C++ language software encompasses programs developed using the C++ programming language, which is known for its efficiency and performance in system-level and application-level development.
Here's a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
History of C++
C++ was developed in the early 1980s by Bjarne Stroustrup at Bell Labs as an extension of the C programming language. It incorporated features of object-oriented programming, which was gaining popularity at the time. C++ has evolved through several versions, introducing new standards that include modern language features while maintaining backward compatibility with C. Key milestones include the 1998 C++98 standard and the more recent C++11 and C++20 standards, each bringing significant enhancements to the language.
Key Features of C++
C++ is renowned for its versatility and performance, boasting several key features that make it a preferred choice among developers:
- Object-oriented programming (OOP): This allows for data encapsulation, inheritance, and polymorphism, enabling code reuse and organization.
- Low-level memory manipulation: Developers can interact directly with hardware, making it efficient for system-level programming.
- Standard Template Library (STL): This powerful library provides a collection of templates for algorithms and data structures, enhancing productivity and code quality.
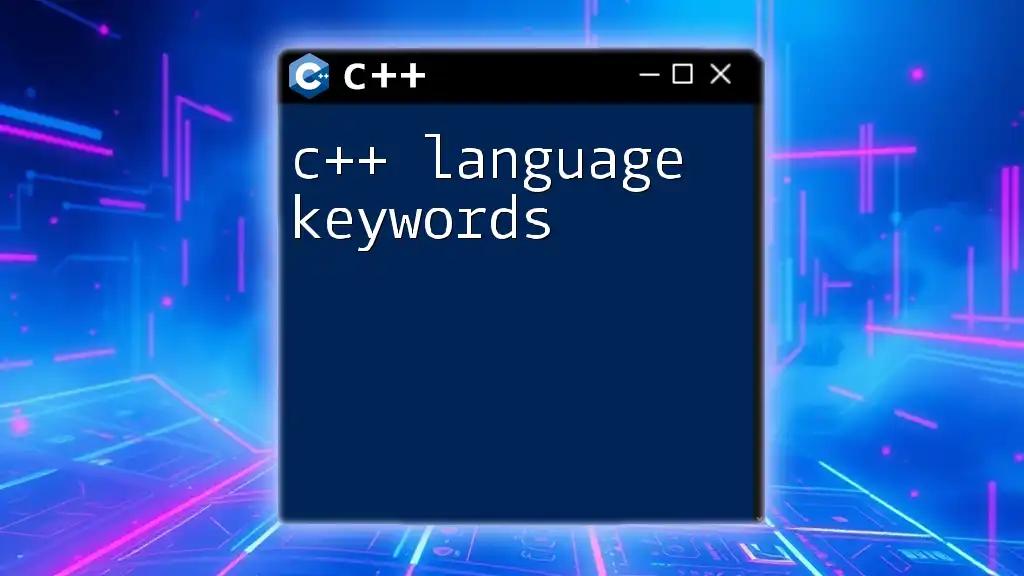
C++ Development Environment
Setting Up the Development Environment
To begin programming in C++, you need to set up a suitable environment. Popular Integrated Development Environments (IDEs) include:
- Visual Studio: A comprehensive IDE for Windows that offers powerful debugging tools.
- Code::Blocks: A free, cross-platform IDE that supports multiple compilers.
- CLion: A commercial IDE from JetBrains that provides excellent libraries for C++.
You will also need a C++ compiler to convert your code into executable programs. Common choices are GCC (GNU Compiler Collection) and Clang.
Creating Your First C++ Program
Let us dive into creating your very first C++ program with a simple "Hello World" example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, `#include <iostream>` is a preprocessor directive that includes the Input/Output stream library, allowing us to output text. The `main()` function serves as the entry point of any C++ program. The line `std::cout << "Hello, World!"` prints the output to the console, concluding with `return 0;`, which indicates success.
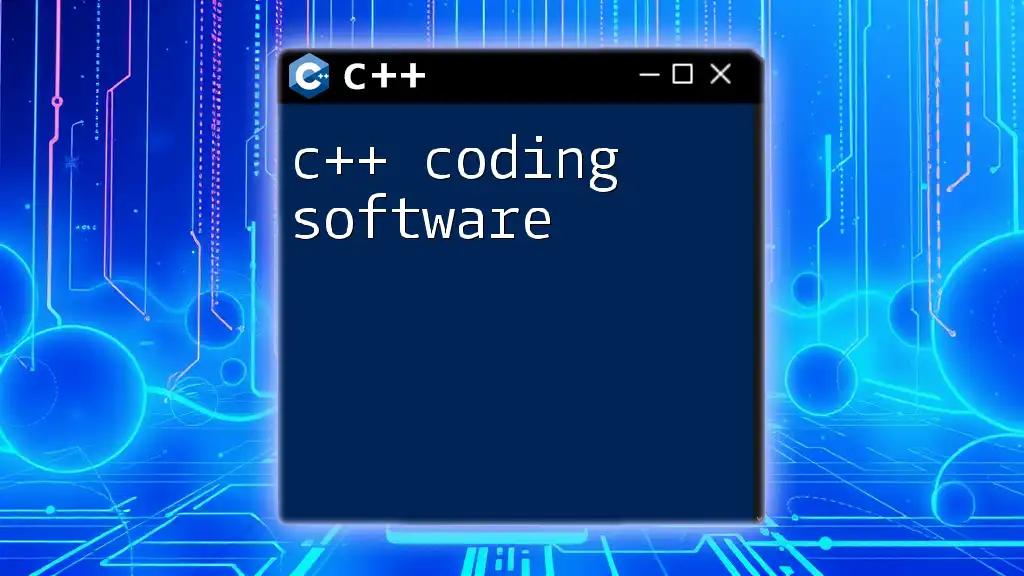
Core C++ Concepts
Basic Syntax and Structure
Understanding the basic syntax of C++ is crucial. The language is case-sensitive, and statements end with a semicolon. Curly braces `{}` are used to define the beginning and end of functions, classes, and conditional blocks.
Data Types and Variables
C++ supports a variety of built-in data types including:
- Integers: e.g., `int age = 30;`
- Floating-point numbers: e.g., `float salary = 50000.50;`
- Characters: e.g., `char initial = 'A';`
- Booleans: e.g., `bool isAdult = true;`
Control Structures
Conditional Statements
Implementing logic in code often requires conditional statements. The following example demonstrates usage of `if`, `else if`, and `else`:
if (age > 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
Here, the condition `age > 18` checks whether the person is an adult or a minor.
Loops
Loops facilitate code repetition. Understanding loops can optimize the performance of your program. Here's an example of a `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
This loop iterates five times, printing the numbers 0 through 4.
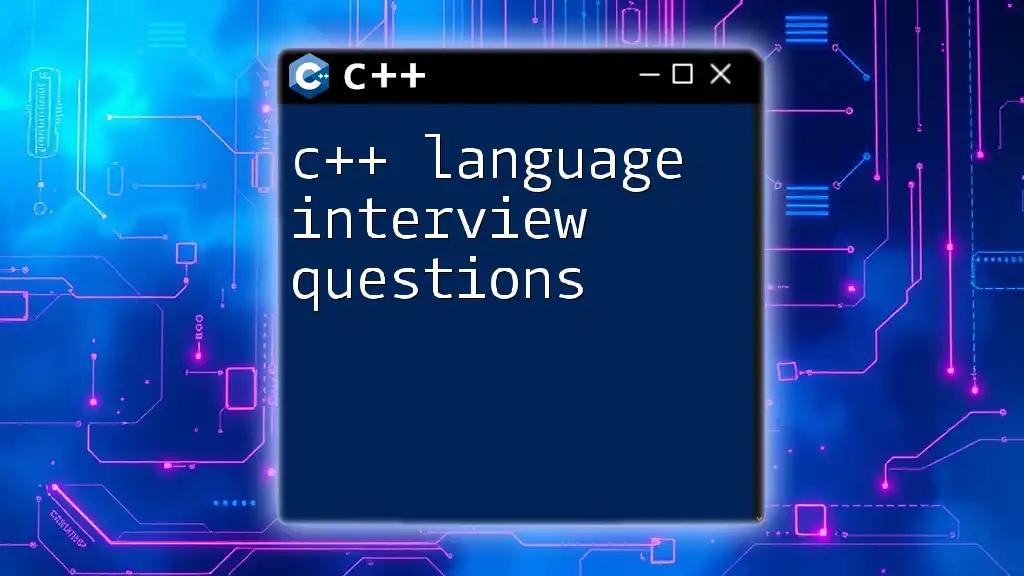
Functions in C++
What are Functions?
Functions are fundamental in C++ as they allow for code modularization and reusability. A typical syntax for declaring a function is as follows:
return_type function_name(parameters) {
// function body
}
Example of a Simple Function
Let’s look at a straightforward function that adds two integers:
int add(int a, int b) {
return a + b;
}
This example defines a function named `add`, which takes two integer parameters and returns their sum. Understanding the concept of parameters and return types aids in effective function usage.
Function Overloading
C++ enables function overloading, allowing multiple functions with the same name but different parameter types or counts. This feature enhances flexibility in function usage.
int add(int a, int b);
double add(double a, double b);
Each function performs addition, but the approach changes based on the data types of the parameters.
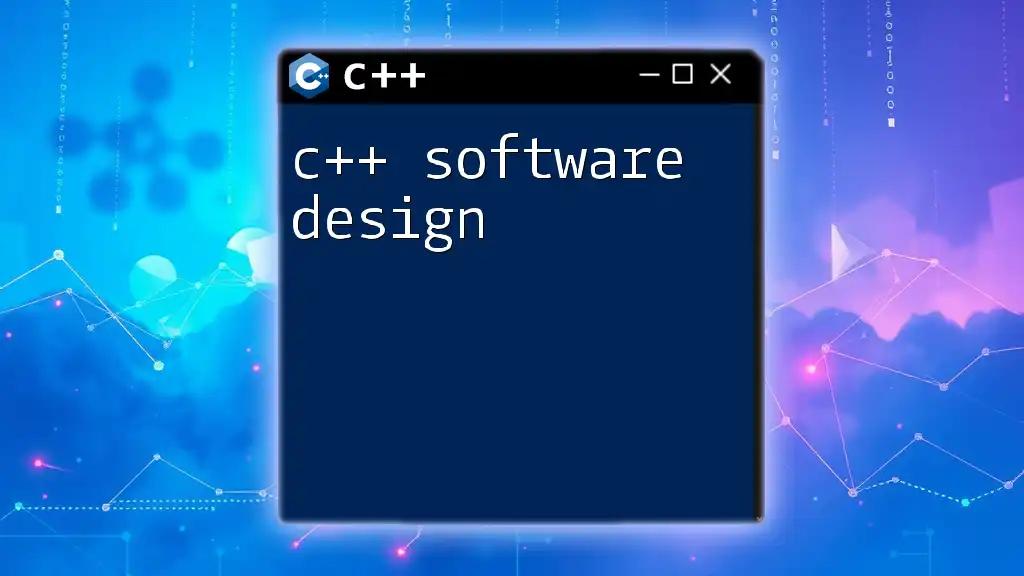
Object-Oriented Programming in C++
Classes and Objects
C++ embodies the principles of Object-Oriented Programming (OOP) through the use of classes and objects. Here’s an example of how to define a class and create an object:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this example, `Dog` is a class containing a public method `bark()` that outputs a barking sound. Understanding public vs. private access specifiers is essential for encapsulation.
Inheritance
Inheritance allows one class to inherit attributes and methods from another, fostering code reuse. Here’s a simple demonstration:
class Animal {
public:
void eat() {
std::cout << "Eating..." << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
The `Dog` class inherits from the `Animal` class, gaining access to its methods while also defining new behaviors.
Polymorphism
Polymorphism enables you to invoke methods of a derived class through a base class reference. This can be achieved through virtual functions. Consider the following example:
class Animal {
public:
virtual void sound() { }
};
class Cat : public Animal {
public:
void sound() override { std::cout << "Meow!" << std::endl; }
};
By declaring `sound()` as virtual in the base class, you allow derived classes to override it, achieving runtime polymorphism.
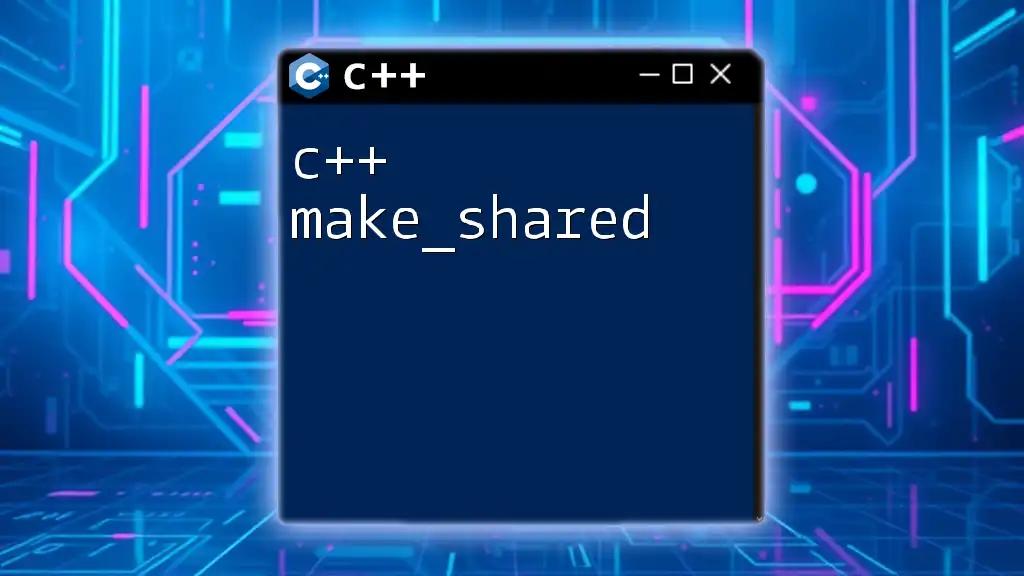
C++ Standard Library
Introduction to STL (Standard Template Library)
The Standard Template Library (STL) is a powerful feature of C++, which provides a rich set of functions and data structures that allow you to perform common programming tasks efficiently. It enhances productivity through ready-to-use templates.
Commonly Used Containers
Vectors and Lists
STL includes various container types, with vectors and lists being the most utilized. Vectors allow dynamic array functionality while lists are useful for linked data structures.
Here’s how to define a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
Vectors are versatile and manage their memory automatically, making them ideal for use when the size of the dataset is uncertain.
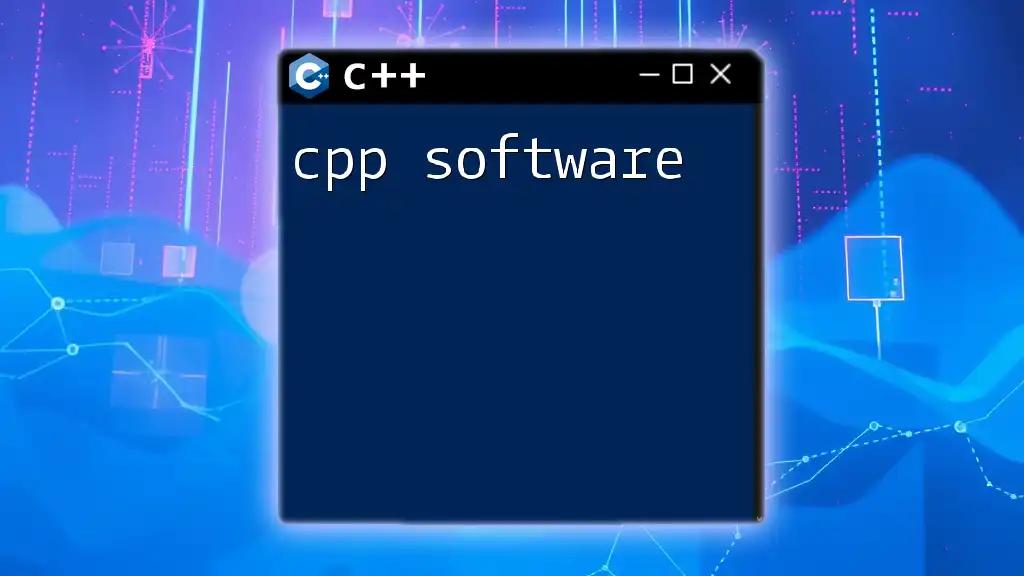
Best Practices in C++ Programming
Writing Clean Code
Creating clean and maintainable code should be your priority as a developer. Use meaningful variable names, comment your code effectively, and adhere to consistent indentation. This maximizes readability and long-term maintainability.
Error Handling
C++ employs exceptions to handle errors, a robust mechanism for managing runtime issues. Use try, catch, and throw blocks to manage potential failures efficiently:
try {
throw std::runtime_error("Error occurred");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
This code catches exceptions and provides meaningful output, preventing unexpected program termination.
Memory Management
Managing memory correctly is crucial in C++. Smart pointers such as `unique_ptr` and `shared_ptr` help automate memory management and prevent memory leaks:
#include <memory>
std::unique_ptr<int> p(new int(5));
Here, `unique_ptr` automatically deallocates memory when it goes out of scope, simplifying memory management.
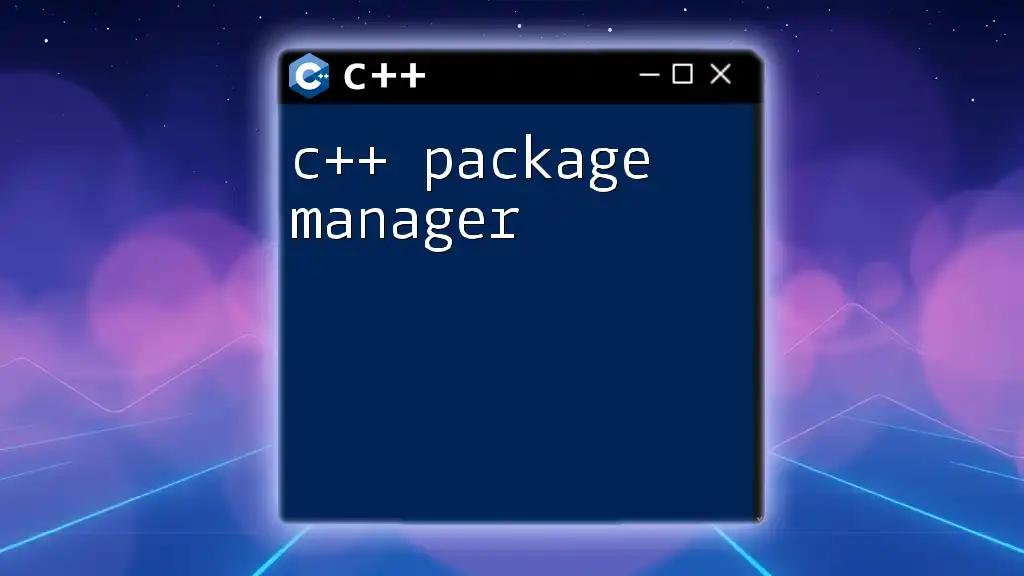
Conclusion
C++ is a rich and powerful language, offering a wealth of features that aid developers in crafting high-performance software applications. By grasping its core concepts and principles such as OOP, STL, and effective memory management, you can harness the full potential of C++ language software development. The journey into C++ can be enriching, and your practice will lead you toward mastery.
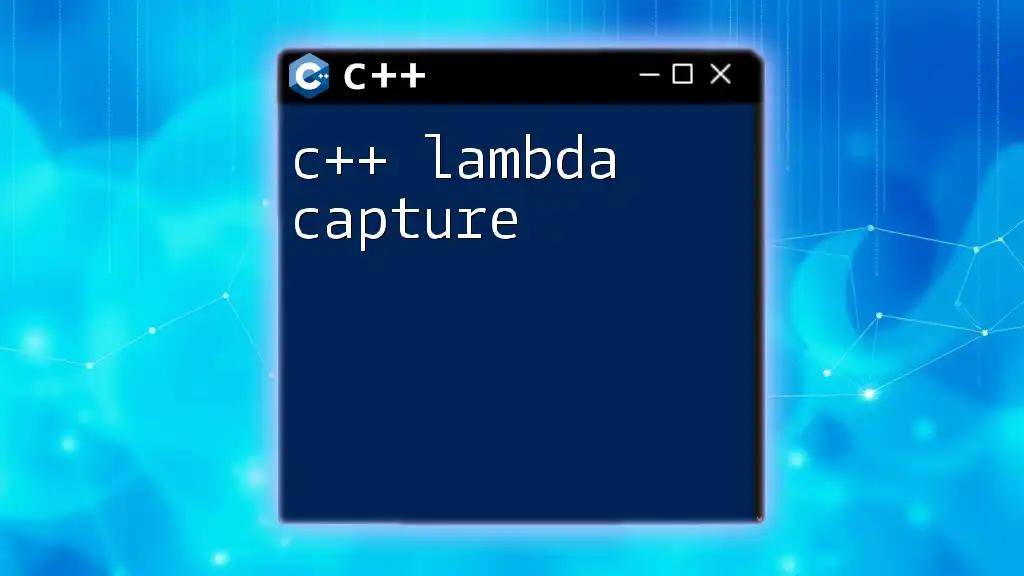
Additional Resources
For those eager to dive deeper into the world of C++, beneficial resources are readily available. Recommended books, engaging online courses, and vibrant community forums await you and will provide the guidance necessary to enhance your understanding and skills.
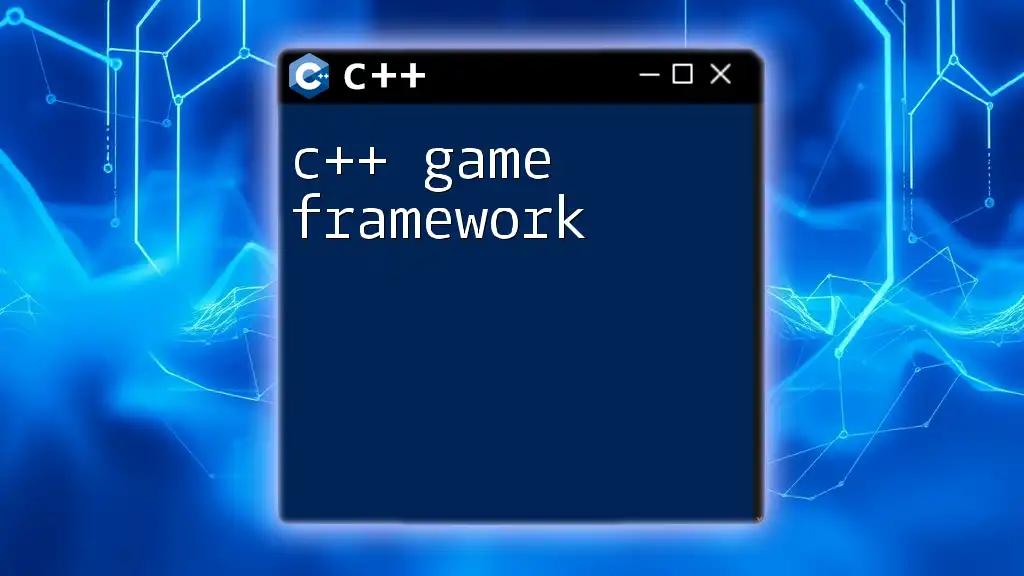
Call to Action
We encourage you to share your thoughts on this guide and join our workshops to improve your C++ skills. The journey to mastering C++ starts with a single program. Let’s take that journey together!