To determine the number of digits in an integer using C++, you can divide the number by 10 repeatedly until it becomes zero, counting the divisions as you go; here’s a simple code snippet demonstrating this:
#include <iostream>
int countDigits(int n) {
int count = 0;
while (n != 0) {
n /= 10;
count++;
}
return count;
}
int main() {
int number = 12345;
std::cout << "Number of digits: " << countDigits(number) << std::endl;
return 0;
}
Understanding C++ and the Cout Command
What is C++?
C++ is a powerful, high-level programming language that enables developers to create efficient and complex software systems. Renowned for its performance and versatility, it remains a vital tool for applications ranging from system software to game development.
Introduction to cout
In C++, `cout` is the standard output stream, part of the iostream library, which allows developers to display information on the console. Its basic syntax is straightforward:
cout << "Hello, World!";
This command outputs "Hello, World!" to the console. Understanding how to effectively use `cout` is crucial, especially when working with numerical data, including the number of digits in integers.
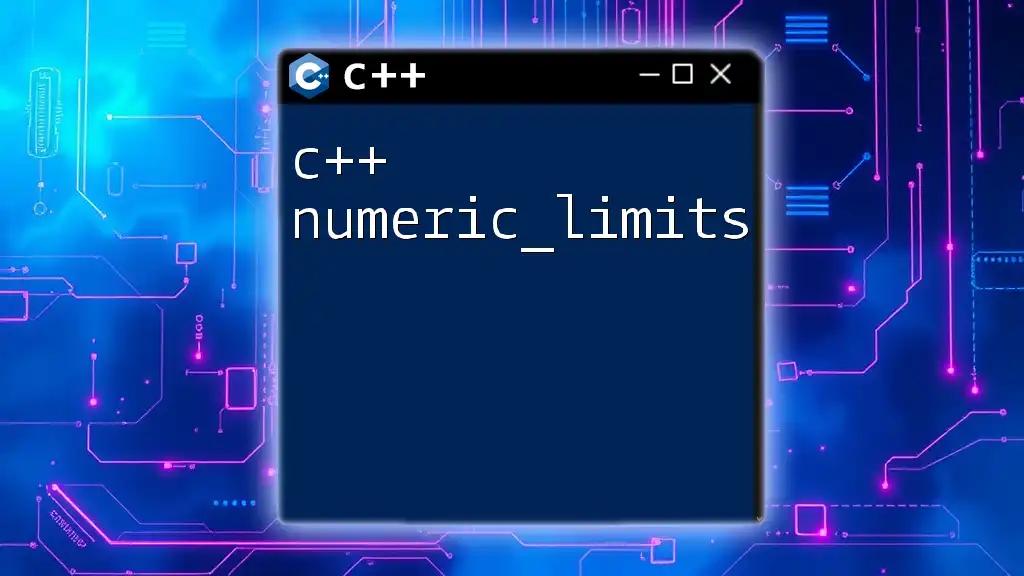
Determining the Number of Digits in a Number
Why Count Digits?
Counting the number of digits in a number is a common requirement in programming. It can serve various purposes, such as data validation, formatting output, or even for algorithms that need to process numerical values differently based on their lengths. Mastering this skill will allow you to enhance your C++ programming repertoire.
Different Approaches to Count Digits
Using Mathematical Operations
One of the most intuitive ways to count the digits in an integer is by utilizing mathematical operations. The method involves repeatedly dividing the number by 10 until it becomes zero. Here’s how it works in code:
int countDigits(int number) {
int count = 0;
if (number == 0) return 1; // Special case for zero
while (number != 0) {
count++;
number /= 10;
}
return count;
}
In this code snippet, we start with a `count` of zero. We check if the number is zero; if so, we return 1 (a special case). Then, we enter a loop that increments the count each time we divide the number by 10, effectively peeling off each digit. This method is reliable and easy to understand.
Using Logarithmic Function
Another efficient technique for counting digits is through the use of logarithms. By utilizing the logarithm base 10, you can determine the number of digits without needing loops or conditionals.
#include <cmath>
int countDigits(int number) {
if (number == 0) return 1; // Special case
return floor(log10(abs(number))) + 1;
}
In this snippet, we apply `log10` to the absolute value of the number and use `floor` to round down to the nearest whole number. By adding 1, we arrive at the total digit count. This method is excellent for its performance, especially with large numbers.
String Conversion Method
Lastly, a simple yet effective approach is converting the number to a string. By doing this, you can leverage string properties to retrieve the count.
#include <string>
int countDigits(int number) {
return std::to_string(abs(number)).length();
}
In this example, we convert the absolute value of the number to a string and then retrieve its length. This method is particularly clean and straightforward, making it easier to implement for beginners.

Displaying the Number of Digits Using Cout
Once we have counted the digits using one of the methods outlined above, we can easily display the result with `cout`. Here is a complete example program demonstrating this:
#include <iostream>
#include <cmath>
#include <string>
using namespace std;
int countDigits(int number) {
return std::to_string(abs(number)).length();
}
int main() {
int number = 12345;
cout << "The number of digits in " << number << " is: " << countDigits(number) << endl;
return 0;
}
This program first defines the `countDigits` function using the string conversion method. In the `main` function, we declare an integer, then use `cout` to output the number of digits for the specified number. The syntax is clean and direct, making it easy to follow.

Edge Cases and Considerations
Handling Negative Numbers
When counting digits, it is important to consider negative numbers. Mathematically, the number of digits should ignore the negative sign, so we use the `abs()` function in our calculations. This ensures that we are only counting the actual digits.
Zero and Special Cases
Zero is a unique case in digit counting. While it may seem that zero has no digits, mathematically it is defined to have a single digit. Thus, all methods discussed must account for this by specifically checking when the input is zero.
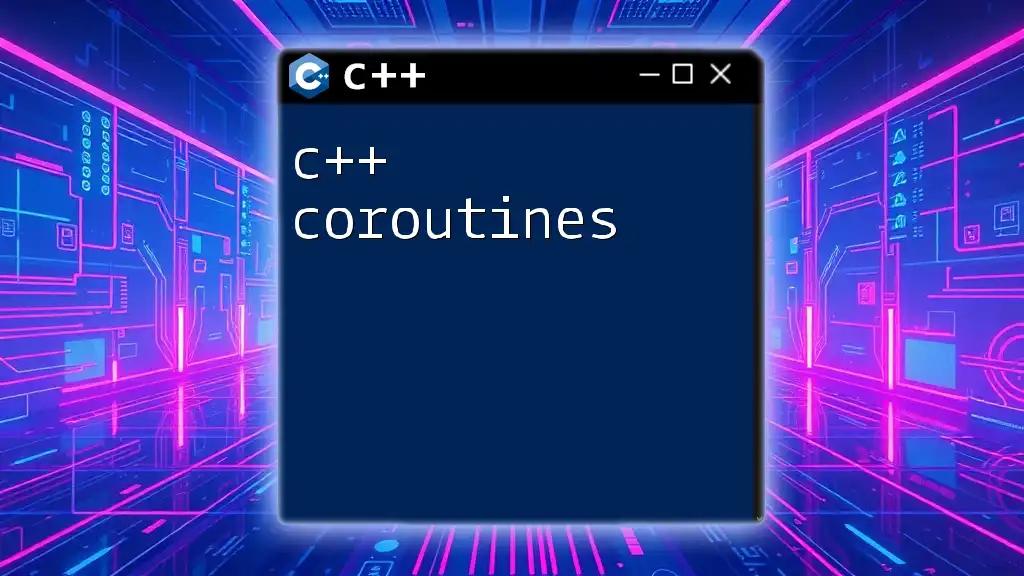
Performance Considerations
When choosing the method to count digits, consider the context and requirements of your application. The mathematical approaches and logarithmic techniques are generally faster, especially for large numbers, as they avoid the overhead of string manipulation. However, the string conversion method is easier for beginners and can be preferable for smaller or less performance-critical applications.
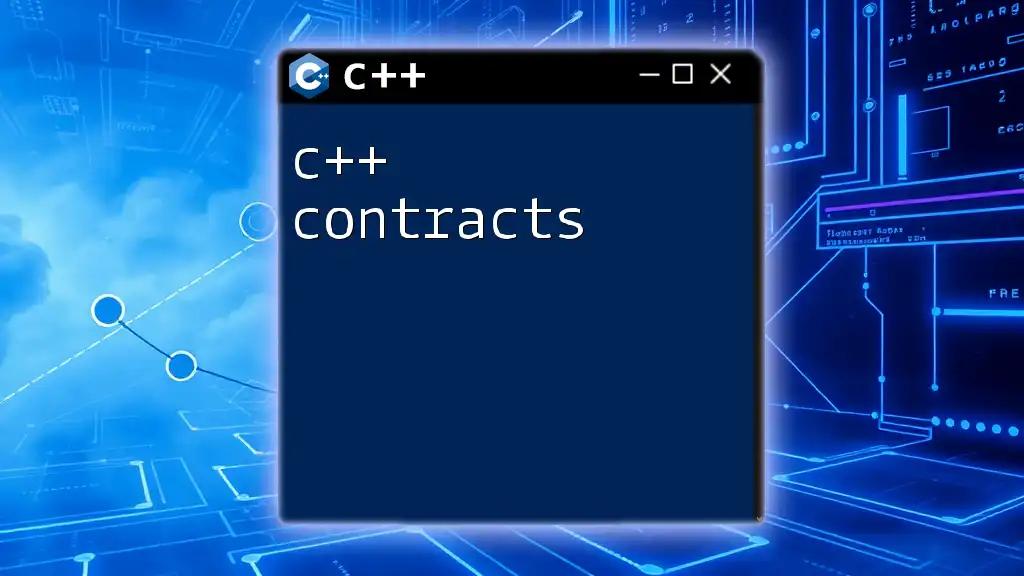
Conclusion
Understanding how to determine and display the c++ cout number of digits equips you with a valuable skill in programming. Each method we discussed—mathematical calculations, logarithmic checks, and string conversions—has its advantages. Practicing these techniques will not only enhance your coding capabilities but also prepare you for more complex numerical manipulations and data processing in C++.
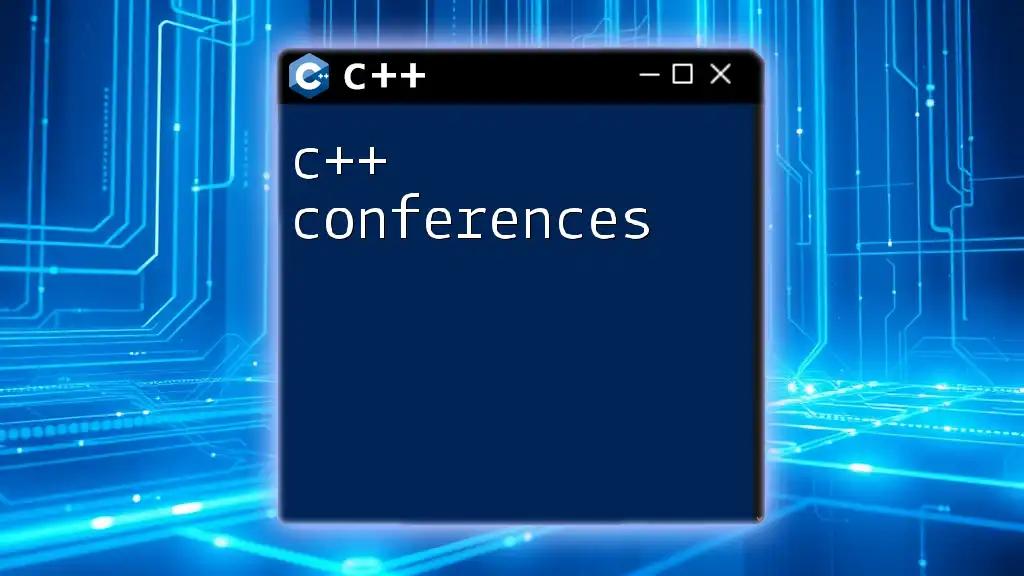
Frequently Asked Questions
Common Queries on Digit Counting
- What if I need to count digits in a floating-point number?
- For floating-point numbers, the approach varies slightly. It often involves converting to a string or separating out the integer part for precision.
- Can I count digits in binary, octal, or hexadecimal?
- Yes, by adjusting the base in the logarithmic approach or converting the value to the desired base format.
Additional Resources
For further exploration, consider delving into advanced C++ topics, particularly focusing on data structures or algorithms that require similar numerical manipulations. Online platforms and C++ documentation are excellent places to expand your knowledge even further.