An ordered map in C++ is a container that stores key-value pairs sorted by keys, allowing for efficient retrieval and maintaining the order of insertion.
Here's a simple code snippet demonstrating how to use an `std::map` (which is inherently ordered) in C++:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> orderedMap;
orderedMap[3] = "Three";
orderedMap[1] = "One";
orderedMap[2] = "Two";
for (const auto& pair : orderedMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
What is an Ordered Map?
An ordered map in C++ is a collection of key-value pairs that automatically maintains the order of the keys. Unlike standard maps that may not retain any order, an ordered map ensures that keys are sorted according to specified criteria. This characteristic makes ordered maps useful for applications where data retrieval in a predictable sequence is essential.
When comparing ordered maps to regular maps (like `std::unordered_map`), it’s crucial to note that ordered maps provide log(n) search, insertion, and deletion time complexities, thanks to their underlying balanced tree structure. This is an important advantage when data order is paramount.

C++ Standard Library and Ordered Map
In C++, ordered maps are managed using the `<map>` header from the Standard Template Library (STL). There are two primary types of ordered maps available:
-
std::map: This type stores unique keys. If an attempt is made to insert a duplicate key, the existing value is overwritten.
-
std::multimap: This variation allows for multiple entries with the same key, maintaining order.
Characteristics of Ordered Maps
Key Features
-
Automatic Sorting of Keys: The keys in an ordered map are arranged according to their specific order, which is typically ascending.
-
Unique vs. Non-Unique Keys: With `std::map`, each key must be unique, while `std::multimap` allows duplicates.
-
Balanced Tree Structure: The data is organized in a balanced tree structure, commonly a Red-Black tree, which ensures efficient data access.
Time Complexity
When discussing time complexity, it is essential to understand that the fundamental operations in ordered maps—insertions, deletions, and lookups—usually occur in O(log n) time. This makes ordered maps suitable for scenarios with a significant number of operations.
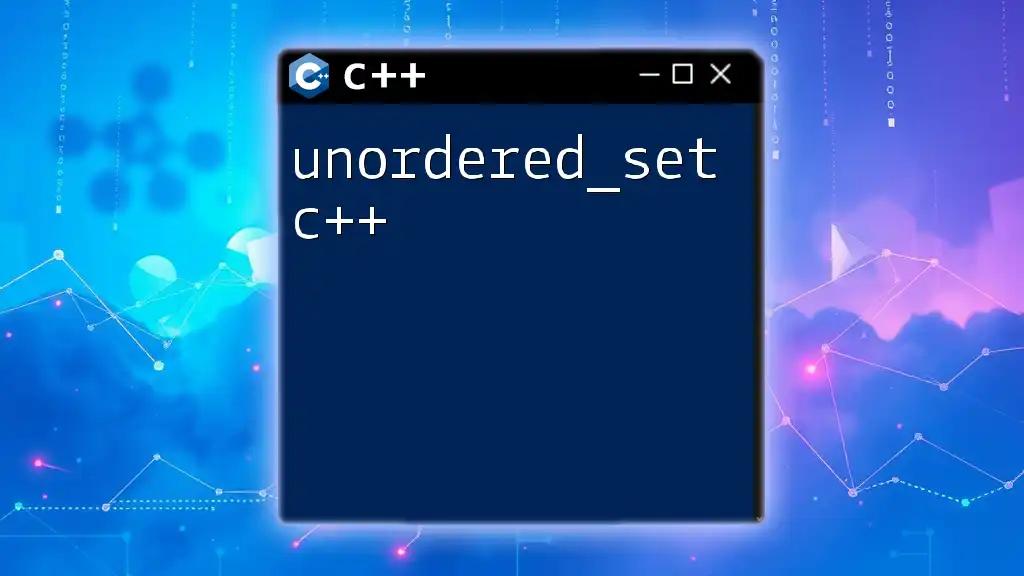
Creating an Ordered Map
To start using ordered maps in your C++ projects, you need to include the appropriate header and use a `std::map` or `std::multimap`.
Setting Up Your Environment
Make sure to include the necessary header file:
#include <iostream>
#include <map>
Basic Syntax of std::map
To instantiate an ordered map, you can use the following syntax, which declares a map that associates integers with strings:
int main() {
std::map<int, std::string> orderedMap;
return 0;
}

Common Operations on Ordered Maps
Insertion of Elements
Inserting entries into an ordered map can be done in two ways. You can either use array indexing or the `insert` method:
orderedMap[1] = "One"; // Using array indexing
orderedMap.insert({2, "Two"}); // Using insert method
Both methods will ensure that the map is updated properly while maintaining the order of the keys.
Accessing Elements
Retrieving values by their keys is straightforward with an ordered map. You can access an element using the key directly:
std::cout << orderedMap[1]; // Output: One
If the key does not exist, this will insert a new entry with the default value, so care should be taken when using this method.
Iterating Through an Ordered Map
Iterating through an ordered map allows you to access key-value pairs in sorted order. You can use iterators to loop over the map:
for(auto it = orderedMap.begin(); it != orderedMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
This will output the entire contents of the map in the order of the keys, enhancing readability and usability.
Removing Elements
Deleting entries from an ordered map is as simple as calling the `erase` method with the key you want to remove:
orderedMap.erase(1); // Removes the entry with key 1
This operation maintains the order of the remaining elements, showcasing the dynamic nature of ordered maps.

Advantages of Using Ordered Maps
The main advantage of using ordered maps lies in their ability to maintain a predictable order of keys. This characteristic is particularly helpful when you require sorted data for various algorithms or for direct access in display operations.
Moreover, ordered maps automatically sort their entries as new elements are added. This auto-sorting minimizes the need for additional sorting operations and simplifies data management.
The O(log n) time complexity for common operations—insertions, deletions, and lookups—makes ordered maps efficient for scenarios that involve frequent data manipulation.
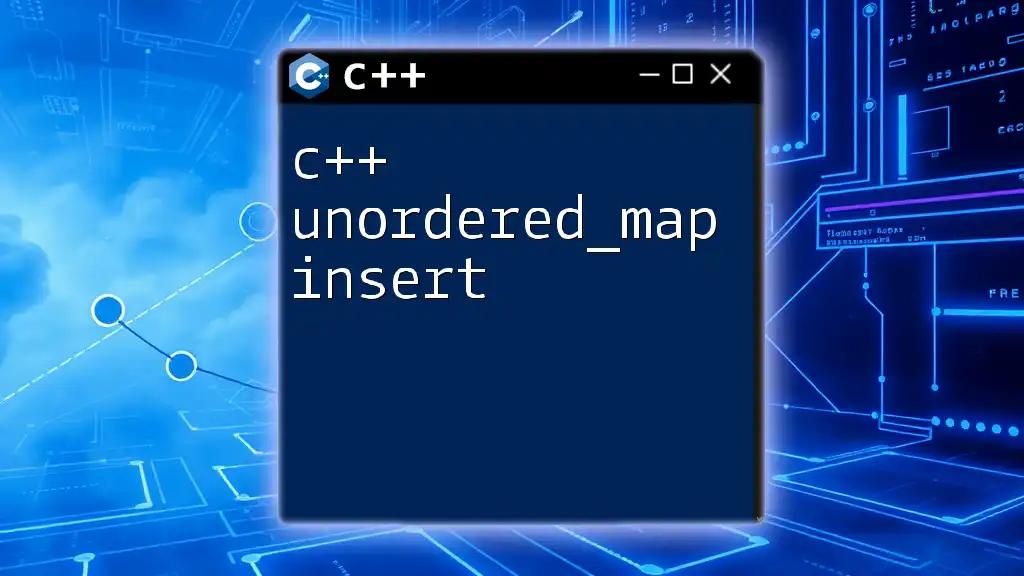
Potential Downsides of Ordered Maps
Despite their advantages, ordered maps come with some downsides. One of the primary considerations is performance. The overhead related to maintaining order can result in slower performance compared to unordered maps for certain operations.
Another factor is memory usage. Because ordered maps must maintain a complex structure to sort and balance the elements, they generally have a higher memory footprint than simpler data structures.
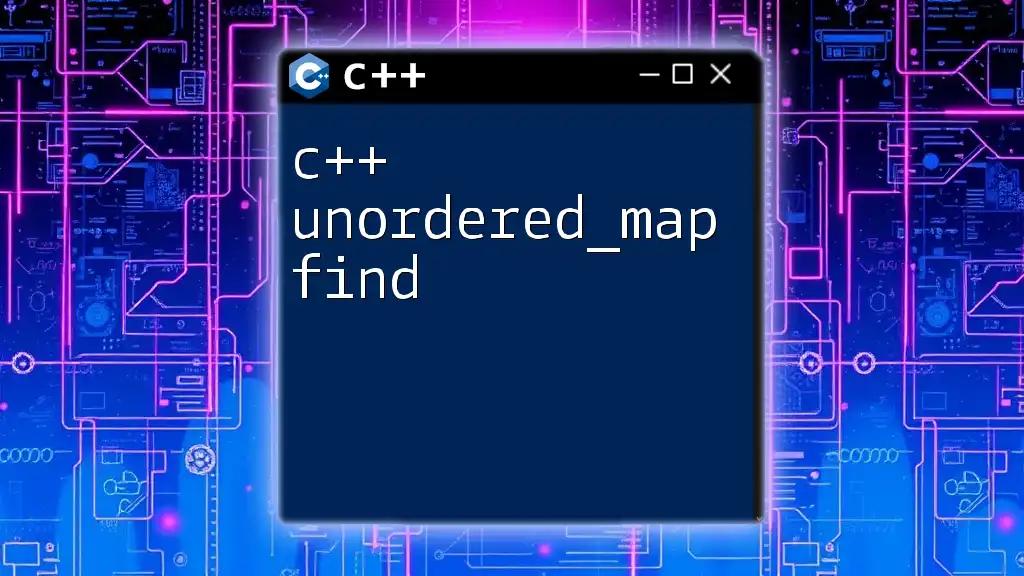
Use Cases for Ordered Maps
Ordered maps find extensive use in various applications. They can be particularly useful in scenarios where you need frequency counting, such as counting the occurrences of words in a document. Because of their inherent order, they simplify operations like reporting statistics in sorted order.
They can also be beneficial in caching mechanisms where maintaining order based on access times is essential for optimizing performance.
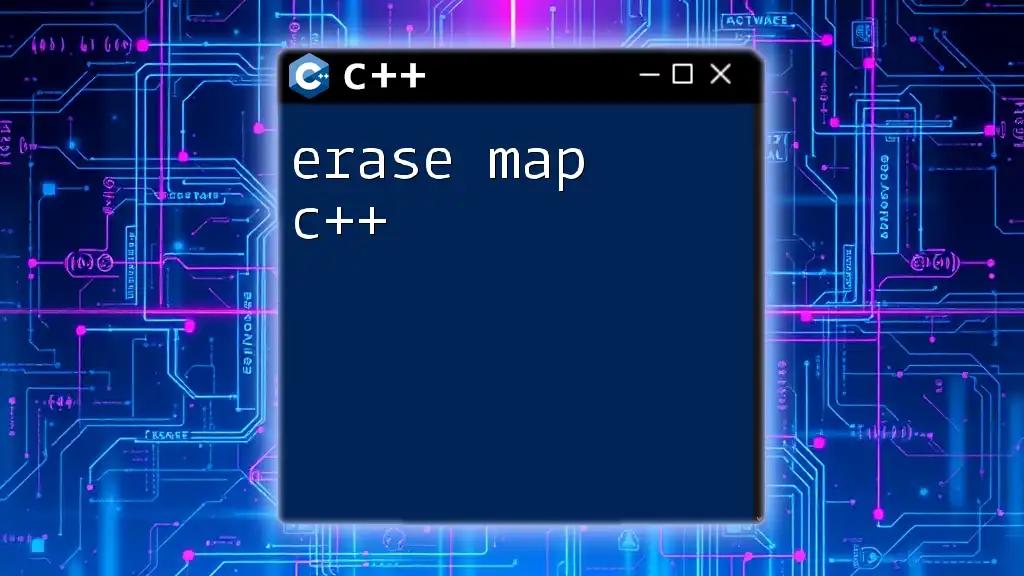
Advanced Features
Using Comparators with Ordered Maps
C++ allows for custom ordering of keys in an ordered map using comparators. By defining a comparator structure, you can change the default sorting behavior:
struct customCompare {
bool operator()(const int &a, const int &b) const {
return a > b; // Descending order
}
};
std::map<int, std::string, customCompare> customOrderedMap;
This example demonstrates how to create a map that sorts keys in descending order, offering flexibility based on your requirements.
Multimaps: Allowing Duplicate Keys
Consider using `std::multimap` if your application requires duplicate keys. This variant retains all entries for a given key, maintaining their sorted order:
std::multimap<int, std::string> multiMap;
multiMap.insert({1, "One"});
multiMap.insert({1, "Uno"}); // Allowing duplicates
Using multimaps can prove beneficial when you need to store multiple values for a single key without losing information.
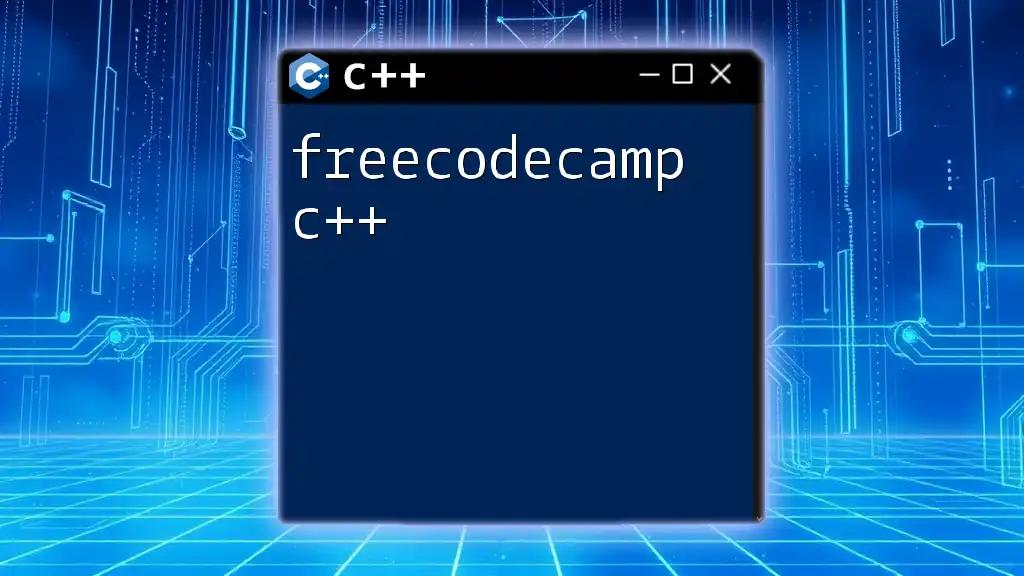
Conclusion
In summary, ordered maps in C++ offer a robust mechanism for maintaining sorted key-value pairs with efficient performance characteristics. From basic operations like insertion and deletion to more complex use cases involving custom comparators and multimaps, they provide versatile solutions for a wide range of programming challenges. Understanding how to effectively employ ordered maps will undoubtedly enhance your ability to optimize your C++ applications.