C++ (or cpp) software refers to programs developed using the C++ programming language, which is known for its performance and versatility in various applications.
Here's a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a powerful general-purpose programming language created by Bjarne Stroustrup, first released in 1985. It built upon the foundation laid by its predecessor, C, while introducing object-oriented programming (OOP) features. The significance of C++ in software development lies in its versatility, efficiency, and performance. The language is widely used in various applications, including:
- Game development
- System/software development
- Embedded systems
- Real-time simulations
These applications highlight the language’s capability to handle tasks ranging from low-level programming to complex graphical applications.
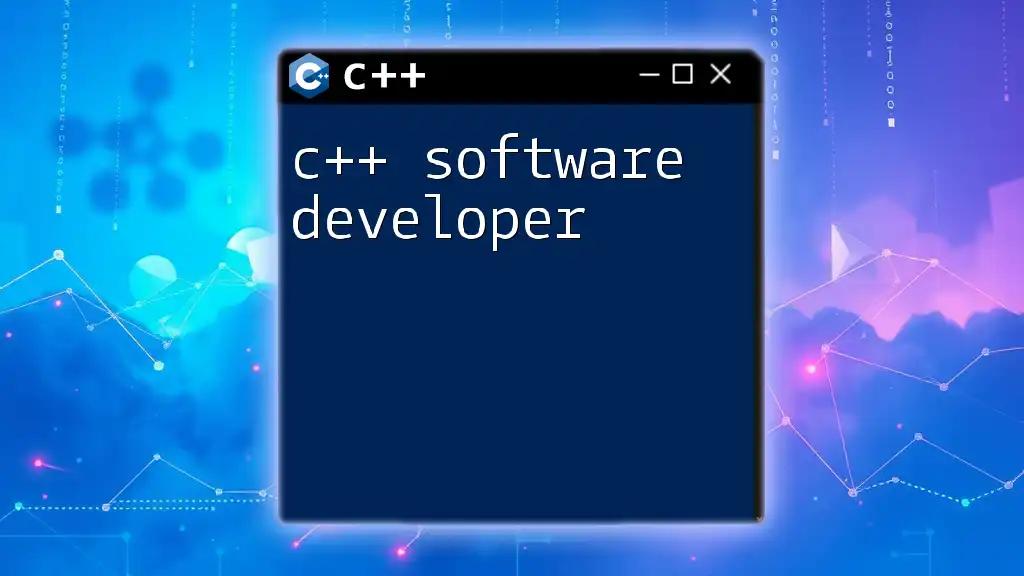
Key Features of C++
Object-Oriented Programming (OOP)
One of the core features of C++ is its support for object-oriented programming. OOP is centered around four key principles:
-
Encapsulation: This binds together data and functions that manipulate the data, restricting direct access to some of the object's components. An example would be a class that encapsulates user data and methods to manipulate that data.
-
Inheritance: C++ allows classes to inherit traits from other classes. This promotes code reusability and establishes a hierarchical relationship between classes. For example:
class Animal { public: void eat() { cout << "Eating..." << endl; } }; class Dog : public Animal { public: void bark() { cout << "Barking..." << endl; } };
-
Polymorphism: C++ supports polymorphism, allowing methods to have the same name while functioning differently based on their object type. This flexibility can be demonstrated with function overloading and virtual functions.
Performance and Efficiency
C++ is known for its high performance and resource efficiency, making it an ideal choice for software where speed is paramount. Unlike some interpreted languages, C++ is compiled into machine language, allowing for faster execution. Applications like game engines, which require real-time performance, heavily rely on this feature.
For example, C++ is used in game development to handle graphics and processing better than languages like Java or Python, which introduce overhead.
Portability
C++ offers a robust mechanism for writing portable code. Through the use of standard libraries and adherence to language standards, software developed in C++ can run on various platforms without major modifications. Cross-platform development is made considerably easier through tools like CMake and the wide availability of compilers on different operating systems.
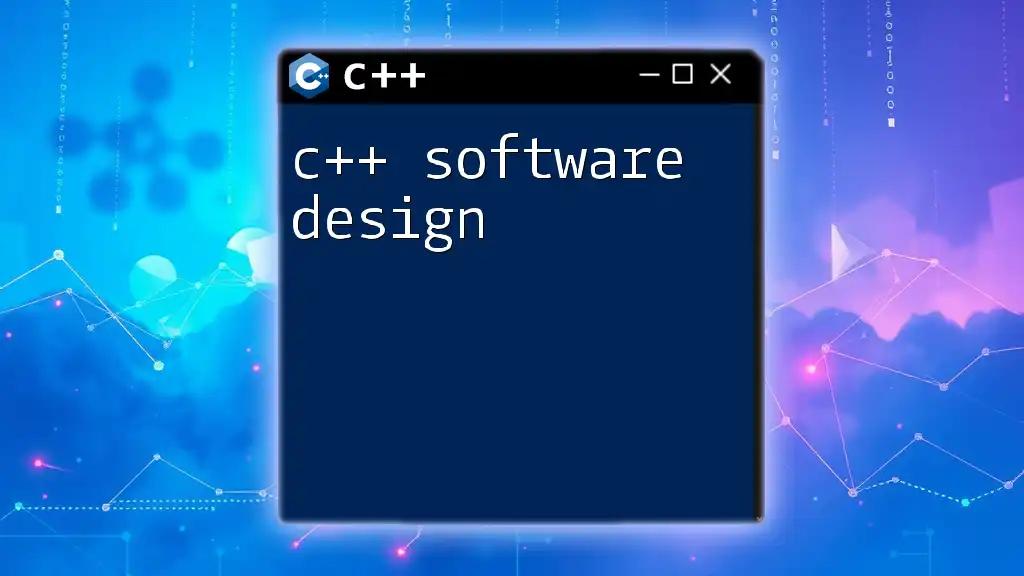
Basics of C++ Commands
Understanding the C++ Syntax
C++ syntax can be intimidating at first glance, but it follows a logical structure. A basic C++ program typically consists of the following components:
- Headers
- Namespace definitions
- Main function
- Statements
Here’s a simple example of a "Hello, World!" application:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This concise program introduces core concepts such as libraries, the main function, and output.
Datatypes and Variables
C++ has rich data type support which includes integers, floating-point numbers, characters, and strings. Fundamental types include:
- `int`: For integer values
- `float`: For single-precision floating point
- `double`: For double-precision floating point
- `char`: For single characters
- `string`: For collections of characters
Declaring and initializing variables is straightforward:
int age = 25;
float salary = 3500.50;
char initial = 'A';
Control Structures
Control structures are essential for decision-making and looping in programming. The main types include:
- Conditional Statements (if, else)
- Loops (for, while)
This example illustrates a for loop in action:
for (int i = 0; i < 5; i++) {
cout << "Number: " << i << endl;
}
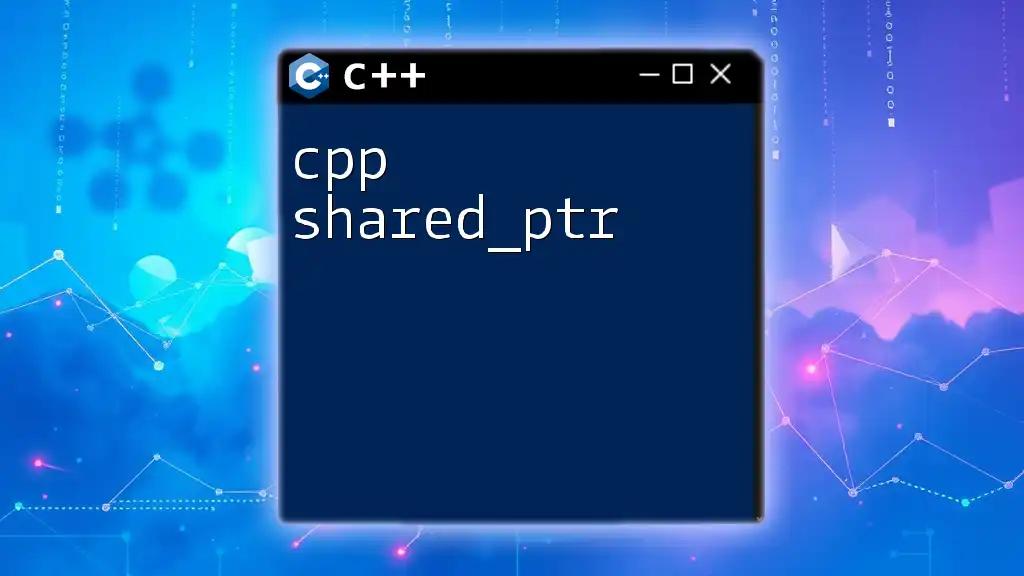
Advanced C++ Concepts
Pointers and Memory Management
C++ provides direct control over memory via pointers, which can point to variables and dynamically allocate memory. This capability is crucial for performance-sensitive applications, but it demands careful management to avoid memory leaks and corruption.
To illustrate dynamic memory allocation:
int* ptr = new int; // dynamically allocate an integer
*ptr = 100; // assign value
delete ptr; // free allocated memory
Standard Template Library (STL)
The Standard Template Library (STL) in C++ offers a powerful and flexible set of template classes for handling data structures and algorithms. STL improves productivity and code quality by providing pre-written templates for:
- Containers: Include `vector`, `list`, `map`, etc.
- Algorithms: Built-in functions for searching, sorting, etc.
A brief example of using a vector:
#include <vector>
using namespace std;
vector<int> numbers = {1, 2, 3, 4, 5};
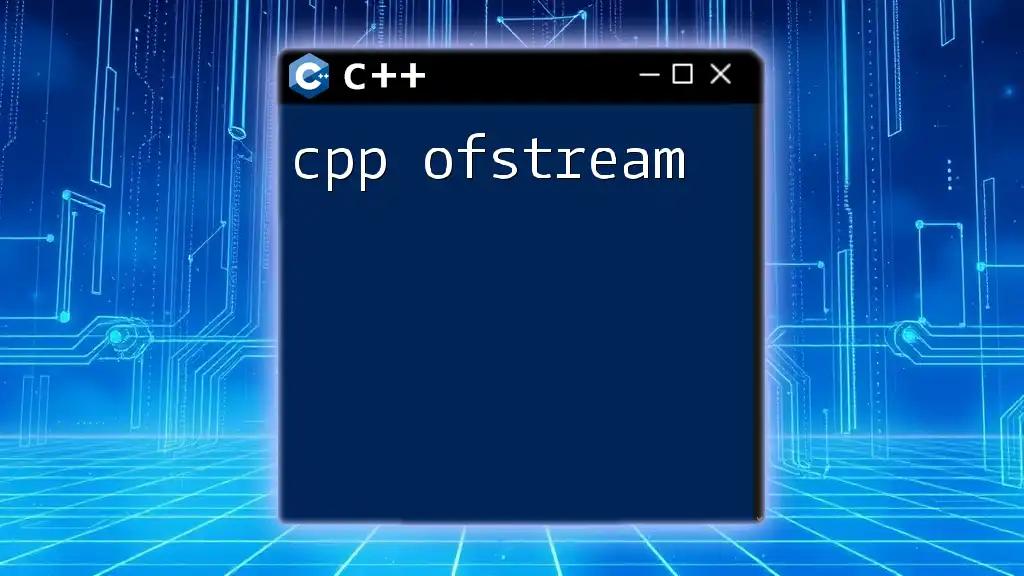
Best Practices for C++ Software Development
Code Readability and Organization
Writing clean and maintainable code is crucial in software development. Adopting consistent naming conventions, utilizing comments effectively, and organizing projects into directories can greatly enhance code readability. Consider these tips for organizational clarity:
- Group related functions and classes
- Use meaningful variable names
- Implement a consistent commenting style
Error Handling
C++ provides mechanisms for handling errors through exceptions. Proper error handling is essential for building robust software that can gracefully manage unexpected situations.
A simple try-catch example illustrates this:
try {
// Code that may throw an exception
throw runtime_error("An error occurred!");
} catch (const exception& e) {
cout << "Error: " << e.what() << endl;
}
Testing and Debugging
Testing and debugging are crucial steps for ensuring software quality. Unit testing frameworks like Google Test can aid in verifying that each module performs as expected. Effective debugging can be achieved through tools such as GDB or IDE-integrated debuggers.
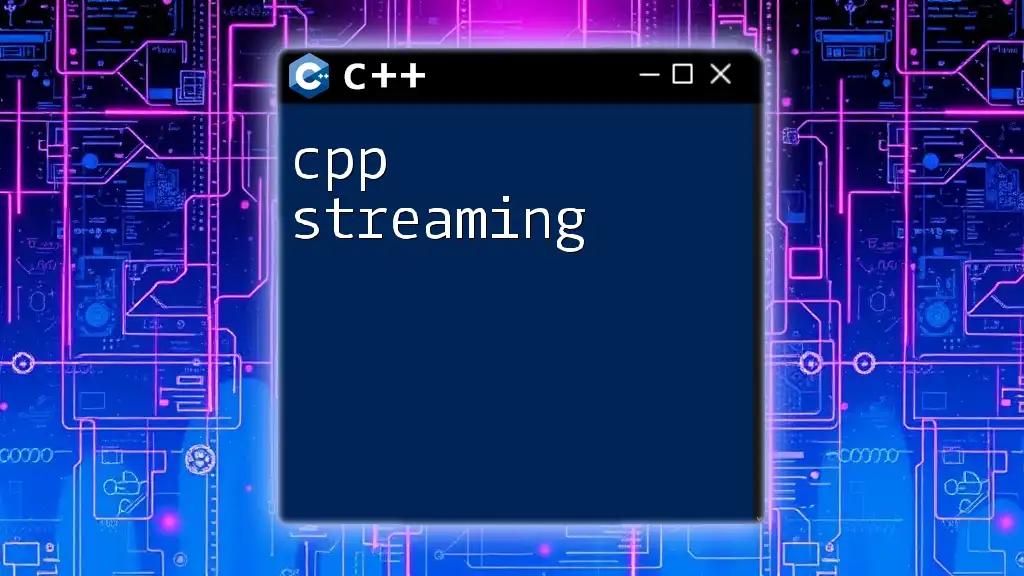
Real-World Applications of C++ Software
Game Development
C++ has found its niche in the gaming industry, particularly in high-performance game engines such as Unreal Engine. The ability to manipulate hardware resources directly makes it ideal for rendering graphics and processing game logic in real time.
System Software
Operating systems such as Windows and Linux rely on C++ for performance-critical components. Its low-level capabilities and direct memory management allow for efficient hardware interaction.
Embedded Systems
In the realm of IoT and embedded programming, C++ is preferred due to its efficiency in low-resource environments. It grants developers the ability to write code that maximizes performance while minimizing resource consumption.
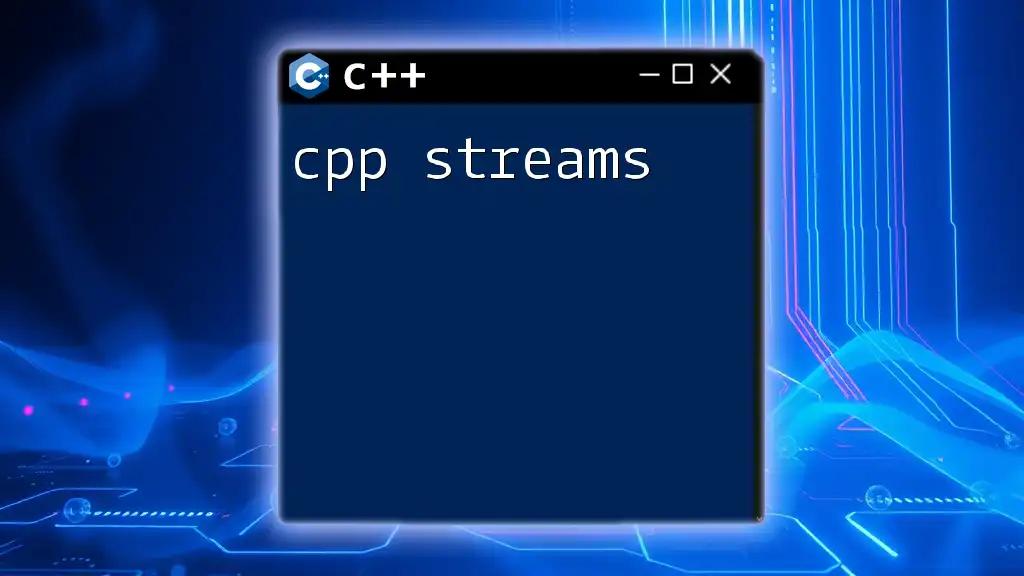
Conclusion
In summary, C++ software embodies a powerful and versatile programming landscape with its object-oriented principles, efficiency, and rich ecosystem of tools and libraries. For newcomers and seasoned developers alike, mastering C++ commands will unlock doors to developing a wide array of applications, from high-performance games to cutting-edge technological innovations.
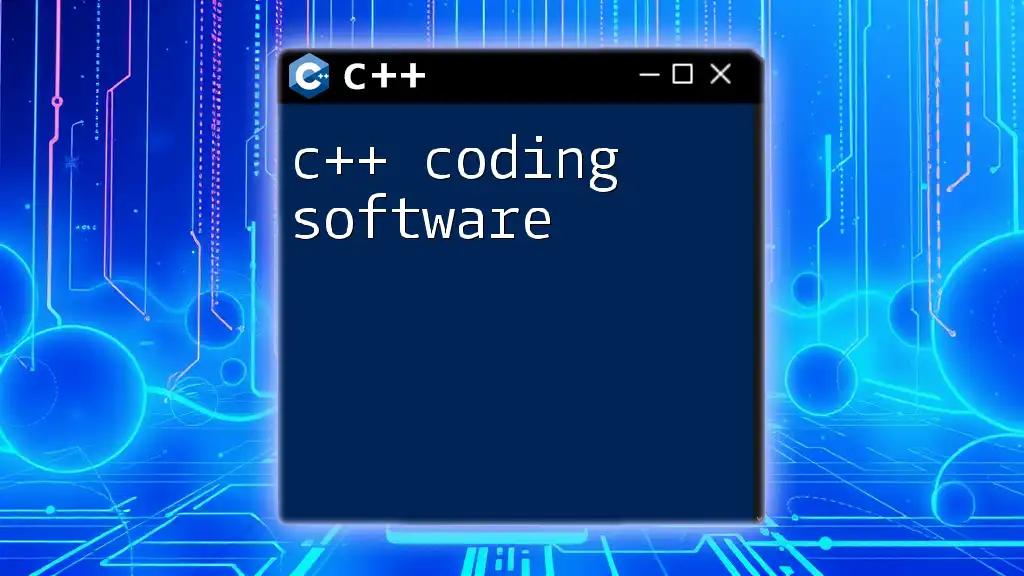
Additional Resources
To further your journey into C++ software development, consider exploring the following:
- Books such as "The C++ Programming Language" by Bjarne Stroustrup
- Online courses and tutorials on platforms like Coursera or Udemy
- Engaging with communities on Stack Overflow or GitHub for peer support
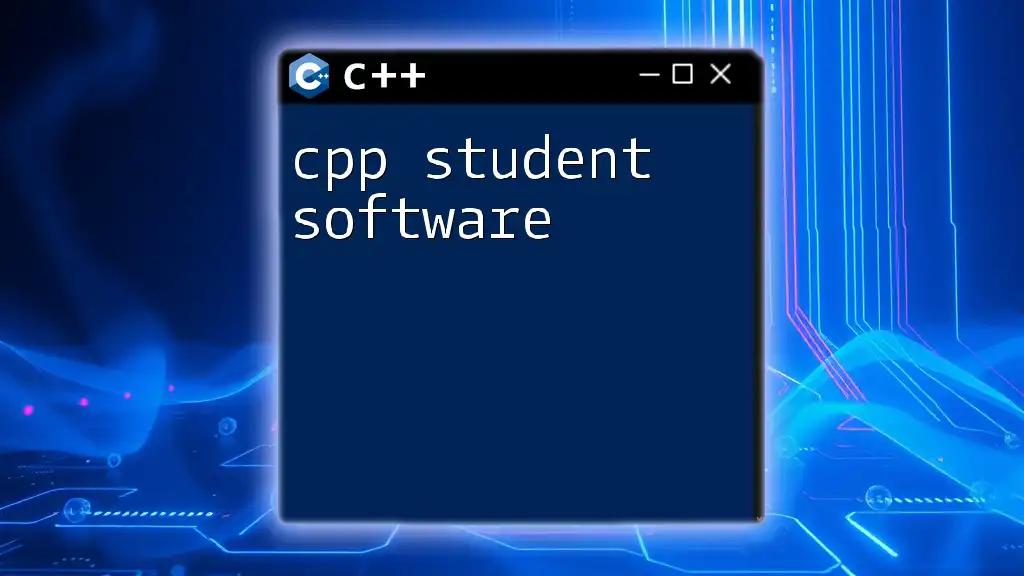
Call to Action
Join our platform today to access concise tutorials on C++ commands and immerse yourself in the world of C++ software. Share your experiences, ask questions, and learn from a community of developers striving for excellence in coding!