The HTTP C++ library allows developers to easily manage HTTP requests and responses within their C++ applications, enabling seamless web communication.
#include <iostream>
#include <cpprest/http_client.h>
using namespace web; // Common features like URIs.
using namespace web::http; // Common HTTP features.
using namespace web::http::client; // HTTP client features.
int main() {
http_client client(U("http://www.example.com"));
client.request(methods::GET).then([](http_response response) {
if (response.status_code() == status_codes::OK) {
std::cout << "Response: " << response.to_string() << std::endl;
}
}).wait();
return 0;
}
What is an HTTP Library in C++?
An HTTP library in C++ is a collection of classes and functions designed to facilitate the sending and receiving of HTTP requests and responses. These libraries abstract the complexities involved in making network calls, allowing developers to focus on the application's functionality rather than the lower-level networking details.
Using a library eliminates the need to handle HTTP protocol intricacies manually, saving time and reducing the likelihood of bugs. For example, here's how you might send an HTTP GET request without a library:
// Example of manual HTTP request (simplified)
boost::asio::io_context io_context;
tcp::resolver resolver(io_context);
auto endpoints = resolver.resolve("example.com", "80");
tcp::socket socket(io_context);
boost::asio::connect(socket, endpoints);
const std::string request = "GET / HTTP/1.1\r\nHost: example.com\r\n\r\n";
boost::asio::write(socket, boost::asio::buffer(request));
In contrast, utilizing an HTTP library simplifies this process significantly.
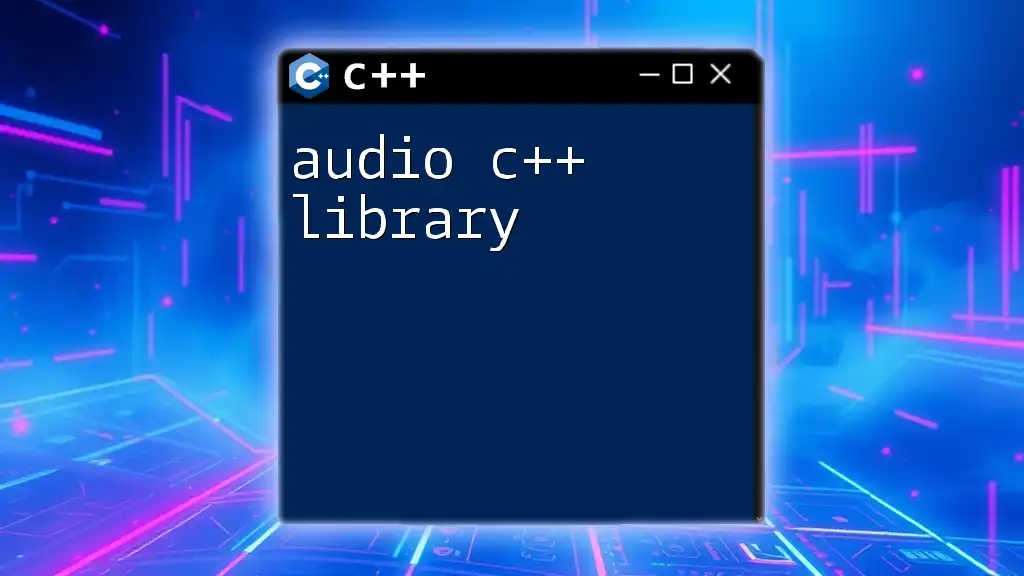
Key Features of C++ HTTP Libraries
Support for Different Protocols
Most modern C++ HTTP libraries support multiple versions of the HTTP protocol, especially HTTP/1.1 and HTTP/2. Each version has its own set of features, with HTTP/2 providing better performance through multiplexing and header compression. Understanding the differences allows developers to optimize their applications and make the best use of available resources.
Asynchronous Operations
One of the main benefits of using an HTTP library is its support for asynchronous operations. Non-blocking calls allow other processes to run while waiting for server responses, improving the application's responsiveness. In networking applications, where waiting for a response can introduce latency, asynchronous operations are crucial.
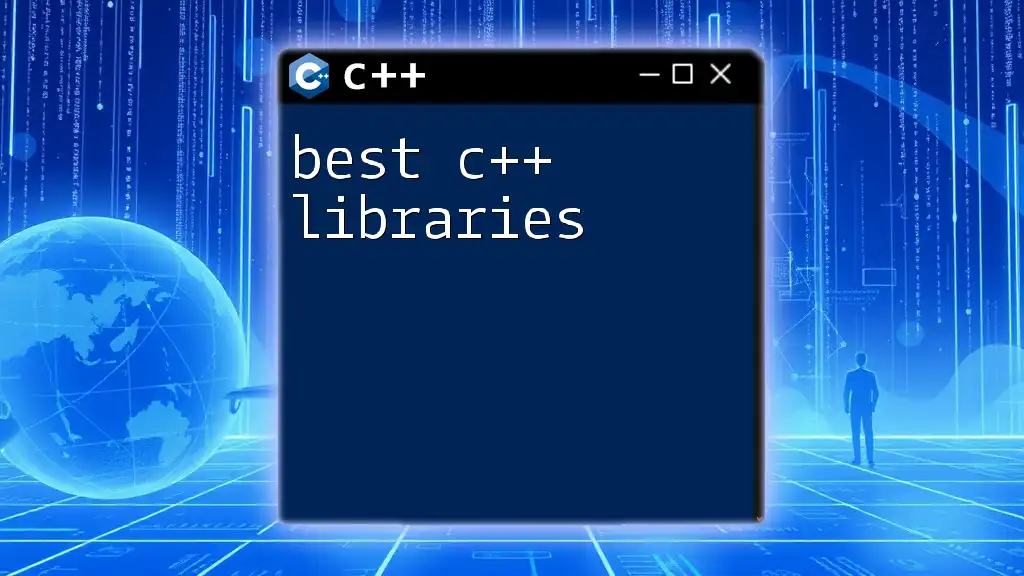
Popular C++ HTTP Libraries
cURL
cURL is perhaps the most widely recognized C++ HTTP library. It's versatile, supports a wide range of protocols, and boasts extensive documentation.
Key Features:
- Protocol Support: HTTP, HTTPS, FTP, SMTP, and more.
- Comprehensive Documentation: Easy to find examples and troubleshooting help.
Code Example: Here's a simple example of how to make an HTTP GET request using cURL:
#include <curl/curl.h>
#include <iostream>
int main() {
CURL* curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
CURLcode res = curl_easy_perform(curl);
if(res != CURLE_OK) {
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
curl_easy_cleanup(curl);
}
return 0;
}
Boost.Beast
Boost.Beast builds on the foundations of the Boost library, providing robust mechanisms for asynchronous programming and HTTP communication.
Key Features:
- Integration with Boost.Asio for networking.
- Offers both synchronous and asynchronous APIs, enhancing flexibility.
Code Example: Here’s how you implement a simple HTTP client:
#include <boost/beast/core.hpp>
#include <boost/beast/http.hpp>
#include <boost/asio.hpp>
namespace beast = boost::beast;
namespace http = beast::http;
int main() {
boost::asio::io_context io_context;
// Create a resolver and perform a DNS lookup
boost::asio::ip::tcp::resolver resolver(io_context);
// Rest of the code for sending the HTTP GET request
}
Poco C++ Libraries
The Poco C++ Libraries provide an easy-to-use HTTP client interface that integrates seamlessly with other Poco components.
Key Features:
- Simple and powerful interface.
- Tight integration with other Poco libraries for database access, XML parsing, etc.
Code Example: A simple GET request can be implemented as follows:
#include <Poco/Net/HTTPClientSession.h>
#include <Poco/Net/HTTPResponse.h>
#include <Poco/Stream.h>
#include <iostream>
int main() {
Poco::Net::HTTPClientSession session("example.com");
Poco::Net::HTTPRequest request(Poco::Net::HTTPRequest::HTTP_GET, "/");
session.sendRequest(request);
Poco::Net::HTTPResponse response;
std::istream& rs = session.receiveResponse(response);
// Print response status
std::cout << response.getStatus() << " " << response.getReason() << std::endl;
return 0;
}
Restbed
Restbed is tailored for creating RESTful applications and simplifies asynchronous programming.
Key Features:
- High performance and scalability due to its non-blocking model.
- Flexible session handling and middleware capability.
Code Example: Creating a simple REST service can be easily done using Restbed:
#include <restbed>
void get_handler(const std::shared_ptr<restbed::Session> session) {
// Logic for handling GET request
}
int main() {
auto resource = std::make_shared<restbed::Resource>();
resource->set_path("/example");
resource->set_method_handler("GET", get_handler);
restbed::Service service;
service.publish(resource);
service.start();
}
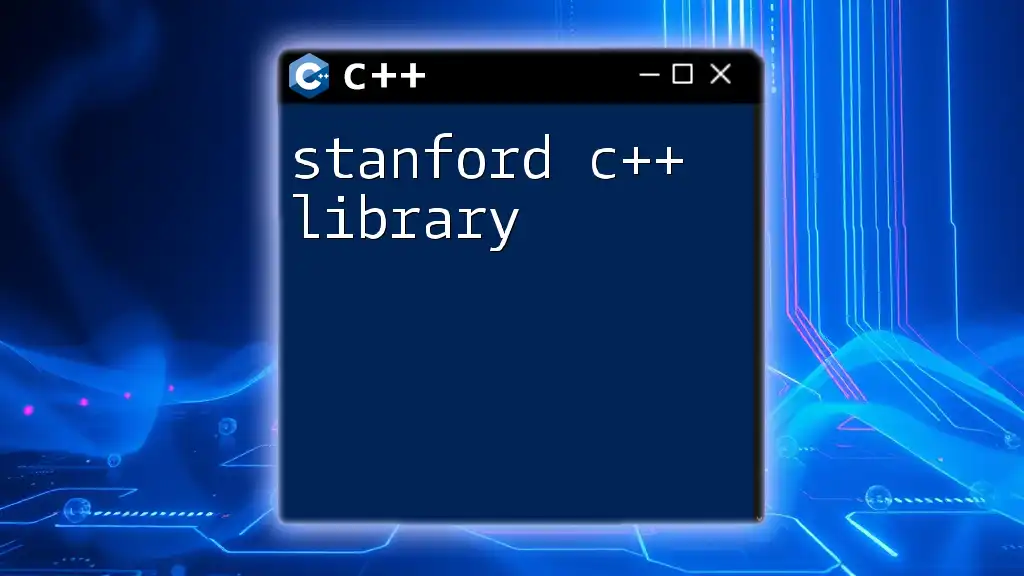
How to Choose the Right C++ HTTP Library
Consider Your Project Requirements
When selecting an HTTP C++ library, assess the specific needs of your project. A small utility tool may not require all the features of a heavy library. Similarly, a high-performance application may benefit from libraries that prioritize speed and efficiency.
Documentation and Community Support
Choose a library with strong documentation and an active community. This ensures that you'll have access to resources and support, making debugging and enhancing your implementation significantly easier.
Performance Metrics
Evaluate the performance through benchmarking. Consider how each library responds to concurrent requests and the throughput under different loads to ensure it will meet your application's demands.
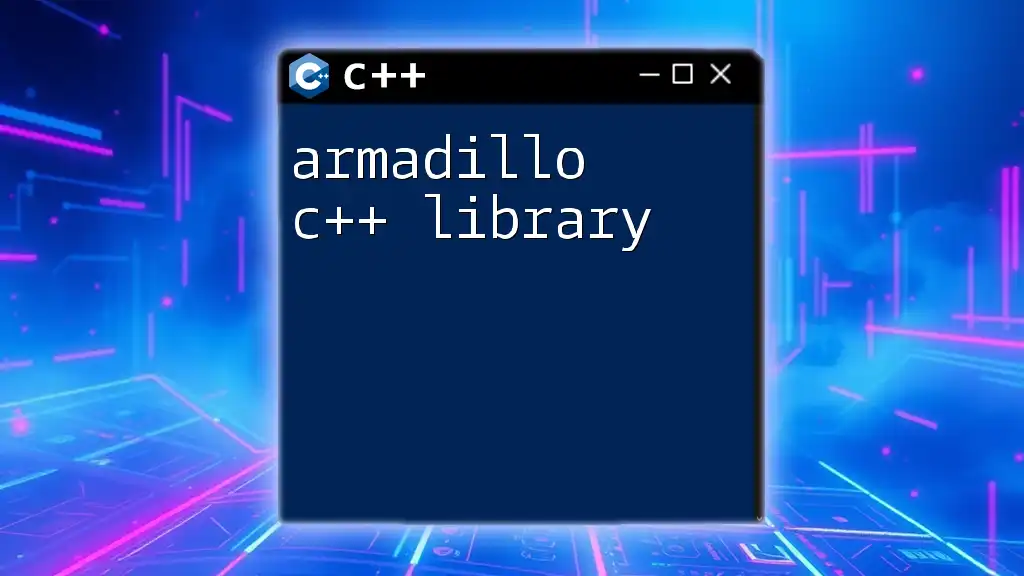
Best Practices for Using C++ HTTP Libraries
Understanding Configuration Options
Take the time to familiarize yourself with configuration options offered by the library. Settings such as timeouts, maximum connections, and retry policies can have a significant impact on how your application interacts with remote servers.
An example code snippet showing how to configure the request:
curl_easy_setopt(curl, CURLOPT_TIMEOUT, 10L); // Set timeout to 10 seconds
curl_easy_setopt(curl, CURLOPT_FOLLOWLOCATION, 1L); // Allow redirections
Security Considerations
Always prioritize security. Utilize HTTPS instead of HTTP to encrypt data in transit. Be aware of common vulnerabilities such as man-in-the-middle attacks and SQL injection, ensuring that your code follows best practices for security.
Testing Your HTTP Code
Testing is vital for reliable HTTP interactions. Utilize frameworks like Catch2 for unit testing your code or tools like Postman to manually inspect API responses. Consider both functional tests to verify correct behavior and performance tests to load test your application.
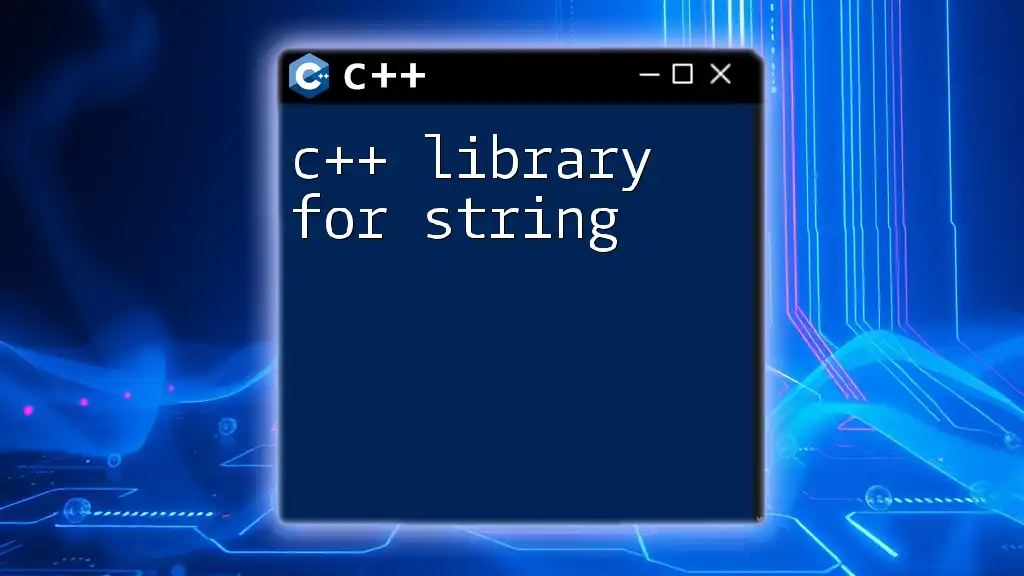
Conclusion
C++ HTTP libraries are indispensable tools for developers aiming to build robust and efficient networked applications. With various libraries available, each with unique features and capabilities, choosing the right one is crucial. By experimenting with these libraries and integrating best practices, developers can enhance their proficiency in HTTP communications and optimize their applications for a wide range of use cases.
Be sure to explore the libraries mentioned in this guide and consider implementing your own HTTP requests today!