In C++, an integer array is a collection of variables of the same type stored in contiguous memory locations, which can be efficiently accessed using an index.
Here’s a simple example of how to declare and initialize an integer array in C++:
#include <iostream>
int main() {
int myArray[5] = {10, 20, 30, 40, 50};
for (int i = 0; i < 5; i++) {
std::cout << myArray[i] << " ";
}
return 0;
}
Understanding C++ Arrays
What is an Array?
An array is a collection of elements, all of the same type, stored in contiguous memory locations. In C++, arrays serve as a data structure that allows developers to organize and manage collections of related data efficiently. They can be accessed using indices, which provide a way to refer to individual elements.
Types of Arrays in C++
C++ supports various types of arrays, the primary ones being:
-
Single-Dimensional Arrays: These are linear arrays that store elements in a single row. For instance, an array of integers where each integer can be accessed using a single index.
-
Multi-Dimensional Arrays: These arrays are essentially arrays of arrays, allowing you to store data in a tabular format, such as rows and columns. For example, a two-dimensional array can represent a matrix.
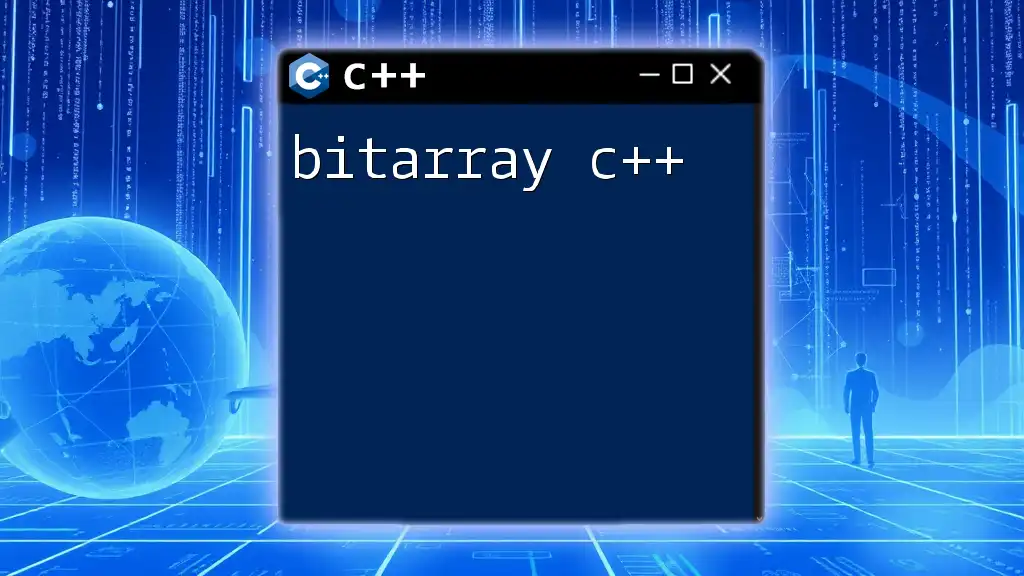
Creating and Initializing Int Arrays in C++
Syntax for Declaring an Int Array
To declare an integer array in C++, you use the following syntax:
int myArray[5];
In this example, `myArray` can hold 5 integer elements.
Initializing Int Arrays
Arrays can be initialized in various ways:
- At Declaration: You can initialize an array at the time of declaration:
int myArray[5] = {1, 2, 3, 4, 5};
- Using a Loop: If you prefer to fill the array elements using programming logic, you can use a loop:
for (int i = 0; i < 5; i++) {
myArray[i] = i + 1; // Fills myArray with values 1 through 5
}
Dynamic Array Initialization
For cases where the size of the array is not known at compile time, dynamic memory allocation is useful. You can create an integer array dynamically using the `new` keyword:
int* dynamicArray = new int[5];
This creates an array of integers that can be accessed like a regular array.
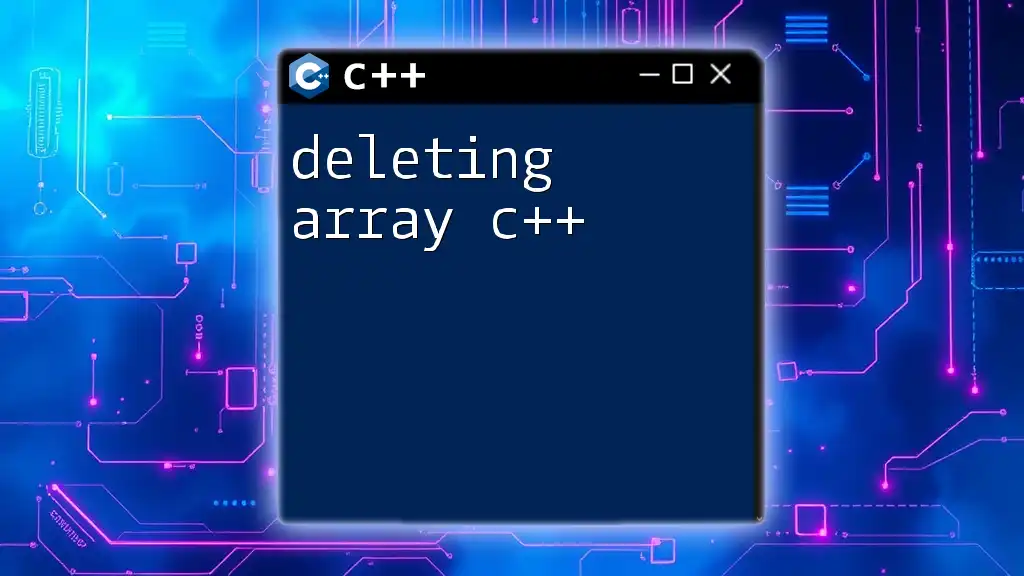
Accessing Elements in an Int Array
How to Access Elements
Each element in an int array can be accessed using an index, which starts at 0. For example:
int value = myArray[2]; // Accessing third element, which is '3' if initialized as above.
Modifying Elements
You can easily change an element in the array by assigning a new value to it:
myArray[2] = 10; // Sets the third element to 10.
This flexibility allows for dynamic data manipulation within your program.
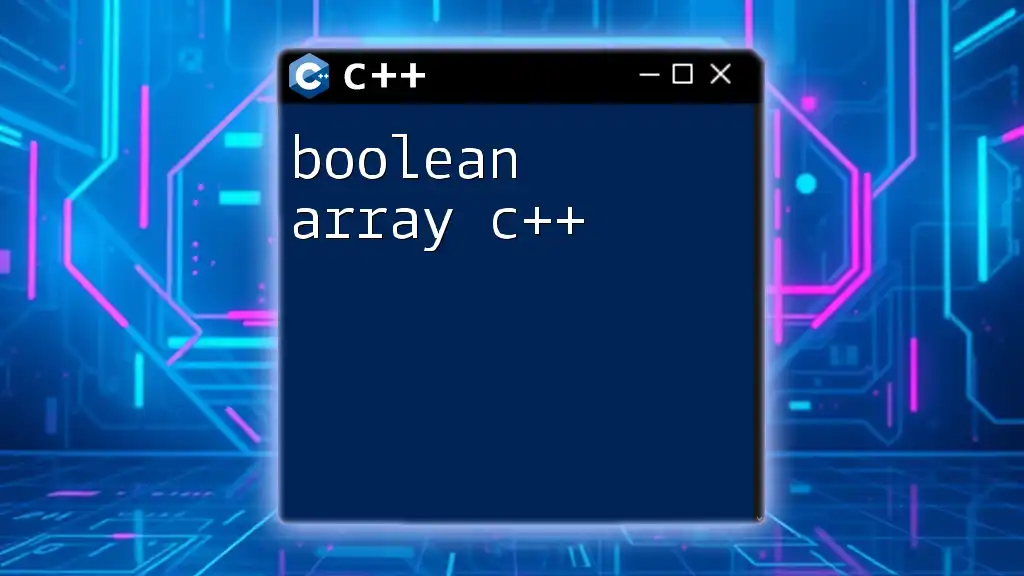
Common Operations on Int Arrays
Looping Through an Int Array
To perform operations like displaying all array elements or summing them up, you often need to loop through the array. A `for` loop is the most common way to achieve this:
for(int i = 0; i < 5; i++) {
std::cout << myArray[i] << " "; // Outputs all elements in the array.
}
This statement iterates through the `myArray` and outputs each element.
Finding the Size of an Int Array
To find the number of elements in a statically declared array, you can use the `sizeof` operator:
int size = sizeof(myArray) / sizeof(myArray[0]);
However, it’s crucial to remember that `sizeof` only works for arrays that are declared with a fixed size. Be cautious when using `sizeof` with pointers or dynamically allocated arrays, as it will not yield the correct size.
Sorting Int Arrays
Sorting is a common operation you might want to perform on an array. One simple sorting algorithm is Bubble Sort. Here’s a basic implementation:
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n-1; i++)
for (int j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
This example demonstrates how to sort an integer array in ascending order using the Bubble Sort algorithm.
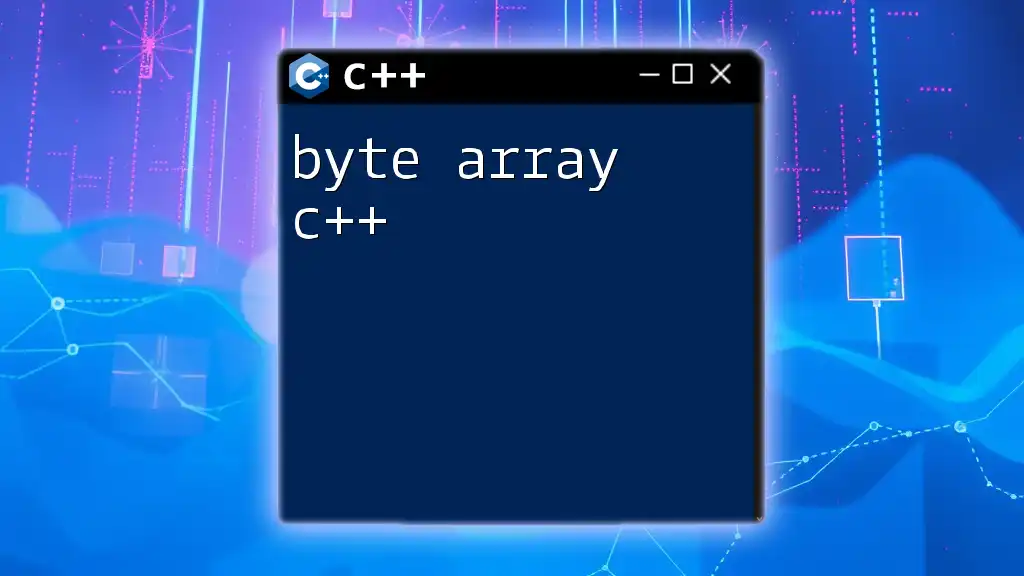
Key Error Handling with Int Arrays
Out of Range Errors
One of the most common pitfalls when working with arrays is attempting to access an index that is out of bounds. In C++, accessing such an index leads to undefined behavior. Hence, always ensure that your index values fall within the range `[0, size-1]`.
Memory Management in Dynamic Arrays
When using dynamic arrays, it's essential to manage memory effectively. Neglecting to free memory can lead to memory leaks. To delete a dynamically allocated integer array, you use:
delete[] dynamicArray;
Employing this practice will maintain your application’s memory efficiency.
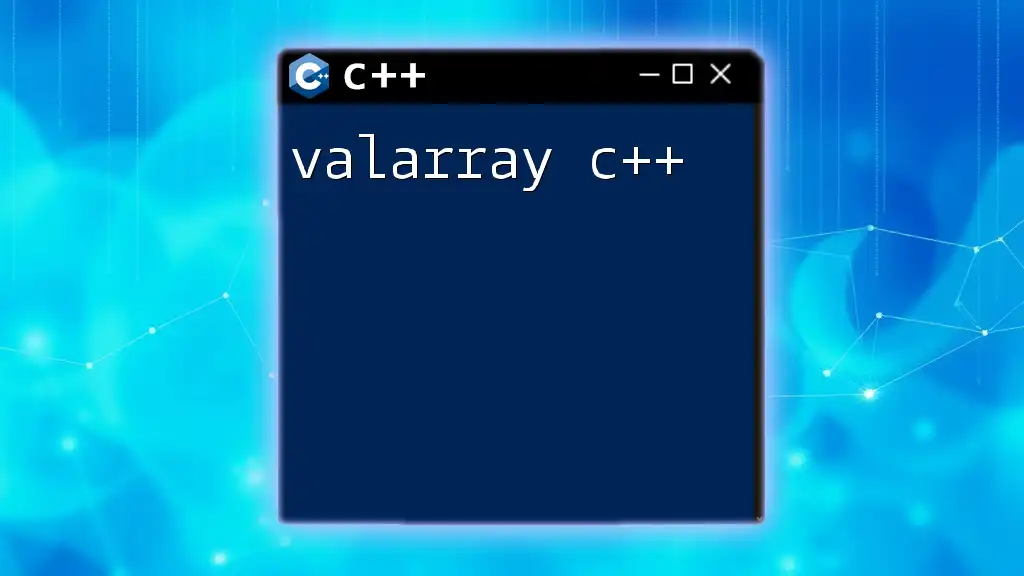
Conclusion
Int arrays in C++ are foundational structures that enable efficient data storage and manipulation. This guide has walked you through their creation, initialization, and common operations, equipping you with knowledge for your programming journey.
Remember, continuous practice and exploration of resources will help you to gain proficiency in managing integer arrays and enhance your overall C++ skills. Happy coding!
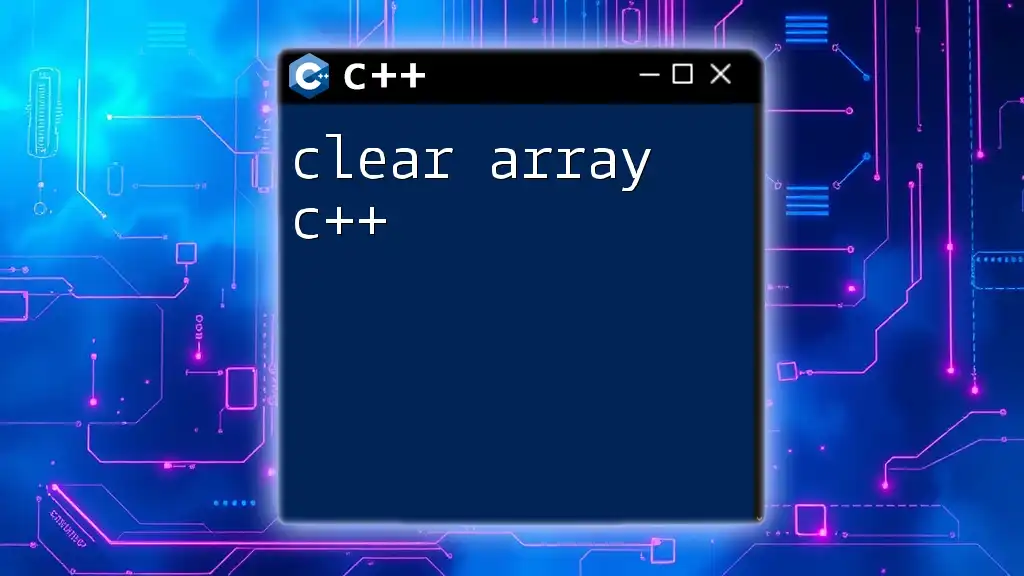
Additional Resources
For further learning about int arrays in C++, explore the C++ official documentation and online coding platforms that offer interactive coding exercises. These resources will solidify your understanding and offer practical experiences.
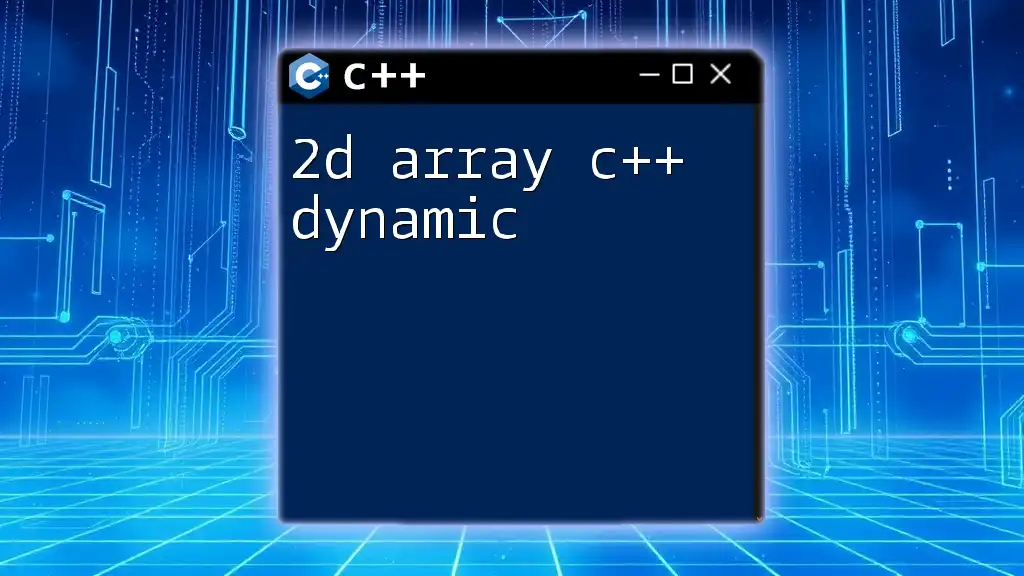
Call to Action
Stay tuned for more engaging tutorials designed to boost your knowledge and expertise in C++. Subscribe now for the latest tips and techniques!