A char pointer in C++ is a variable that stores the memory address of a character, allowing for dynamic handling of strings and character arrays.
#include <iostream>
int main() {
char *str = "Hello, World!";
std::cout << str << std::endl; // Output: Hello, World!
return 0;
}
What is a Char Pointer?
A char pointer in C++ is a type of pointer specifically designed to point to character data types. It holds the memory address of a character variable or an array of characters. Understanding char pointers is essential for any C++ programmer, as they play a crucial role in string manipulation, memory management, and efficient data handling.
Benefits of Using Char Pointers
Using char pointers offers several advantages:
- Memory Efficiency: Directly managing memory allocation and deallocation allows for optimized usage of resources.
- Dynamic Memory Allocation: Char pointers enable the creation of dynamic arrays that can adjust during runtime, allowing for more flexible data management.
- Pointer Arithmetic Advantages: Char pointers facilitate operations on character data through arithmetic manipulation, enhancing control over data structure navigation.
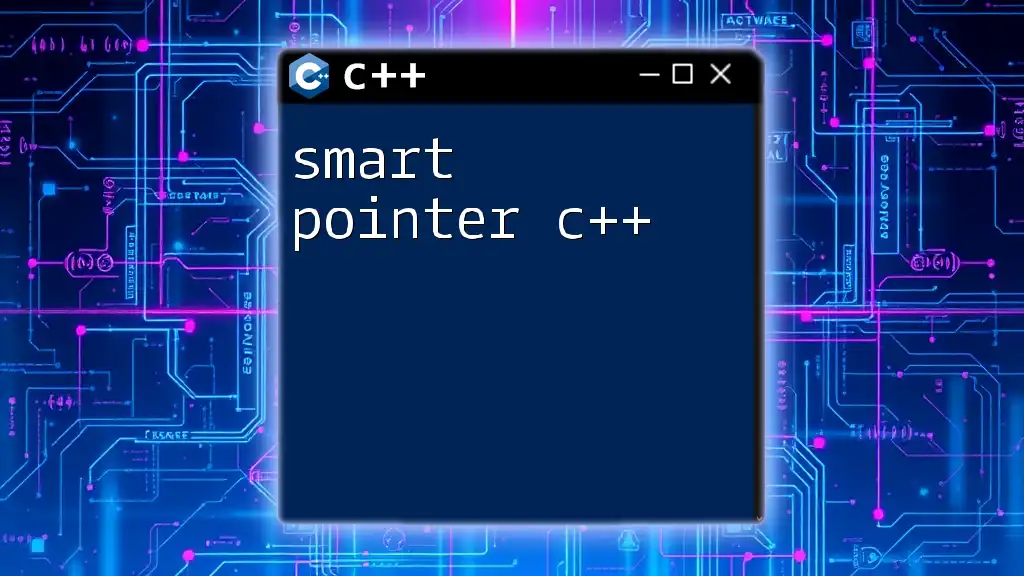
Understanding the Basics
What is a Pointer?
In C++, a pointer is a variable that stores the memory address of another variable. It acts as a reference, enabling indirect access to the value stored at a particular memory location. This indirect access is crucial for building complex data structures and effectively managing memory.
What is a Character in C++?
A character in C++ is represented by the `char` data type, which typically occupies one byte of memory. Characters are stored in ASCII format, where each character corresponds to a specific integer value, facilitating easy manipulation and representation.
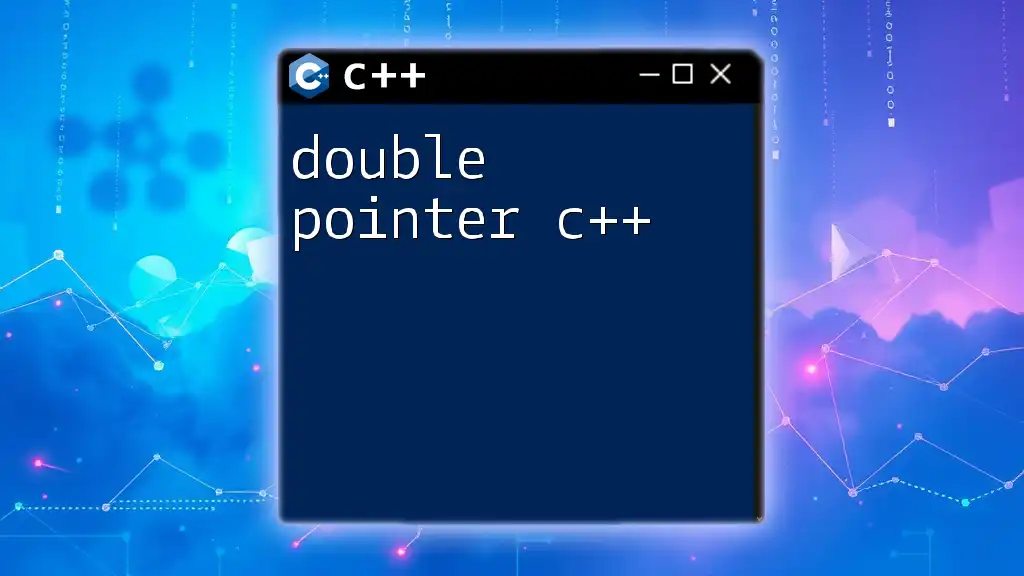
Char Pointer Syntax in C++
Declaration of Char Pointers
To use a char pointer, you must first declare it. The syntax for declaring a char pointer is as follows:
char* myCharPointer;
In this example, `myCharPointer` is a pointer that can hold the address of a character variable.
Initialization of Char Pointers
Once declared, a char pointer must be initialized before use. There are several ways to initialize char pointers:
-
Direct Initialization: Assign the address of a character variable to the pointer.
char ch = 'A'; char* ptr = &ch;
-
Using String Literals: A char pointer can also be initialized with string literals.
char* message = "Hello, World!";
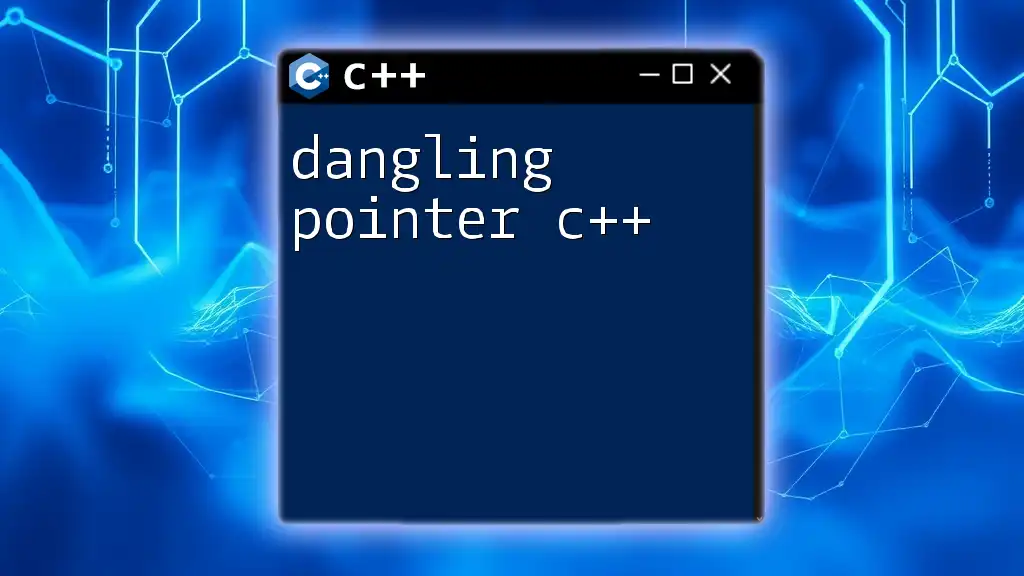
Working with Char Pointers
Accessing Character Values
To access the value pointed to by a char pointer, you use the dereferencing operator (`*`). This allows you to read or modify the character stored in that memory location.
For example:
char ch = 'B';
char* ptr = &ch;
std::cout << *ptr << std::endl; // Outputs: B
Pointer Arithmetic with Char Pointers
Pointer arithmetic can be performed on char pointers, enabling navigation through arrays of characters. When you increment a char pointer, it moves to the next character in memory, allowing for convenient string manipulation.
For instance:
char str[] = "Hello";
char* p = str;
while (*p != '\0') {
std::cout << *p;
p++; // Move to the next character
}
This example prints each character of the string "Hello" until it reaches the null terminator.
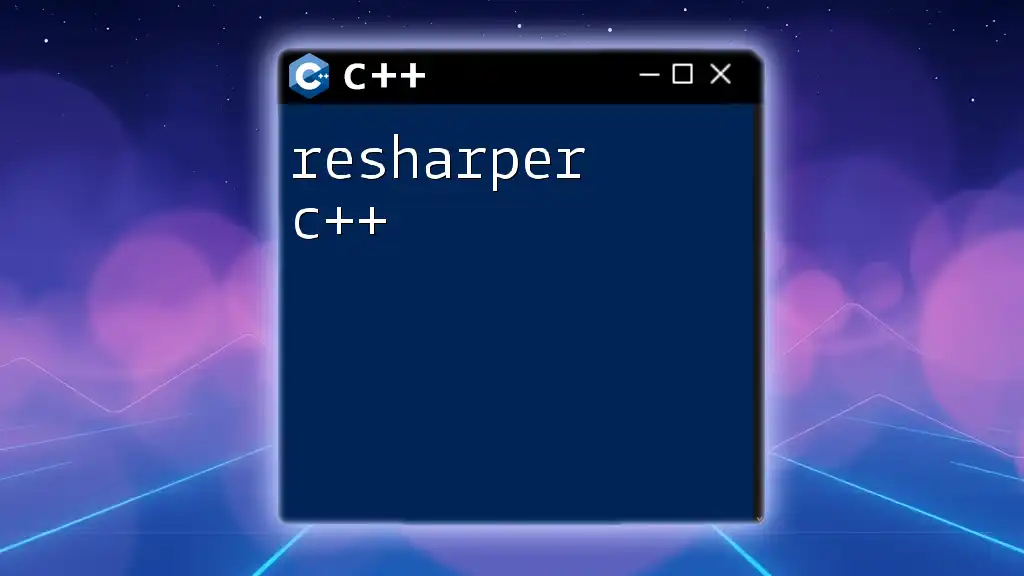
Char Pointers with Arrays and Strings
Char Pointers and Character Arrays
Character arrays are collections of characters terminated with a null character (`'\0'`). Char pointers can be used to navigate and manipulate these arrays efficiently.
For example:
char myArray[] = "Hello";
char* ptr = myArray;
std::cout << *ptr << std::endl; // Outputs: H
Manipulating Strings with Char Pointers
Char pointers can be used to manipulate strings through various standard functions. For instance, you can use functions like `strcpy` to copy strings from one pointer to another.
char source[] = "World";
char destination[6];
strcpy(destination, source);
std::cout << destination << std::endl; // Outputs: World
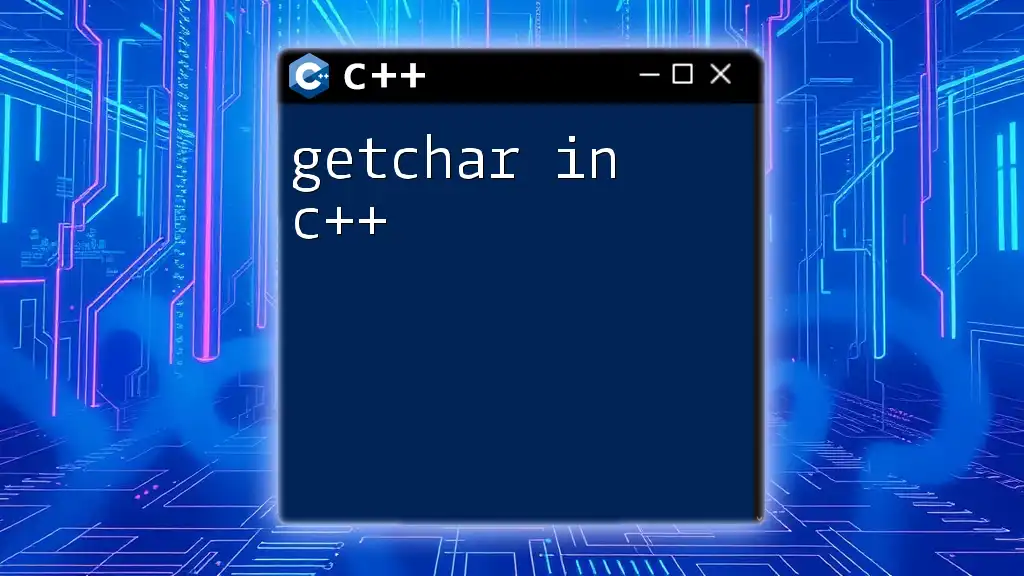
Advanced Topics in Char Pointers
Dynamic Memory Allocation for Character Arrays
Char pointers can be employed for dynamic memory allocation using the `new` keyword, which allows developers to create arrays with sizes determined at runtime.
For instance:
char* dynamicArray = new char[20]; // Dynamically allocates 20 characters
// Remember to delete later to avoid memory leaks
delete[] dynamicArray;
Const Char Pointers and the `const` Keyword
In certain situations, you might want to have a pointer that cannot modify the data it points to. This is achieved by declaring a const char pointer.
const char* constPtr = "Constant";
Here, `constPtr` points to a constant string that should not be altered.
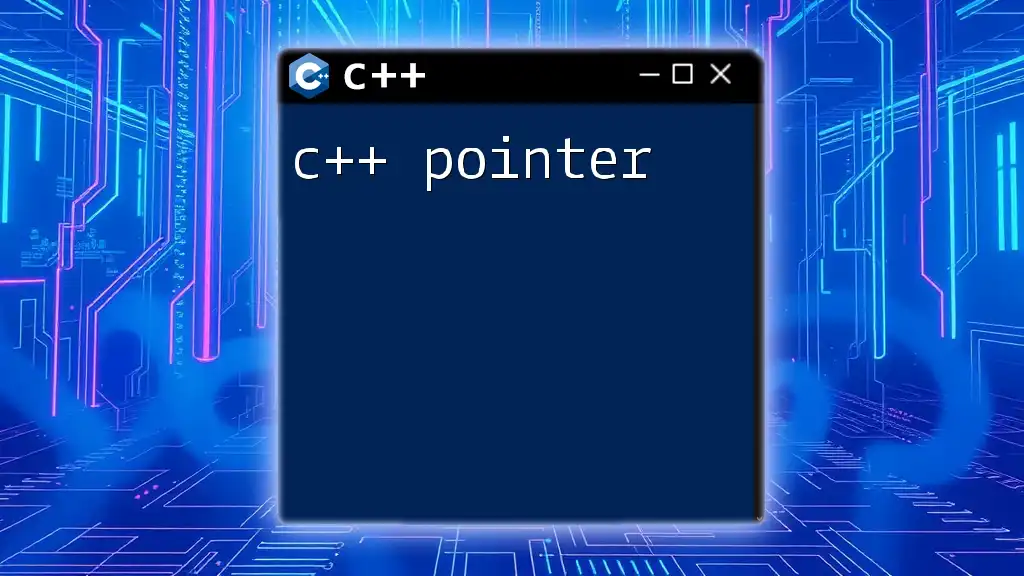
Common Mistakes and Troubleshooting
Common Pitfalls with Char Pointers
New programmers often encounter pitfalls when working with char pointers:
- Dereferencing Null Pointers: Attempting to access memory via a null pointer leads to undefined behavior. Always ensure your pointers are initialized before dereferencing.
- Memory Leaks in Dynamic Memory Allocation: Allocate and free memory appropriately to avoid leaks, which can compromise performance.
- Buffer Overflow Issues: When copying string data, ensure the destination buffer is large enough to hold the data, including the null terminator.
Best Practices for Using Char Pointers
To ensure effective management of char pointers, follow these best practices:
- Avoid dangling pointers by ensuring all pointers are properly initialized before use.
- Implement consistent memory management by freeing any dynamically allocated memory using `delete` to prevent leaks.
- Use smart pointers (`std::unique_ptr` or `std::shared_ptr`) when possible to manage pointers automatically and safely.
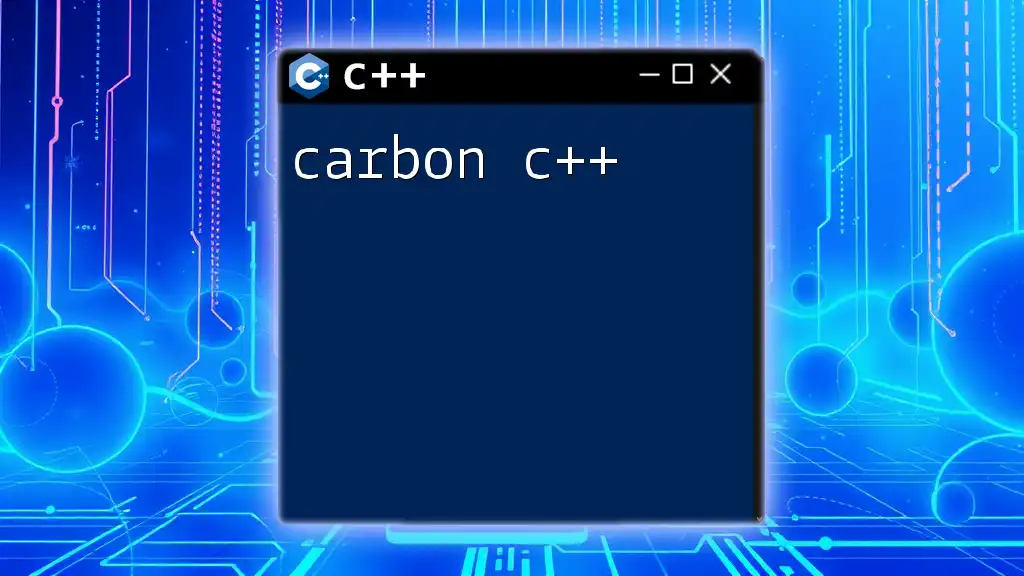
Conclusion
Understanding char pointers in C++ is essential for effective programming and memory management. Mastery of char pointers allows for dynamic string manipulation, efficient memory use, and a greater understanding of how C++ manages data in memory. By applying the principles and techniques discussed in this guide, programmers can enhance their proficiency and confidence in using char pointers effectively.
Further Resources
For those looking to deepen their knowledge, various online resources, documentation, and textbooks are available that cover pointers and memory management in detail. Consider exploring C++ Standard Library resources, tutorials, and comprehensive programming books to further your learning journey in C++.