C++ coding software encompasses a variety of development environments and tools that facilitate writing, compiling, and debugging C++ code efficiently.
Here’s a simple C++ code snippet as an example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++ Coding Software?
C++ coding software encompasses a variety of tools designed to assist in the development, testing, and management of C++ programs. These tools are essential for programmers, as they streamline the coding process, enhance productivity, and help prevent errors. By understanding the features and capabilities of different C++ coding software options, developers can choose the one that best fits their needs and workflow.
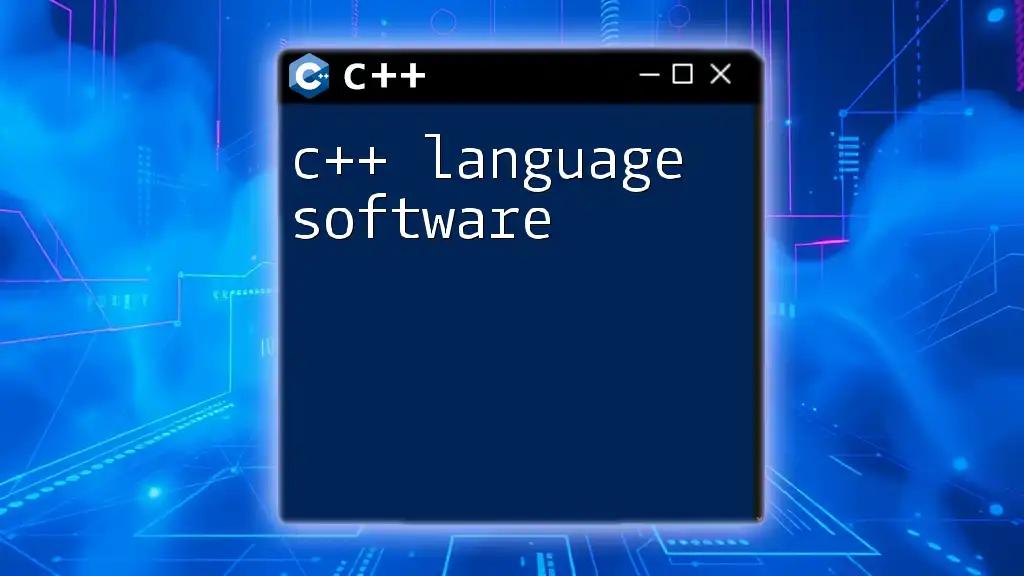
Types of C++ Coding Software
Integrated Development Environments (IDEs)
IDEs play a crucial role in C++ programming by providing a comprehensive suite of tools in a single application. They typically include a code editor, debugger, compiler, and build automation tools.
Popular C++ IDEs
Visual Studio
Visual Studio is a widely-used IDE that offers an extensive range of features tailored for C++ development.
- Features: Robust IntelliSense for code suggestions, real-time code analysis, powerful debugging tools, and project management options.
Example: Setting up a console application in Visual Studio is straightforward. After creating a new project via "File > New > Project," select "Console Application" and follow the prompts. You can then write basic C++ code in the editor:
#include <iostream>
using namespace std;
int main() {
cout << "Hello World" << endl;
return 0;
}
Code::Blocks
Code::Blocks is another popular IDE known for its flexibility and multi-platform support.
- Features: A customizable interface allowing developers to tailor their environment, as well as support for various compilers.
Example: To create a simple "Hello World" application, open Code::Blocks, create a new project, choose "Console Application," and input the same C++ code shared above.
CLion
CLion, developed by JetBrains, focuses heavily on smart code assistance and development efficiency.
- Features: Advanced smart code completion, an intelligent code navigation system, and a powerful refactoring utility.
Example: With CLion, you can easily refactor your code by selecting the variable or function name, right-clicking, and choosing the refactor option.
Code Editors
While IDEs offer a comprehensive toolset, code editors provide a more streamlined approach with fewer features, making them lighter and often faster to load.
Famous Code Editors for C++
Sublime Text
Sublime Text is a popular code editor known for its speed and simplicity.
- Features: Syntax highlighting, multiple selections for quicker edits, and a distraction-free writing mode.
Example: You can create code snippets in Sublime by using its snippet feature, allowing you to save time on repetitive tasks.
Visual Studio Code
Visual Studio Code (VS Code) has gained immense popularity due to its flexibility and wide range of extensions.
- Features: An integrated terminal, support for extensions, version control integration, and real-time collaboration.
Example: By using the C++ extension for VS Code, you can easily debug your code. Simply install the extension, open your .cpp file, and set breakpoints. You can start the debugging session with a simple command.
Command-Line Tools
Command-line tools are a powerful alternative for those who prefer a minimalistic approach to C++ development.
Examples of Command-Line Tools
GCC (GNU Compiler Collection)
GCC is a versatile compiler that supports a variety of programming languages, including C++.
- Features: Highly portable with support for numerous languages, but primarily known for its strong support for C and C++.
Example: You can compile and run a simple C++ program using GCC with the following commands:
g++ hello.cpp -o hello
./hello
Clang
Clang offers fast compilation and excellent diagnostics, making it a strong choice for C++ programmers.
- Features: Supports modern C++ standards and facilitates productive code analysis.
Example: To utilize Clang, compile your code similarly to GCC, adjusting the command to use `clang++`:
clang++ hello.cpp -o hello
./hello
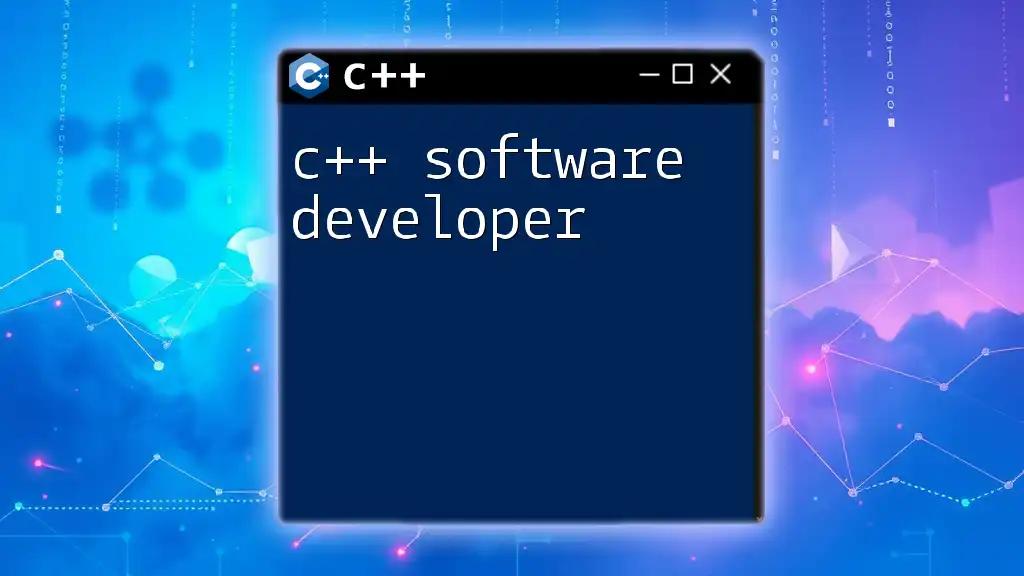
Features to Look for in C++ Coding Software
User Interface
An intuitive user interface is vital for enhancing productivity. A well-designed UI can reduce the learning curve and improve the coding experience. For instance, Visual Studio’s clean layout and shortcut capabilities allow developers to navigate their projects seamlessly.
Debugging and Error Handling
Robust debugging tools are essential for efficiently identifying and resolving issues in your code. IDEs like Code::Blocks and Visual Studio provide graphical debugging options, allowing you to step through code, inspect variables, and set breakpoints, making error handling much easier.
Example: In Visual Studio, you can start debugging by selecting "Debug > Start Debugging," which allows you to view your code’s flow in real time and address any issues as they arise.
Performance Optimization Tools
Performance optimization is crucial, especially for C++ applications that require efficiency. Many IDEs integrate profiling tools that analyze how your program uses resources.
Example: Visual Studio includes a performance profiler that helps identify bottlenecks in your code, allowing you to optimize functions or memory usage for better performance.
Cross-Platform Compatibility
When developing C++ applications, cross-platform compatibility allows you to build software that runs on different operating systems. IDEs and code editors that support multiple platforms, like Code::Blocks and CLion, enable developers to write code once and deploy it across various environments seamlessly.
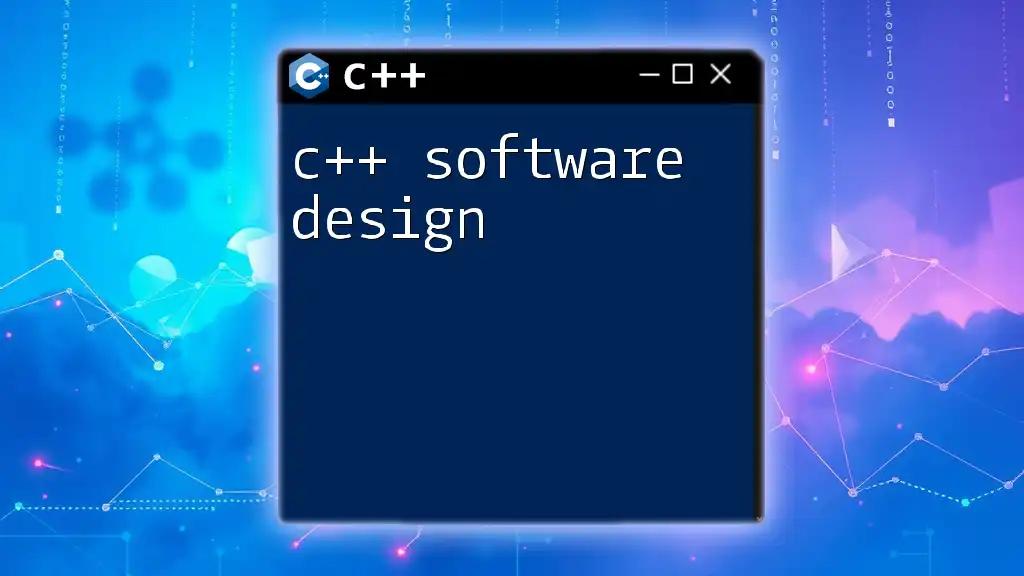
Setting Up Your C++ Coding Environment
Downloading and Installing Software
To get started with C++ coding, first, download and install your chosen software. Most IDEs have straightforward installation processes, which you can find on their respective websites.
Configuring the Environment
Once installed, configuring your environment can significantly improve your workflow. This may involve setting environment variables or configuring paths for compilers.
Example: In Visual Studio, you can set the path for GCC by navigating to "Tools > Options > Projects and Solutions > VC++ Directories," ensuring your programming tools are easily accessible.
Creating Your First C++ Project
Creating your first C++ project is an exciting step into programming. In Visual Studio, for instance, you start by choosing "File > New > Project," then selecting the type of project you want.
Example: By following the steps highlighted earlier in the IDE section, you can write a function similar to a basic calculator that accepts inputs and performs operations.
#include <iostream>
using namespace std;
int main() {
double num1, num2, result;
char op;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter an operator (+, -, *, /): ";
cin >> op;
cout << "Enter second number: ";
cin >> num2;
switch (op) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
default:
cout << "Invalid operator!";
return 1;
}
cout << "Result: " << result << endl;
return 0;
}
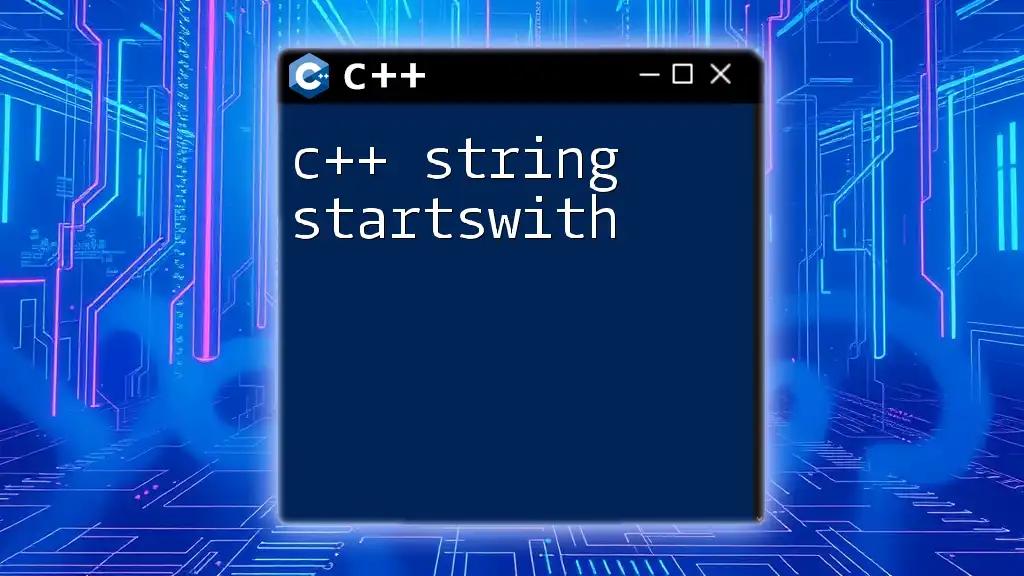
Best Practices for Using C++ Coding Software
Organizing Your Code
Keeping your code organized is fundamental to successful development. Use consistent naming conventions and comments to clarify functions and processes within your code.
Example: An organized project folder structure with separate directories for headers and implementation files can make it easier to navigate and maintain your C++ applications.
Version Control Integration
Utilizing version control (like Git) alongside your C++ coding software allows you to track changes, collaborate with others, and manage project versions efficiently.
Example: Most IDEs, including Visual Studio Code, allow you to integrate Git easily, letting you commit changes, create branches, and resolve conflicts directly from the interface.
Testing and Code Review
Regularly testing your code and conducting peer reviews enhances software quality. Many IDEs have built-in tools for unit testing and code evaluation that aid in identifying issues early in the development process.
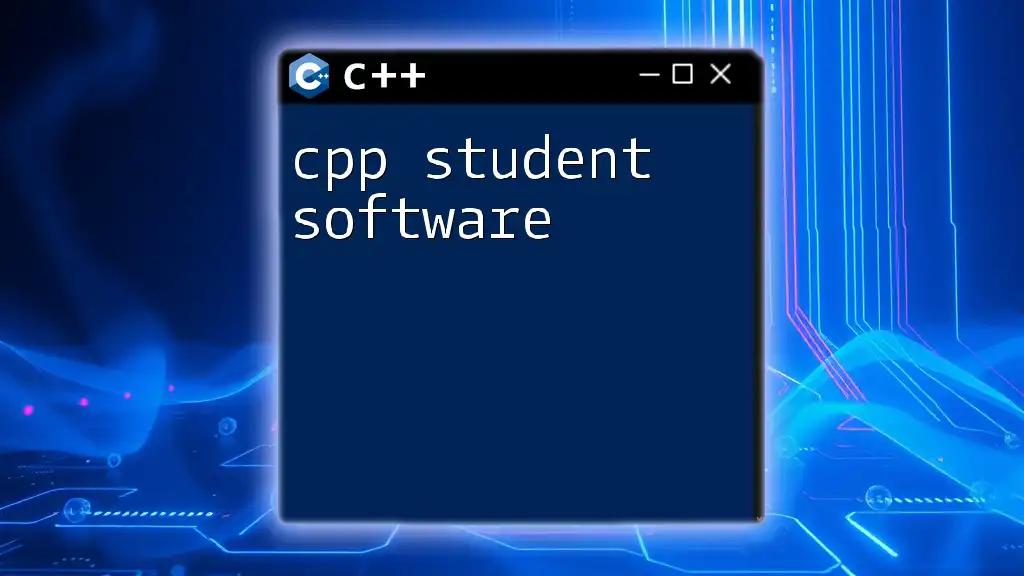
Conclusion
In conclusion, selecting the right C++ coding software is pivotal in shaping your programming experience. Understanding the features, tools, and options available can help developers make informed decisions that enhance their productivity and coding skills. Experimenting with various software allows you to find the tools that best support your programming style and project requirements.
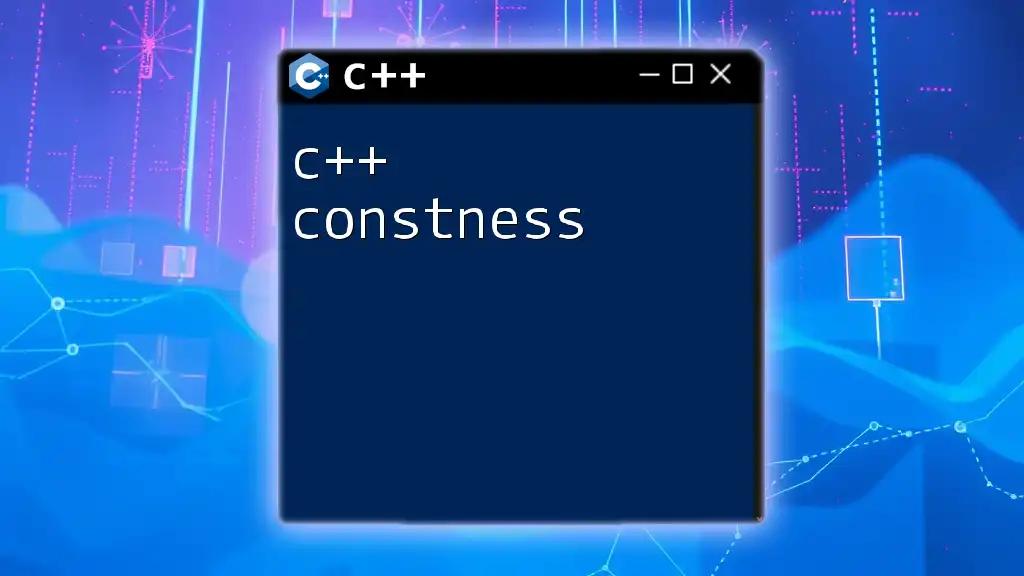
Additional Resources
For further learning and exploration, consider checking out official documentation, tutorials, and forums dedicated to C++ development. Staying connected with the C++ community can provide valuable insights and foster continued growth in your programming journey.