In C++, an array is a collection of items stored at contiguous memory locations, allowing you to efficiently manage a fixed-size sequence of elements of the same data type.
#include <iostream>
using namespace std;
int main() {
int numbers[5] = {10, 20, 30, 40, 50}; // declaring an array
for (int i = 0; i < 5; i++) {
cout << numbers[i] << " "; // accessing array elements
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of elements, all of the same type, stored in contiguous memory locations. This fundamental data structure allows developers to store and manage multiple values under a single variable name, making it easier to work with related data.
Arrays play a crucial role in programming as they allow efficient storage and retrieval of data. Instead of declaring individual variables for each item, arrays provide a way to manage multiple items under a single identifier.
Types of Arrays
Arrays come in various types, which can be categorized primarily as:
Single-dimensional arrays
These are the simplest form of arrays, which can be thought of as a list of items. For example, if you want to store ten integers, you can declare a single-dimensional array called `numbers`.
Multi-dimensional arrays
These arrays contain two or more dimensions (or indices). They are particularly useful for representing matrices or more complex data structures. For instance, a two-dimensional array can represent a table of data.
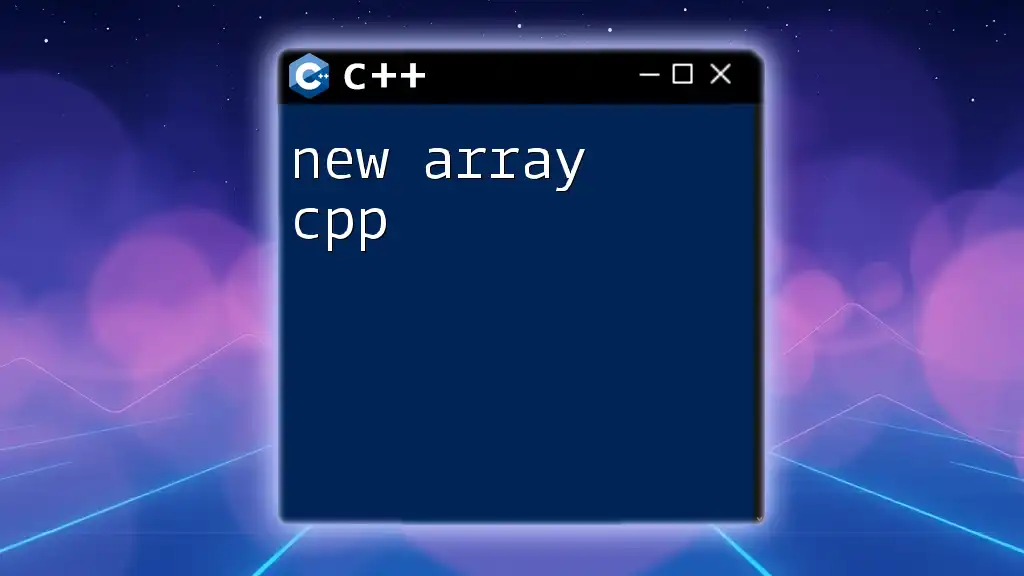
Declaring and Initializing Arrays
Declaring Arrays
The syntax for declaring arrays in C++ is straightforward. You specify the data type, followed by the array name and the number of elements it will hold.
For example:
int numbers[5]; // Declaration of a single-dimensional array
Initializing Arrays
Arrays can be initialized using different methods:
Static Initialization
This method initializes arrays at the time of declaration. For instance:
int numbers[5] = {1, 2, 3, 4, 5};
Dynamic Initialization
This is used when the size of the array is not known at compile time. You may allocate memory dynamically using pointers:
int size;
std::cout << "Enter the size of array: ";
std::cin >> size;
int* dynamicArray = new int[size]; // Dynamic memory allocation
Always remember to release dynamically allocated memory to avoid memory leaks.
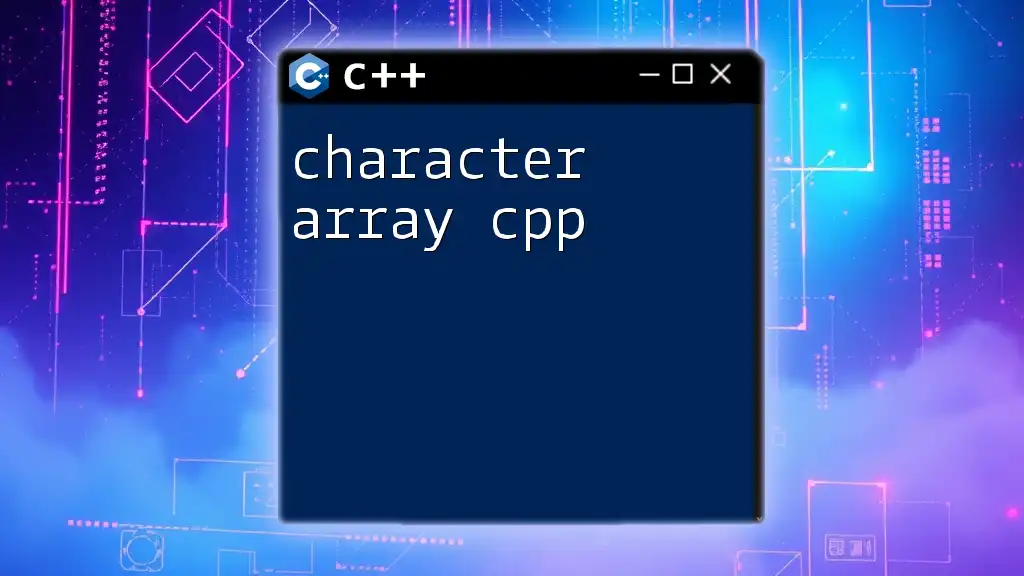
Accessing and Modifying Array Elements
Accessing Array Elements
You can access elements of an array using their index. In C++, indices start at zero, which means `numbers[0]` retrieves the first element of the array.
For example:
std::cout << numbers[0]; // Accessing the first element
Modifying Array Elements
Changing the value of array elements is also straightforward. To modify an element, assign a new value to its index:
numbers[0] = 10; // Modifying the first element
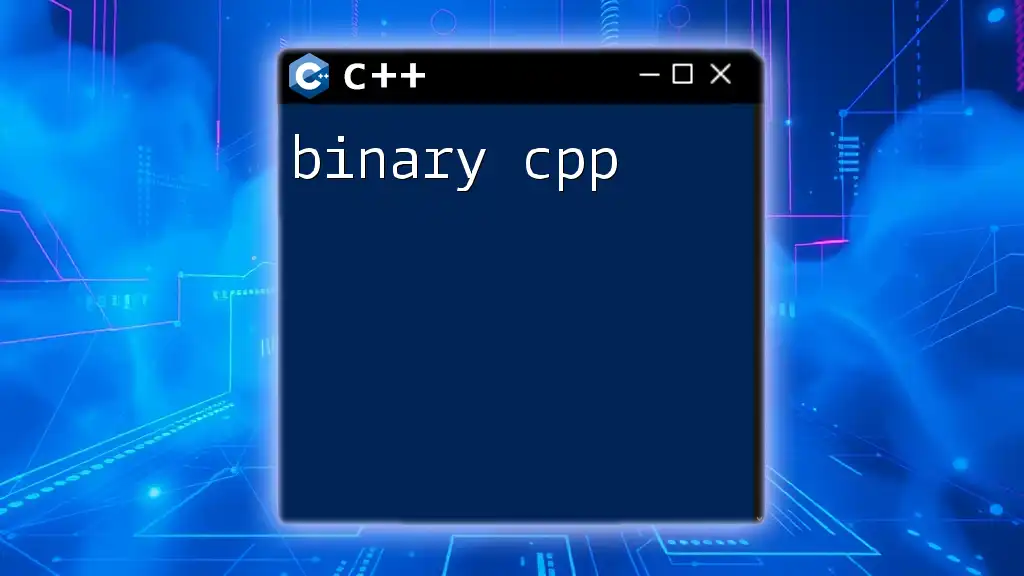
Array Operations
Traversing Arrays
To process each element in an array, you can traverse the array using loops. The `for` loop is often the simplest method:
for (int i = 0; i < 5; i++) {
std::cout << numbers[i] << " "; // Output each element
}
Finding the Length of an Array
For statically sized arrays, finding the length is easy using the `sizeof` operator. However, for dynamically allocated arrays, keep track of the size manually.
For example, to obtain the length of a static array:
int length = sizeof(numbers) / sizeof(numbers[0]); // Length of static array
Common Array Algorithms
Searching Algorithms
Linear Search is the simplest algorithm to find an element:
bool linearSearch(int arr[], int size, int key) {
for (int i = 0; i < size; i++) {
if (arr[i] == key) {
return true; // Key found
}
}
return false; // Key not found
}
Binary Search is more efficient but requires a sorted array:
int binarySearch(int arr[], int size, int key) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == key) {
return mid; // Key found
}
if (arr[mid] < key) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Key not found
}
Sorting Algorithms
Sorting algorithms like Bubble Sort help to arrange elements in a particular order:
void bubbleSort(int arr[], int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
std::swap(arr[j], arr[j + 1]); // Swap elements
}
}
}
}
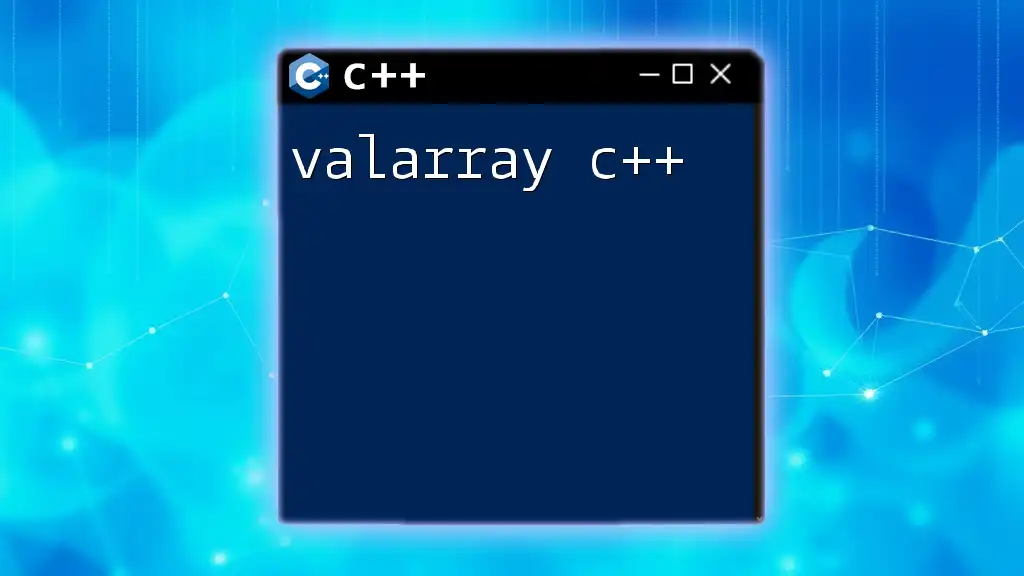
Two-Dimensional Arrays
Declaring and Initializing 2D Arrays
To declare a two-dimensional array, specify the size for each dimension:
int matrix[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
Accessing and Modifying 2D Array Elements
Accessing elements in a two-dimensional array requires two indices:
std::cout << matrix[1][2]; // Accessing element in the second row, third column
To modify an element, simply assign a new value as shown:
matrix[0][0] = 10; // Changing first element
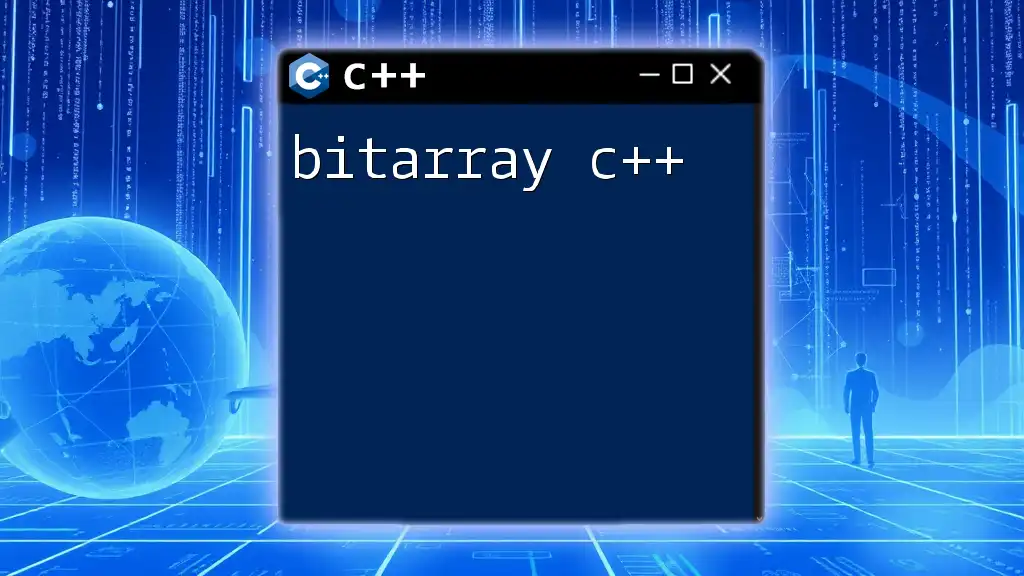
Memory Management with Arrays
Dynamic Arrays and Pointers
When using dynamic arrays, it is crucial to manage memory properly. Dynamic arrays are created using pointers and are allocated at runtime. Always remember to free the allocated memory once it is no longer needed to prevent memory leaks:
delete[] dynamicArray; // Freeing the dynamic array
Memory Leaks and Best Practices
Memory leaks occur when allocated memory is not deallocated, leading to wasted resources. To avoid this, always match every `new` with `delete` and ensure that pointer variables are not left dangling.
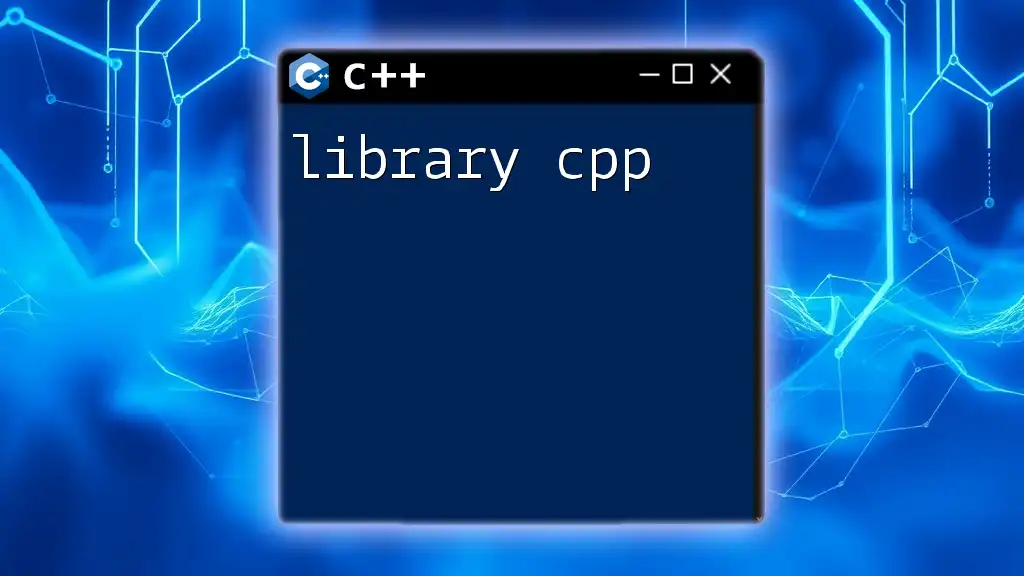
Conclusion
Recap of Key Concepts
In this article, we explored the essentials of arrays in C++. We covered their definition, various types, how to declare and initialize them, and the operations you can perform with arrays, such as accessing and modifying elements, common algorithms for searching and sorting, and memory management.
Further Learning Resources
For those looking to deepen their understanding of arrays and their applications in C++, consider exploring programming textbooks, online tutorials, and coding platforms that offer hands-on experience with array manipulations.
Call to Action
Encourage your peers and fellow developers to practice creating and manipulating arrays in their C++ projects. The more you practice, the more proficient you will become in leveraging this powerful data structure in your programming journey.