C++ templates allow developers to create generic and reusable code components that can work with any data type, enhancing flexibility and reducing code duplication.
Here's a simple example of a function template:
#include <iostream>
using namespace std;
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
cout << "Sum of integers: " << add(5, 3) << endl; // Outputs 8
cout << "Sum of doubles: " << add(5.5, 2.3) << endl; // Outputs 7.8
return 0;
}
Introduction to C++ Templates
C++ templates are a powerful feature of the C++ programming language that allows developers to write generic and reusable code. They can be thought of as blueprints for creating functions or classes without specifying the exact data type. This structure allows for flexibility and can lead to more maintainable and efficient code. By leveraging templates, you can handle a variety of data types with a single implementation.
What is a Template?
A template allows you to define a function or class where the data type is specified as a parameter. The syntax generally looks like this:
template <typename T>
T add(T a, T b) {
return a + b;
}
Here, `T` is a placeholder for any data type. When you call this function with integers, doubles, or any other type, the compiler generates the appropriate version of the function based on the data type passed during the function's invocation.
How Templates Work
Templates operate at compile time rather than run time. When the compiler encounters a template, it creates specific versions of the function or class based on the arguments provided. This means that template functions are just as fast as functions written directly for specific types, which makes them highly efficient.
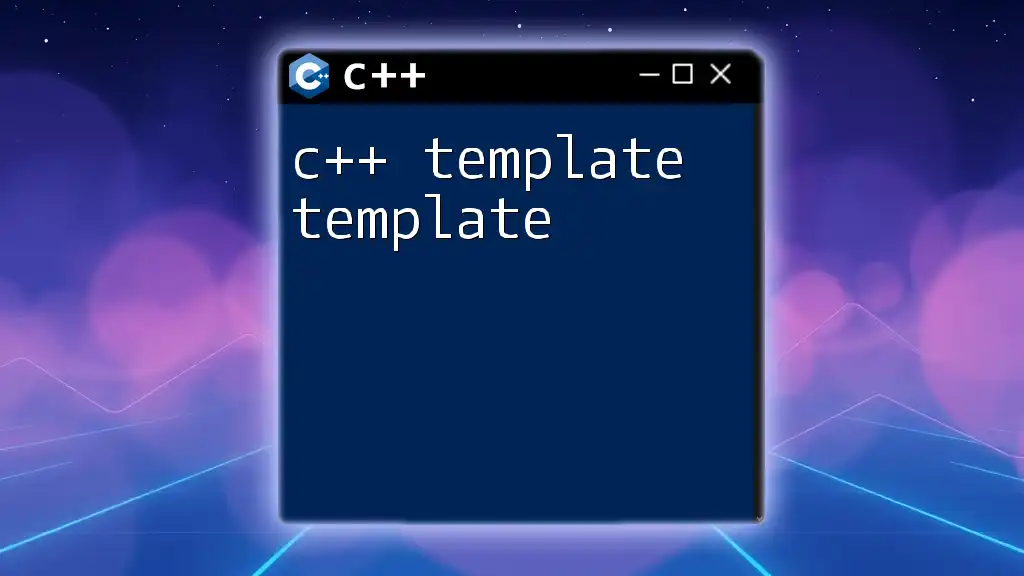
Types of C++ Templates
Function Templates
Function templates allow you to create functions that can operate with different data types. The syntax for a function template is similar to the standard function syntax but includes a template parameter list.
Example: Creating a Simple Function Template
template <typename T>
T max(T a, T b) {
return (a > b) ? a : b;
}
Here, `max` can take any data type, whether it be integers, floats, or user-defined types that support comparison operations.
Benefits of Using Function Templates
- Code Reusability: Write once, reuse across data types.
- Type Safety: The compiler checks types to ensure correctness.
Class Templates
Class templates provide a way to define classes that can handle any data type.
Example: Creating a Class Template for a Stack
template <typename T>
class Stack {
public:
void push(T value);
T pop();
bool isEmpty();
private:
std::vector<T> elements;
};
In this example, the `Stack` class can accommodate any data type specified when creating an object.
Advantages of Class Templates
- Flexibility: Dynamically use different types while keeping a unified interface.
- Scalability: As requirements change, you can easily refactor to accommodate new data types.
Variadic Templates
Variadic templates allow you to accept an arbitrary number of template parameters, making your code even more flexible.
Example: Implementing a Variadic Template Function
template <typename T>
T sum(T t) {
return t;
}
template <typename T, typename... Args>
T sum(T t, Args... args) {
return t + sum(args...);
}
This function can sum multiple values of any data type, focusing on concise and generic code.
Use Cases for Variadic Templates
- Functions that need to accept varying amounts of data: Like logging functions or mathematical operations.
- Reducing boilerplate code: Streamlining implementations without duplicating functionality.
Template Specialization
Template specialization allows you to customize the behavior of templates for specific types.
What is Template Specialization?
When you provide specific implementations for particular data types, the template will use the specialized version for those types rather than the generic one.
Full vs Partial Specialization
- Full specialization specifies completely unique types.
- Partial specialization allows customization based on certain qualities of the type.
Example: Specialized Templates in Action
template <>
class Stack<bool> {
public:
void push(bool value);
bool pop();
private:
std::bitset<10> elements; // More efficient storage for boolean values
};
This specialized class can be optimized for boolean values, enhancing performance.
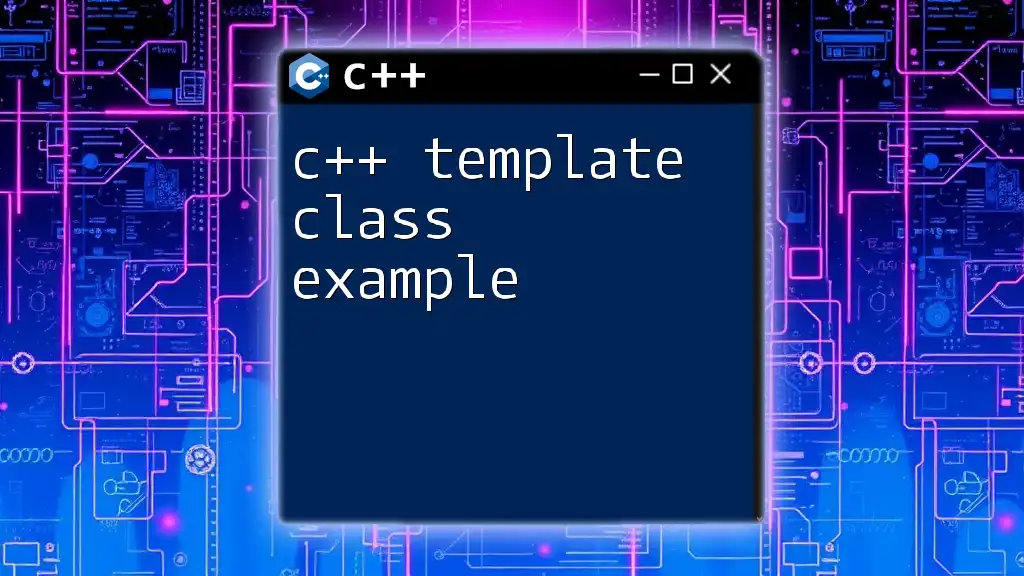
Advanced Template Concepts
Non-type Template Parameters
Non-type template parameters allow you to pass values instead of types.
Explanation and Syntax
Here's how you define a non-type template parameter:
template <typename T, int size>
class Array {
public:
T& operator[](int index);
private:
T arr[size];
};
Example: Creating an Array Wrapper Class
Array<int, 10> myArray;
In this example, `size` is a non-type template parameter, and you create a fixed-size array at compile time.
Template Metaprogramming
Template metaprogramming leverages templates to perform computations at compile time.
What is Template Metaprogramming?
This allows developers to write code that executes during compilation rather than execution.
Example: Compile-Time Factorial Calculation
template<int N>
struct Factorial {
static const int value = N * Factorial<N - 1>::value;
};
template<>
struct Factorial<0> {
static const int value = 1;
};
You can use `Factorial<5>::value` to get the factorial of 5 at compile time.
Benefits of Using Metaprogramming
- Optimized code: Reduce run-time calculations.
- Enhanced type safety: Compile-time errors can catch issues early in development.
The Curiously Recurring Template Pattern (CRTP)
CRTP is a design pattern used in C++ to facilitate code reuse while preserving efficient operations.
Explanation of CRTP
The pattern involves a class template containing a derived class as its template argument.
Example: Implementing CRTP for Code Reuse
template <typename T>
class Base {
public:
void interface() {
static_cast<T*>(this)->implementation();
}
};
class Derived : public Base<Derived> {
public:
void implementation() {
// Derived class implementation
}
};
Here, `Base` relies on its derived class for implementations, promoting code reuse.
Advantages of CRTP in Design Patterns
- Static polymorphism: Achieve polymorphic behavior without the overhead of dynamic dispatch.
- Easier testing and maintenance: Each class can be treated in isolation while benefiting from the common base.
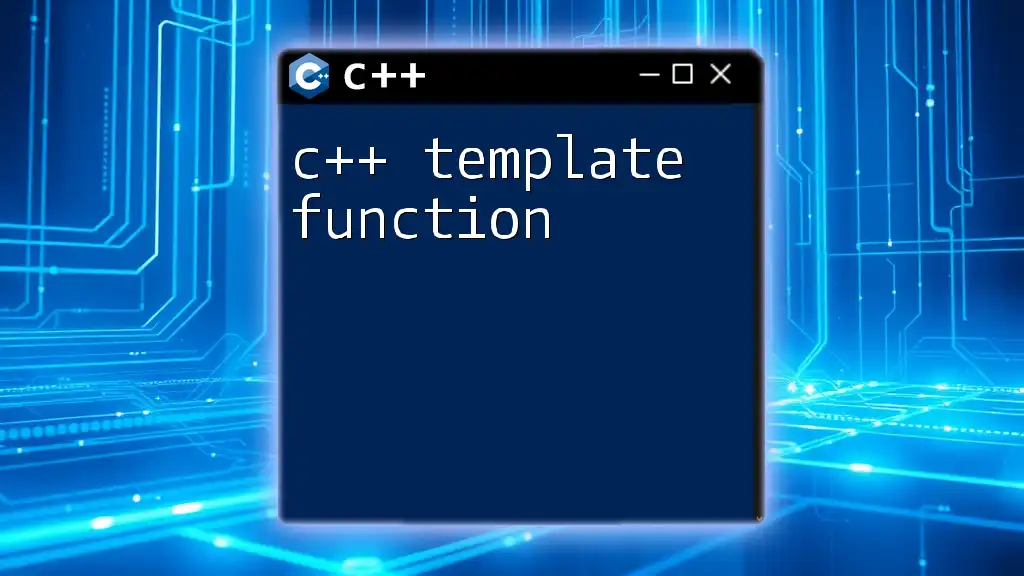
Best Practices for Using C++ Templates
When to Use Templates
Templates should be employed when you need a function or class to work with different types while maintaining type safety and flexibility. Ideal scenarios include:
- Libraries that operate on generic data.
- Algorithms that shouldn’t be rewritten for every data type.
Template Code Readability
Maintaining readability in template-heavy code is crucial. Here are some tips:
- Use Meaningful Names: Avoid single-character type parameters unless they are universally understood.
- Comment Generously: Clear documentation can help others (and future you!) understand complex template logic.
Performance Considerations
While templates are powerful, they can impact compile time and code bloat. It's essential to:
- Profile Your Code: Determine if template usage is causing performance bottlenecks.
- Be Cautious of Heavy Templates: Use template features judiciously to prevent slow compile times.
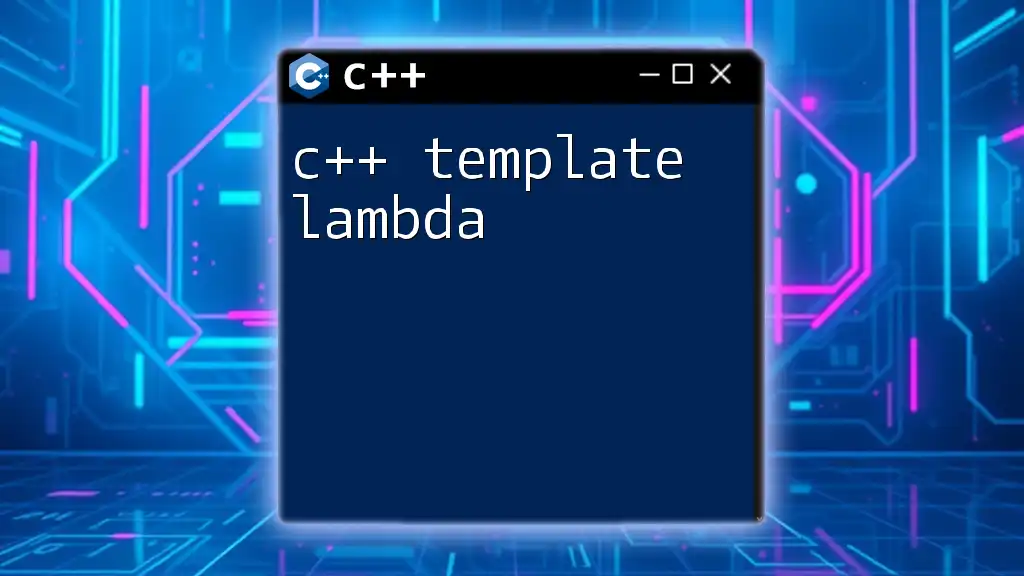
Conclusion
In this C++ template complete guide, we've explored the fundamentals and complexities of C++ templates, including function templates, class templates, variadic templates, template specialization, and more. Templates offer a versatile toolset for developers aiming to write generic, type-safe, and reusable code. Embrace the power of templates as you continue your journey in C++ programming!
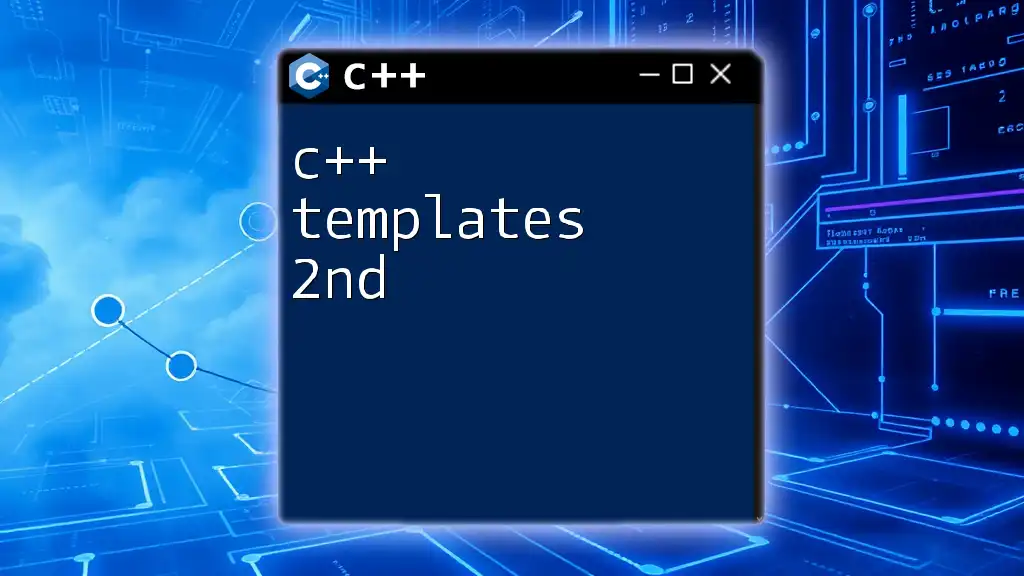
Additional Resources
To deepen your learning, consider exploring the following resources:
- Recommended Books on C++ Templates
- Online Forums and Communities for C++ Template Discussions
- Links to Popular C++ Template Libraries and Tools
As the landscape of C++ continues to evolve, staying current with the latest practices and patterns will ensure you remain an effective and efficient programmer. Keep experimenting with templates, and they will soon become an invaluable part of your coding toolkit.