In C++, a template lambda is a lambda expression that can accept template parameters, allowing you to create more flexible and reusable code for different types.
auto myLambda = [](auto x, auto y) { return x + y; };
auto result = myLambda(5, 3); // result is 8
Understanding Lambda Expressions
What is a Lambda Expression?
A lambda expression in C++ is an anonymous function that you can define inline, which allows you to write short snippets of code that can be executed within the context of your program. This feature enhances code readability and modularity by enabling localized functionality without the overhead of defining separate named functions.
Basic Components of a Lambda
A lambda expression consists of several key components:
- Capture Clause: This part is crucial for allowing the lambda to capture variables from the surrounding scope. You can use `[ ]` to define what is captured.
- Parameters: Just like regular functions, you can specify parameters for your lambda using parentheses `( )`.
- Body: The body encloses the logic of the lambda within `{ }`. You can utilize single-line or multi-line expressions.
Simple Lambda Example
Here’s a straightforward example of a lambda that adds two integers:
auto add = [](int a, int b) { return a + b; };
std::cout << add(3, 4); // Output: 7
In this snippet, the lambda captures no variables and directly uses its parameters. The output, 7, demonstrates the successful addition.
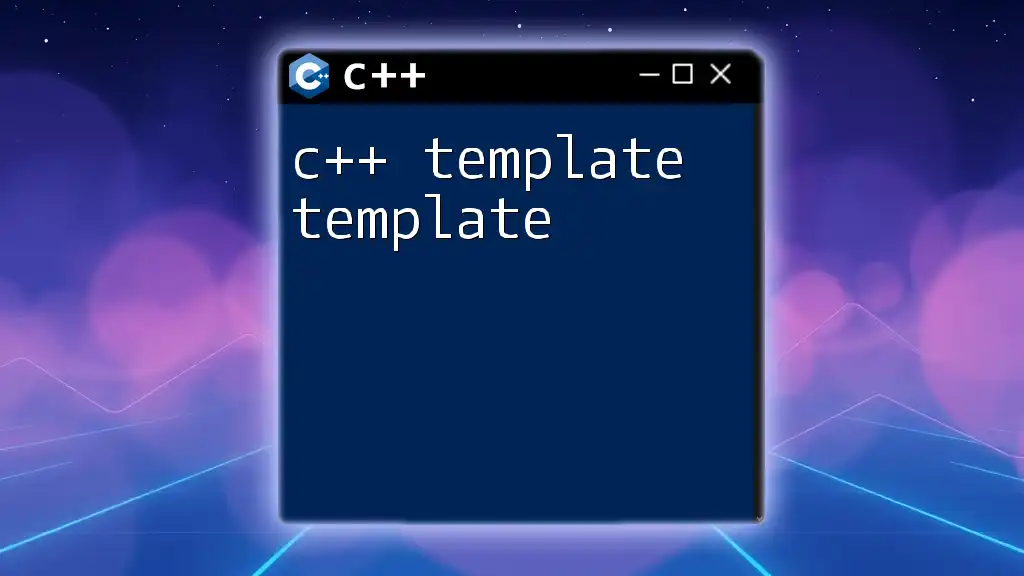
Introduction to Templates in C++
What Are Templates?
Templates in C++ are a feature that allows functions and classes to operate with generic types, enhancing code reusability and flexibility. Rather than defining a function or a class for each data type, you can define one template that works for any type.
Benefits of Using Templates
Using templates introduces several advantages:
- Code Reusability: Write your logic once and apply it across multiple data types—this keeps your codebase cleaner.
- Type Safety: The compiler checks the types at compile time, ensuring that you catch type-related errors before running the code.
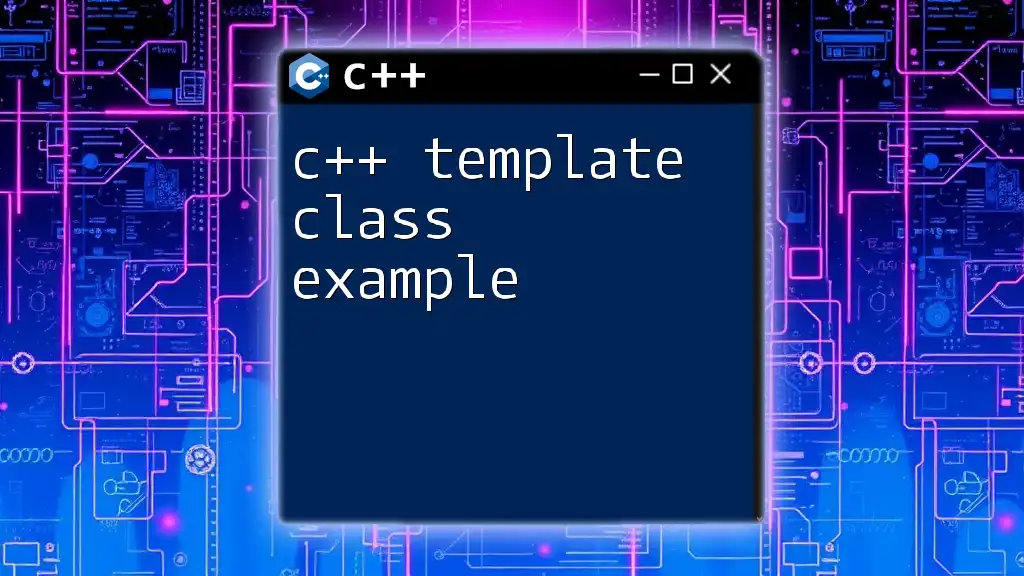
Combining Templates with Lambda Expressions
Why Use Template Lambdas?
Combining lambda expressions with templates provides a powerful way to create flexible and reusable functions. Template lambdas can deduce types automatically, allowing for more generic and versatile code.
Template Lambda Syntax
The syntax for defining a template lambda looks like this:
auto lambda = [](auto arg) { /* body */ };
Here, `auto` is used to indicate that the type will be deduced at compile time, making the lambda capable of accepting different types.
Template Lambda Example
Consider the following example of a lambda that multiplies two values, regardless of their type:
auto multiply = [](auto a, auto b) {
return a * b;
};
std::cout << multiply(5, 10); // Output: 50
std::cout << multiply(2.5, 4.0); // Output: 10.0
In this instance, the lambda demonstrates its versatility by handling both integers and floating-point numbers, producing the expected outputs.
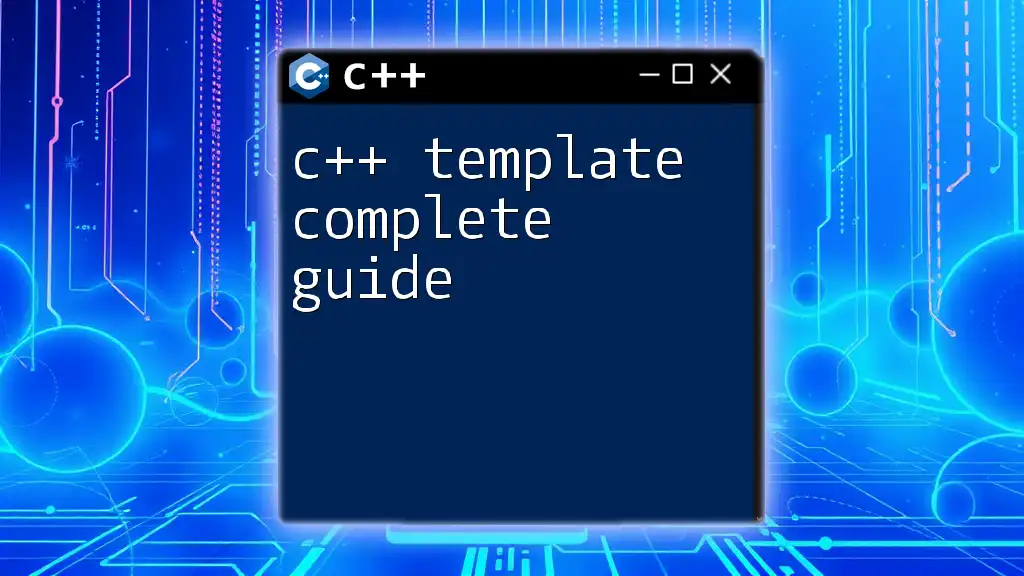
Advanced Topics in Template Lambdas
Higher-Order Template Lambdas
Higher-order functions are those that either take functions as arguments or return a function. Template lambdas can serve as higher-order functions as well. Consider this example:
auto compose = [](auto f, auto g) {
return [f, g](auto x) { return f(g(x)); };
};
In this code, `compose` accepts two functions and returns a new lambda that applies these functions in sequence.
Capturing Templates in Lambdas
Template parameters can also be captured by lambdas. This allows you to create versatile functions that also use specific template types. Here's an example:
template<typename T>
auto make_adder(T x) {
return [x](T y) { return x + y; };
}
Here, `make_adder` is a template function that produces a lambda capable of adding values of type `T`. When invoked, this adds the captured `x` to `y`, making it flexible across different types.
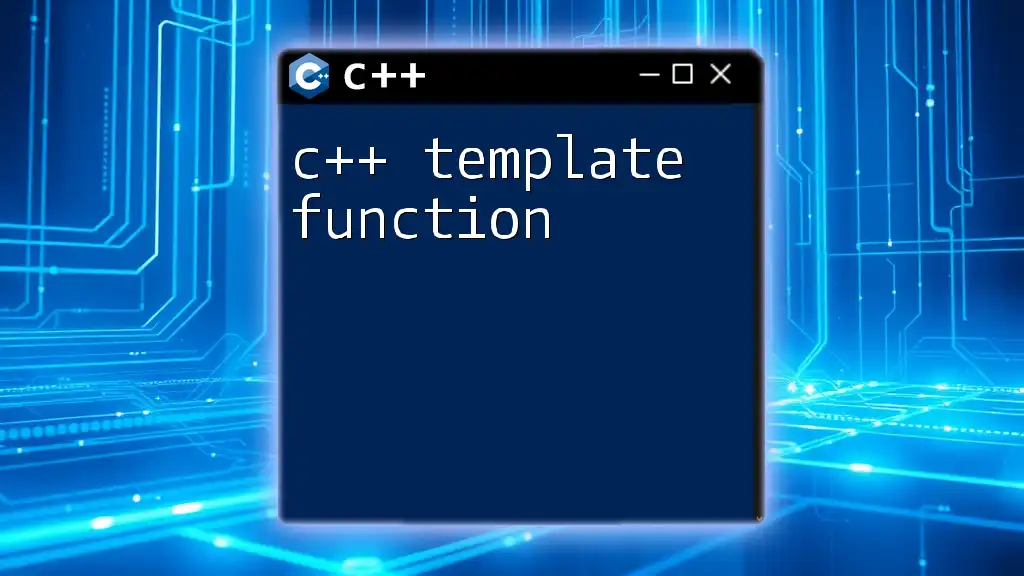
Performance Considerations
Efficiency of Using Template Lambdas
Using template lambdas can yield significant performance benefits. The compiler generally inlines lambdas, effectively reducing function call overhead. Moreover, templates are often specialized at compile time, leading to optimized code that can be as fast as hand-written type-specific functions.
Potential Pitfalls
While powerful, template lambdas do come with certain compromises:
- Complexity in Debugging: The abstraction introduced by templates can complicate the debugging process, as template errors may not be intuitive.
- Compilation Time: Increased usage of templates can lead to longer compilation times due to the instantiation of multiple types.
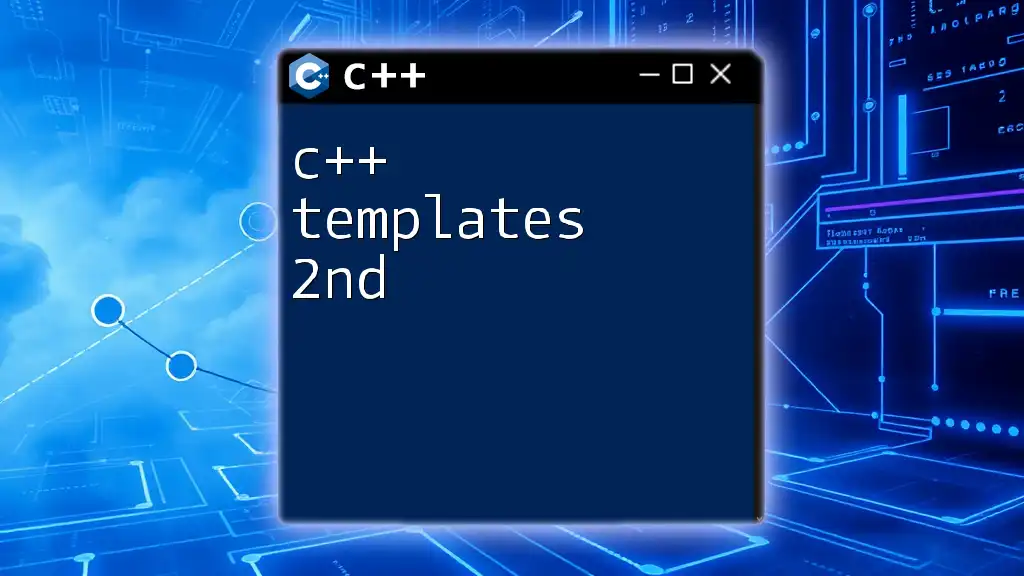
Real-World Applications of Template Lambdas
Use Case in Standard Library Algorithms
Standard library algorithms in C++ offer a practical example of template lambdas. For instance, you can pass a template lambda to `std::transform`:
std::vector<int> vec = {1, 2, 3, 4};
std::transform(vec.begin(), vec.end(), vec.begin(), [](auto x) { return x * x; });
In this example, the lambda squares each element in the vector, illustrating how template lambdas provide an elegant and effective way to manipulate collections.
Practical Example in Applications
In more complex applications, template lambdas can enhance data processing workflows. Here’s how you might define a generic function that processes data using a template lambda:
template<typename Func>
void process_data(const std::vector<int>& data, Func func) {
for (const auto& d : data) {
std::cout << func(d) << "\n";
}
}
By allowing any callable as a parameter, `process_data` shows how template lambdas can be integrated into broader functional programming paradigms within C++.
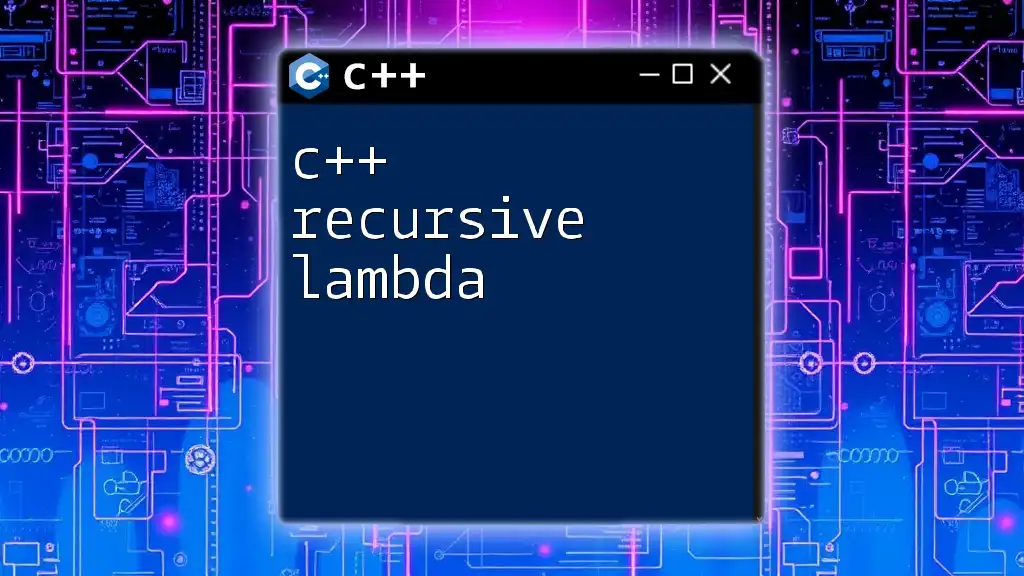
Conclusion
Throughout this guide, you've learned about the intricacies of C++ template lambda expressions, their syntax, use cases, and practical applications. Template lambdas enhance the versatility of your C++ programming, allowing for cleaner, more maintainable, and efficient code. As you continue to explore this powerful feature, you’ll find that it significantly benefits generic programming practices.
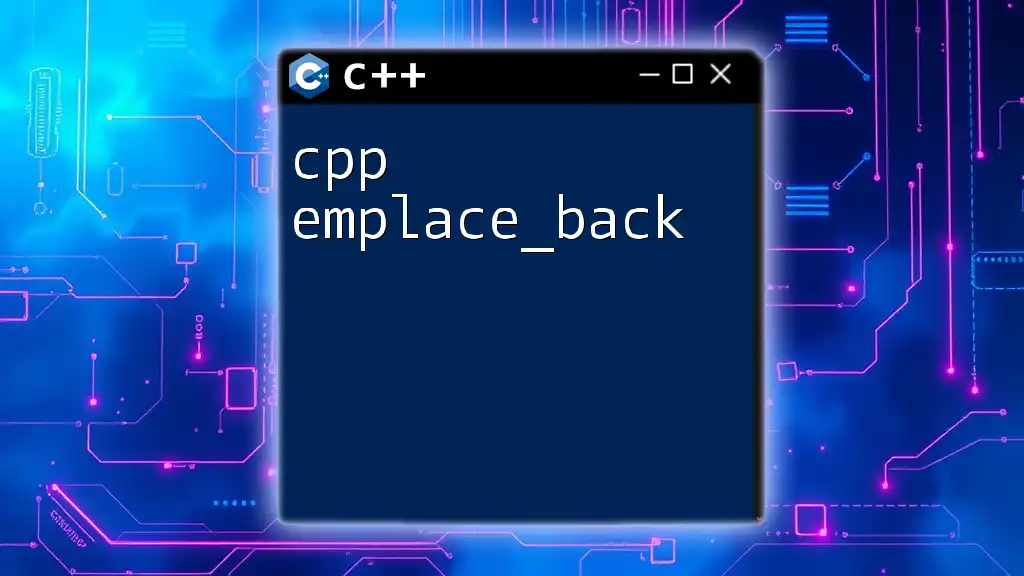
Additional Resources
To further your understanding, consider delving into books focused on advanced C++ programming, exploring online tutorials, and making use of community forums to discuss and troubleshoot your experiences with C++ template lambdas.