C++ overloading allows the same function name or operator to be used with different types or numbers of parameters, enhancing code readability and usability.
#include <iostream>
// Function Overloading
void display(int i) {
std::cout << "Integer: " << i << std::endl;
}
void display(double d) {
std::cout << "Double: " << d << std::endl;
}
void display(std::string s) {
std::cout << "String: " << s << std::endl;
}
int main() {
display(5); // Calls display(int)
display(5.5); // Calls display(double)
display("Hello"); // Calls display(string)
return 0;
}
What is Overloading in C++?
C++ overloading refers to the ability to define multiple functions or operators with the same name but with different parameters or operands. This feature enhances the flexibility and readability of your code by allowing functions to behave differently based on their inputs. Essentially, it enables a programmer to make a method or operator work with different data types or numbers of arguments seamlessly.
Importance of C++ Overloading: Overloading is particularly useful in situations where you want to perform similar operations on different types of data without cluttering your code with multiple function names. This leads to cleaner, more organized code that’s easier for both the programmer and others who may read the code later.
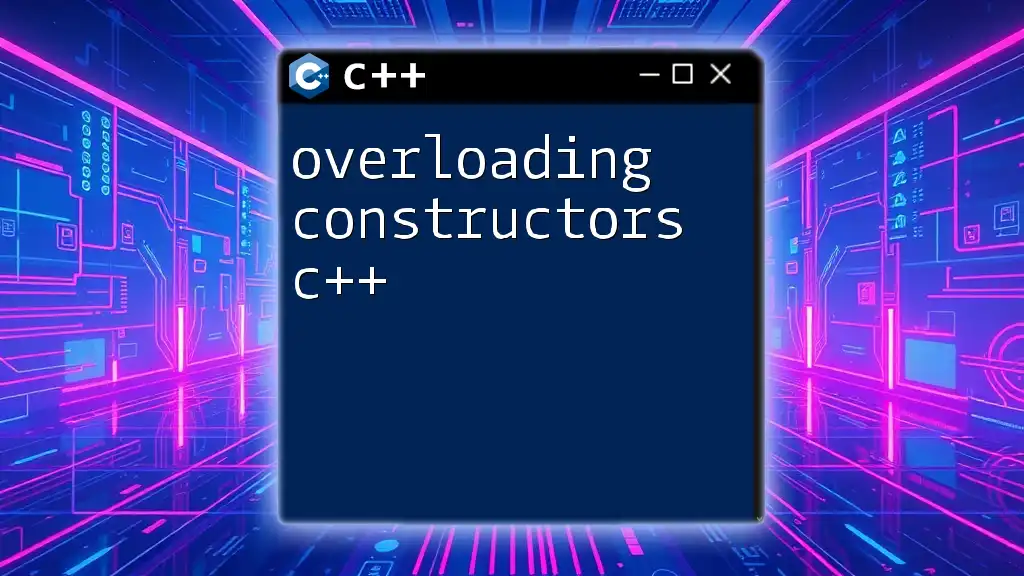
Types of Overloading
C++ supports two primary types of overloading: function overloading and operator overloading.
Function Overloading
Function overloading is the process of having multiple functions with the same name but different signatures (i.e., different parameter lists).
Examples of Function Overloading
Consider the following example demonstrating basic function overloading:
void print(int i) {
std::cout << "Integer: " << i << std::endl;
}
void print(double d) {
std::cout << "Double: " << d << std::endl;
}
void print(std::string s) {
std::cout << "String: " << s << std::endl;
}
In this example, we have defined three `print` functions, each capable of handling a different data type. The compiler determines which function to invoke based on the argument type passed during the function call. The resolution occurs at compile time, making this approach efficient.
Advantages and Disadvantages of Function Overloading
Advantages:
- Improved Readability: Overloading reduces the need for multiple, often cumbersome function names, making the code more intuitive.
- Maintainability: Code that uses overloaded functions is generally easier to manage and extend.
Disadvantages:
- Potential Confusion: Overloading may create confusion for those unfamiliar with the specific function overloads.
- Debugging Complexity: Having multiple versions of a similar function can complicate bug tracking.
Operator Overloading
Operator overloading in C++ allows developers to redefine the way operators work with user-defined types (classes).
Example of Operator Overloading
To illustrate the concept, let’s consider overloading the `+` operator for a simple class representing complex numbers:
class Complex {
public:
float real;
float imag;
Complex operator + (const Complex &obj) {
Complex temp;
temp.real = real + obj.real;
temp.imag = imag + obj.imag;
return temp;
}
};
In this case, the `+` operator has been redefined to add together two `Complex` objects. When this operator is used, the compiler invokes the class's overloaded `+` operator function instead of the default behavior.
Overloading the `<<` Operator for Output
Another common example is overloading the insertion (<<) operator for output:
#include <iostream>
class Complex {
public:
float real;
float imag;
friend std::ostream& operator<<(std::ostream &out, const Complex &c) {
out << c.real << " + " << c.imag << "i";
return out;
}
};
By using a friend function, this code allows complex numbers to be easily printed using `std::cout`, making it feel natural to use in the context of C++.
Benefits and Drawbacks of Operator Overloading
Benefits:
- Expressiveness: Overloaded operators enable users to interact with objects in a way that is similar to built-in types, enhancing the readability of operations.
- Custom Behavior: You can tailor how operators behave for your own classes, providing a powerful tool for abstraction.
Drawbacks:
- Misleading Use: If not handled carefully, overloaded operators can lead to code that is confusing or behaves unexpectedly for someone who is not familiar with the customizations.
- Complexity in Understanding: Programmers new to your code must not only understand the class itself but also how its operators are defined.
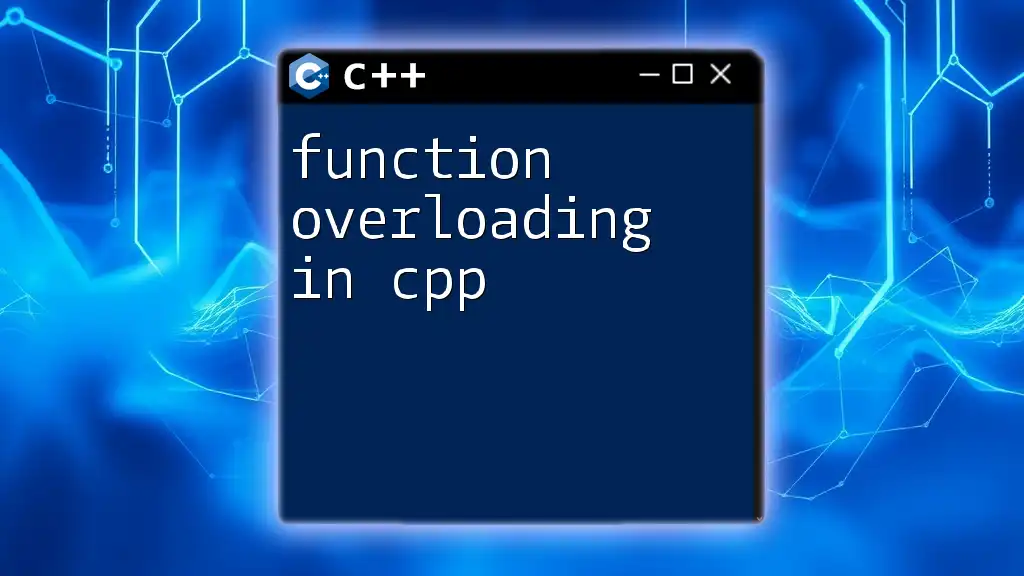
Best Practices for Overloading in C++
When to Overload Functions and Operators
When considering overloading functions or operators, ask yourself if it will add clarity or usability to your code. If two functions perform similar tasks but on different types or numbers of arguments, overloading can simplify the interface. However, avoid overloading when it might confuse future maintainers of your code.
How to Correctly Overload Functions and Operators
To effectively overload functions and operators:
- Maintain clear and consistent function signatures. An overload should be intuitively similar to the others; varying the parameters should feel natural.
- Document your overloads thoroughly, explaining the intended usage and any subtle nuances.
Common Mistakes to Avoid
Avoiding ambiguity is critical in function overloading—ensure your overloads are distinct enough to prevent confusion. Also, use operator overloading judiciously; overloaded operators can lead to unexpected behaviors if used carelessly or without proper context.
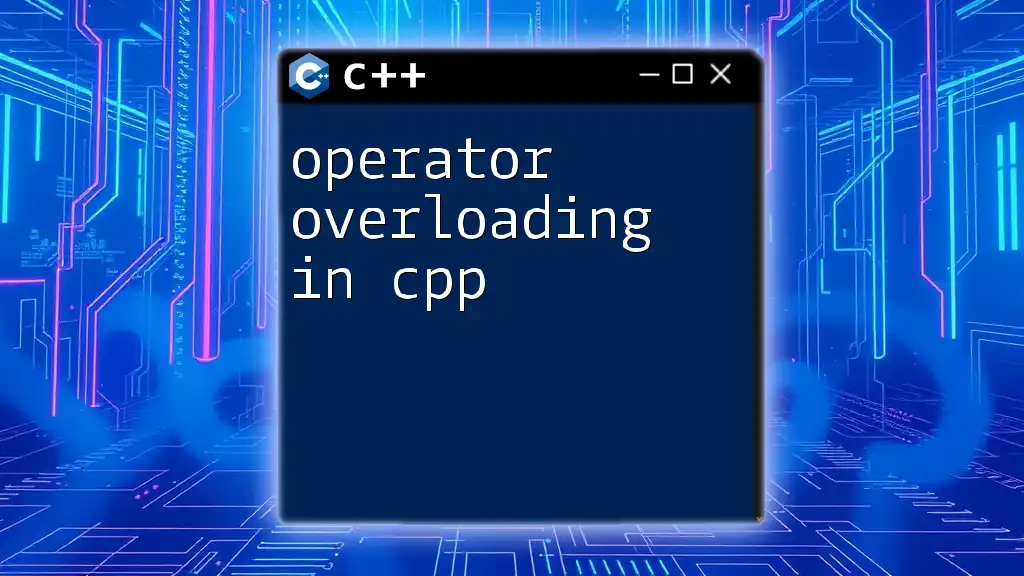
Summary of C++ Overloading
In conclusion, C++ overloading is a powerful feature that offers flexibility, clarity, and conciseness in coding. By allowing multiple forms of the same function or operator, it enhances the usability of custom types, making your code more intuitive and user-friendly.
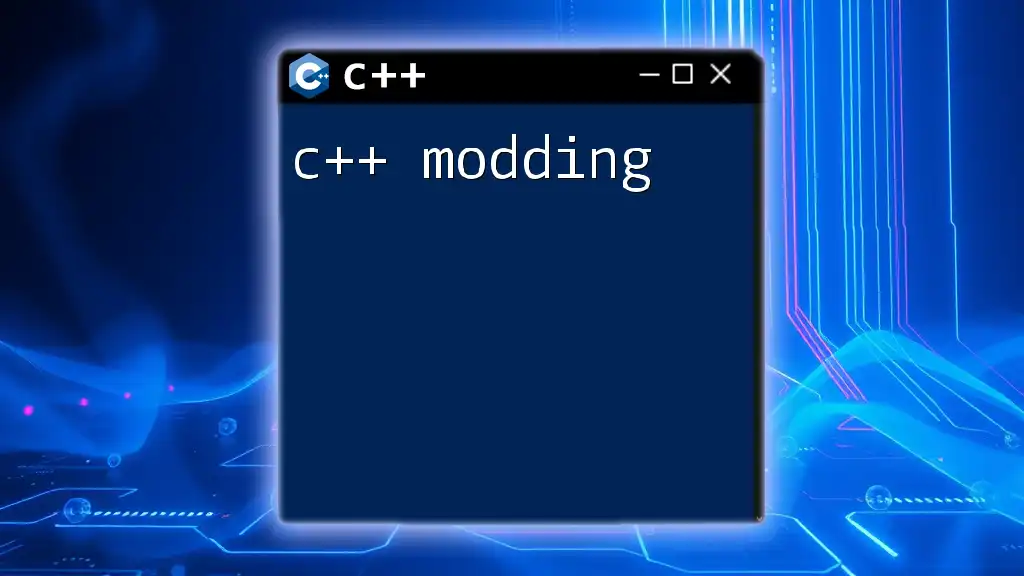
Further Learning Resources
For those looking to deepen their understanding of C++ overloading, several resources are available. Textbooks on C++ programming and comprehensive online tutorials can provide additional examples and exercises. Furthermore, the official C++ documentation offers insights into standards and best practices.
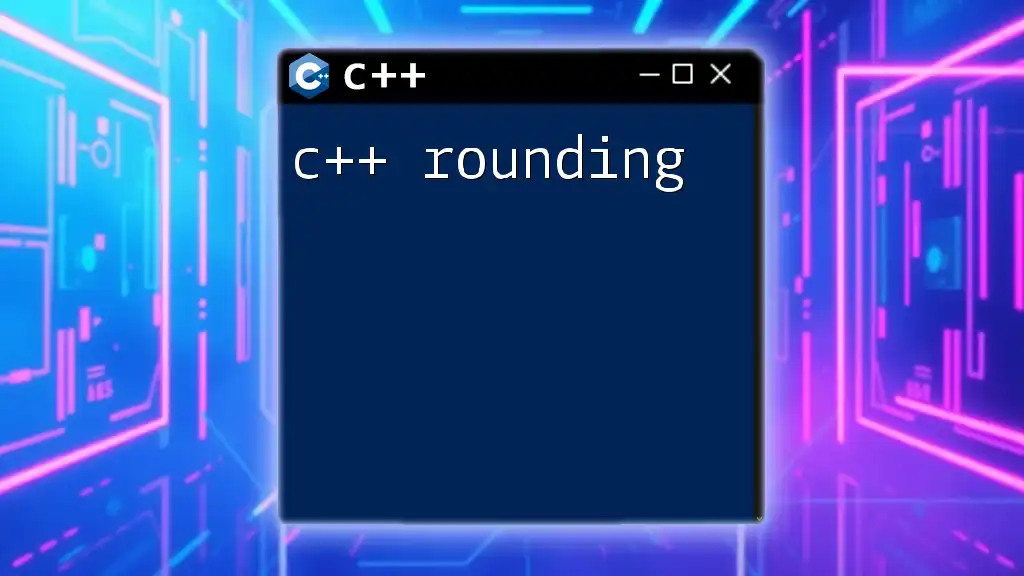
Call to Action
Try implementing function and operator overloading in your own projects. Experiment with creating custom classes and observe how overloading can streamline operations. Share your experiences or any questions in the comments section to foster discussion and learning within our community.