The C++ Stack library provides a container adapter that follows the Last In First Out (LIFO) principle, allowing users to efficiently manage and manipulate a collection of elements.
Here's a simple example of how to use the Stack library in C++:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
// Push elements onto the stack
myStack.push(1);
myStack.push(2);
myStack.push(3);
// Access the top element
std::cout << "Top element: " << myStack.top() << std::endl;
// Pop the top element
myStack.pop();
std::cout << "Top element after pop: " << myStack.top() << std::endl;
return 0;
}
Understanding the Stack Data Structure
A stack is a fundamental data structure widely used in programming, characterized by the Last In, First Out (LIFO) principle. It means that the last element added to the stack is the first one to be removed. This structure is especially useful in scenarios where you need to maintain an order of operations or track changes that can be undone.
Real-world applications of stacks include managing function calls (the call stack), undo functionalities in applications, and parsing expressions in compilers.
Basic Operations on Stacks
The core operations that a stack supports include:
- Push: Adds an element to the top of the stack.
- Pop: Removes the top element from the stack.
- Peek/Top: Retrieves the top element without removing it from the stack.

C++ Stack Library Overview
The stack library in C++ is a part of the Standard Template Library (STL). It treats stacks as template classes, allowing for a vast range of data types to be used, enhancing the library’s usability. This template-based approach also ensures that various data types can leverage stack functionalities without needing to implement custom stack logic.
How the Stack Library is Implemented
Internally, the C++ stack is implemented with a combination of data structures such as deques or vectors. This design decision offers flexibility in terms of memory management and performance.

Getting Started with C++ Stack Library
To utilize the stack library in C++, you must first include the appropriate header file:
#include <stack>
This inclusion gives you access to the stack functionalities provided by the STL.
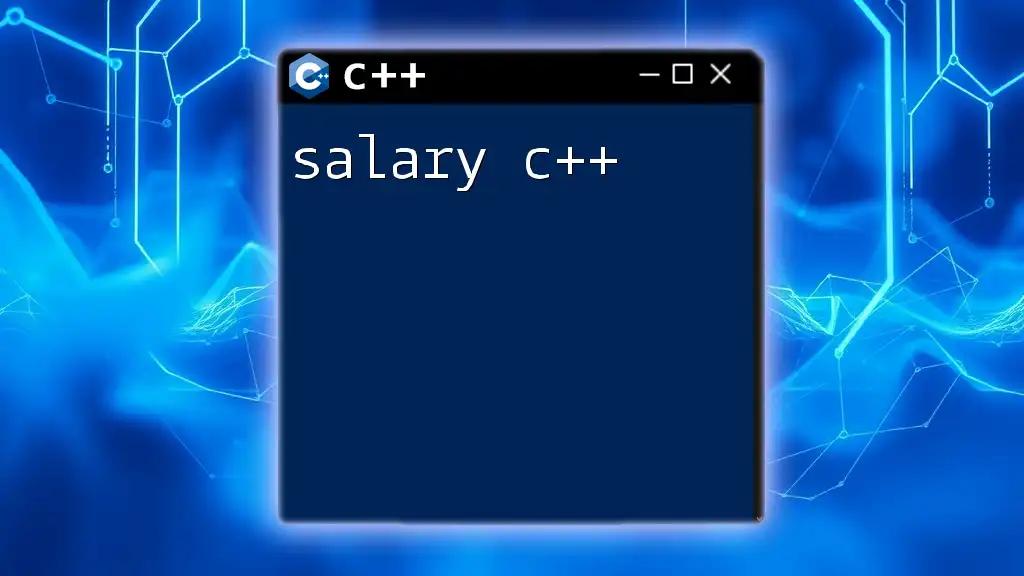
Creating a Stack in C++
Creating a stack in C++ is straightforward. You can declare a stack of any data type you wish. For example, to create a stack that holds integers, you can do the following:
std::stack<int> myStack;
Initializing the Stack with Values
You can also initialize the stack with values. For instance, if you want to push five integers onto the stack, you can do this using a loop:
for(int i = 0; i < 5; ++i) {
myStack.push(i);
}

Common Stack Operations
Pushing Elements onto the Stack
The `push()` method allows you to add elements to your stack. For example:
myStack.push(10);
myStack.push(20);
Each call to `push` places a new integer at the top of the stack.
Popping Elements from the Stack
To remove the top element from the stack, you can use the `pop()` method. This method ensures that the top element is discarded, effectively reducing the stack size by one:
myStack.pop(); // Removes the top element
Accessing the Top Element
To get the value of the top element without removing it, the `top()` method is utilized. It provides a way to access the stack's most recent addition:
int topElement = myStack.top();
Checking Stack Size
To check how many elements are currently in the stack, use the `size()` method:
std::cout << "Stack size: " << myStack.size() << std::endl;
This operation will return the number of elements currently held within the stack.
Checking if the Stack is Empty
Before performing `pop()` or `top()`, it's wise to confirm that the stack isn't empty. The `empty()` method allows you to check this:
if(myStack.empty()) {
std::cout << "Stack is empty." << std::endl;
}
Using this method prevents exceptions related to accessing or removing elements from an empty stack.

Best Practices for Using the Stack Library
When working with the stack library in C++, consider the following best practices:
- Choosing the Right Data Type: Ensure that the datatype you consist of aligns with your program’s needs.
- Avoiding Memory Leaks: Properly manage the lifecycle of the stack, particularly in complex applications.
- Error Handling: Always check for conditions (like stack underflow) when performing `pop()` and `top()` operations.

Performance Considerations
The stack operations in C++ have well-defined time complexities:
- Push: O(1)
- Pop: O(1)
- Top: O(1)
- Size: O(1)
- Empty: O(1)
These operations are very efficient, making the stack library an excellent choice for applications requiring rapid access and modification of data.

Common Use Cases for Stacks in C++
Understanding how to leverage stacks can elevate your programming skills. Here are some common applications:
- Undo Mechanisms: Many text editors use stacks to manage the previous state of documents, allowing for undo and redo functionalities.
- Syntax Parsing: Stacks are crucial in parsing expressions in compilers and interpreters, helping to evaluate mathematical and logical expressions.
- Backtracking Algorithms: Algorithms like Depth-First Search (DFS) utilize stacks to keep track of paths and nodes visited.
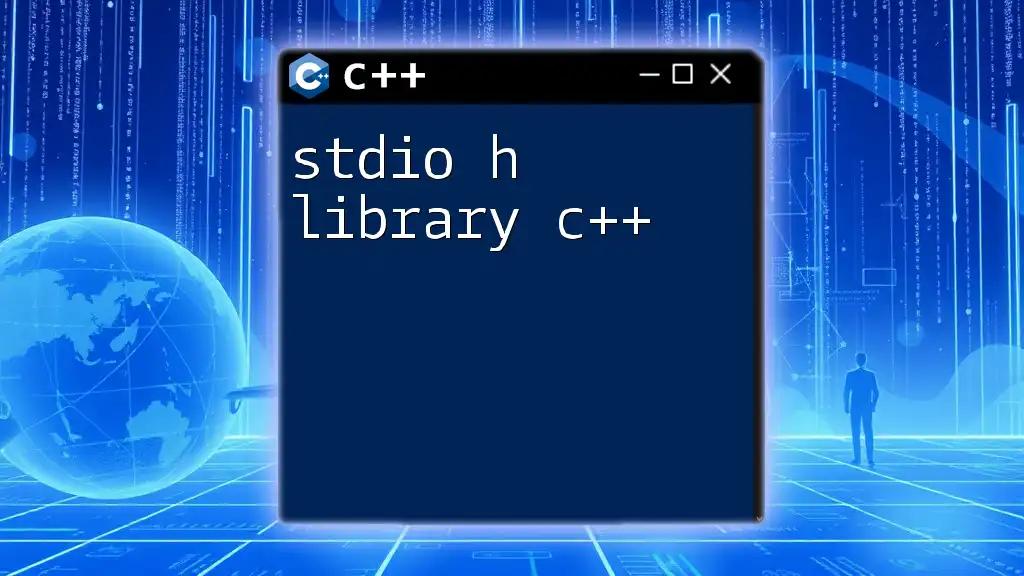
Conclusion
The stack library in C++ is a powerful and intuitive tool for managing data in a specific LIFO order. By understanding its fundamental structure and operations, you can implement efficient solutions to various computational problems. Utilize this guide as a starting point to explore more complex data structures and their applications in your programming journey.

Additional Resources
To further your knowledge of the stack library in C++, consider diving into the recommended books and tutorials available online. Engaging with online communities and forums can also provide additional support and enhance your learning experience.