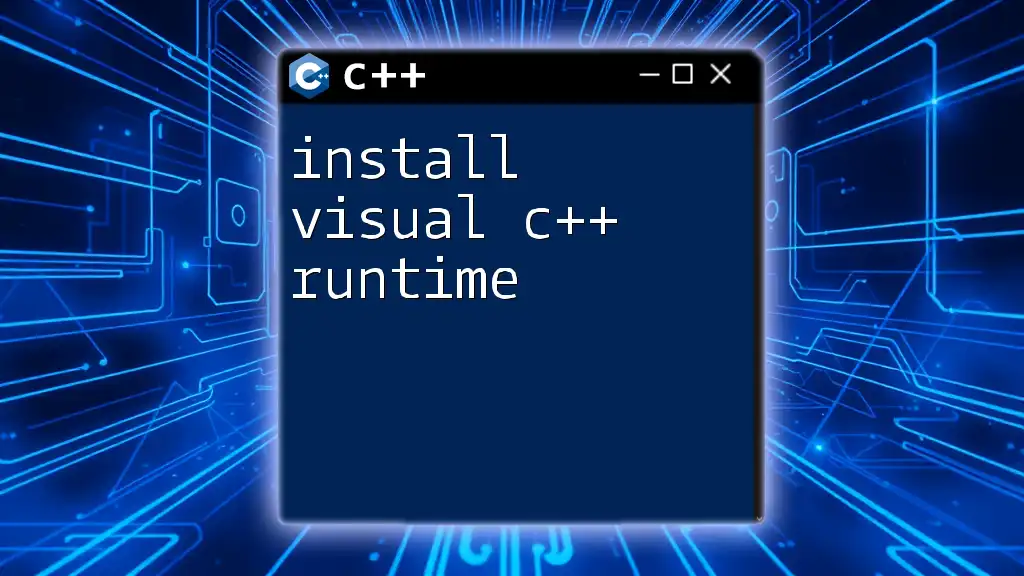
Installation and Setup
Pre-requisites
Before working with the Visual C++ Runtime, it’s important to ensure that your development environment is correctly set up. Ensure you have:
- A compatible version of Windows (Windows 7 or later).
- Visual Studio installed, preferably the latest version to ensure compatibility with the recent updates.
Downloading Visual C++ Redistributable
Visual C++ Runtime libraries are typically part of the Visual C++ Redistributable package. You can find these packages on the official Microsoft website. Look for the version that matches your development environment, whether it's 32-bit or 64-bit.
Installing Visual C++ Runtime
Once you've downloaded the Redistributable package, follow these steps to install:
- Run the installer.
- Follow the on-screen prompts to complete the installation.
Common errors may arise due to incompatible versions or missing prerequisites, so ensure to resolve any alerts provided during installation.
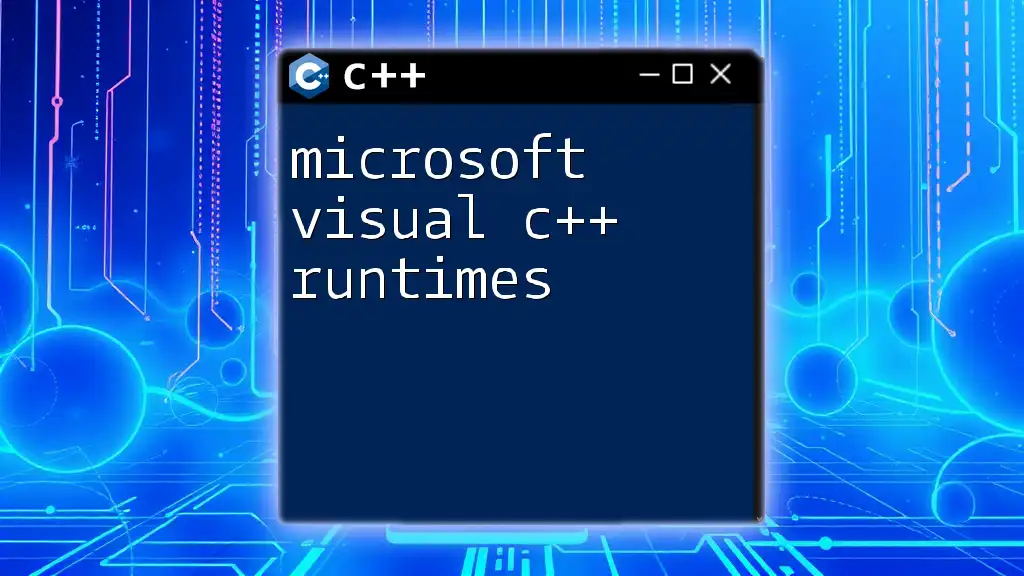
Using Visual C++ Runtime in Your Application
Linking with Visual C++ Runtime
Linking your application with the Visual C++ Runtime is straightforward when using Visual Studio. During project setup:
- Right-click on your project in the Solution Explorer.
- Choose ‘Properties’ and navigate to Configuration Properties > C/C++ > Code Generation.
- Select the appropriate Runtime Library option.
This ensures that your application has access to the required libraries when executed.
Code Snippets for Common Tasks
Basic Input/Output
Creating a simple console application using the Visual C++ Runtime requires knowledge of standard I/O functions:
#include <iostream>
int main() {
std::cout << "Hello, Visual C++ Runtime!" << std::endl;
return 0;
}
This code snippet illustrates the basic usage of the iostream library to print a message to the console.
File Handling
File operations are an essential part of many applications. Below is an example of writing to and reading from a file:
#include <fstream>
#include <iostream>
int main() {
// Writing to a file
std::ofstream outFile("example.txt");
outFile << "Sample text for Visual C++ Runtime!" << std::endl;
outFile.close();
// Reading from a file
std::ifstream inFile("example.txt");
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
This code demonstrates both writing to a file and reading from it, showcasing the versatility of file handling in Visual C++.
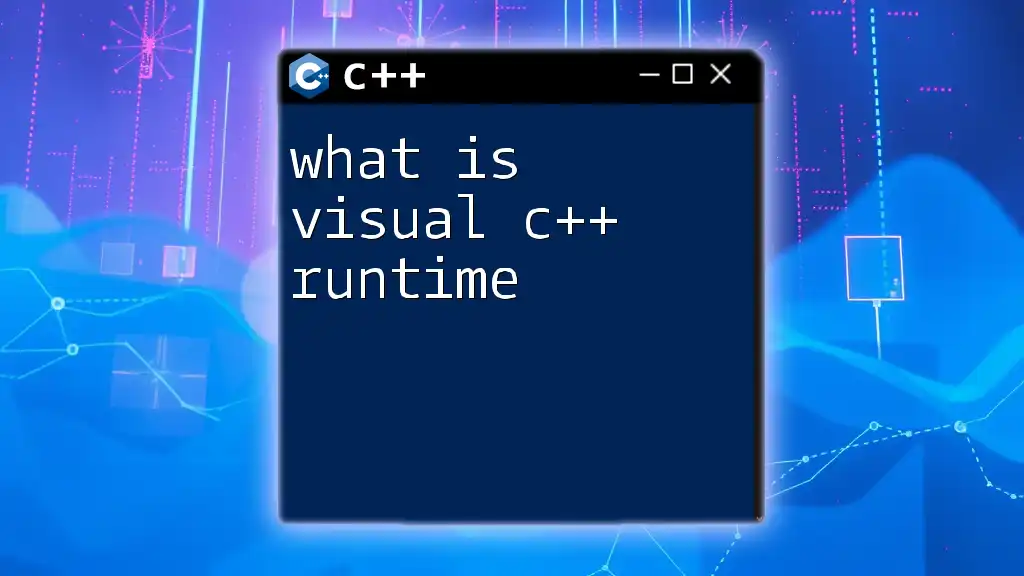
Debugging and Troubleshooting
Common Runtime Errors
When working with Visual C++ Runtime, you might encounter errors such as:
- 0xc000007b: This error often indicates a mismatch between 32-bit and 64-bit applications. Ensure that you are operating with the correct architecture throughout your development.
- 0xc0000005: This is an access violation error, often due to improper memory allocation or invalid pointers. Use debugging tools to trace the source.
Tools for Debugging
Visual Studio is equipped with powerful debugging tools, allowing you to step through your code, inspect variables, and monitor application flow. Familiarize yourself with features like breakpoints, watch windows, and call stacks to diagnose issues efficiently.
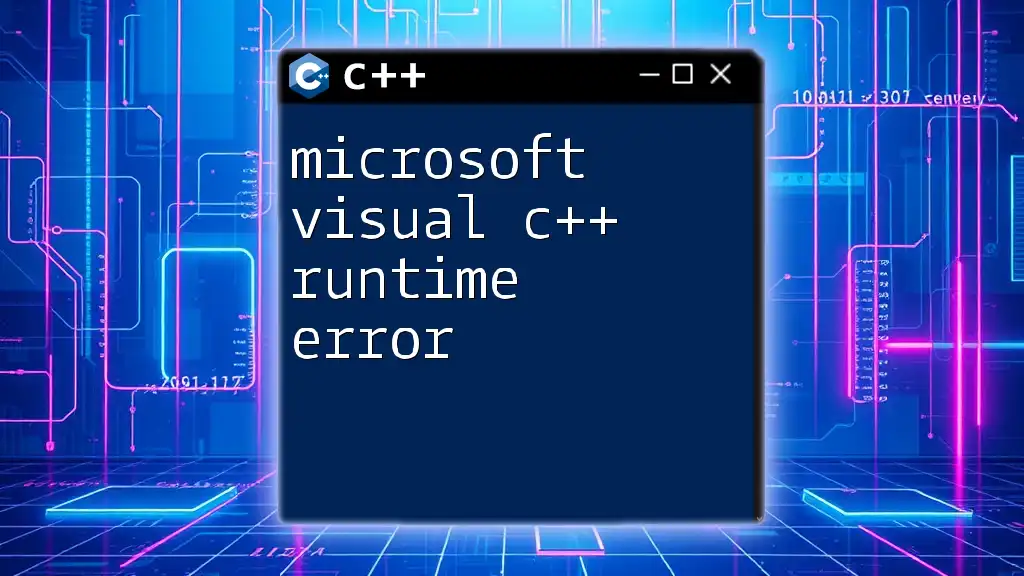
Best Practices for Working with Visual C++ Runtime
Optimizing Performance
To maximize your application's performance, consider these tips:
- Use efficient data structures: Depending on your use case, select the appropriate containers from the STL.
- Manage memory carefully: Avoid memory leaks by ensuring that every `new` has a corresponding `delete`.
Ensuring Compatibility
Writing portable code is crucial for broader application compatibility. Stick to standardized functions and avoid platform-specific features when possible. Regular testing on multiple environments can help catch inconsistencies early on.
Keeping the Runtime Updated
Regular updates to the Visual C++ Runtime ensure that you benefit from security patches, performance improvements, and new functionalities. Periodically check the Microsoft website for updates and apply them as necessary.
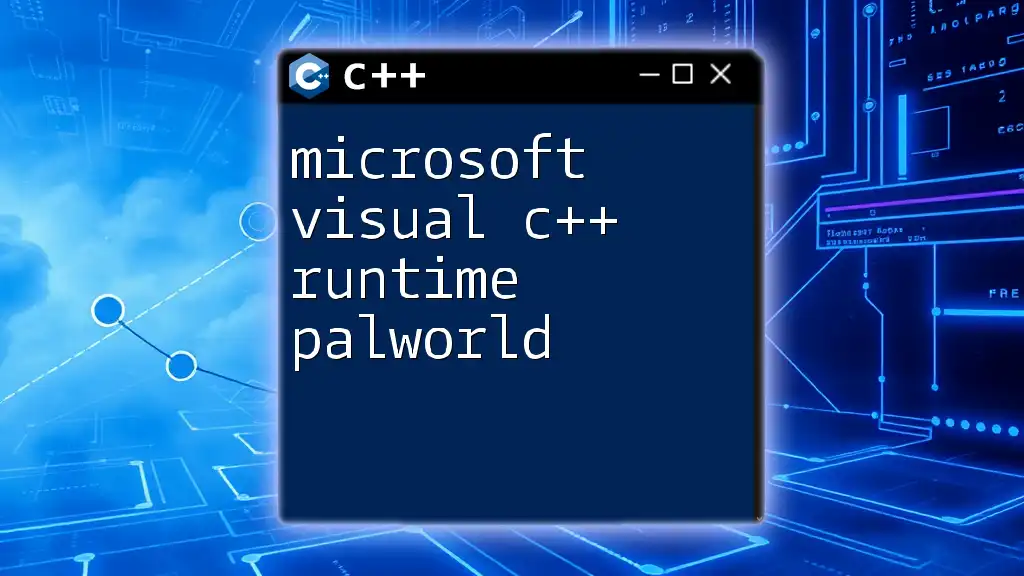
Conclusion
Visual C++ Runtime is a fundamental aspect of C++ application development, streamlining various coding processes and enhancing application performance. By understanding its components, setup procedures, and best practices, developers can leverage its capabilities to create robust and efficient applications.
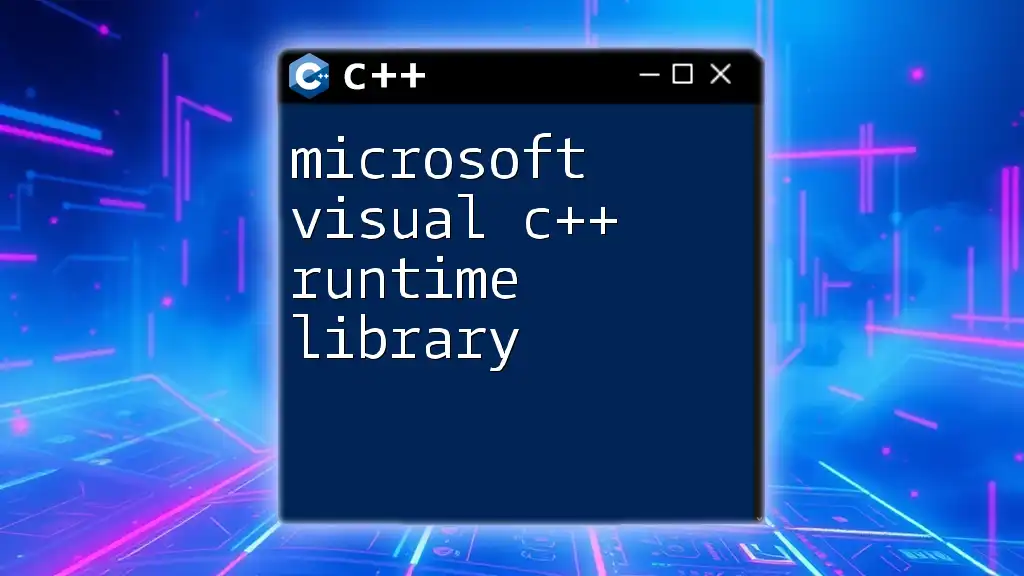
Additional Resources
For further exploration, consider these resources:
- Recommended books on C++ programming and Visual Studio.
- Online tutorials that cover advanced features and common pitfalls.
- Official Microsoft documentation and community forums for troubleshooting and support.
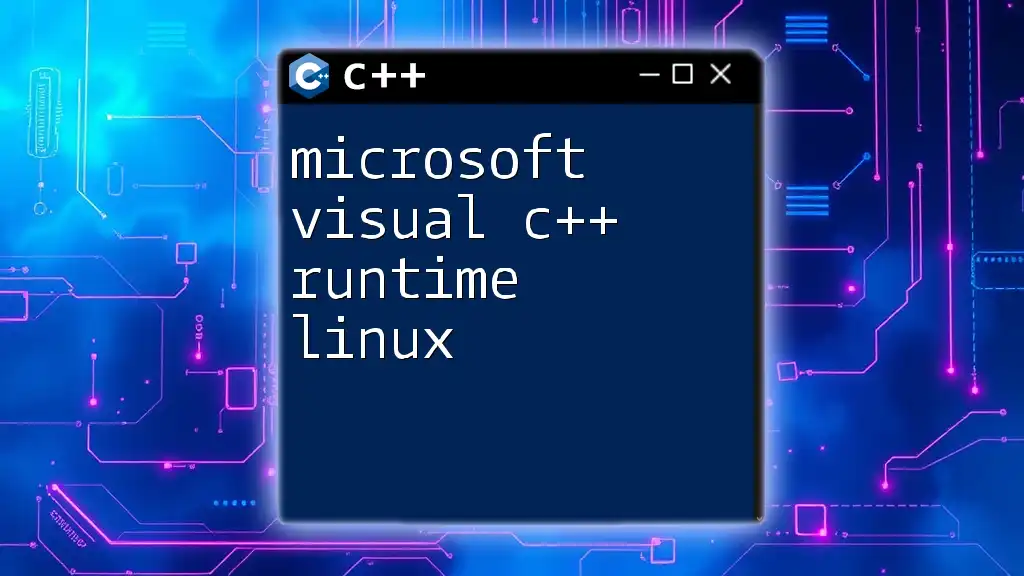
FAQs
-
What if my application doesn’t run on a system with Visual C++ Runtime?
- Ensure the correct version of the Visual C++ Redistributable is installed on the target machine.
-
How can I check which version of Visual C++ Redistributable is installed?
- Go to the Control Panel, select Programs and Features, and look for the installed versions of Microsoft Visual C++ Redistributable.
-
Can I install multiple versions of Visual C++ Runtime simultaneously?
- Yes, you can install different versions side by side. This is useful for applications targeting various versions of the runtime.
By following the suggestions outlined in this guide, you can effectively navigate the world of Visual C++ Runtime and enhance your proficiency in developing C++ applications.