In C++, a 2D array can be declared by specifying the data type, followed by the array name and its dimensions in square brackets.
Here’s a code snippet demonstrating the declaration of a 2D array:
int myArray[3][4]; // Declares a 2D array with 3 rows and 4 columns
Understanding 2D Arrays
What is a 2D Array?
A 2D array is essentially an array of arrays, providing a more complex structure that allows data storage in a tabular format—think of it as a grid or matrix. Unlike 1D arrays, which store data in a single line, 2D arrays can store data in rows and columns, facilitating various applications such as matrix operations, gaming grids, and image representation.
Syntax of Declaring a 2D Array in C++
To declare a 2D array in C++, the syntax is straightforward. The general format is:
dataType arrayName[rows][columns];
Here, `dataType` could be any valid C++ data type (like `int`, `float`, etc.), while `rows` and `columns` specify the dimensions of the array.
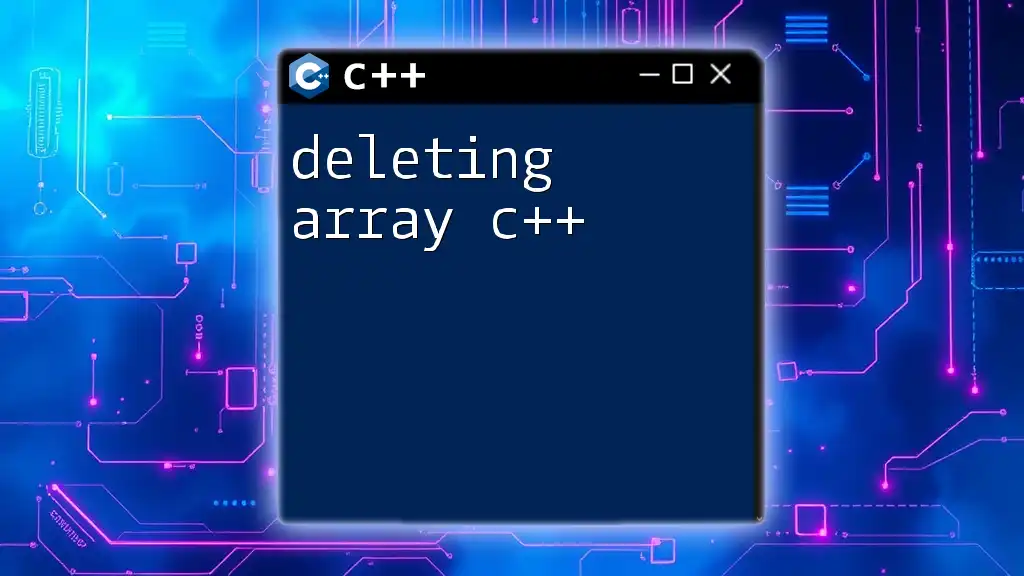
Declaring 2D Arrays in C++
Declaring a Fixed Size 2D Array
A fixed size declaration defines the dimensions of the array at compile time, which means the size cannot change during program execution. Here is an example:
int numbers[3][4]; // Declaration of a fixed 3x4 array
In this instance, an array named `numbers` is created, capable of storing 3 rows and 4 columns. Each element can be accessed using two indices—the first for the row and the second for the column.
Declaring a Dynamic Size 2D Array
Dynamic arrays enable flexibility, allowing your program to allocate memory at runtime. This can be achieved using pointers. The example below illustrates how to declare and allocate a dynamic 2D array:
int** dynamicArray;
dynamicArray = new int*[rows]; // Creating Rows
for (int i = 0; i < rows; i++) {
dynamicArray[i] = new int[columns]; // Creating Columns
}
In this snippet, we first allocate memory for the array of pointers (which point to the rows) and then allocate memory for each row, thus creating the full 2D array. It is crucial to manage memory properly by deallocating it when the array is no longer needed:
for (int i = 0; i < rows; i++) {
delete[] dynamicArray[i]; // Deallocate each row
}
delete[] dynamicArray; // Deallocate the main array
This process prevents memory leaks and ensures efficient memory management.
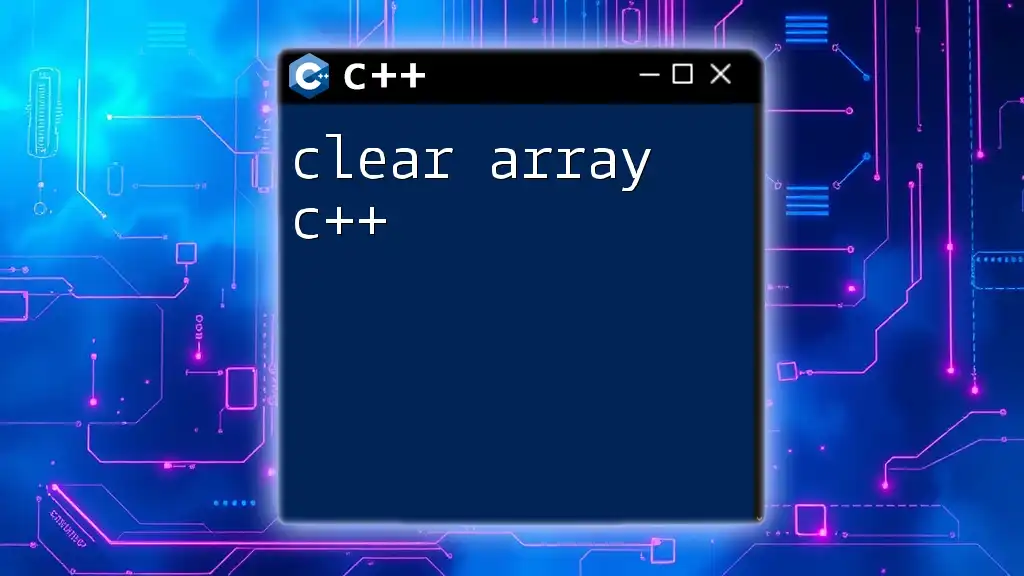
Initializing 2D Arrays
Initializing with Specific Values
You can initialize a 2D array during its declaration. Here's an example:
int primeNumbers[2][3] = { {2, 3, 5}, {7, 11, 13} };
In this case, a 2x3 array is initialized with predefined values. Each row contains three integer values.
Partial Initialization
C++ allows for partial initialization, meaning that if you don't provide values for all elements, the remaining ones will be initialized to zero:
int scores[2][3] = { {78, 85} }; // The rest will be initialized to 0
In this array, the first row contains the values 78 and 85, while all other elements are set to zero by default.
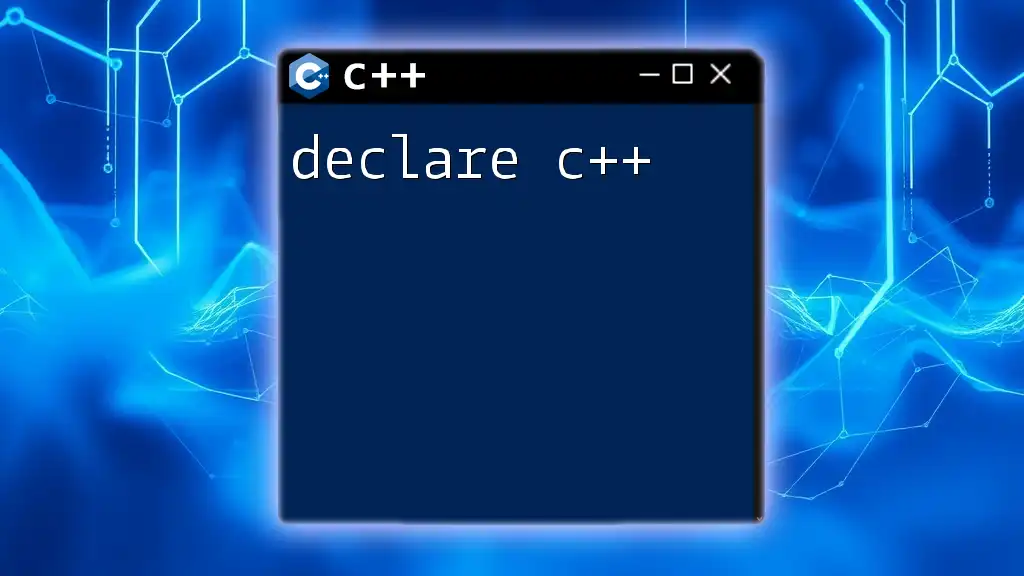
Accessing Elements in a 2D Array
Syntax for Accessing Elements
The syntax for accessing an element within a 2D array utilizes two indices. For instance:
cout << primeNumbers[1][2]; // Output: 13
Here, `primeNumbers[1][2]` accesses the element located in the second row and third column, which returns the value `13`.
Iterating Through a 2D Array
Iterating through the elements of a 2D array can be done using nested loops. Example code for such iteration is shown below:
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
cout << primeNumbers[i][j] << " ";
}
}
This code snippet prints all the values of the `primeNumbers` array, delving into each row and column through the nested structure. Note that the outer loop controls the rows, while the inner loop controls the columns.
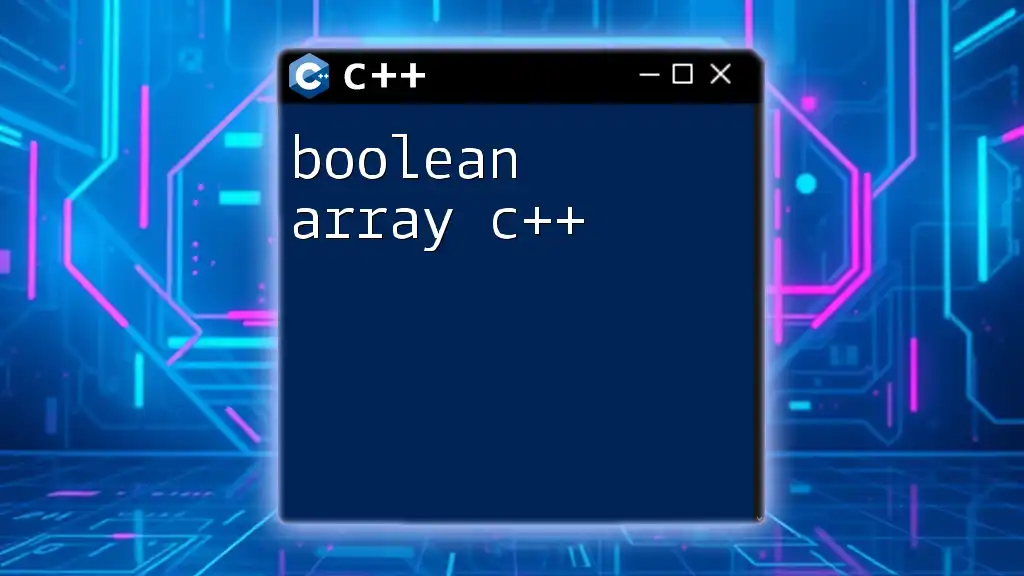
Common Mistakes When Working with 2D Arrays
Off-By-One Errors
One of the prevalent issues when dealing with arrays is the off-by-one error. Since C++ uses zero-based indexing, failing to account for this can lead to accessing out-of-bounds indices, causing runtime errors or unexpected behavior.
Memory Leaks with Dynamic Arrays
Dynamic arrays require careful memory management since failure to deallocate memory correctly can lead to memory leaks. Always ensure that every `new` has a corresponding `delete`. For example:
delete[] dynamicArray[i]; // Deallocate each row
delete[] dynamicArray; // Deallocate the main array
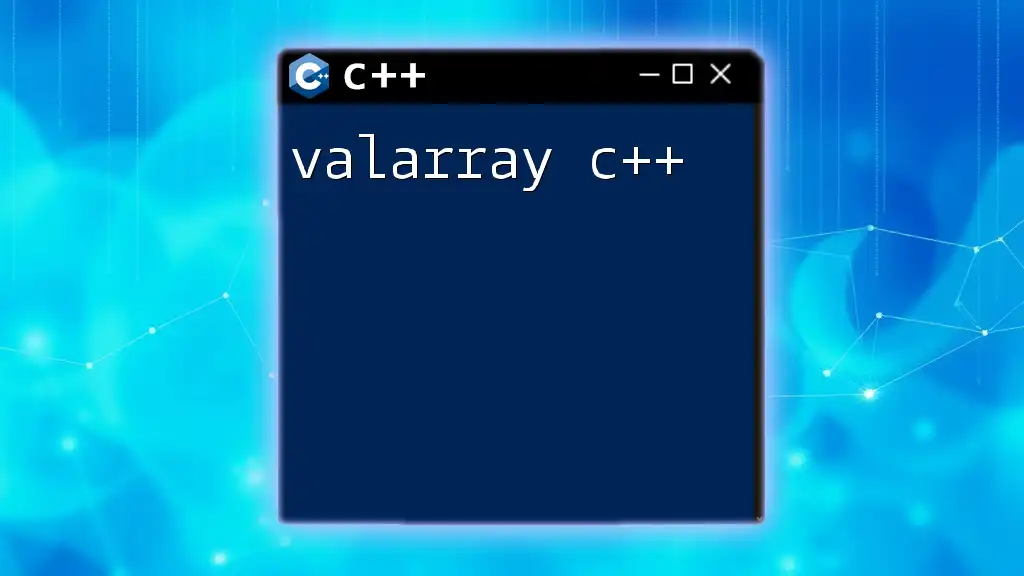
Best Practices for Using 2D Arrays in C++
To work efficiently with 2D arrays, adhere to the following best practices:
-
Use constants for size definitions: Instead of hardcoding numbers, define constants for the number of rows and columns to make your code more maintainable.
-
Consider std::vector for flexibility: When appropriate, utilize C++’s `std::vector`, as it provides dynamic resizing and simplifies memory management. Unlike raw arrays, vectors automatically handle memory allocation and deallocation.
-
Avoid hardcoding: Instead of statically defining sizes, consider using variables or configurations to make your program adaptable and easier to modify.
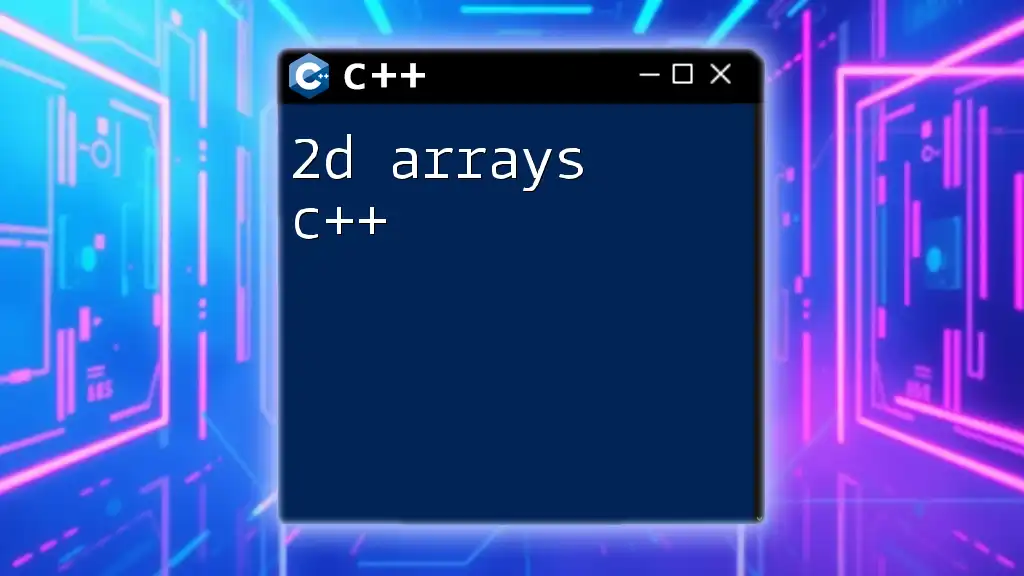
Conclusion
Understanding how to declare and manipulate 2D arrays in C++ is essential for efficient programming in a variety of applications, from simple grids to intricate algorithms. By practicing these concepts and utilizing the examples provided, you will become adept at working with 2D arrays and enhance your proficiency in C++. Make sure to explore the nuances discussed and apply them in your coding projects to solidify your learning.
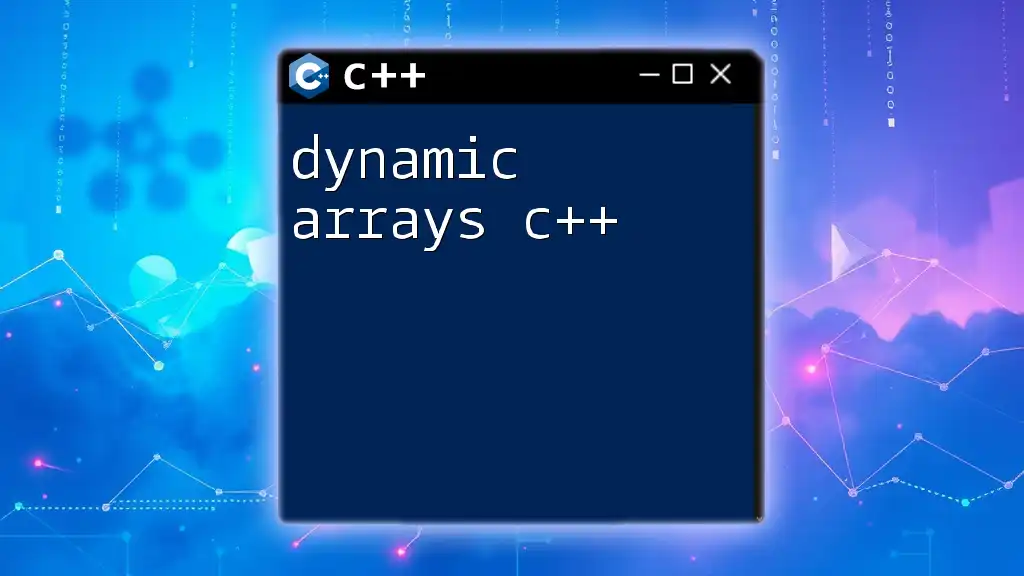
Further Reading and Resources
For more information on this topic, refer to the official C++ documentation, explore textbooks on C++ programming, or find online tutorials that can offer exercises and further examples. Happy coding!