A C++ tree map is a data structure that stores key-value pairs in a sorted order, allowing for quick lookup, insertion, and deletion operations.
Here’s a simple code snippet demonstrating how to use a `std::map` in C++:
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> treeMap;
// Inserting key-value pairs
treeMap[1] = "One";
treeMap[2] = "Two";
treeMap[3] = "Three";
// Accessing elements
std::cout << "Key 2: " << treeMap[2] << std::endl;
// Iterating through the tree map
for (const auto& pair : treeMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
Key Features of C++ Tree Maps
Balanced Binary Search Tree Implementation
A tree map is fundamentally based on a balanced binary search tree (BST), typically implemented as a Red-Black Tree in C++. This structure ensures that the tree remains balanced, allowing for efficient data retrieval and modification operations. The rebalancing guarantees that the height of the tree remains logarithmic concerning the number of elements, thereby optimizing performance for various operations.
Using a balanced tree provides numerous benefits:
- Fast lookups due to efficient traversals.
- Insertions and deletions are performed in logarithmic time, making the tree map highly efficient compared to linear data structures.
Key-Value Pair Storage
In C++, a tree map stores entries as key-value pairs, where each key is unique. This structure allows for rapid access, insertion, and deletion based on keys. Unlike other associative containers such as `std::unordered_map`, which uses hash tables, the `std::map` maintains order based on keys.
This ordered feature is crucial for scenarios where sequential access or ordered output is required. For example, retrieving a sorted list of entries is straightforward with a tree map, as it naturally organizes its keys.

The C++ Standard Library: `std::map`
Basic Syntax and Usage
The C++ Standard Library provides `std::map`, which implements a tree map with a set of operations and features that make it essential for developers. When using `std::map`, you leverage the power of the tree map without implementing it from scratch.
Creating a Tree Map with `std::map`
Creating a tree map in C++ is simple. The standard syntax involves including the necessary header and declaring a map. Here’s a basic example:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> treeMap;
treeMap["apple"] = 1;
treeMap["banana"] = 2;
treeMap["grape"] = 3;
return 0;
}
In this snippet, we declare a tree map named `treeMap`, where the keys are strings and the values are integers. This demonstrates how easy it is to store associative data using `std::map`.
Iterating Over a Tree Map
To access elements from a tree map, you can iterate through its entries using a simple range-based loop:
for (const auto &pair : treeMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This code will output each key-value pair in the map, showcasing the ordered nature of the tree map. By iterating in this manner, you not only retrieve the values but also maintain the natural order of the keys.
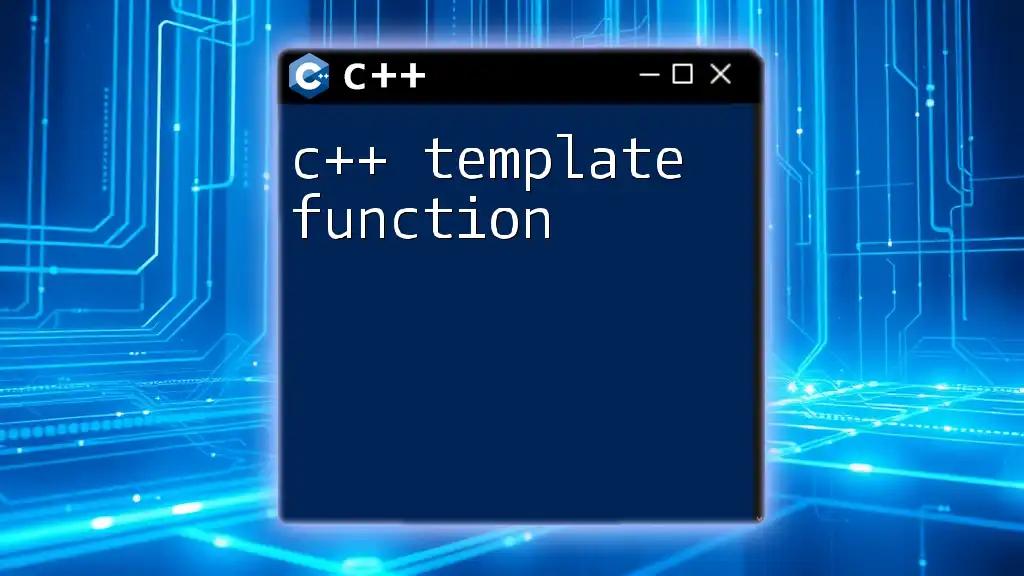
Operations on Tree Maps
Insertion of Elements
Inserting elements into a tree map is done using the insertion operator (`=`) or the `insert` method. For example:
treeMap["orange"] = 4; // Using the assignment operator
treeMap.insert(std::make_pair("kiwi", 5)); // Using the insert method
Both methods add entries to the map efficiently while maintaining order.
Accessing Elements
Accessing elements by key is straightforward and can be done using the `at()` function or the indexing operator (`[]`):
int value = treeMap.at("banana"); // Retrieves value associated with 'banana'
Be aware that using `at()` will throw an exception if the key does not exist, making it safer for accessing elements. In contrast, the indexing operator will create a new entry with a default value if the key doesn’t exist, which may not always be desired.
Finding Elements
To check for the presence of a key, you can utilize the `find` method:
auto it = treeMap.find("apple");
if (it != treeMap.end()) {
std::cout << "Found: " << it->second << std::endl;
}
In this code snippet, `find` returns an iterator to the key if it exists; otherwise, it returns `end()`. This method enables efficient key lookups without raising exceptions, thereby allowing for graceful handling of missing keys.
Removing Elements
Elements can be removed from a tree map using the `erase()` method:
treeMap.erase("grape");
This command removes the entry with the key "grape" from the tree map, and all subsequent operations will reflect this change.
Checking Size and Emptiness
You can easily determine the size of a tree map or whether it’s empty by using:
if (treeMap.empty()) {
std::cout << "The tree map is empty." << std::endl;
} else {
std::cout << "Size: " << treeMap.size() << std::endl;
}
These convenience methods provide useful insight into the current state of the map.

Advanced Features of Tree Maps
Custom Comparing Functions
One of the advantages of using `std::map` is the ability to define custom comparators, allowing you to modify the ordering of the keys. For instance:
struct CustomCompare {
bool operator()(const std::string &a, const std::string &b) const {
return a.length() < b.length(); // Order by string length
}
};
std::map<std::string, int, CustomCompare> customMap;
This enables you to sort the entries based on a criterion of your choice, such as string length in this example.
Lower and Upper Bounds
The `std::map` class also provides functions to obtain lower and upper bounds of keys:
auto lower = treeMap.lower_bound("banana");
auto upper = treeMap.upper_bound("banana");
These functions are useful for determining the range of keys that meet certain criteria, adding a layer of efficiency when handling ordered data.
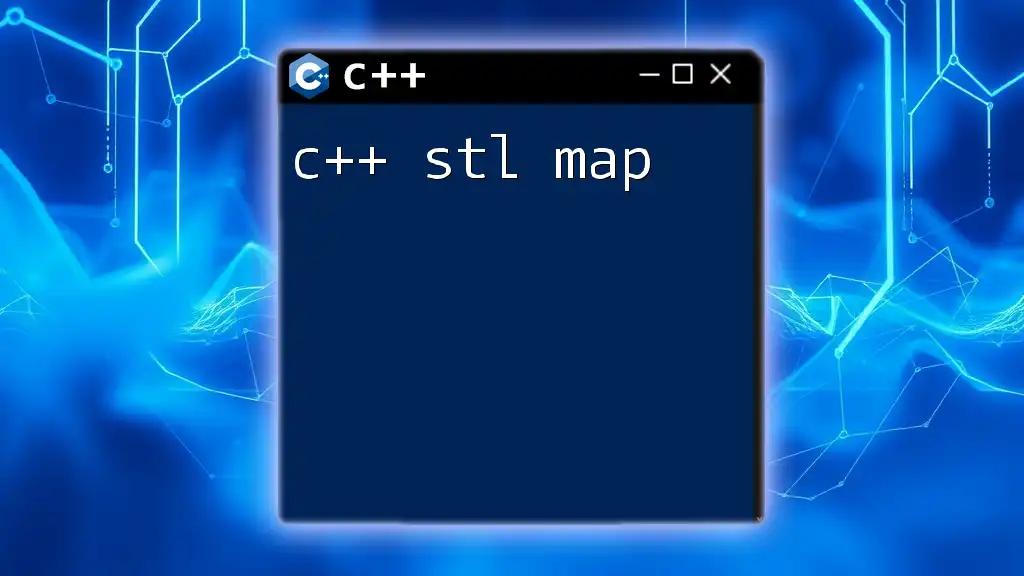
Performance Considerations
Time Complexity Analysis
When assessing the performance of `std::map`, it’s important to note that the key operations have logarithmic time complexities:
- Insertion: O(log n)
- Lookup: O(log n)
- Deletion: O(log n)
Compared to linear data structures, this makes tree maps an excellent choice for maintaining and accessing sorted collections of data.
When to Use Tree Maps
C++ tree maps are particularly useful in scenarios where:
- Order matters (retrieving data in a sorted manner).
- Frequent insertions and deletions are anticipated, and you want logarithmic performance.
- You need unique keys associated with values while maintaining efficient access.

Conclusion
In summary, the C++ tree map, implemented via `std::map`, provides a robust solution for associative data storage, supporting a wide range of operations while maintaining order. With features like custom key comparison and logarithmic performance, tree maps are a powerful asset in any developer's toolkit. By leveraging the capabilities of `std::map`, you can efficiently manage your data in a structured way, ultimately enhancing the performance and organization of your C++ applications.
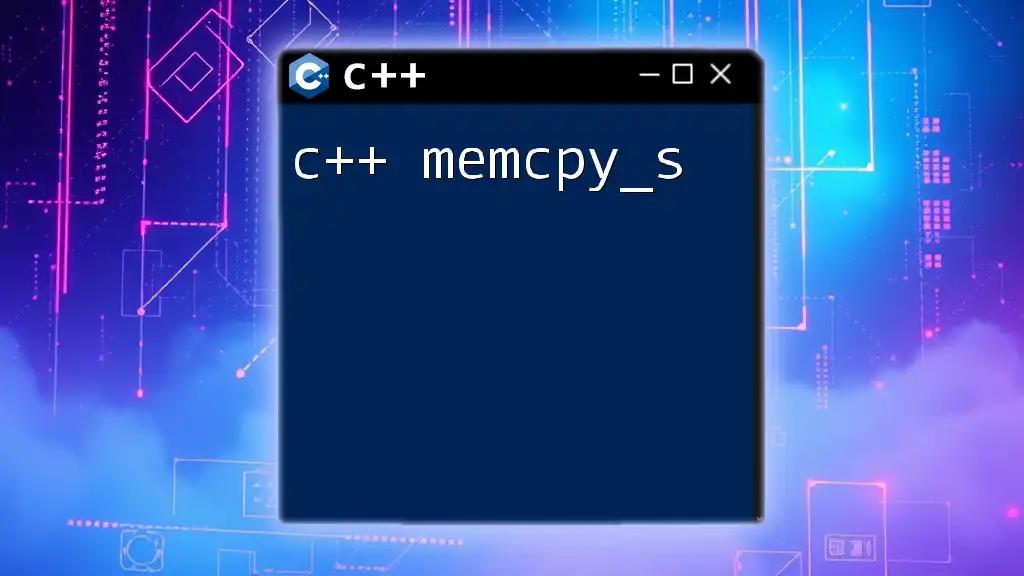
Call to Action
Now that you have a comprehensive understanding of `c++ tree map`, I encourage you to practice implementing and experimenting with tree maps in your own projects. Share your experiences or any challenges you face in the comments below, and let me know if there are specific topics you'd like to explore further!