The C++ Standard Library provides a rich set of functions, classes, and templates that enhance the functionality of the C++ language, allowing developers to perform common tasks with ease.
Here's a simple example demonstrating the use of the `<iostream>` library to output text:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Introduction to C++ Libraries
Importance of C++ Libraries
Libraries play a crucial role in programming by providing pre-written code that can be reused, significantly enhancing productivity and reducing error rates. In C++, libraries allow developers to access a rich set of functionalities without needing to write everything from scratch. This not only saves time but also ensures that the code is optimized and widely tested.
What is a C++ Library?
A C++ library is a collection of precompiled routines that a program can use. Libraries can be divided mainly into two categories: Standard Libraries and Third-party Libraries.
- Standard Libraries are included with the C++ language and provide fundamental functionalities.
- Third-party Libraries offer additional features and capabilities, often developed by community or commercial sources.
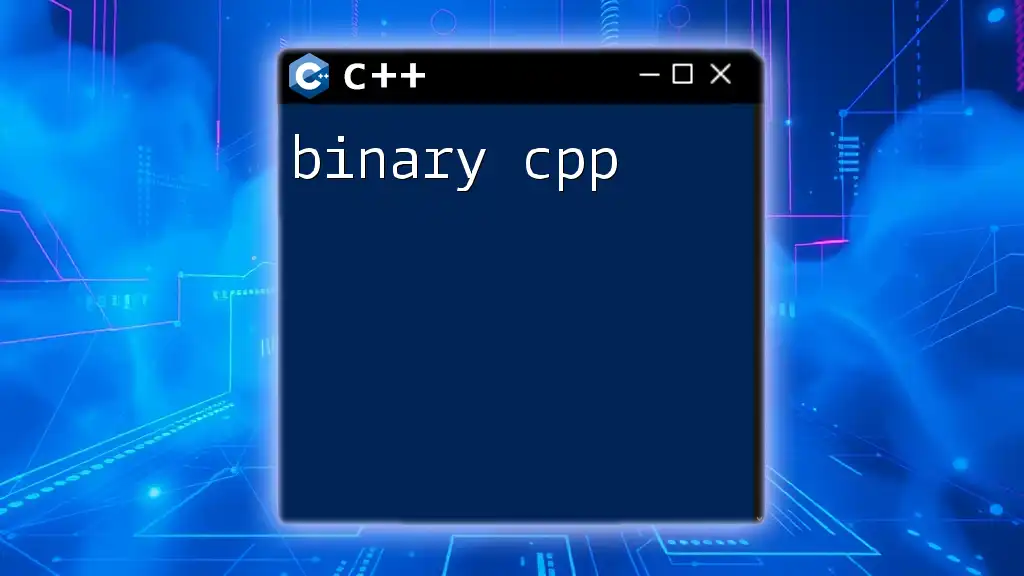
Standard C++ Libraries
Overview of the Standard Template Library (STL)
The Standard Template Library (STL) is a powerful set of C++ template classes to provide generalizations for operations. STL includes:
- Containers: Data structure implementations such as arrays, lists, and maps.
- Algorithms: Functions for searching, sorting, and manipulating data.
- Iterators: Objects that enable the traversal of container contents.
Common Containers in STL
Vectors
Vectors are dynamic arrays that can change size during runtime. They offer several benefits, including fast random access and automatic memory management.
Example of using a vector:
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adding an element
return 0;
}
Lists
The list container implements a doubly linked list. This allows for efficient insertions and deletions at any point within the list.
Example of using a list:
#include <list>
int main() {
std::list<std::string> fruits = {"apple", "banana", "cherry"};
fruits.push_back("date"); // Adding an element
return 0;
}
Maps
Maps store key-value pairs and are commonly used for associative data. They are implemented as binary search trees, which allows for efficient searching and sorting.
Example of using a map:
#include <map>
int main() {
std::map<int, std::string> studentNames = {{1, "John"}, {2, "Alice"}};
studentNames[3] = "Bob"; // Inserting a new pair
return 0;
}
Algorithms in STL
Sorting Algorithms
STL provides various sorting algorithms, such as `std::sort`, which is a highly optimized sorting function that sorts elements in ascending order.
Example of using a sorting algorithm:
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {4, 1, 3, 2};
std::sort(nums.begin(), nums.end());
return 0;
}
Searching Algorithms
Searching algorithms in STL, like `std::find`, allow you to locate elements within a container efficiently.
Example of using a searching algorithm:
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {4, 1, 3, 2};
auto it = std::find(nums.begin(), nums.end(), 3);
return 0;
}
Iterators
Iterators are essential components in STL that provide a way to access elements of a container without exposing the underlying structure. They are categorized into several types:
- Input Iterators: Read-only access to elements.
- Output Iterators: Write-only access to elements.
- Forward Iterators: Read/write access that supports moving forward.
- Bidirectional Iterators: Read/write access to traverse both forward and backward.
- Random Access Iterators: Full access to elements, similar to pointers.
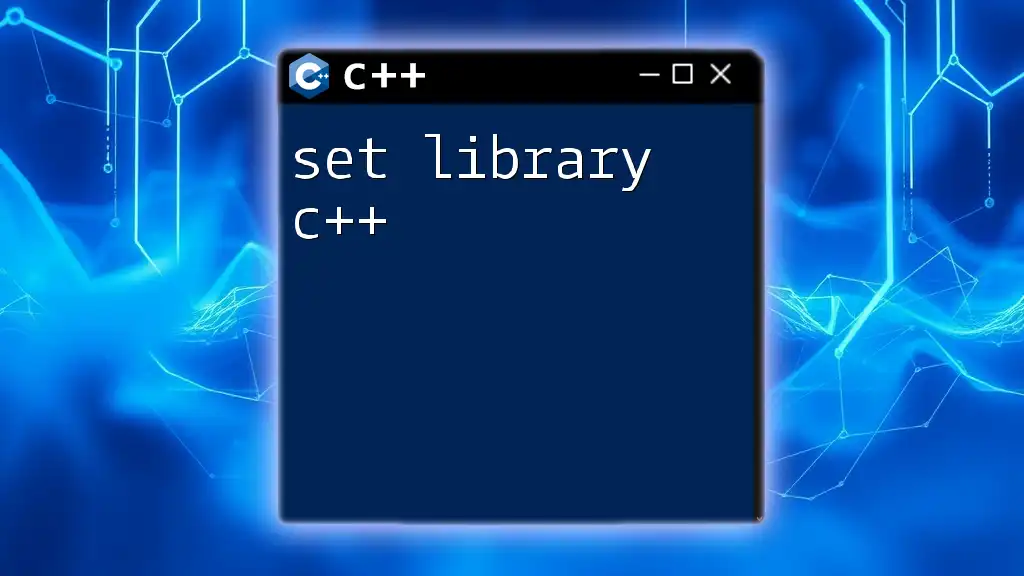
Third-Party C++ Libraries
Popular Third-Party Libraries
Boost
Boost is an influential collection of C++ libraries that extend the functionality of the C++ Standard Library. It offers support for tasks such as multithreading, file manipulation, and smart pointers.
Example of using Boost for smart pointers:
#include <boost/shared_ptr.hpp>
int main() {
boost::shared_ptr<int> ptr(new int(10));
return 0;
}
Qt
Qt is a comprehensive framework designed for developing cross-platform GUI applications. It provides tools for user interface design, networking, and database management.
Basic example in Qt:
#include <QApplication>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
// Additional Qt code to create a window
return app.exec();
}
Advantages of Using Third-Party Libraries
Leveraging third-party libraries allows developers to tap into a wealth of functionalities that can speed up development time. They come with comprehensive documentation, community support, and real-world examples, making it easier to find solutions to common programming challenges.
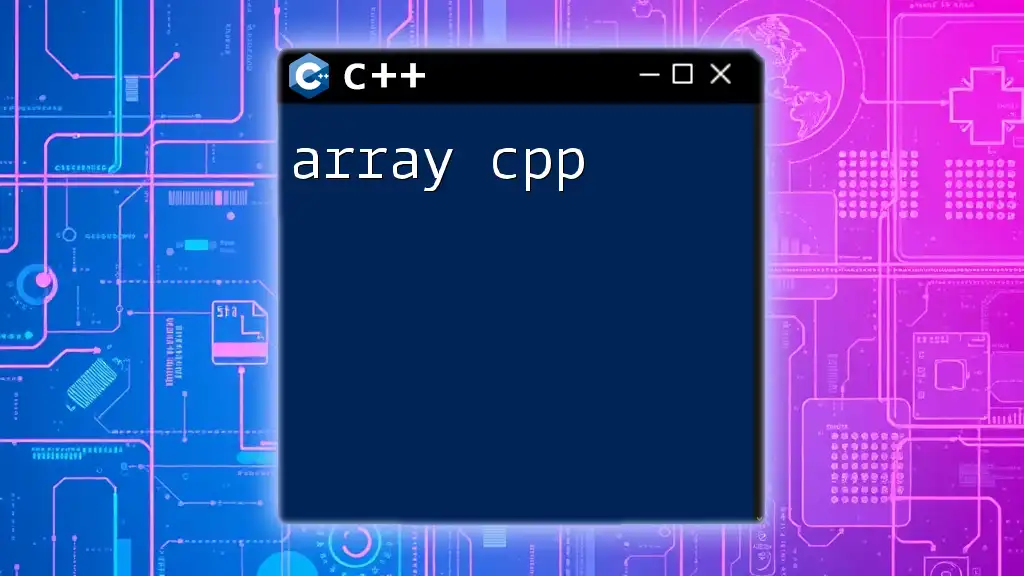
Creating Your Own C++ Library
Why Create Your Own Library?
Creating a custom library allows developers to encapsulate functionality, making it reusable across different projects or applications. This caters to specific needs that might not be addressed by existing libraries.
Steps to Create a C++ Library
Design Considerations
Prior to writing a library, it's vital to establish clear objectives and a structured design. This ensures consistency and maintainability.
Writing Your Library
A straightforward library structure could include a header file and a corresponding implementation file.
Example code snippet for a custom library:
// MyLibrary.h
class MyLibrary {
public:
void display();
};
// MyLibrary.cpp
#include "MyLibrary.h"
#include <iostream>
void MyLibrary::display() {
std::cout << "Hello from MyLibrary!" << std::endl;
}
Compiling and Distributing Your Library
After writing the library, compile it appropriately and consider using package managers or share it through GitHub for broader accessibility.
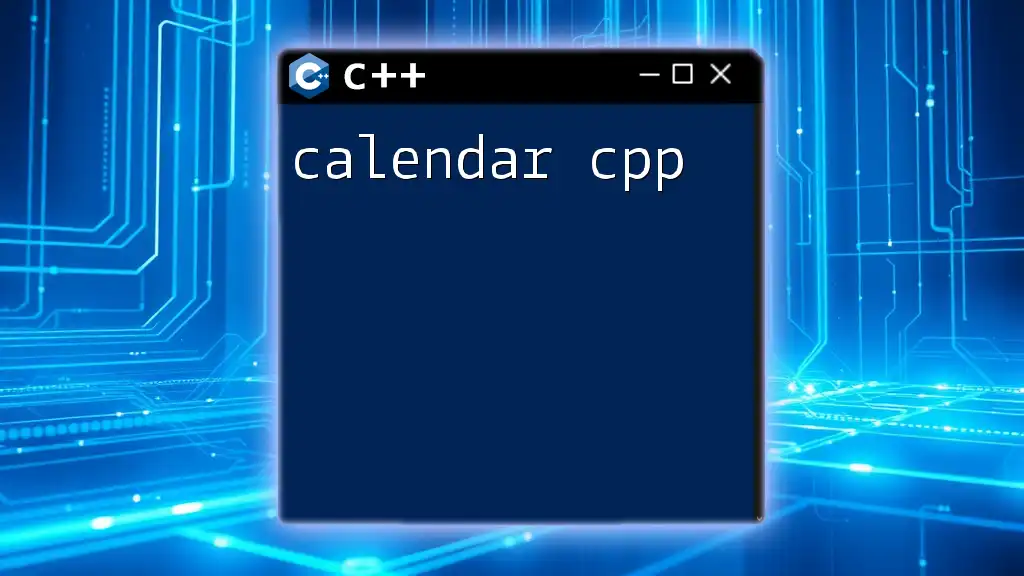
Best Practices for Using C++ Libraries
Documentation
Utilizing proper documentation is crucial when working with libraries. It not only makes it easier to understand how to implement the library but also helps maintain the codebase in the long run. Tools such as Doxygen or Sphinx can generate useful documentation from annotated source code.
Version Control
Managing library versions is vital for ensuring compatibility and preventing issues when integrating into existing projects. Keeping track of changes helps in maintaining an organized development process.
Performance Considerations
Performance deeply influences how libraries impact an application. It's necessary to evaluate the performance of libraries prior to incorporating them; some libraries may introduce overhead that could affect computational efficiency.
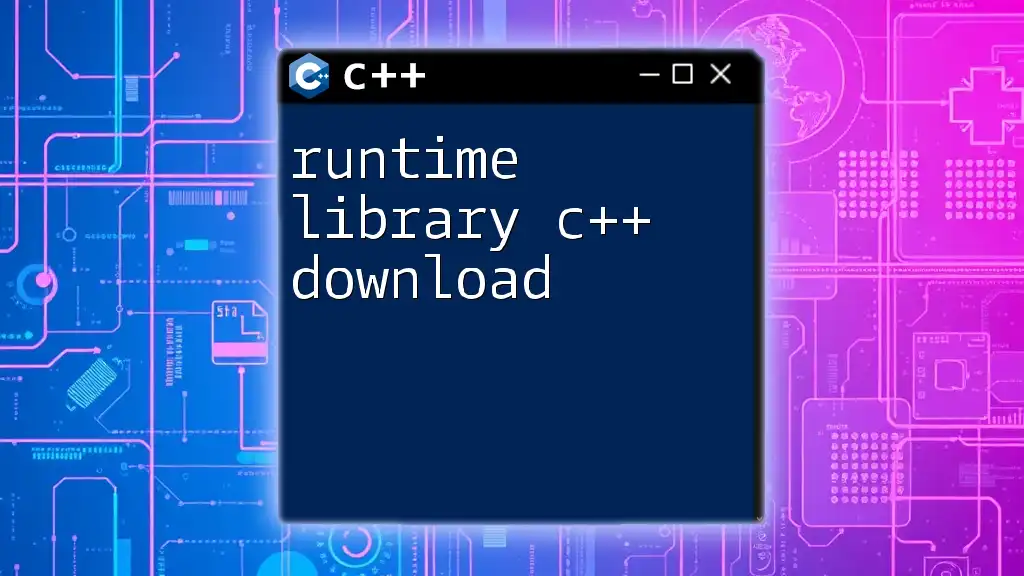
Conclusion
In summary, C++ libraries, whether standard or third-party, provide a crucial foundation for efficient and effective programming. By understanding and utilizing libraries, you can accelerate your projects, produce cleaner code, and unlock powerful functionalities. Embrace the vast world of C++ libraries, as it invites exploration and experimentation that will significantly enhance your development journey.