A software engineer specializing in C++ designs efficient algorithms and systems by utilizing the language's powerful features, such as object-oriented programming and memory management.
Here's a simple example of a C++ program that defines a class and creates an object:
#include <iostream>
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Understanding C++
What is C++?
C++ is a powerful general-purpose programming language that has been widely used since its creation in the late 1970s. It is an extension of the C programming language and introduces several features that allow developers to write complex and high-performance software. Key features that distinguish C++ from other programming languages include:
- Object-Oriented Programming (OOP): This paradigm emphasizes the use of objects, encapsulation, inheritance, and polymorphism, enabling better data organization and reuse.
- Low-Level Manipulation: C++ allows developers granular control over system resources, enabling efficient memory use and higher performance.
- Standard Template Library (STL): A powerful library that includes various data structures and algorithms, enhancing productivity and efficiency.
C++ is utilized across various industries, from developing video games to building robust financial systems and systems programming in operating systems.
Advantages of Using C++
Adopting C++ in software development brings several advantages that enrich the programming experience:
- Performance: C++ is renowned for its speed and efficiency, making it an excellent choice for performance-critical applications.
- Control Over System Resources: With direct access to memory and system resources through pointers, C++ enables fine-tuned optimization.
- Object-Oriented Programming: By allowing developers to create modular and reusable code, C++ facilitates maintenance and scalability.
- Cross-Platform Capabilities: Write once, run anywhere is pronounced more vividly in C++. Software written in C++ can run on various operating systems with little to no changes.
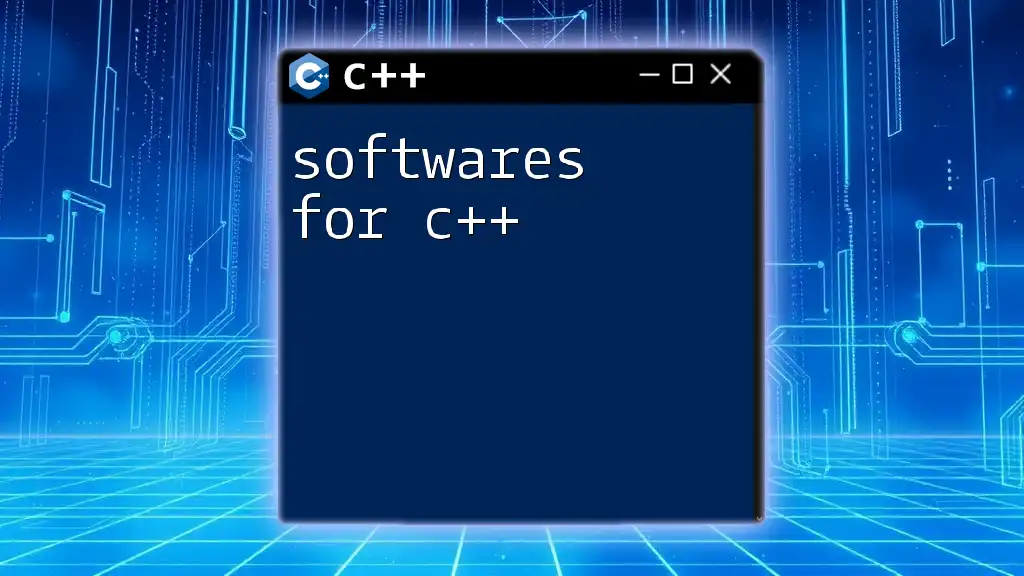
The Role of a C++ Engineer
Responsibilities of a C++ Software Engineer
As a C++ software engineer, the roles and responsibilities span numerous tasks integral to software development:
- Designing and Developing Efficient Algorithms: The engineer is tasked with creating algorithms that meet performance requirements and ensure optimal resource use.
- Debugging and Optimizing Existing C++ Code: Troubleshooting and refining existing code is crucial to maintaining application performance and reliability.
- Collaborating in a Team Environment: Effective communication and thorough documentation methodologies quality in team projects, ensuring collective understanding and contributions.
- Keeping Up-to-Date with Industry Trends and Technologies: Continuous learning is essential in keeping pace with advancements in programming practices and tools.
Required Skills and Qualifications
To flourish as a software engineer in C++, certain skills and qualifications are necessary:
- Proficiency in C++: This includes understanding syntax, functions, data structures, and advanced topics such as memory management and templates.
- Familiarity with Object-Oriented Design Principles: Comprehending concepts like encapsulation, inheritance, and polymorphism plays a key role in effective software design.
- Understanding Data Algorithms and Their Complexities: Being adept at analyzing algorithms ensures the ability to make efficient decisions in software design.
- Additional Skills: Knowledge of other programming languages, familiarity with frameworks, and experience in utilizing version control systems (e.g., Git) enrich a C++ engineer's toolkit.
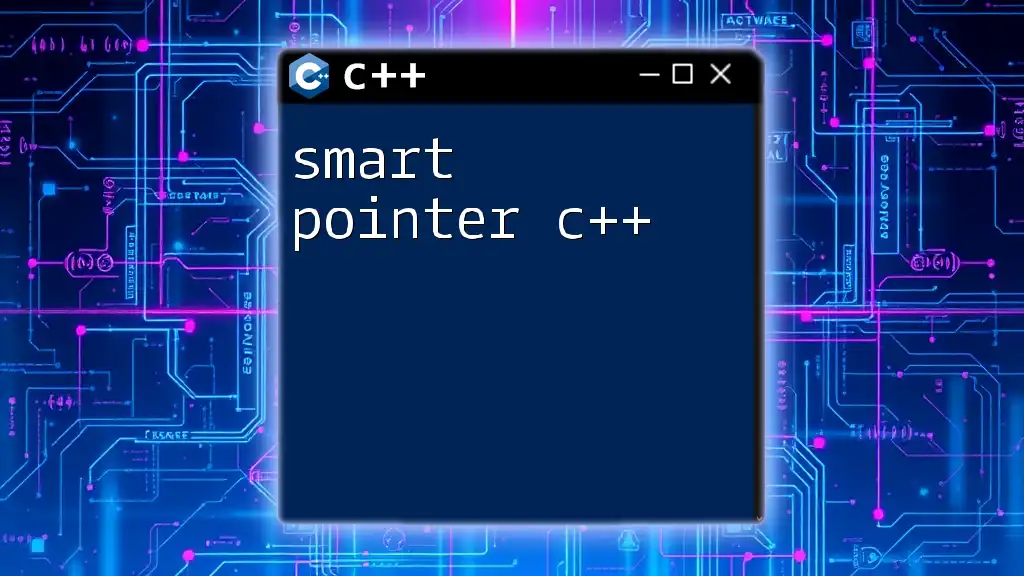
Core Concepts in C++
Syntax and Basics
Grasping the fundamental concepts of C++ is pivotal. Let's explore some of the core syntax and basics:
-
Data Types: A clear understanding of data types is foundational for any programmer. The main data types in C++ include:
int age = 30; float salary = 45000.50; char grade = 'A';
-
Control Structures: Control structures allow the execution of specific code blocks based on conditions. For instance, using loops such as for and while:
for(int i = 0; i < 5; i++) { std::cout << "Iteration: " << i << std::endl; }
-
Functions: Functions enable code reusability and modularity, defined by parameters and return types. A simple function example:
int add(int a, int b) { return a + b; }
Object-Oriented Programming in C++
C++ provides robust support for object-oriented programming, a paradigm that enhances code organization and efficiency.
-
Classes and Objects: The foundation of OOP lies in classes and objects. Consider this class definition:
class Car { public: string model; int year; void drive() { cout << "Driving a " << model << endl; } };
-
Inheritance and Polymorphism: These features allow developers to create new classes that inherit properties and methods from existing classes, fostering code reuse and the ability to design flexible systems.
-
Encapsulation: Encapsulation is essential in OOP, hiding internal states and requiring all interaction to occur through specified methods, improving maintainability.
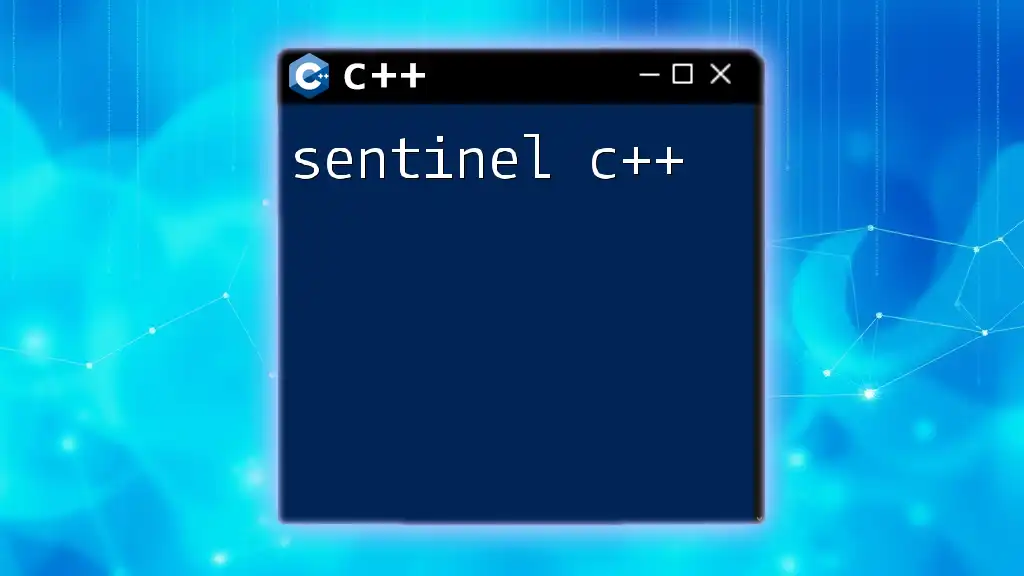
Advanced C++ Concepts
Memory Management
Memory management is a crucial aspect of C++, offering programmers fine-grain control over resource allocation. This includes understanding pointers and references.
-
Dynamic Memory Allocation: Allocating memory during runtime allows the creation of flexible applications. An example demonstrating usage:
int* ptr = new int(42); // ... use ptr delete ptr; // freeing memory
Templates and Generic Programming
Templates contribute immensely to the flexibility and reusability of code in C++. They allow the creation of functions and classes that can operate with any data type. For instance:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function works seamlessly with different data types, promoting code reuse and consistency.
Exception Handling
Robust software applications must incorporate error handling. C++ uses exception handling to manage errors gracefully. By utilizing try, catch, and throw, developers can create resilient applications. Here’s an example:
try {
// Code that may throw an exception
} catch (const std::exception &e) {
std::cerr << "Error: " << e.what() << std::endl;
}
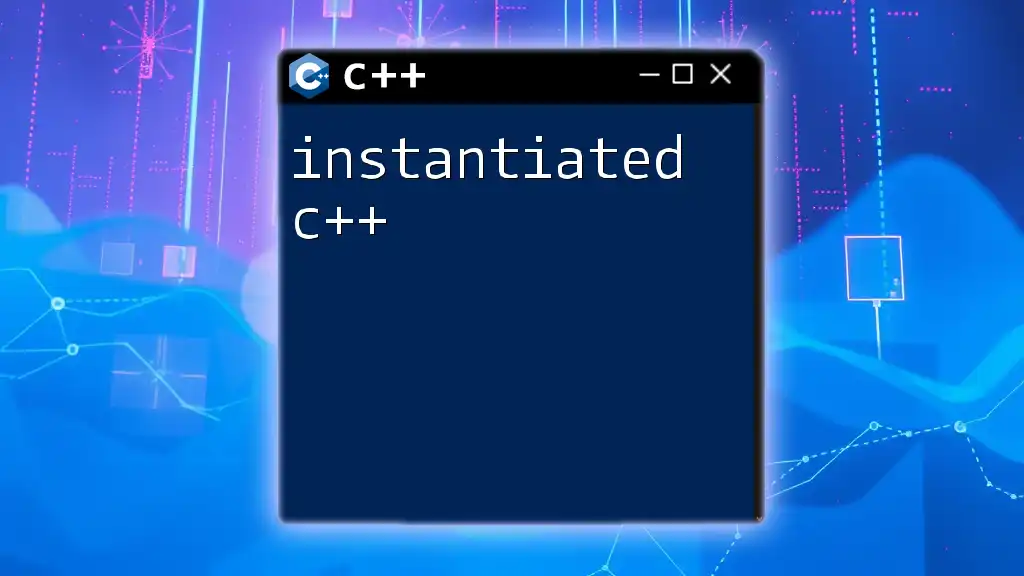
Tools and Libraries for C++ Development
Integrated Development Environments (IDEs)
Choosing the right IDE can significantly enhance productivity for a C++ software engineer. Popular choices include:
- Visual Studio: Feature-rich with debugging tools and code completion.
- Code::Blocks: Lightweight and versatile, ideal for portability.
- CLion: Integrated with powerful refactoring and code analysis tools.
Each IDE provides different features tailored to the needs of C++ developers, making it essential to select one that fits your development style.
Standard Template Library (STL)
The Standard Template Library (STL) offers a variety of data structures and algorithms. STL is a game-changer, providing developers with several out-of-the-box solutions that save time and effort.
For example:
#include <vector>
#include <iostream>
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << std::endl;
}
This simple snippet demonstrates how STL can streamline the process of working with dynamic arrays.
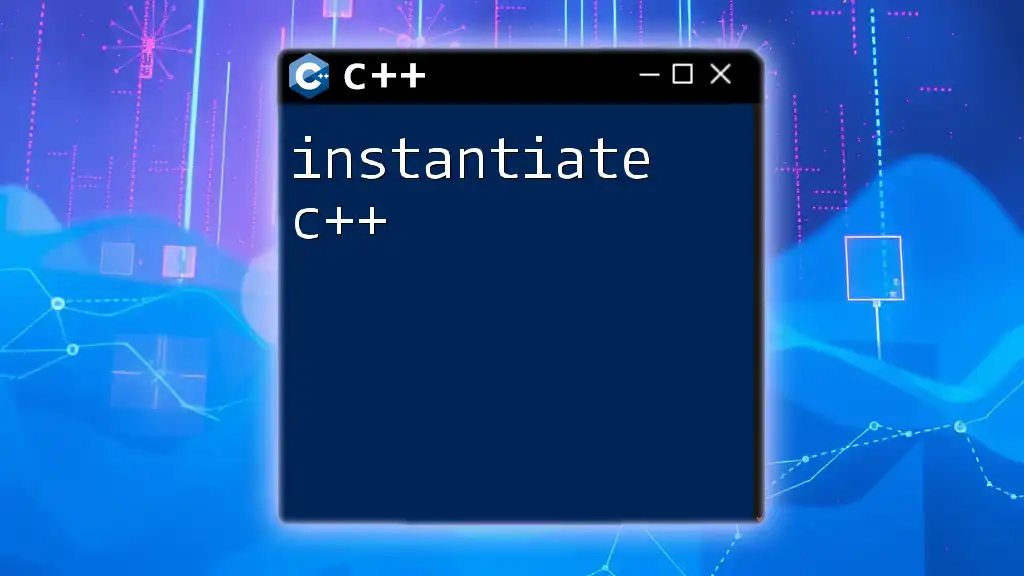
Best Practices for C++ Engineers
Writing Clean and Maintainable Code
The principle of "clean code" is paramount in software engineering. A C++ software engineer should prioritize code readability and maintainability through careful documentation and consistent naming conventions.
By using meaningful identifiers, proper commenting, and organizing code logically, the future maintainability of the software is greatly improved.
Performance Optimization Techniques
Regularly profiling code is essential to identify performance bottlenecks. Efficient algorithms from STL can often perform tasks more quickly than custom code, so leveraging STL ensures both performance and maintainability.
By understanding complexities, C++ engineers can select the best algorithm for the job, ensuring optimal performance.
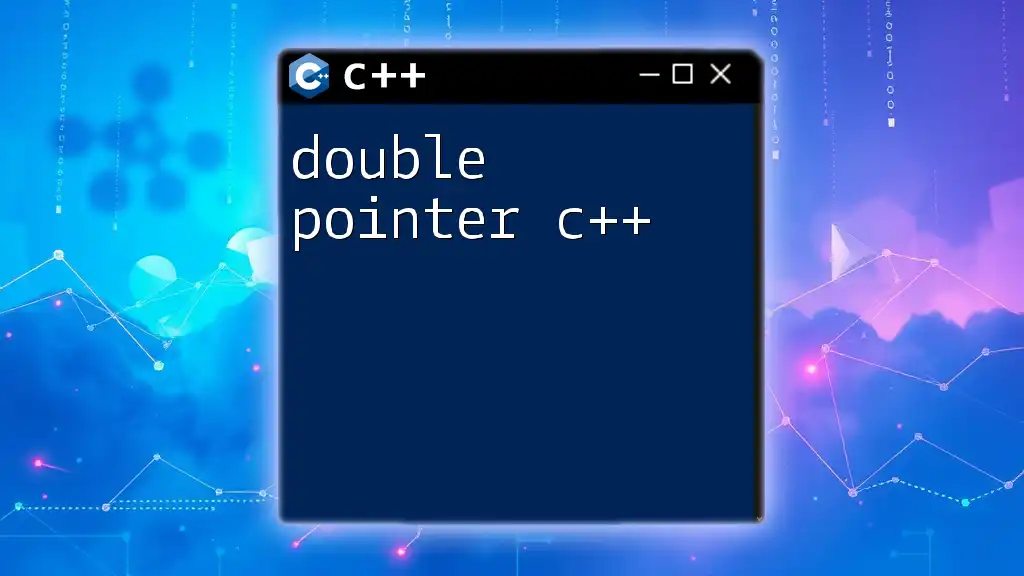
Conclusion
In summary, becoming a software engineer in C++ entails grasping both foundational concepts and advanced techniques that contribute to effective software design and development. The journey requires continuous learning, practice, and an eagerness to adapt to the ever-evolving software landscape. By mastering C++, one can unlock a wealth of opportunities across various industries, showcasing the essential role that C++ engineers play in shaping the future of technology.
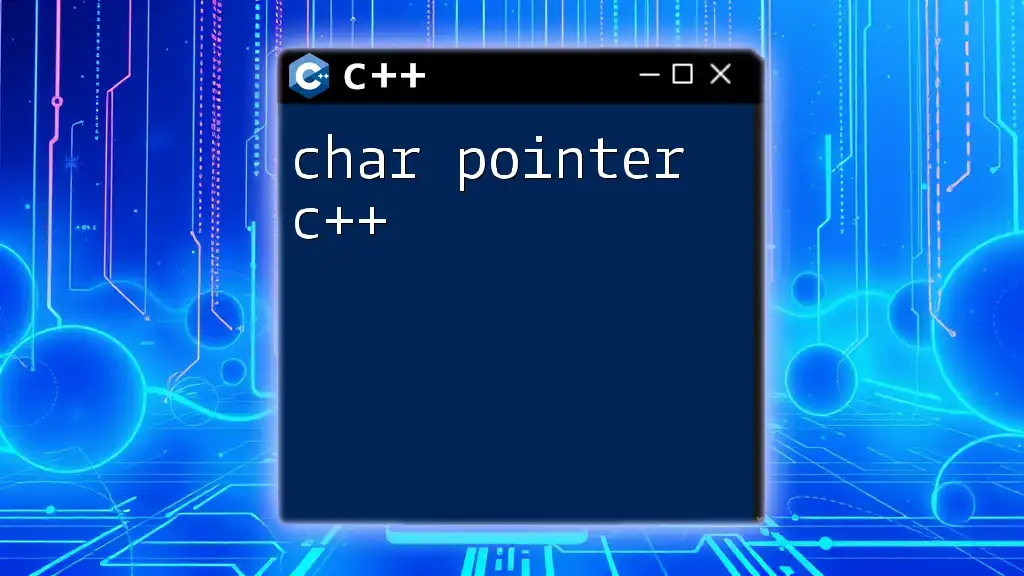
Call to Action
Explore our training programs to enhance your skills in C++ development and maximize your potential as a software engineer. Stay curious, practice continuously, and become part of a thriving community dedicated to mastering C++. Join us and elevate your career today!