The C++ runtime library provides essential functions and support for executing C++ programs, including memory management, input-output handling, and standard library functionalities.
Here's a simple example demonstrating the use of the C++ runtime library to output text to the console:
#include <iostream>
int main() {
std::cout << "Hello, C++ Runtime Library!" << std::endl;
return 0;
}
What is the C++ Runtime Library?
The C++ runtime library is a crucial part of the C++ programming environment. It provides the essential facilities required for running C++ programs, offering a collection of functions and resources that support program execution. The runtime library handles various tasks such as memory allocation, input/output operations, string handling, and exception management, ensuring that a C++ application runs smoothly and efficiently.
One might confuse the runtime library with the standard library, but it's essential to distinguish between the two. The standard library includes all the basic building blocks and utilities of the C++ language, while the runtime library specifically addresses features that require operational support during execution.
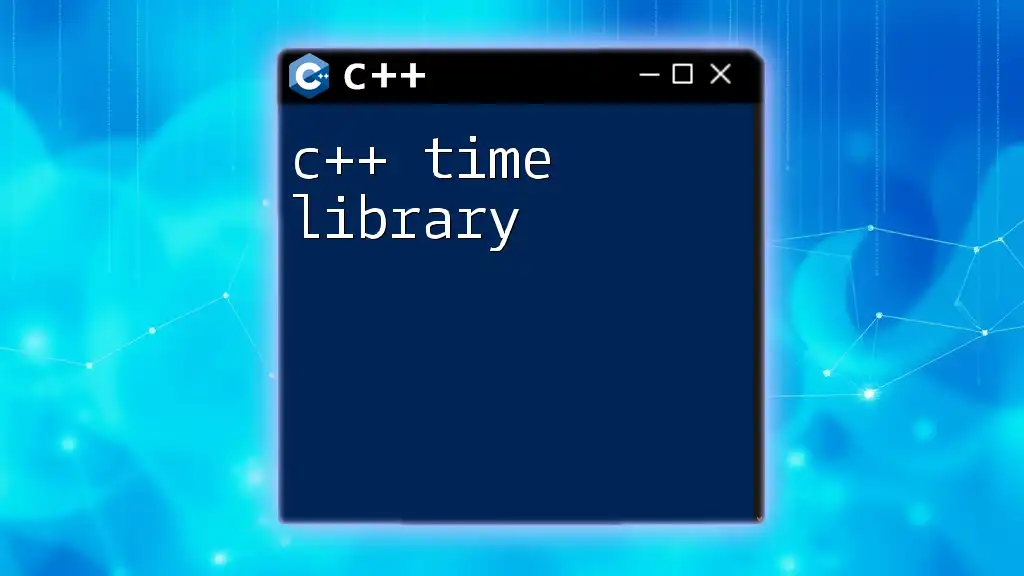
Key Components of the C++ Runtime Library
Standard Input/Output Functions
At the core of any application lies the need for interaction with users, primarily through input and output. The `iostream` library in C++ facilitates this with its powerful stream handling capabilities.
For example, using `cin` and `cout` allows versatile input and output operations:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
cout << "You are " << age << " years old." << endl;
return 0;
}
In this snippet, `cout` outputs text to the console, while `cin` receives input from the user. This basic functionality exemplifies how the C++ runtime library simplifies user interaction within applications.
Memory Management
Memory management is a fundamental aspect of programming, and C++ provides several tools for handling memory dynamically. The `new` and `delete` keywords allow developers to allocate and deallocate memory at runtime:
int* ptr = new int; // Dynamic memory allocation
*ptr = 42;
delete ptr; // Freeing the allocated memory
In this code, `new` allocates an integer on the heap, while `delete` ensures that the allocated memory is freed when no longer needed. This manual control is powerful but also demands responsibility from programmers to avoid memory leaks.
To enhance memory safety and automatically manage resources, C++11 introduced smart pointers, such as `std::unique_ptr` and `std::shared_ptr`. These smart pointers not only simplify memory management but also help prevent common pitfalls, like double deletes or dangling pointers.
Exception Handling
Robust applications anticipate and gracefully handle errors through exception handling. The C++ runtime library provides a standardized way to manage exceptions using the `try`, `catch`, and `throw` keywords.
For instance, consider a simple example of exception handling:
try {
throw std::runtime_error("An error occurred");
} catch (std::runtime_error &e) {
cout << e.what() << endl; // Output the error message
}
Here, a runtime error is simulated with the `throw` statement. The program captures it using a `catch` block, effectively isolating error handling from normal execution flow. This approach enhances code readability and maintainability by separating error logic from the main program logic.
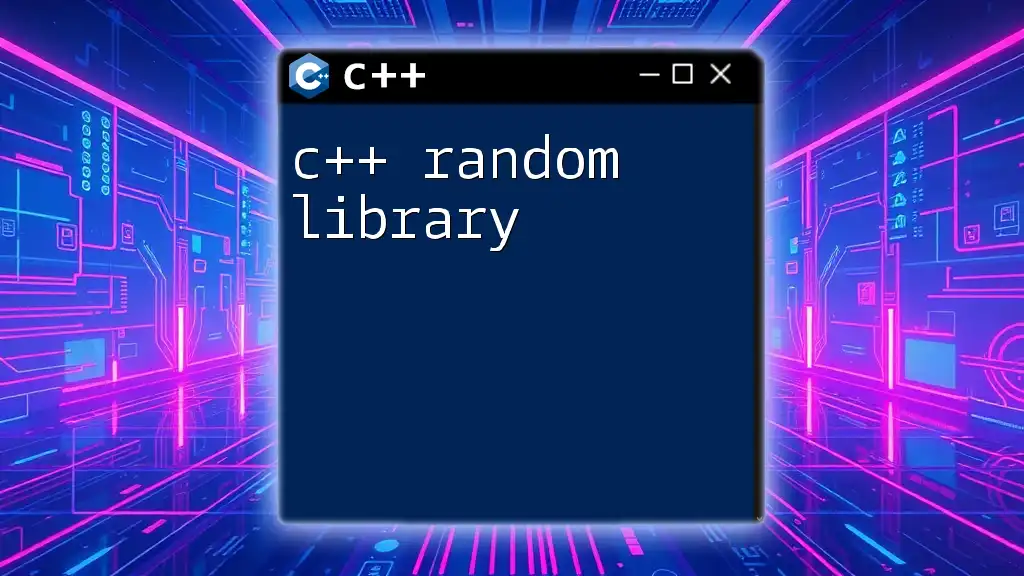
C++ Runtime Library Functions
Mathematical Functions
Mathematical operations play a crucial role in many applications. The `<cmath>` library in C++ provides a suite of functions for advanced mathematical calculations.
Here’s an example using the `sqrt` function to compute the square root:
#include <cmath>
#include <iostream>
using namespace std;
int main() {
double root = sqrt(16.0); // Outputs 4
cout << "The square root of 16 is: " << root << endl;
return 0;
}
Using `<cmath>`, developers can leverage various mathematical functions like `pow()`, `sin()`, and `cos()` to perform complex calculations robustly and accurately.
String Manipulation
Efficient string management is vital for any application, and the C++ runtime library excels in this area with the `<string>` class. C++ provides several built-in operations for string manipulation, including concatenation, searching, and substring extraction.
For example, consider the following code that demonstrates string concatenation:
#include <string>
#include <iostream>
using namespace std;
int main() {
string str1 = "Hello, ";
string str2 = "World!";
string result = str1 + str2; // Concatenation
cout << result << endl; // Outputs "Hello, World!"
return 0;
}
In this snippet, the `+` operator allows seamless concatenation of two strings. With additional functionality, the `std::string` class simplifies many common string operations, enhancing programming productivity.
Containers and Algorithms
The STL (Standard Template Library) is a powerful aspect of the C++ runtime library and provides a variety of containers, such as vectors, lists, and maps, as well as algorithms for manipulating these containers.
For instance, consider using a vector for storing integers and sorting them:
#include <vector>
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
vector<int> nums = {4, 2, 3, 1};
sort(nums.begin(), nums.end()); // Sorting the vector
for(int num : nums) {
cout << num << " "; // Outputs: 1 2 3 4
}
return 0;
}
In this example, the `sort` algorithm is utilized to arrange elements in ascending order. C++'s container and algorithm combination significantly simplifies data management and manipulation, making coding more efficient.
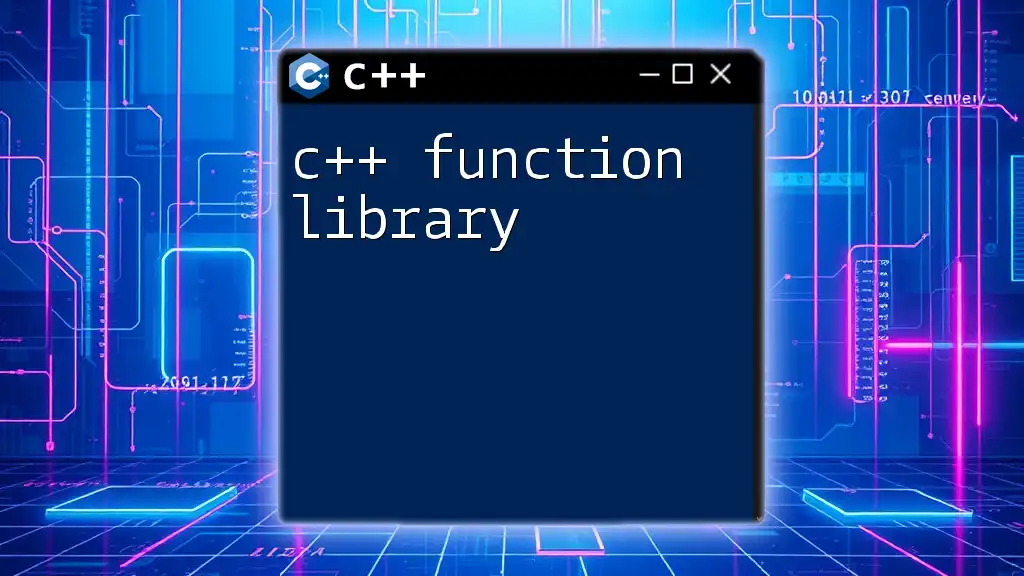
Building Applications with the C++ Runtime Library
Integrating the Standard Library in Projects
When building applications, including the appropriate parts of the C++ runtime library is essential. Utilize `#include` directives to incorporate needed functionalities without inflating your program unnecessarily. It's good practice to organize your code effectively, ensuring modular and maintainable designs.
Performance Considerations
Performance is a pivotal aspect of software development. The C++ runtime library, while providing useful features, can also impact the efficiency of applications. Understanding how to leverage the library without incurring overhead is crucial. This might include preallocating memory, using efficient containers, or opting for in-place algorithms whenever possible.
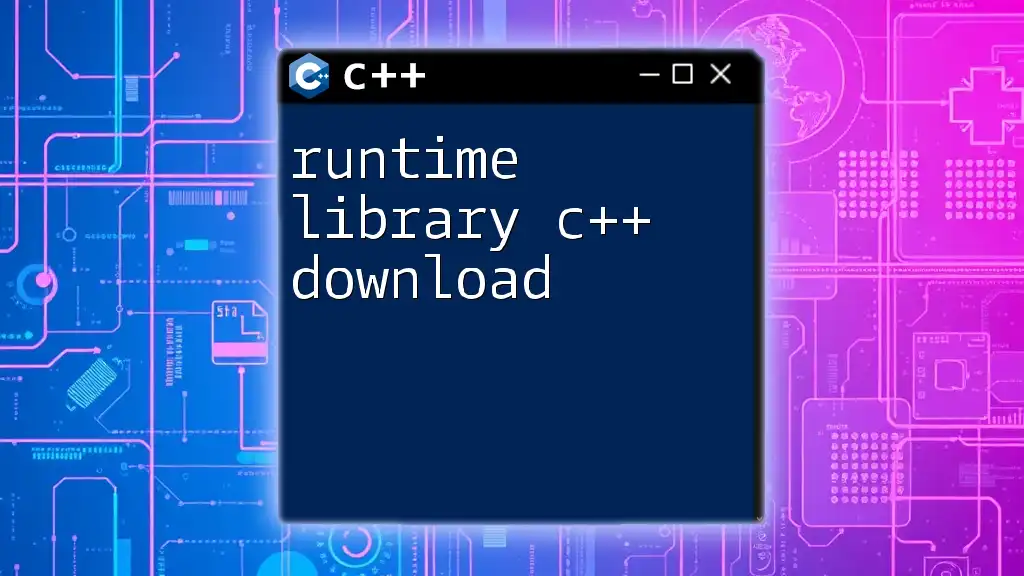
Common Issues and Troubleshooting
Runtime Errors
Working with the C++ runtime library, developers may encounter various runtime errors, including segmentation faults and memory leaks. Identifying and rectifying these errors can significantly improve your application's reliability. Employ tools like valgrind to detect memory issues and familiarize yourself with common error messages provided by the C++ compiler to facilitate debugging.
Compiler vs Runtime Errors
It's critical to differentiate between compile-time and runtime errors. Compile-time errors are detected when the code is compiled, while runtime errors occur during program execution. Here’s a simple distinction using code examples:
-
Compile-time Error:
int main() { int x; x = 5; // if 'x' has not been declared, it will throw a compile-time error return 0; }
-
Runtime Error:
#include <iostream> using namespace std; int main() { int* ptr = nullptr; cout << *ptr; // This dereference causes a runtime error return 0; }
Understanding these differences will aid developers in debugging their applications more effectively.
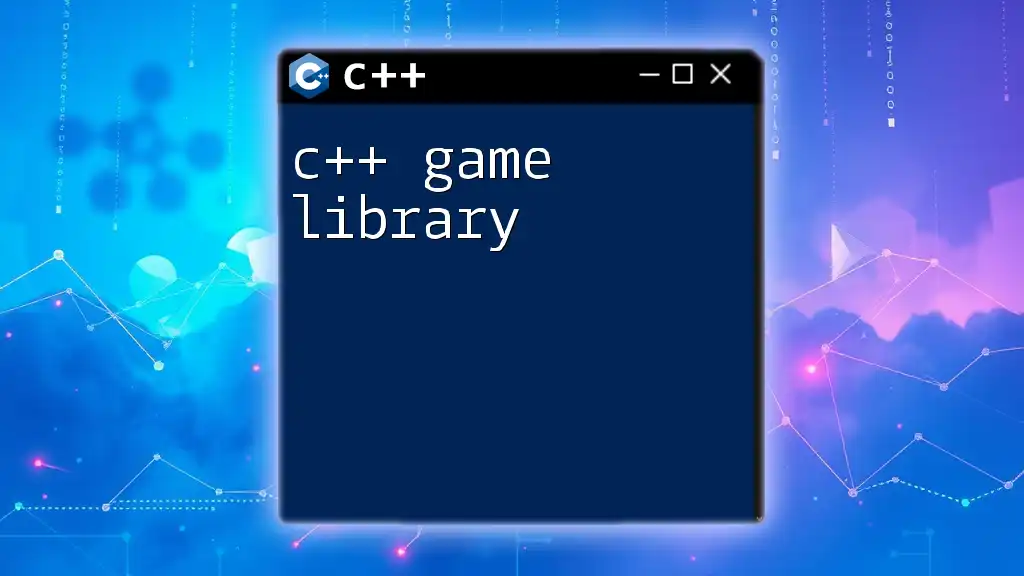
Conclusion
In summary, the C++ runtime library is an integral part of the C++ programming infrastructure. From handling input and output to managing memory and exceptions, its robust features enable developers to create efficient and reliable applications. By mastering the components discussed, programmers can leverage the power of C++ and elevate their software development skills to new heights.
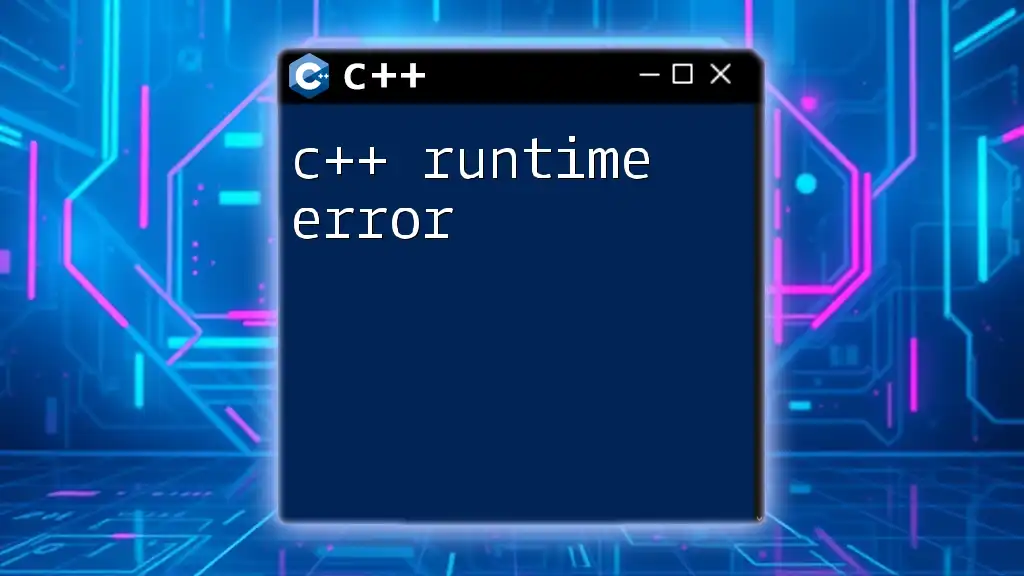
Additional Resources
For those looking to explore the C++ runtime library further, consider consulting the official C++ documentation. Various books and online tutorials are also available to deepen your understanding and practical knowledge.
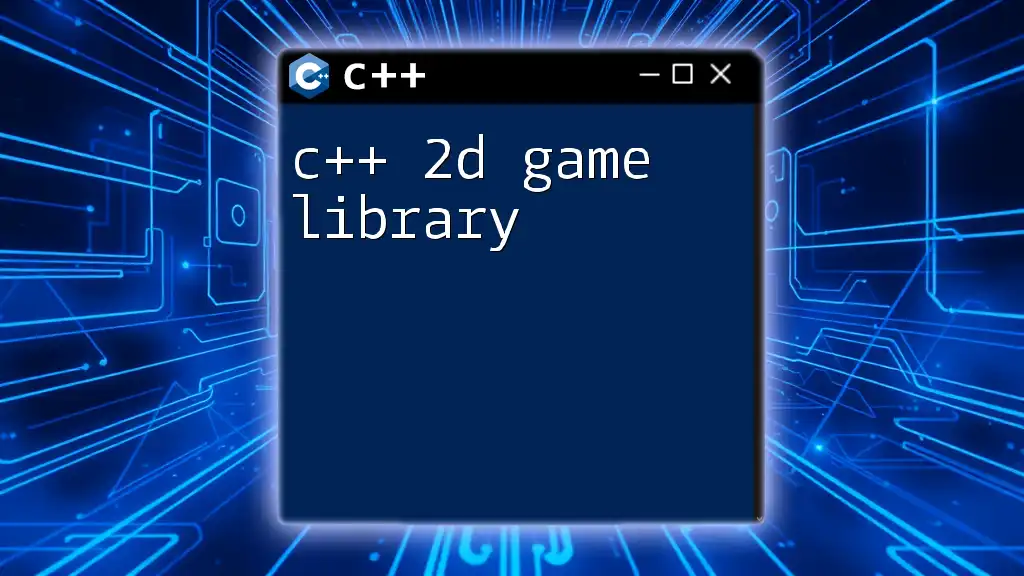
Call to Action
Stay tuned for more programming insights and feel free to share your experiences and tips about the C++ runtime library! Join our community for regular updates, tutorials, and discussions aimed at enhancing your programming journey.