C++ complex numbers are part of the Standard Template Library (STL) and can be easily manipulated using the `std::complex` template class, which allows for arithmetic operations on both real and imaginary components.
Here’s a simple code snippet to demonstrate how to use complex numbers in C++:
#include <iostream>
#include <complex>
int main() {
std::complex<double> num1(3.0, 4.0); // 3 + 4i
std::complex<double> num2(1.0, 2.0); // 1 + 2i
std::complex<double> sum = num1 + num2; // Adding complex numbers
std::cout << "Sum: " << sum << std::endl; // Outputs: Sum: (4,6)
return 0;
}
Understanding Complex Numbers in C++
What are Complex Numbers?
Complex numbers are mathematical entities used extensively in mathematics and engineering. They consist of two parts: the real part and the imaginary part. In mathematical terms, a complex number can be represented as a + bi, where a is the real component, and bi is the imaginary component, with i being the imaginary unit.
The concept of complex numbers extends the traditional number system, providing a remedy for equations that have no real solutions. For example, the equation \(x^2 + 1 = 0\) has solutions in the domain of complex numbers, namely \(i\) and \(-i\).
The Components of Complex Numbers
Real Part
The real part of a complex number is simply the coefficient of the non-imaginary component. For the complex number \(3 + 4i\), the real part is 3. This representation is useful in various applications, including electrical engineering and signal processing.
Imaginary Part
The imaginary part is the coefficient of the imaginary unit i in the complex number. In the previous example, the imaginary part is 4. Together, these components provide a full representation of the complex number, allowing for operations unique to this number set.
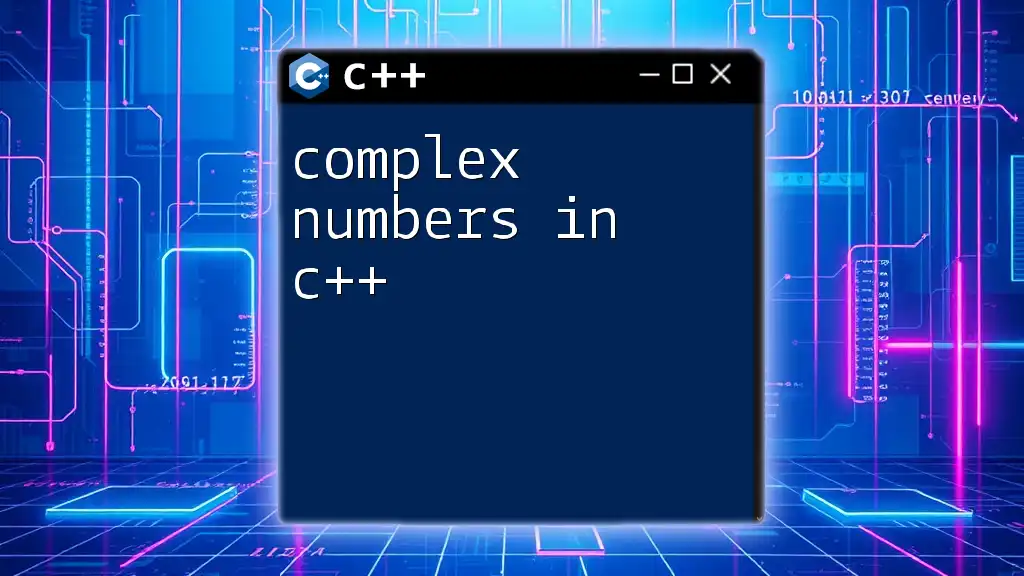
Using the `<complex>` Header
What is the `<complex>` Header?
The `<complex>` header in C++ is part of the Standard Template Library and provides all the necessary functionalities for complex number operations. This library includes functions for arithmetic, relational comparisons, polar representation, and more, making it a powerful tool for developers working with complex numbers.
Including the `<complex>` Header
To use complex numbers in C++, you must include the `<complex>` header at the top of your program. This can be done as follows:
#include <complex>
#include <iostream>
int main() {
// Example usage will be covered below
return 0;
}
Once you include this header, you can declare and manipulate complex numbers effectively.
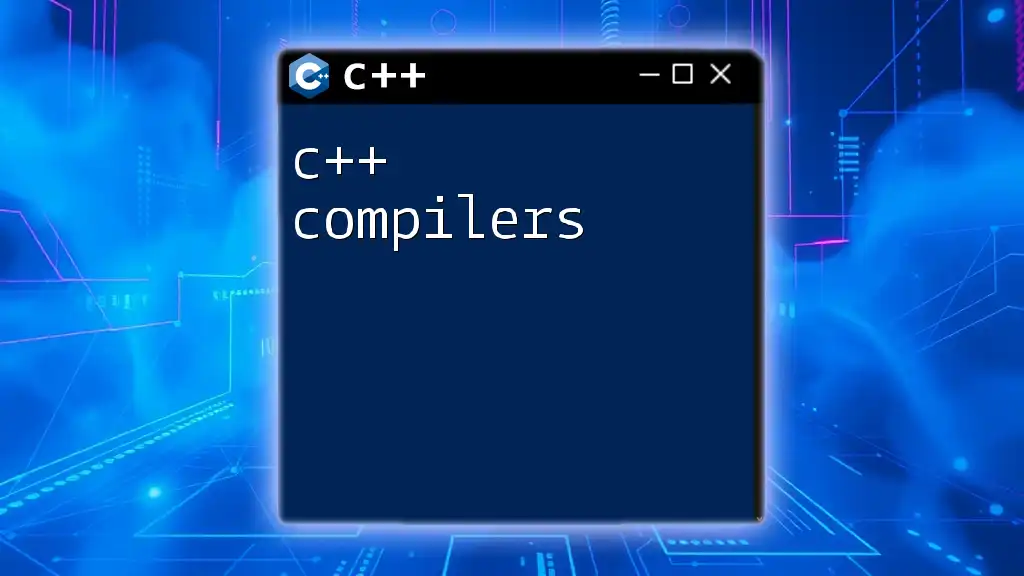
Creating Complex Numbers in C++
Declaring Complex Numbers
Creating complex numbers in C++ is straightforward. You can declare a complex number using the `std::complex` template. For example:
std::complex<double> num1(3.0, 4.0); // Represents the complex number 3 + 4i
With this declaration, num1 can now be utilized in various complex number operations, as shown in the following examples.
Initializing Complex Numbers
Complex numbers can be initialized in different ways, including using default constructors or specifying real values. Here’s an example illustrating different initialization methods:
std::complex<double> num2; // Default constructor initializes to 0 + 0i
std::complex<double> num3(5.0); // Initializes to 5.0 + 0i
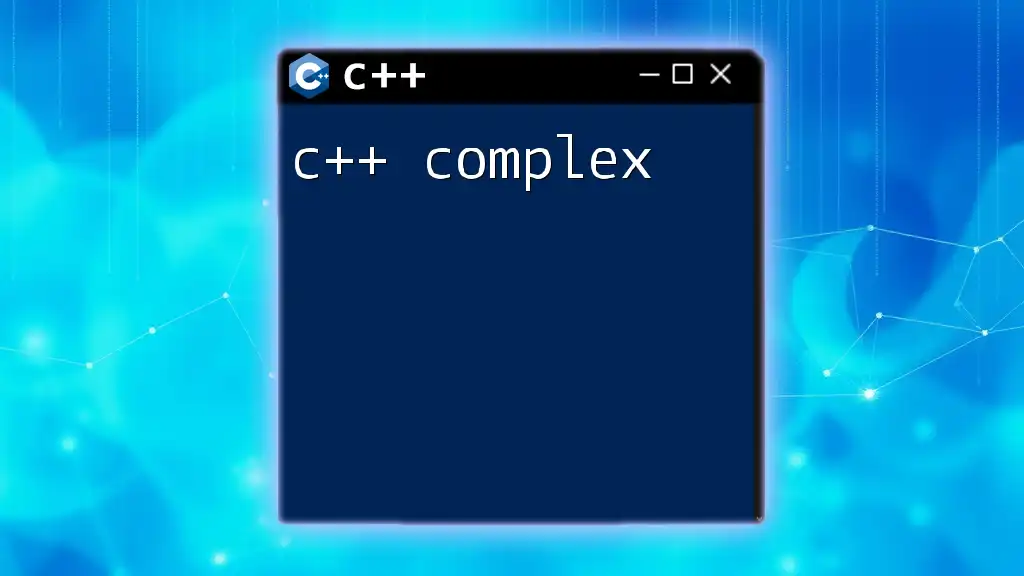
Basic Operations with Complex Numbers
Addition and Subtraction
You can easily add and subtract complex numbers using the `+` and `-` operators. The operations combine the respective real and imaginary parts. Here’s an example:
std::complex<double> num1(3.0, 4.0);
std::complex<double> num2(1.0, 2.0);
std::complex<double> sum = num1 + num2; // Results in 4 + 6i
std::complex<double> difference = num1 - num2; // Results in 2 + 2i
Multiplication and Division
Multiplying and dividing complex numbers may seem more complex, but it follows a straightforward arithmetic rule:
std::complex<double> product = num1 * num2; // (3 + 4i)(1 + 2i) results in -5 + 10i
std::complex<double> quotient = num1 / num2; // (3 + 4i) / (1 + 2i) results in 2.2 - 0.4i
The multiplication involves the distributive property, while division uses the conjugate of the denominator to simplify the result.
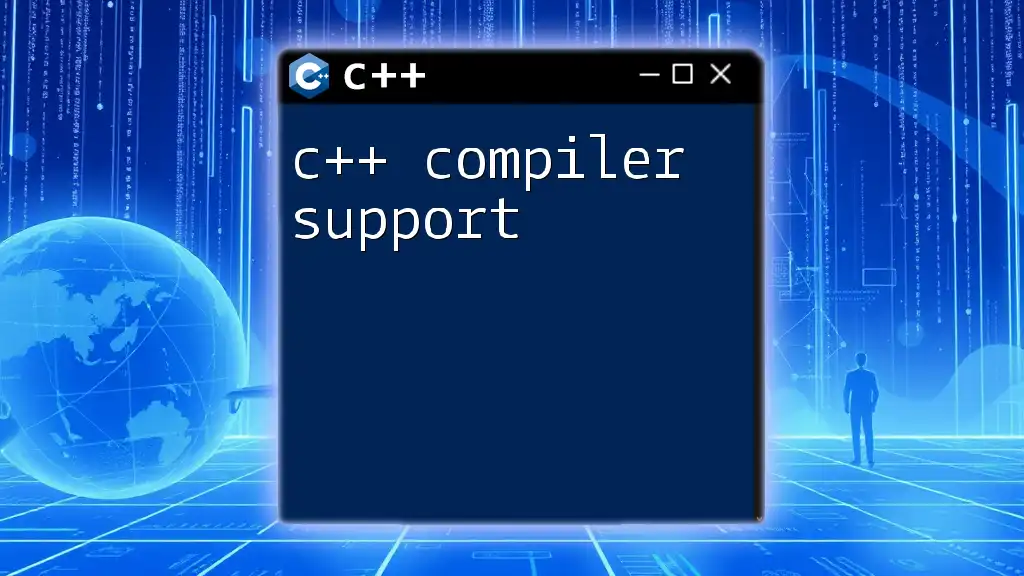
Advanced Features of Complex Numbers
Conjugate of Complex Numbers
The conjugate of a complex number is obtained by changing the sign of the imaginary part. It is crucial in various calculations, particularly when dividing complex numbers. For instance, given num1 = 3 + 4i, the conjugate is computed as follows:
std::complex<double> conjugate = std::conj(num1); // Results in 3 - 4i
Magnitude and Phase
Finding the magnitude and phase of a complex number can provide insights into its properties, such as distance from the origin and direction in the complex plane:
double magnitude = std::abs(num1); // Computes the magnitude using |3 + 4i| = 5
double angle = std::arg(num1); // Computes the phase angle (in radians) of the complex number
These functions are essential in applications such as signal analysis, where magnitude and phase represent amplitude and phase shift, respectively.
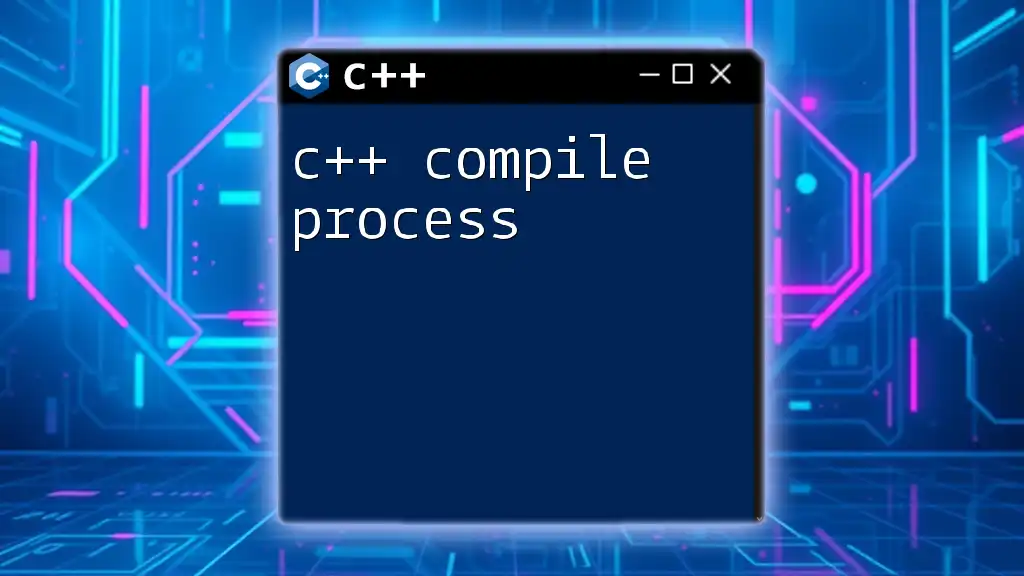
Using Complex Numbers in C++ Functions
Passing Complex Numbers to Functions
Complex numbers can be passed to functions just like any other data type. This allows for modular programming and better code organization. Here’s an example function that takes a complex number as an argument:
void displayComplex(const std::complex<double>& c) {
std::cout << "Complex number: " << c << std::endl;
}
Returning Complex Numbers from Functions
You can also return complex numbers from functions, allowing versatile operations and calculations. Here’s how it works:
std::complex<double> addComplex(const std::complex<double>& c1, const std::complex<double>& c2) {
return c1 + c2; // Returns the sum of two complex numbers
}
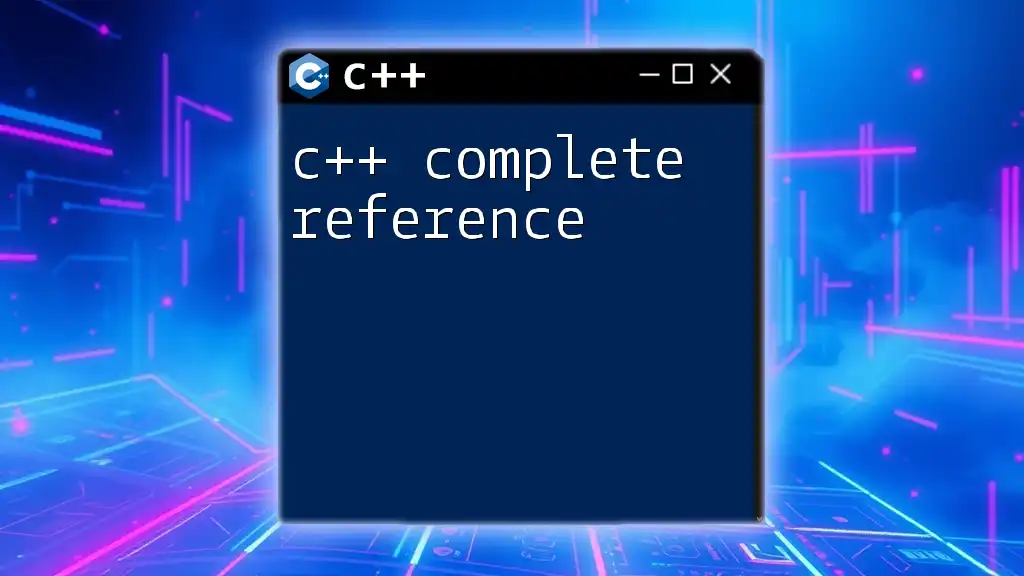
Common Pitfalls and Best Practices
Misunderstandings in Complex Arithmetic
One common pitfall is misunderstanding operations with complex numbers. It's crucial to remember that while the rules of arithmetic apply, the addition of the imaginary unit introduces new challenges. When adding or subtracting complex numbers, always combine corresponding parts cautiously.
Performance Considerations
When working with complex numbers, especially in computational heavy-lifting tasks such as numerical simulations, it's essential to note performance implications. Using the `<complex>` library is generally optimized for real-time calculations; however, unnecessary conversions between complex and real numbers can slow down execution.
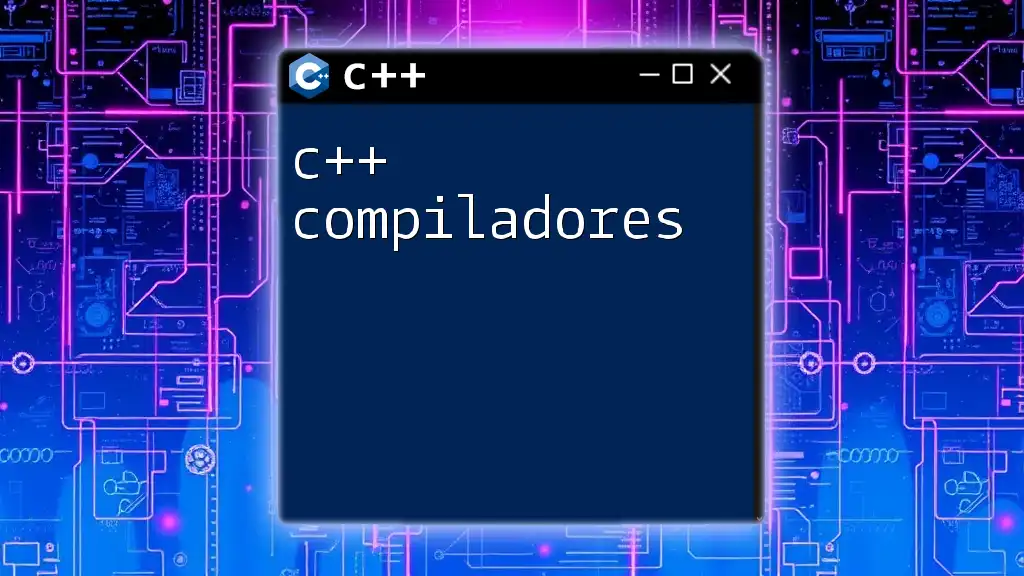
Conclusion
C++ complex numbers offer a powerful means to handle mathematical computations that involve both real and imaginary components. Whether you're working on engineering simulations, graphical programming, or mathematical modeling, mastering complex numbers in C++ can significantly enhance your programming toolkit.
By utilizing the `<complex>` header and understanding the fundamentals surrounding complex arithmetic, you will be well-equipped to leverage this tool in various practical applications in C++. As you continue to explore C++, consider diving deeper into related mathematical concepts and exploring how they can be seamlessly integrated into your programming projects.