The C++ fmt library is a powerful formatting library that provides a type-safe way to format strings and output data, similar to Python's f-strings.
Here’s a simple example of using the fmt library:
#include <fmt/core.h>
int main() {
const int number = 42;
fmt::print("The answer is {}.\n", number);
return 0;
}
What is the FMT Library?
The C++ FMT library is a powerful formatting library designed to provide an easy and safe way to format strings in C++. Unlike traditional methods such as `printf` or using `iostream`, FMT offers modern solutions that promote clarity and type safety in your code. Formatting text is a common task in programming, and having an efficient tool like FMT can significantly enhance readability and maintainability.
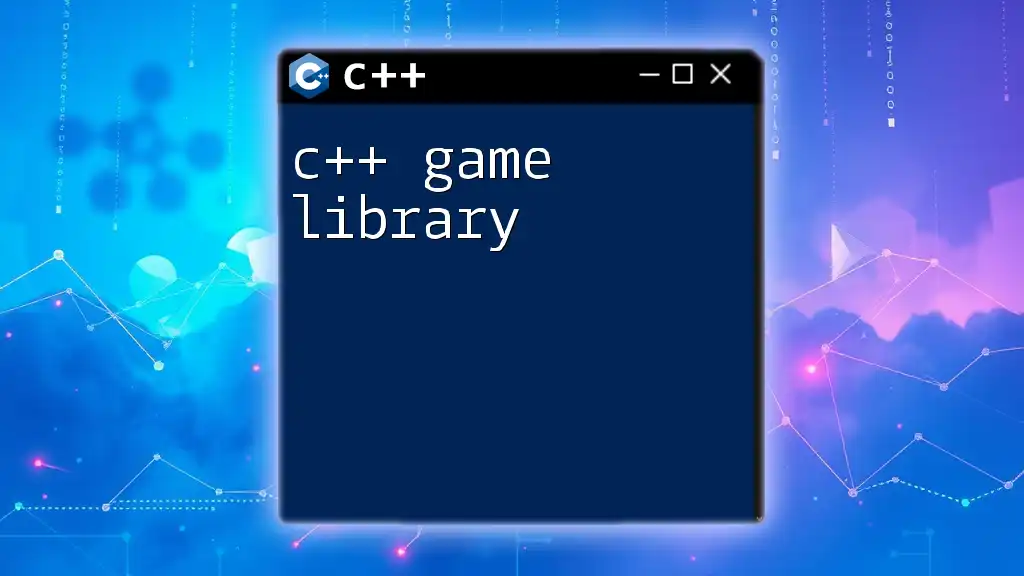
Why Use the FMT Library?
The FMT library stands out for several reasons:
- Type Safety: FMT checks format strings at compile time, reducing runtime errors.
- Ease of Use: Its concise syntax allows developers to format strings with minimal code.
- Performance: FMT is optimized for performance, making it faster than traditional formatting functions.
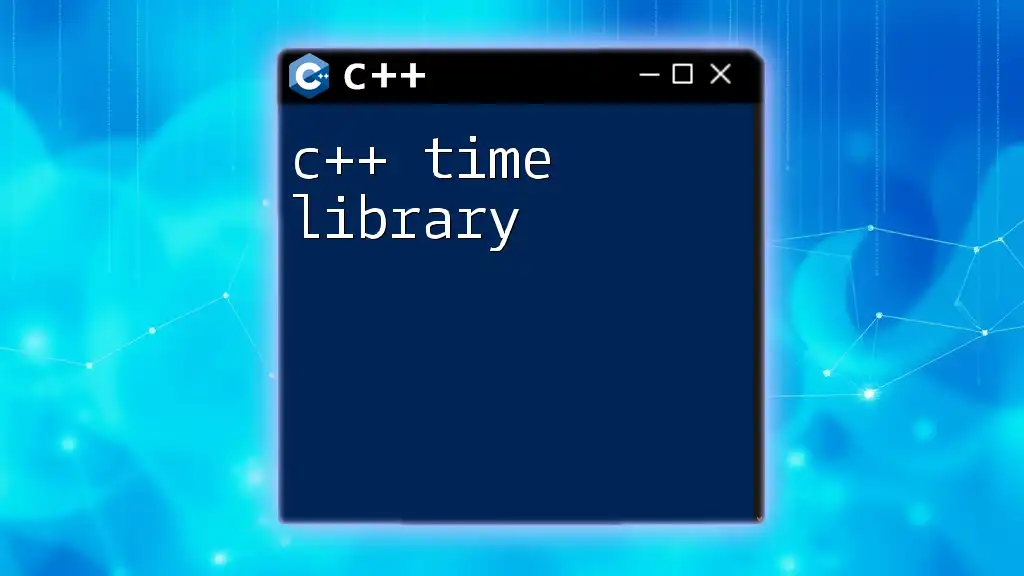
Installation of FMT Library
To get started with the C++ FMT library, you'll first need to install it. Here are the common methods:
-
Using Package Managers: You can install FMT via popular C++ package managers like:
- vcpkg:
vcpkg install fmt
- Conan:
conan install fmt/[version]@bincrafters/stable
- vcpkg:
-
Manual Installation: You can clone the repository and build the library from source:
git clone https://github.com/fmtlib/fmt.git cd fmt mkdir build && cd build cmake .. make sudo make install
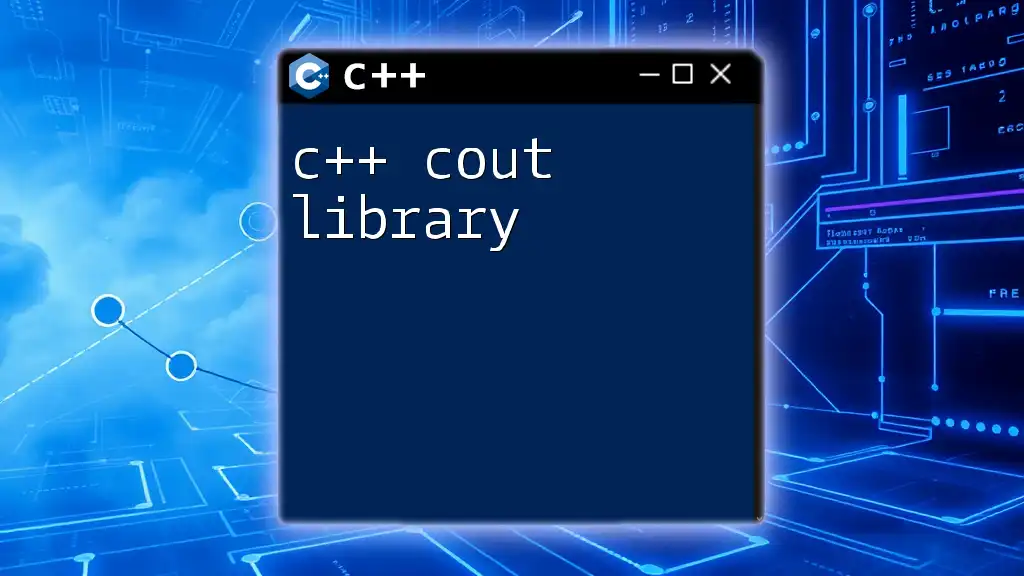
Basic Setup in Your C++ Project
Once installed, you can easily include the FMT library into your C++ projects. Typically, you will add it to your includes:
#include <fmt/core.h>
int main() {
fmt::print("Hello, {}!\n", "World");
return 0;
}
This simple example showcases how FMT allows you to format output cleanly and effectively with the `fmt::print` function.
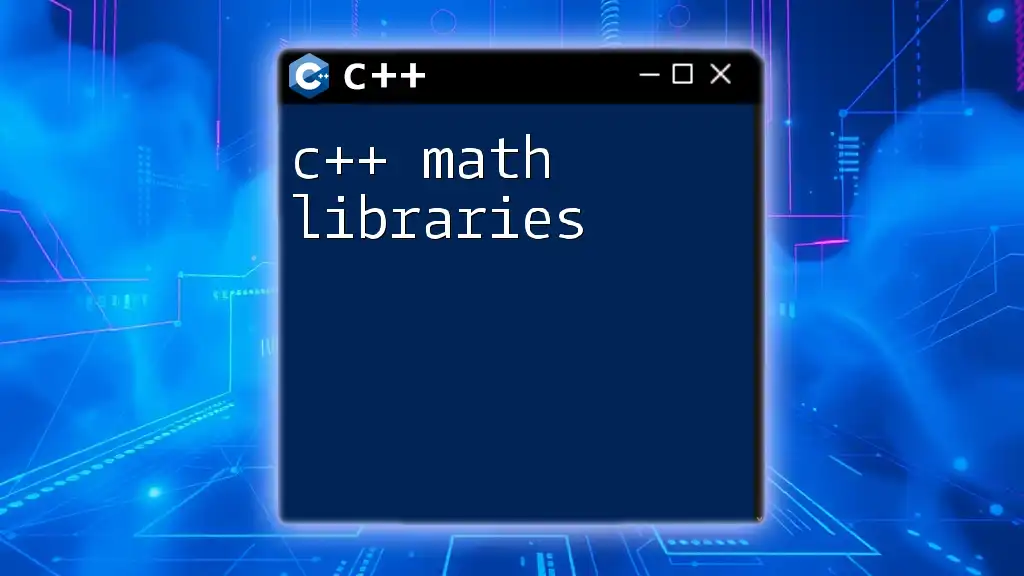
Formatting Strings with FMT
The heart of the FMT library lies in its string formatting capabilities. Using `fmt::format()`, you can format strings with ease. This can be as simple as inserting variables into strings.
Example with Variables:
std::string name = "Alice";
int age = 30;
fmt::print("Hello, {}! You are {} years old.\n", name, age);
In this example, the placeholders `{}` are replaced by the corresponding variables. This approach not only improves readability but also minimizes the risk of format specifier mismatches found in traditional methods.
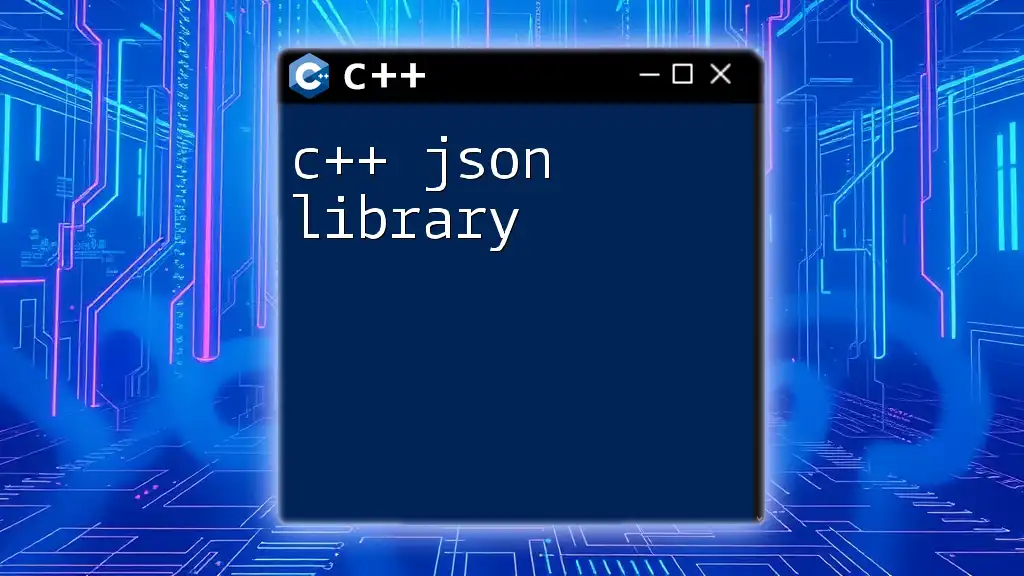
Localization Support
The FMT library also supports localization, which is crucial for building applications that cater to diverse user bases. You can define how numbers, currencies, and dates are formatted based on locale settings, making your application more globally friendly.
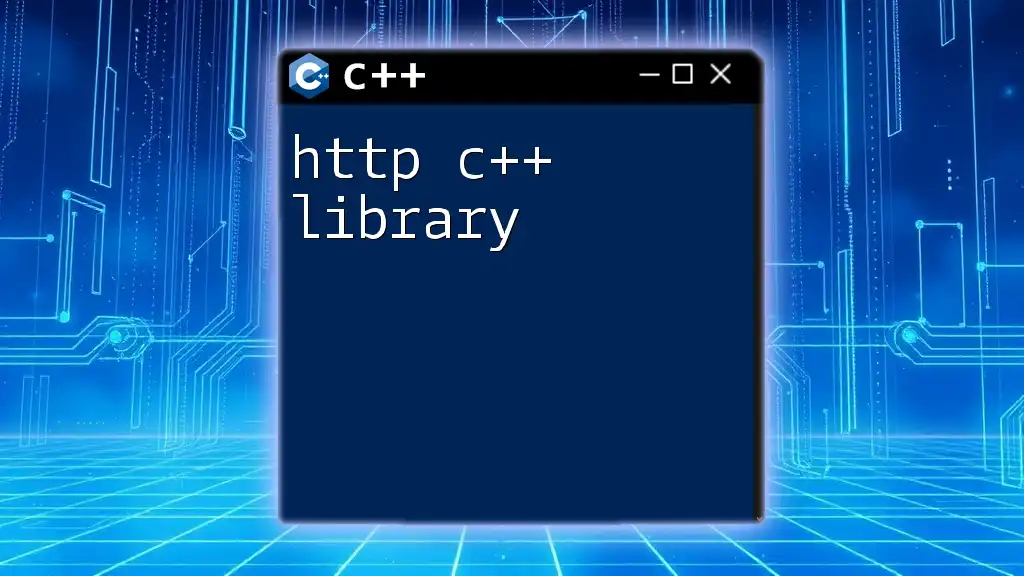
Custom Type Support
One of FMT's strong suits is allowing users to format custom types seamlessly. You can extend FMT to format user-defined types by specializing the `fmt::formatter` template.
Example Code:
struct Point {
int x, y;
};
template <>
struct fmt::formatter<Point> {
template <typename ParseContext>
constexpr auto parse(ParseContext &ctx) {
return ctx.begin();
}
template <typename FormatContext>
auto format(const Point &p, FormatContext &ctx) {
return fmt::format_to(ctx.out(), "({}, {})", p.x, p.y);
}
};
Point p{10, 20};
fmt::print("Point is: {}\n", p);
This customization enables you to define how instances of `Point` will be printed, allowing for readable and formatted output of complex data structures.
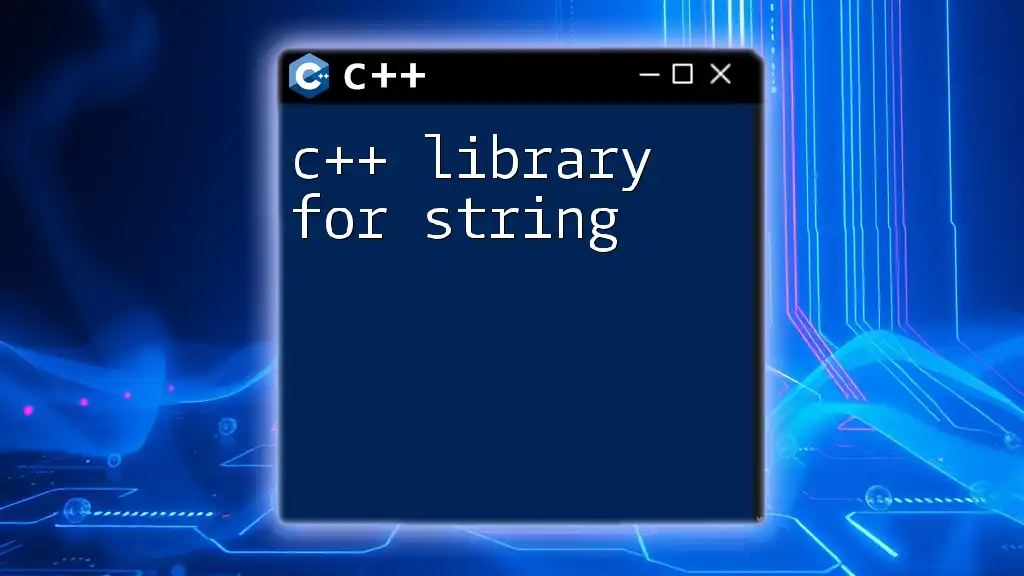
Advanced Formatting Techniques
Formatting Numbers and Dates
Apart from standard string formatting, FMT offers advanced formatting options for numbers and dates. This includes controlling the number of decimal places with precision.
Code Snippet:
double pi = 3.14159;
fmt::print("Pi to 2 decimal places: {:.2f}\n", pi);
In this snippet, the `:.2f` format specifier formats `pi` to two decimal places, showcasing FMT's power to handle numeric formats effortlessly.
Using Named Arguments
Named arguments allow you to improve the clarity of your formatting calls. By specifying names, developers can enhance readability and reduce errors.
Example:
fmt::print("Name: {name}, Age: {age}\n", fmt::arg("name", "Bob"), fmt::arg("age", 25));
This makes it clear what each value represents, as opposed to relying solely on their position within the string.
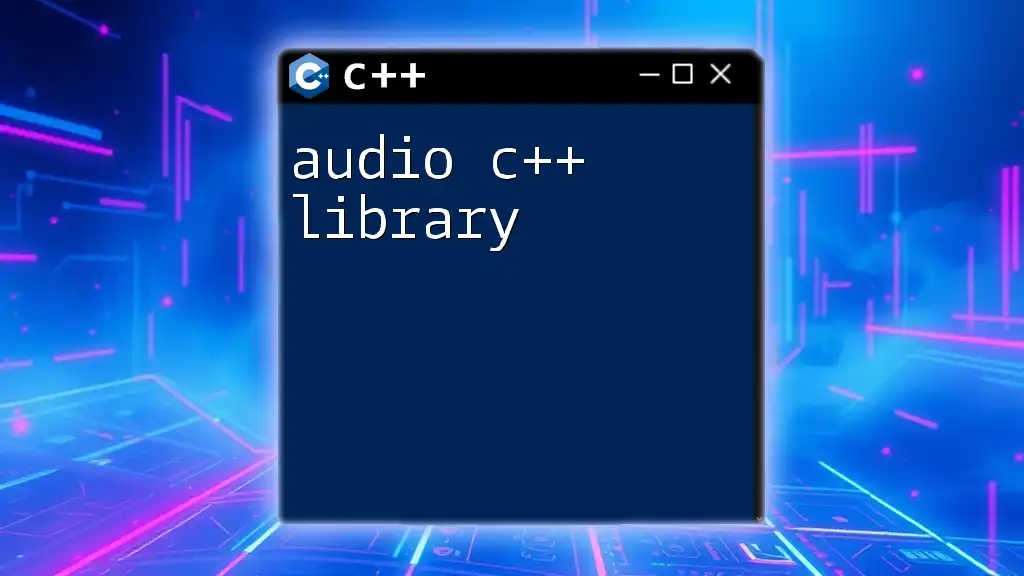
Error Handling and Safety
The FMT library excels in compile-time error checking. It detects mismatches between format specifiers and values during compilation, which helps to catch bugs early in the development process.
Handling User Input Safely
When dealing with user input, it's crucial to ensure that your program is safe from potential issues like buffer overflows. FMT's type-safe nature significantly reduces these risks, making it a preferable choice for string formatting.
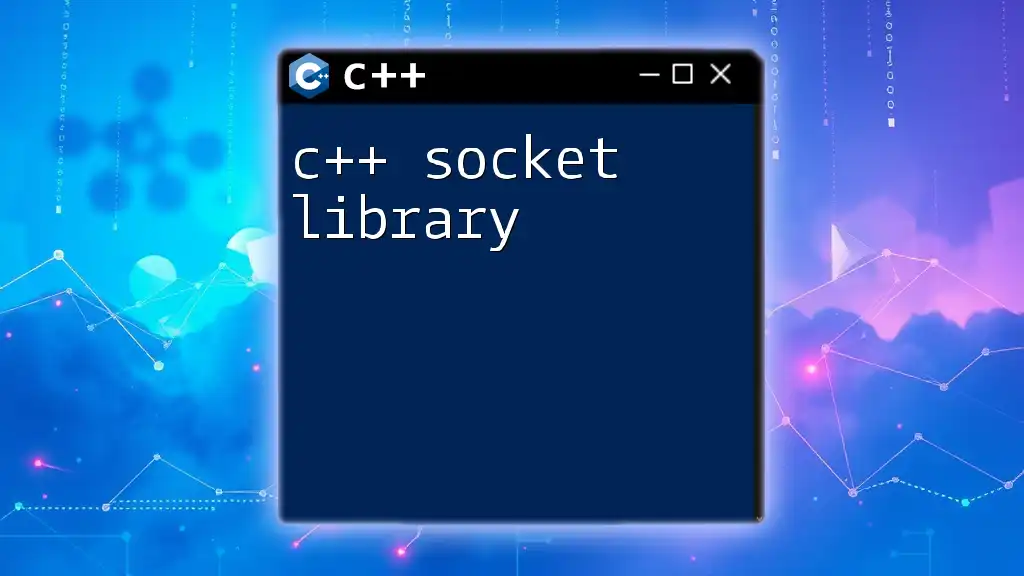
Performance Benefits of FMT
When comparing the performance of the C++ FMT library with traditional functions such as `printf` or iostream, FMT typically outperforms these legacy methods due to its modern architecture and optimizations. Benchmarks show that FMT is faster and uses less memory, making it an excellent choice for performance-critical applications.
Memory Safety
The FMT library's design inherently reduces the risk of buffer overflows, a common vulnerability in C++ applications. By enforcing type-safe format strings, developers can write safer code with major formatting operations.
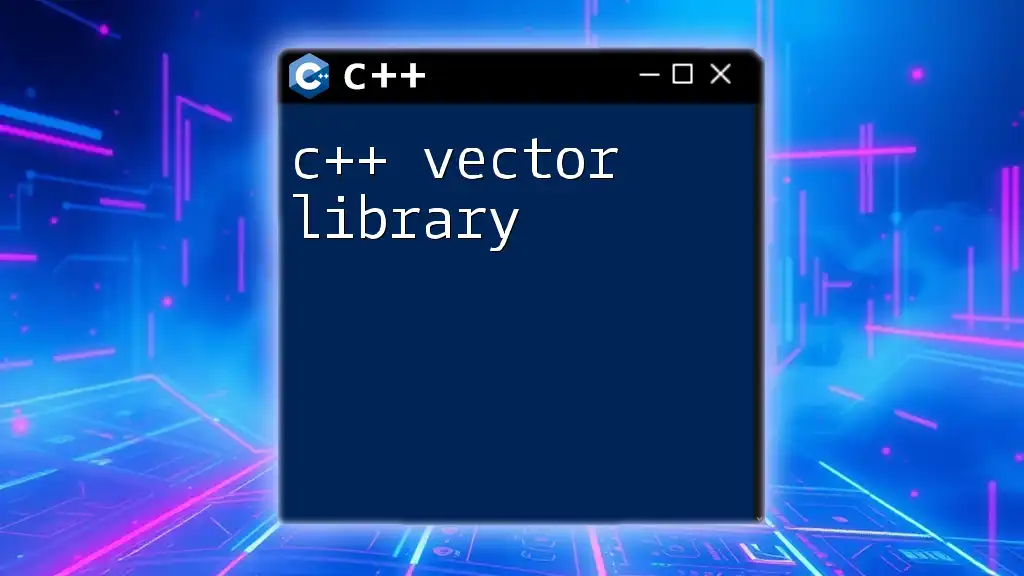
Common Use Cases for FMT
Debugging and Logging
FMT is invaluable for debugging and logging purposes. The ability to format log messages concisely can greatly enhance the clarity of debugging output and error messages in production systems.
Building User Interfaces
When developing GUI applications, having the ability to format strings dynamically is a necessity. With FMT, you can easily construct user-friendly messages and display relevant information with proper formatting.
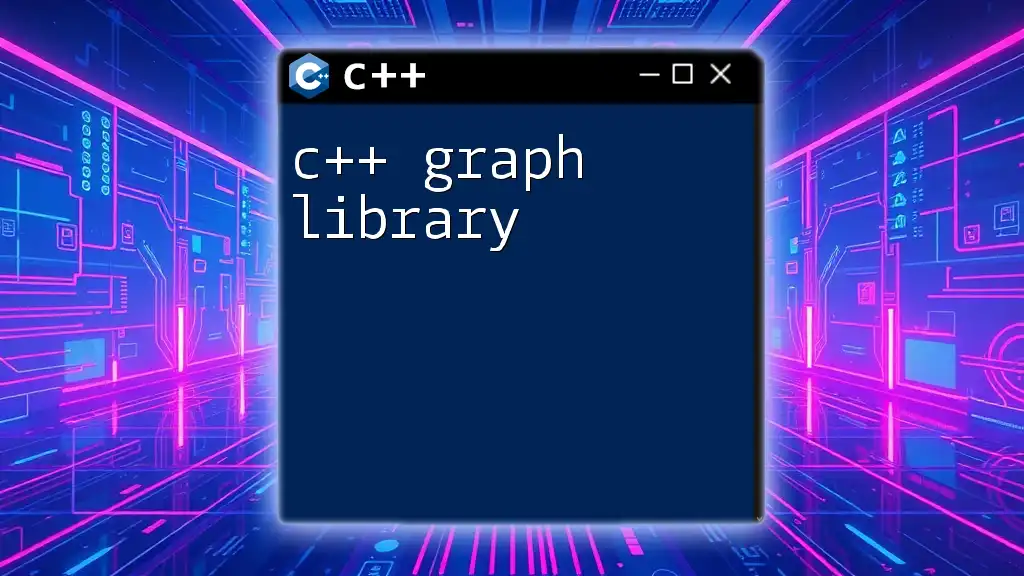
Conclusion
In summary, the C++ FMT library offers significant advantages over traditional formatting methods. Its modern features, such as type safety, ease of use, and performance benefits, make it an essential tool for any C++ developer aiming to produce high-quality, maintainable code. By adopting the FMT library in your projects, you can streamline string formatting and improve both development efficiency and application robustness.
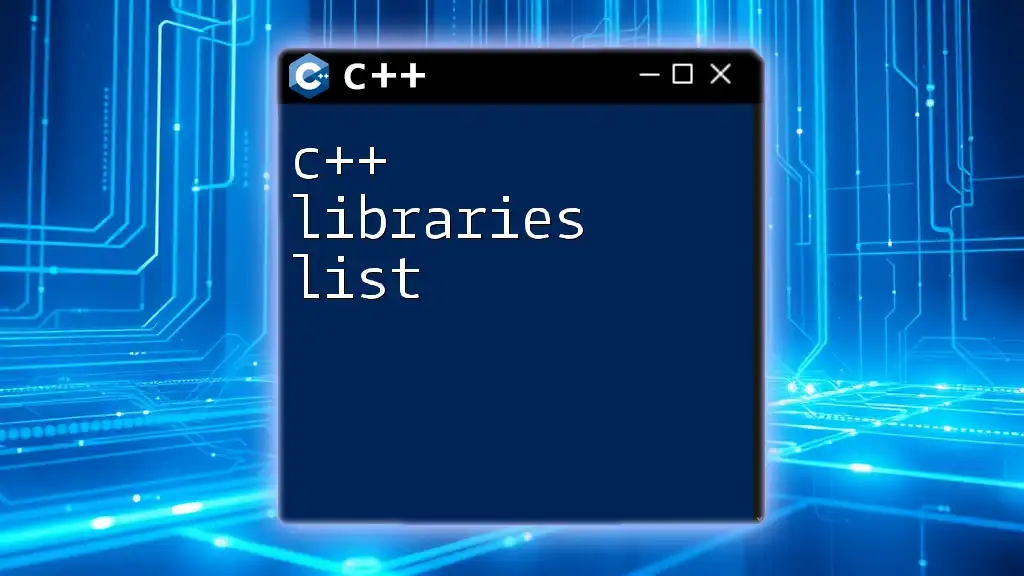
Additional Resources
For further learning, consider diving into the official FMT documentation, which provides in-depth guides and examples. Engaging with community forums and resources can also enhance your understanding and provide additional support as you explore the capabilities of the FMT library.
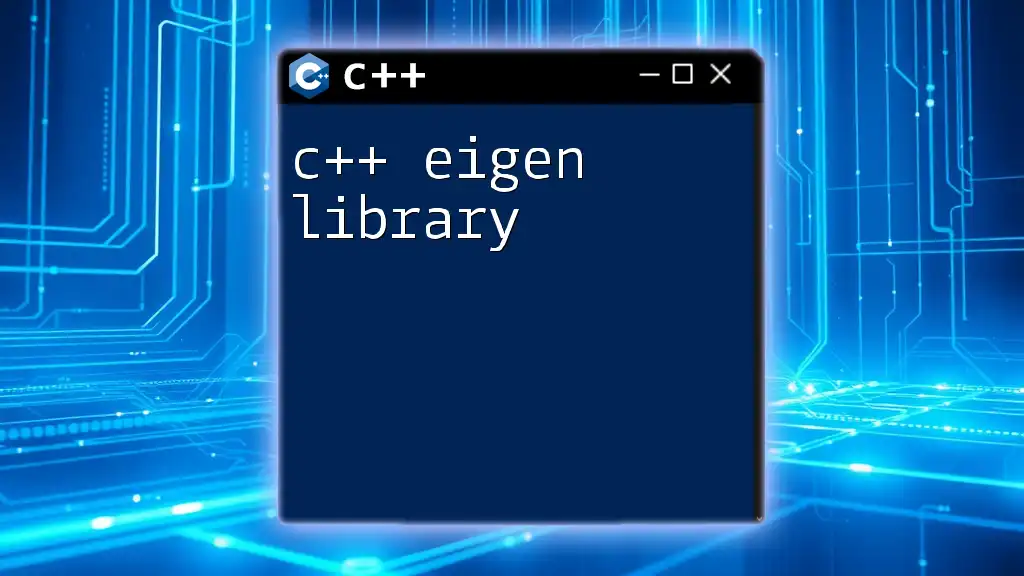
Call to Action
Experiment with the FMT library in your next C++ project. With its powerful formatting capabilities, you're likely to find that it not only improves the quality of your code but also enhances your programming experience. Don't hesitate to share your successes and challenges; tapping into the community can provide invaluable insights!