C++ floating point precision refers to the accuracy and range of representation of decimal numbers, which can be affected by the choice of data type (float, double, or long double) and the precision settings.
Here’s an example code snippet demonstrating the difference in precision between float and double:
#include <iostream>
#include <iomanip>
int main() {
float f = 1.123456789f; // single precision
double d = 1.123456789; // double precision
std::cout << std::setprecision(9) << "Float: " << f << std::endl; // Output: 1.123456
std::cout << std::setprecision(15) << "Double: " << d << std::endl; // Output: 1.123456789000000
return 0;
}
Understanding Floating Point Numbers
What are Floating Point Numbers?
Floating point numbers are designed to represent real numbers in a way that can accommodate a vast range of values. They consist of two parts: the magnitude (the number itself) and the exponent (which determines the scale). This allows for the representation of very large or tiny numbers, which is crucial in many applications such as scientific computations or graphics rendering.
In C++, floating point types can represent decimal numbers unlike integer types, which can hold only whole numbers. This ability makes floating point types essential for calculations that require precision in fractional values.
Floating Point Representation
In C++, floating point numbers follow the IEEE 754 standard, which specifies how these numbers are represented in binary. According to this standard, a floating point number is stored using three components:
- Sign bit: Indicates if the number is positive or negative.
- Exponent: Represents the scale of the number.
- Fraction (mantissa): Holds the significant digits of the number.
Understanding this representation is vital for appreciating both the capabilities and limitations surrounding c++ floating point precision.

Types of Floating Point in C++
C++ Floating Point Types
C++ provides several floating point types, each differing in precision and range:
- `float`: Represents single precision floating point numbers. It typically allocates 32 bits of memory, providing about 7 decimal digits of precision.
- `double`: Represents double precision floating point numbers. This type usually allocates 64 bits, yielding around 15 decimal digits of precision.
- `long double`: Represents extended precision floating point numbers, often offering a higher precision than `double`, depending on the system.
Here's a simple example of how to initialize these types:
float a = 1.23f; // Single precision
double b = 1.23; // Double precision
long double c = 1.23L; // Extended precision
Differences in Precision
C++ float precision varies significantly between types. The choice of floating point type impacts both performance and the ability to handle precision-critical operations. For example, in calculations involving very small or very large numbers, using `double` is often preferable due to its greater precision and wider range.
Here’s a practical example demonstrating the difference in precision:
#include <iostream>
#include <iomanip>
int main() {
float f = 1.0f / 3.0f;
double d = 1.0 / 3.0;
std::cout << std::setprecision(5) << f << std::endl; // Output: 0.33333
std::cout << std::setprecision(15) << d << std::endl; // Output: 0.333333333333333
return 0;
}
In this code, you can observe the precision difference when outputting the results of the same mathematical operation with different data types.

Floating Point Precision Issues
Common Problems with Floating Point Arithmetic
One of the main challenges in using floating point numbers is the potential for rounding errors. These errors occur because many decimal numbers cannot be precisely represented in binary format, resulting in small discrepancies.
Another common issue is cancellation errors, which can occur when subtracting two nearly equal floating point numbers, possibly leading to significant loss of precision.
Consider this example illustrating a rounding error:
#include <iostream>
int main() {
float a = 0.1f + 0.2f;
if (a == 0.3f) {
std::cout << "Equal!" << std::endl;
} else {
std::cout << "Not equal!" << std::endl; // Output: Not equal!
}
return 0;
}
In this case, due to rounding, the result of `0.1f + 0.2f` does not yield an exact representation of `0.3f`.
Precision Limitations
Every floating point type has its precision limits, which can introduce complications when performing arithmetic operations. Machine precision refers to the smallest difference between two representable floating-point numbers. This difference is often referred to as epsilon.
You can check how close two floating point numbers are to each other using the following code snippet:
#include <iostream>
#include <cmath>
bool nearlyEqual(float a, float b, float epsilon) {
return fabs(a - b) < epsilon;
}
int main() {
float f1 = 0.15f + 0.15f;
float f2 = 0.1f + 0.2f;
std::cout << nearlyEqual(f1, f2, 0.0001f) << std::endl; // Output: 1 (true)
return 0;
}
This approach can help manage precision and ensure that your calculations reflect the intended values accurately.
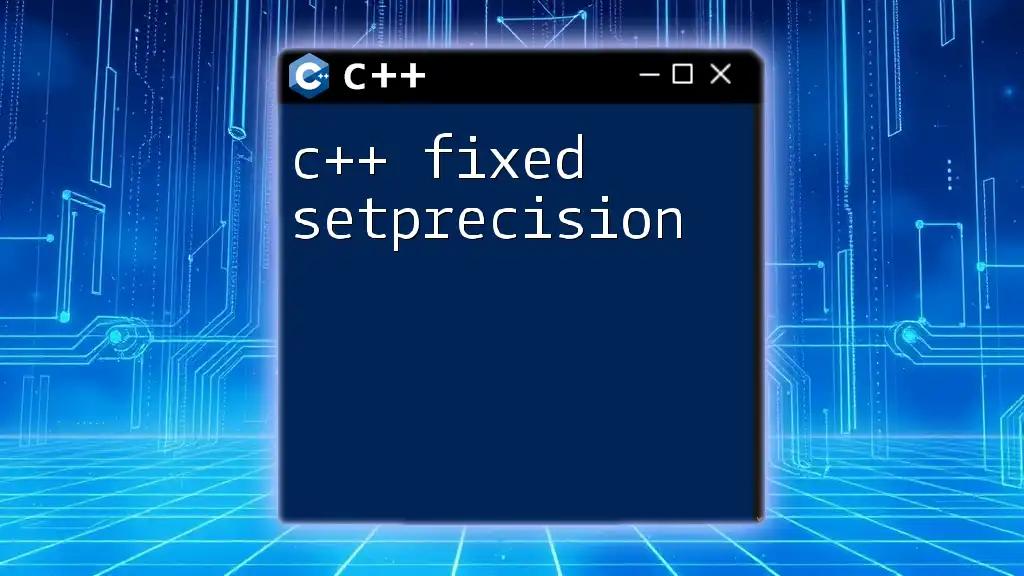
Best Practices for Handling Floating Point Precision in C++
Using Fixed Point Representation
In scenarios demanding high precision, such as financial calculations, a fixed-point representation can sometimes be more appropriate. This approach avoids the pitfalls of floating point arithmetic by representing numbers as integers scaled by a fixed factor (e.g., treating cents as whole numbers).
However, while fixed-point can eliminate certain types of errors, it introduces limitations in terms of range and may require more complex logic for arithmetic operations.
Effective Use of Data Types
When selecting a floating point type, consider the precision required for your application. The best practice is to always choose the highest precision type necessary to minimize rounding errors in important operations. It's essential to memorize the precision ranges of `float`, `double`, and `long double` to make informed decisions.
Here's a quick example calculating the percentage error:
#include <iostream>
#include <cmath>
float calculatePercentageError(float actual, float estimated) {
return fabs((actual - estimated) / actual) * 100.0f;
}
int main() {
float actual = 100.0f;
float estimated = 98.5f;
std::cout << "Percent Error: " << calculatePercentageError(actual, estimated) << "%" << std::endl;
return 0;
}
In this code, calculating the percentage error allows you to quantify how precise your estimated values are compared to actual values.
Utilizing Libraries for Higher Precision
For applications where precision is critical, consider using dedicated libraries like GMP (GNU Multiple Precision Arithmetic Library) or Boost's Multiprecision Library. These libraries offer specialized types and functions for engagement with multiple precision arithmetic, facilitating calculations that exceed the limitations of standard data types within C++.
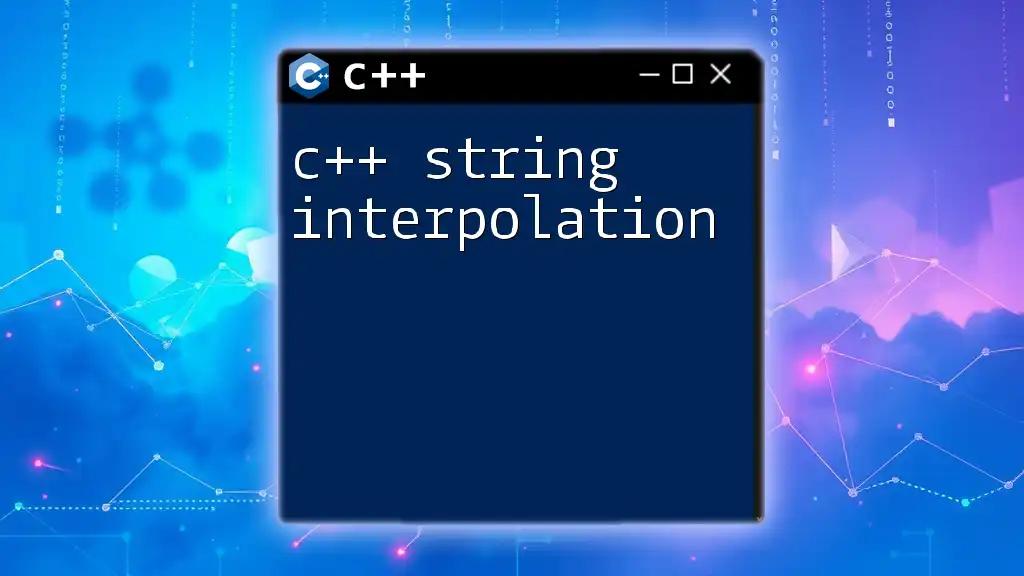
Conclusion
Understanding c++ floating point precision is crucial for developing reliable and robust applications. By recognizing the strengths and weaknesses of floating point types, leveraging best practices, and employing appropriate libraries, you can effectively manage precision and its associated challenges.
As you continue to dive deeper into C++, don’t forget to explore the mathematical foundations that govern floating point arithmetic. Empower yourself with knowledge, and enhance your programming skills!
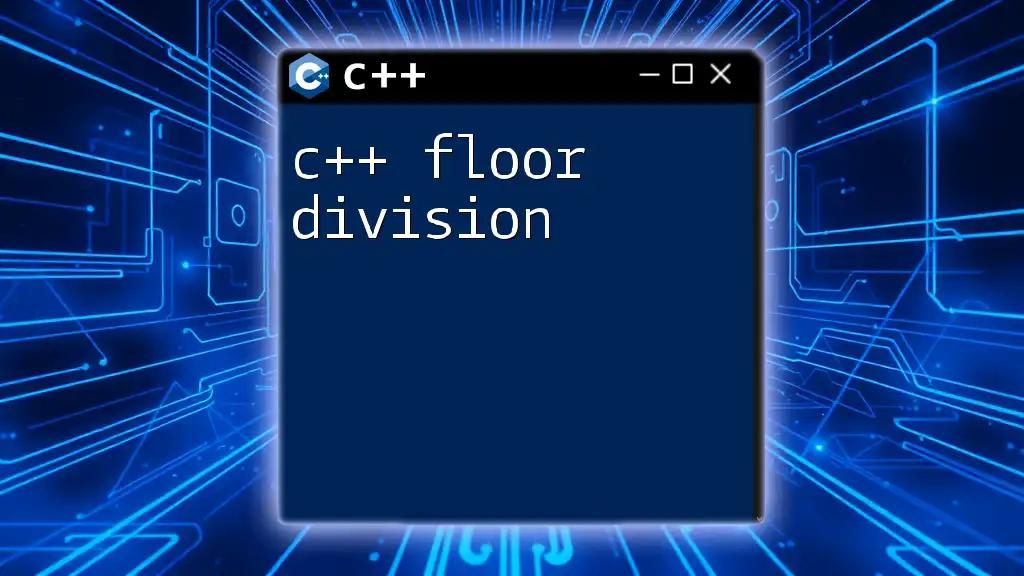
Further Reading and Resources
For those interested in expanding their knowledge on this topic further, consider exploring books on numerical methods or checking out online resources focusing on C++ and floating point mathematics. The C++ documentation and various programming communities can also offer valuable insights and discussions related to this essential aspect of programming.