An unordered map in C++ is a container that stores key-value pairs in no particular order, allowing for fast retrieval based on keys using a hash table.
Here’s a simple code snippet demonstrating how to declare and use an unordered map:
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
// Accessing values
std::cout << "Alice's age: " << ageMap["Alice"] << std::endl;
std::cout << "Bob's age: " << ageMap["Bob"] << std::endl;
return 0;
}
What is an Unordered Map in C++?
A C++ unordered map is a part of the C++ Standard Template Library (STL) that provides an associative container. This data structure allows you to store elements in the form of key-value pairs, where each key is unique. While an ordered map maintains a specific order based on the keys, an unordered map uses a hash table for storage, which allows for faster access times for key lookups, insertions, and deletions on average.
Differences between `unordered_map` and `ordered_map`
-
Storage Mechanism: `unordered_map` employs a hash table, which means that the order of elements is not guaranteed. On the other hand, `ordered_map` maintains a sorted order based on the keys, using a balanced binary search tree structure.
-
Performance: Due to its underlying structure, `unordered_map` typically provides average constant time complexity \(O(1)\) for insertions and lookups, whereas `ordered_map` operations have logarithmic time complexity \(O(\log n)\).
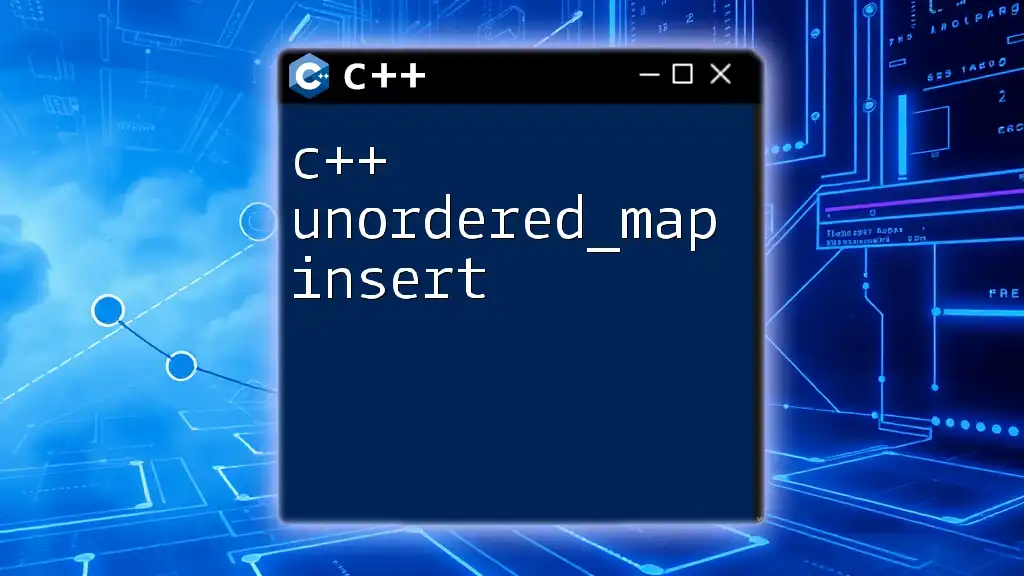
Importance of Unordered Maps in C++
The unordered map in C++ is crucial for scenarios that require frequent access to key-value pairs without a need for ordering. It excels in performance-sensitive applications where immediate data retrieval and insertion are necessary. For example, when counting frequencies of elements (like words in a text), using an unordered map allows for constant time access, making it preferable over ordered structures for this purpose.
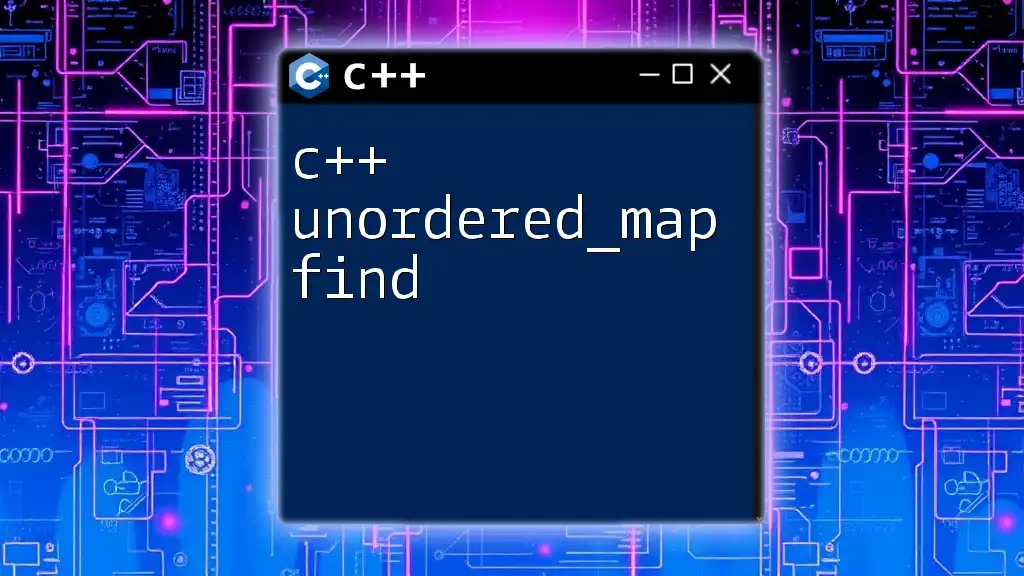
Understanding the C++ `std::unordered_map`
Syntax of `std::unordered_map`
To begin using `unordered_map`, you need to include the appropriate header:
#include <unordered_map>
You can then declare an `unordered_map` as follows:
std::unordered_map<int, std::string> myMap;
In this example, the keys are integers, and the values are strings.
Key Features of `std::unordered_map`
-
Key-Value Pair Storage: An unordered map stores data as key-value pairs, allowing quick access to the value using its corresponding key.
-
Unique Keys: Keys must be unique within the map, meaning that if you attempt to insert a duplicate key, the previous value associated with that key will be overwritten.
-
Hash Function: The performance of an unordered map largely depends on the hash function used. It transforms the key into a size index, allowing fast access based on this hash value.
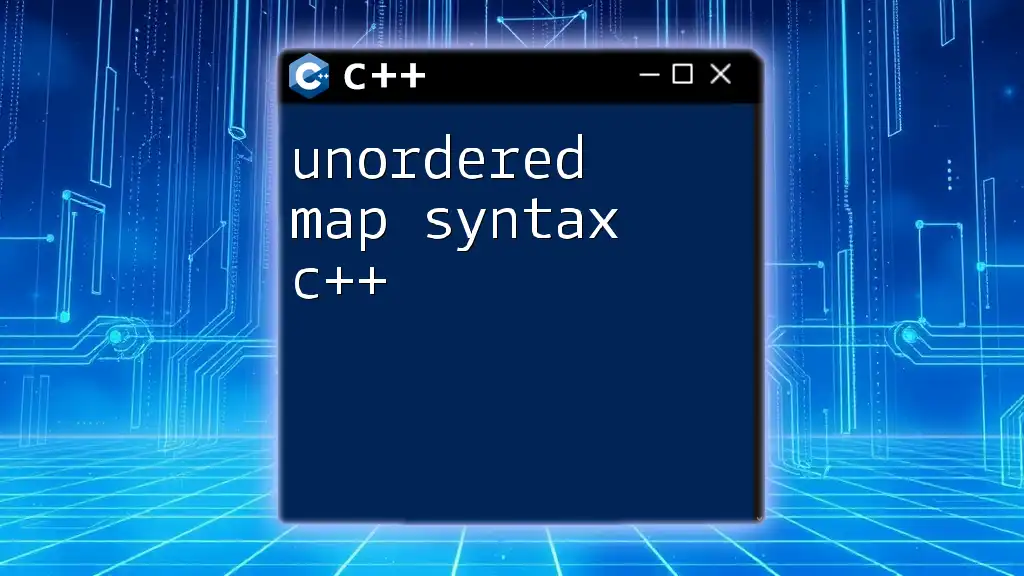
Basic Operations with Unordered Map in C++
Inserting Elements
Inserting elements into an unordered map can be done using either the `emplace()` or `insert()` methods:
myMap.insert({1, "Apple"});
myMap.emplace(2, "Banana");
The `emplace()` method is preferable in scenarios where you want to construct the element in place without making a copy, providing optimal performance.
Accessing Elements
Accessing elements in an unordered map can be done through the key:
std::cout << myMap[1]; // Output: Apple
This retrieves the value associated with the key. If a key does not exist, it will create a new entry with the default value.
Finding Elements
To check for the existence of a key, the `find()` method can be used:
auto it = myMap.find(2);
if (it != myMap.end()) {
std::cout << it->second; // Output: Banana
}
Here, the iterator `it` will point to the element if found; otherwise, it will point to `myMap.end()`.
Deleting Elements
Removing elements is straightforward with the `erase()` method:
myMap.erase(1);
This command removes the element with key `1` from the unordered map.
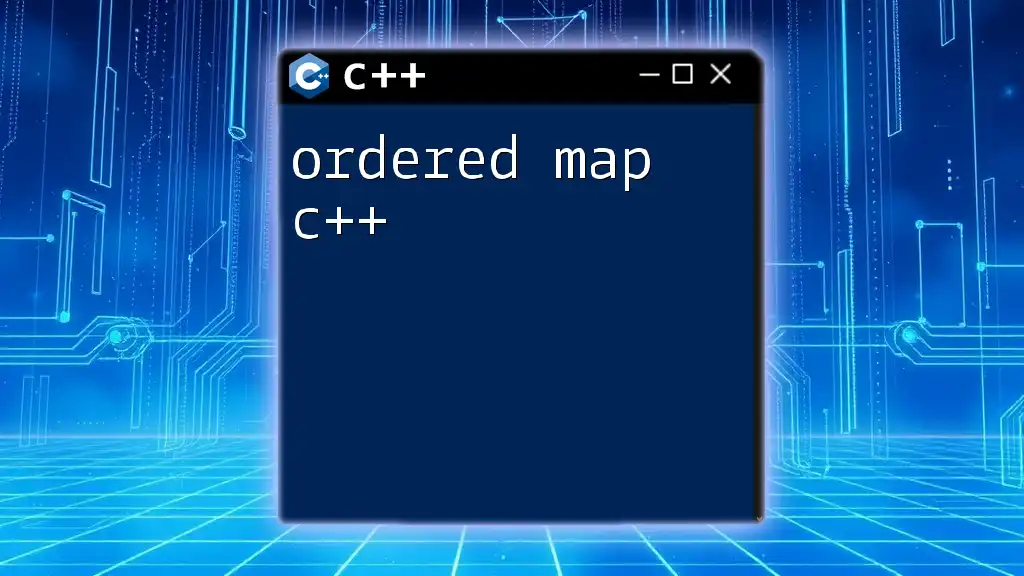
Advanced Features of `std::unordered_map`
Custom Hash Functions
You may occasionally need to customize the way keys are hashed, especially when they are complex types. You can define your own hash function like this:
struct CustomHash {
size_t operator()(const std::string& key) const {
return std::hash<std::string>()(key);
}
};
std::unordered_map<std::string, int, CustomHash> customMap;
This custom hash function allows you to maintain control over how keys are processed, improving performance based on your specific needs.
Iterating Over `unordered_map`
You can iterate over the elements in an unordered map using a range-based for loop or iterators:
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This displays each key-value pair in the map, providing an easy way to access all data stored within.
Performance Considerations
Understanding the performance implications of your unordered map is crucial. Generally, you can expect average-case complexities of \(O(1)\) for both access and insertion. However, under certain conditions (like poor hash function distribution), performance can degrade to \(O(n)\), emphasizing the importance of a well-designed hash function.
Factors such as load factor—a measure of the number of elements in relation to the number of buckets—affect performance significantly. Keeping the load factor low through appropriate resizing and rehashing strategies can enhance performance.
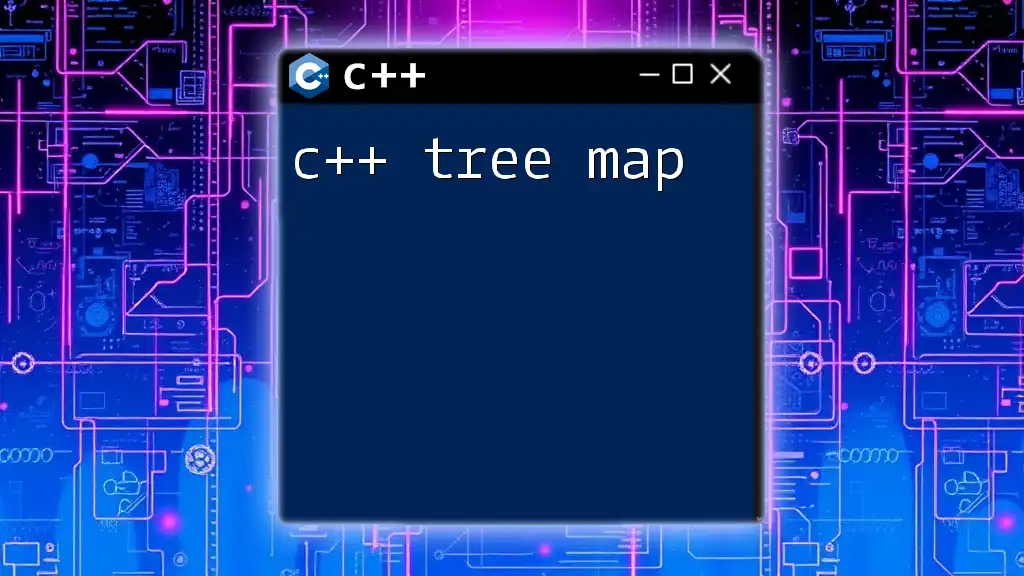
Comparing C++ Unordered Map with Other STL Containers
`unordered_map` vs. `map`
Here’s a quick comparison highlighting the key differences:
- Order: `unordered_map` does not maintain order, whereas `map` keeps elements in sorted order based on keys.
- Time Complexity: Average complexities for `unordered_map` operations are near constant, \(O(1)\), while those for `map` operations are \(O(\log n)\).
- Memory Overhead: Due to its hash table design, `unordered_map` can have more memory overhead compared to `map`.
Use Cases for `unordered_map` in C++
Unordered maps are particularly useful in scenarios such as:
- Counting Frequencies: Quickly tracking the frequency of items (e.g., words in a document).
- Caching: Ideal for implementing caches, where you store items that are frequently accessed, ensuring rapid retrieval.
- Fast Lookup Requirements: In applications that require efficient data retrieval by unique keys, unordered maps provide a quick solution.
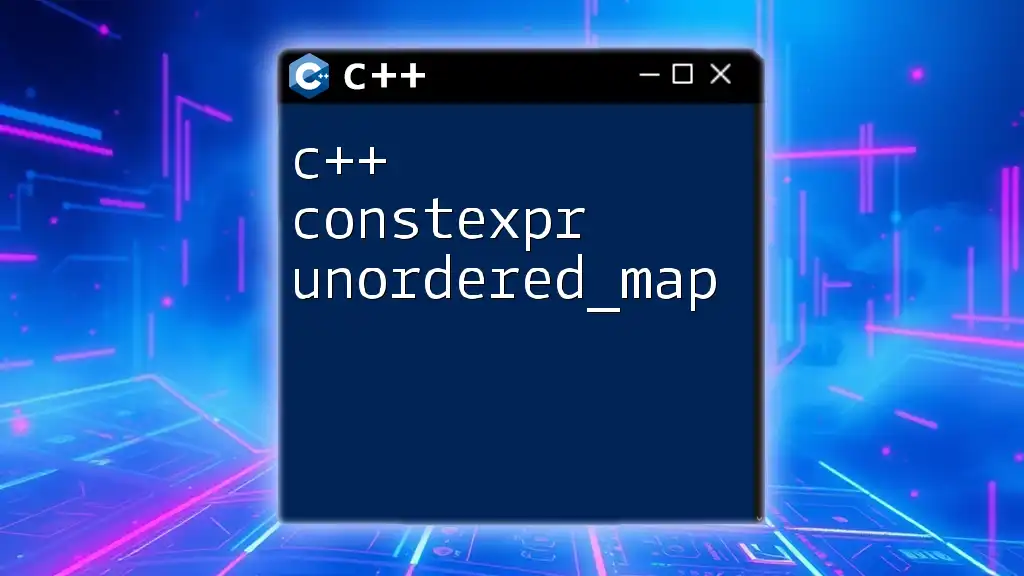
Conclusion
In summary, the C++ unordered map is an essential container that facilitates efficient key-value pair management through its hash-based structure. By providing average constant time complexities for essential operations, `unordered_map` serves as an invaluable tool in developing high-performance applications. Understanding how to leverage its features—like custom hash functions, intelligent insertion methods, and iteration—enables developers to harness the full potential of this container.
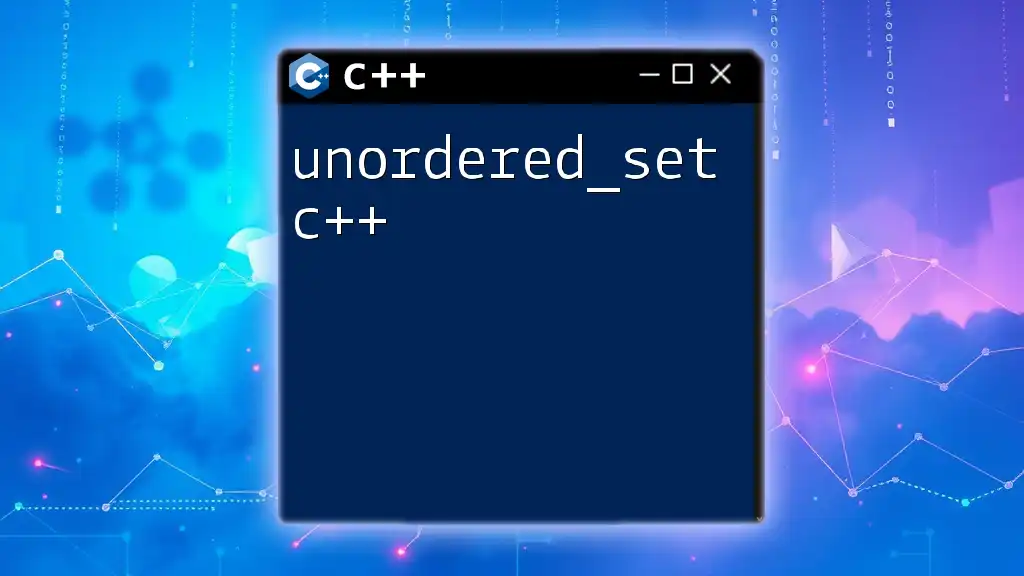
Further Reading and Resources
For more extensive information, consider reviewing the official C++ documentation for further insights into usage patterns, efficiency tips, and advanced techniques. Resources with practical code examples can also assist in mastering the nuances of `unordered_map`.
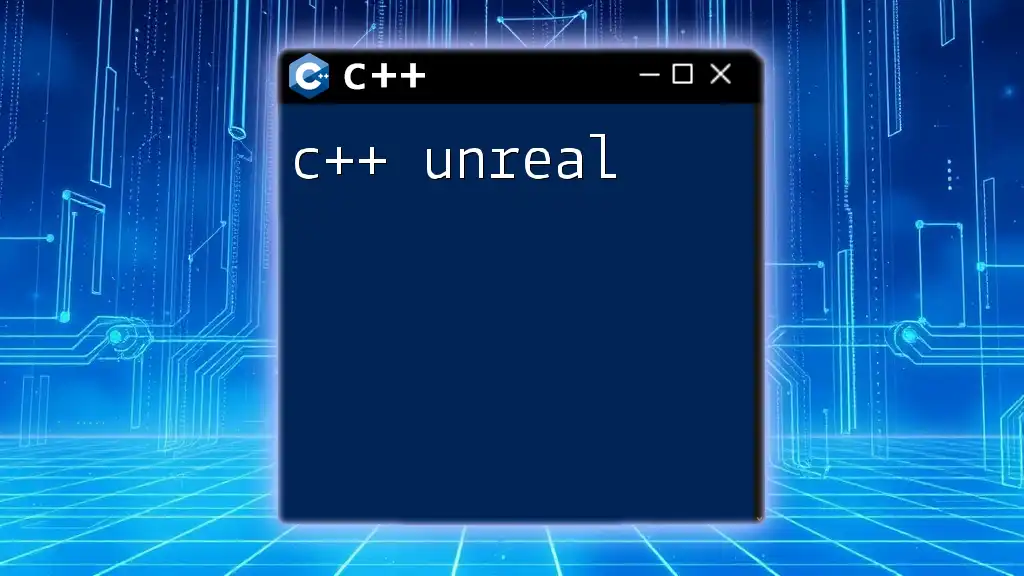
Call to Action
Dive into practice by experimenting with `unordered_map`. Work on exercises that involve manipulating and accessing data efficiently, reaping the benefits of this powerful container in your projects.