In C++, the `vector::insert` method is used to add elements to a vector at a specified position, allowing for dynamic resizing and efficient management of data.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3};
vec.insert(vec.begin() + 1, 10); // Inserts 10 at the second position
for (int num : vec) {
std::cout << num << " "; // Outputs: 1 10 2 3
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a sequence container that encapsulates dynamic size arrays. Unlike traditional arrays, vectors can grow and shrink in size, allowing for more flexible memory management. This is particularly useful in scenarios where the exact number of elements is not known at compile time.
Vectors are part of the C++ Standard Library and are defined in the `<vector>` header. They offer numerous advantages, including:
- Automatic resizing: Vectors automatically manage memory as elements are added or removed.
- Easy access: Elements can be accessed using an index, similar to arrays.
- Rich set of member functions: Vectors come with built-in functions to enhance their usability.
Basic Operations on Vectors
Before diving into the `insert` functionality, it’s essential to understand some basic operations:
-
Declaration and Initialization: Vectors can be declared and initialized in various ways:
std::vector<int> vec; // Empty vector std::vector<int> vec2(5); // Vector of size 5, initialized to 0 std::vector<int> vec3{1, 2, 3}; // Vector with initial values
-
Common vector operations: Besides `insert()`, vectors allow for operations like:
- `push_back(value)`: Adds an element at the end.
- `pop_back()`: Removes the last element.
- `size()`: Returns the current number of elements.
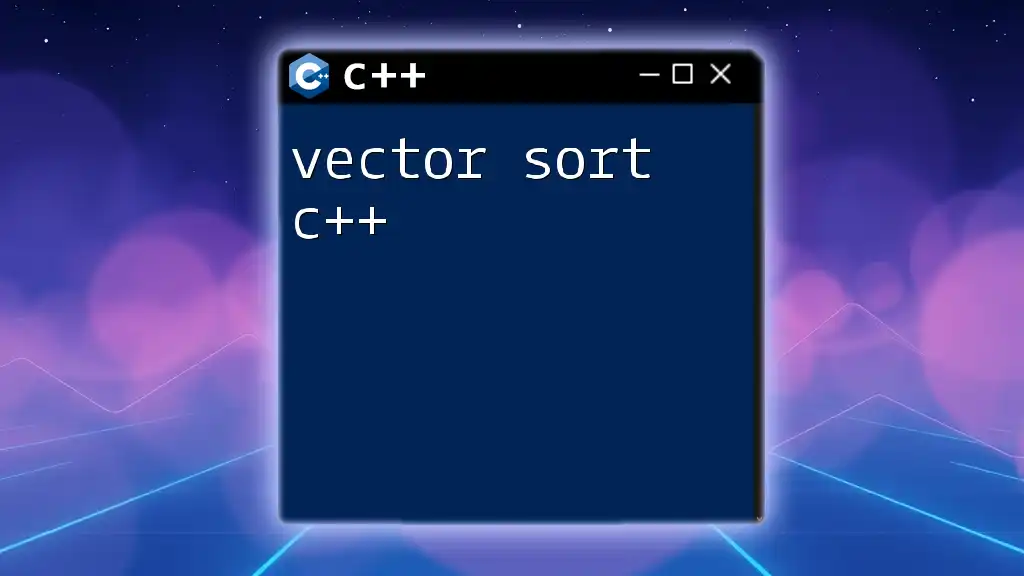
The Insert Function in C++
Syntax of the Insert Function
The `insert` function allows you to add elements into a vector at a specified position. The syntax of the `insert` function is as follows:
iterator insert (const_iterator position, const T& value);
iterator insert (const_iterator position, const T& value, size_type n);
iterator insert (const_iterator position, InputIterator first, InputIterator last);
Here, position is the iterator pointing to the location where the new element(s) will be inserted, value is the element to be inserted, and n is the number of times to insert that value.
How Insert Works
When you call the `insert` function, the vector dynamically reallocates memory if necessary to accommodate new elements. It shifts the subsequent elements to make space for the new elements. This operation causes the vector's size to increase, and if the capacity is exceeded, a reallocation occurs.
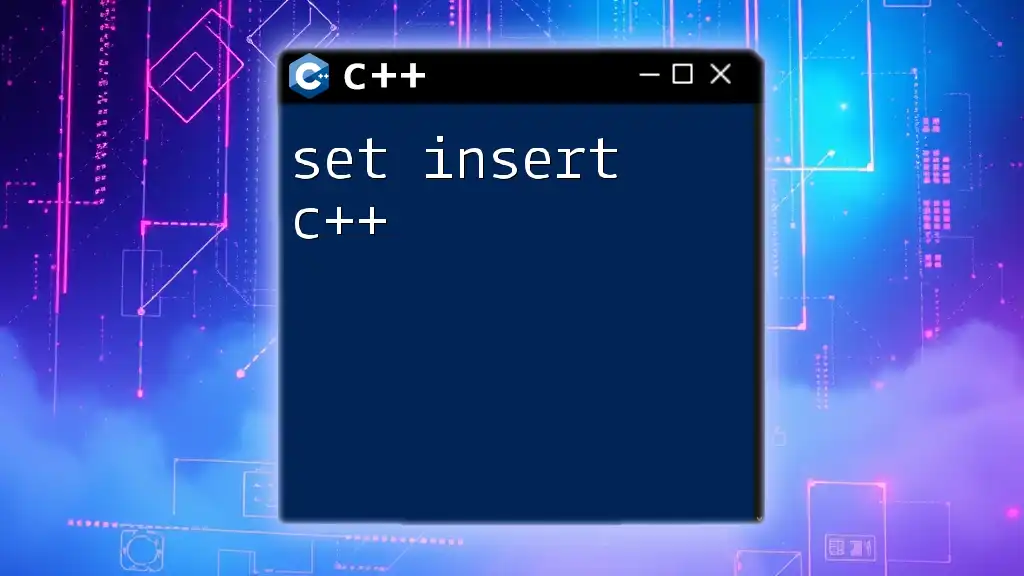
Using the Insert Function
Inserting Single Elements
You can insert a single element at any valid position within the vector. For instance:
std::vector<int> vec {1, 2, 3};
vec.insert(vec.begin() + 1, 10); // Inserting 10 at position 1
In this example, the vector initially contains {1, 2, 3}. After the insertion, the vector will change to {1, 10, 2, 3}. The `insert` function effectively shifts the elements to the right of the insertion point.
Inserting Multiple Elements
The `insert` function can also be used to insert multiple copies of a single value into the vector. For example:
std::vector<int> vec {1, 2, 3};
vec.insert(vec.end(), 3, 5); // Inserting 5 three times at the end
After this operation, the vector will look like {1, 2, 3, 5, 5, 5}. Here, the `count` parameter specifies how many times the value `5` should be inserted.
Inserting an Entire Range
The `insert` function can also take a range of elements from another container. This can be incredibly useful when merging or combining data. For example:
std::vector<int> vec1 {1, 2, 3};
std::vector<int> vec2 {4, 5, 6};
vec1.insert(vec1.end(), vec2.begin(), vec2.end()); // Insert all elements from vec2
The resulting `vec1` will contain {1, 2, 3, 4, 5, 6}. By passing iterators from the second vector, you ensure that all elements are efficiently copied into the first vector.
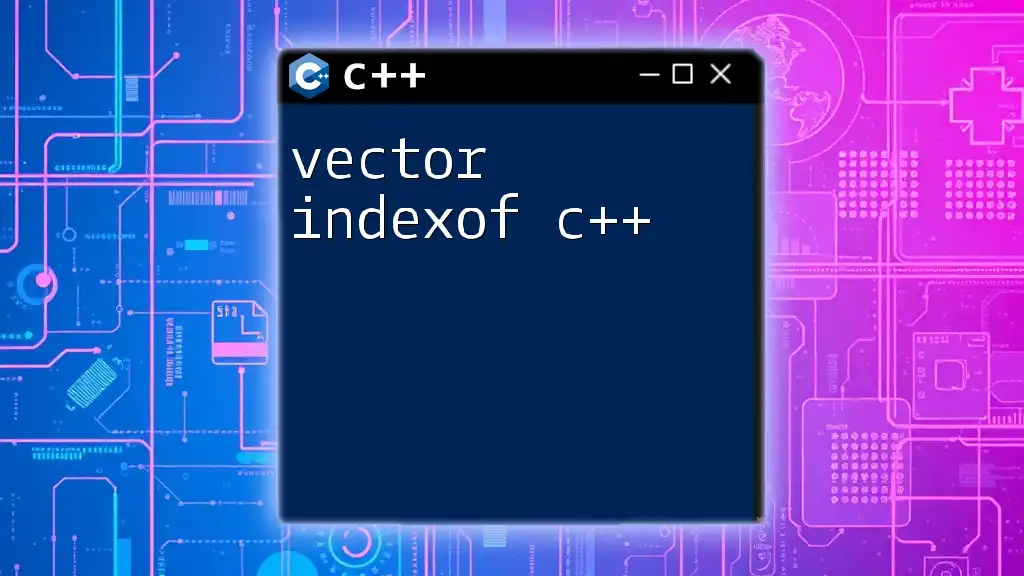
Practical Examples
Common Use Cases for Vector Insert
In practice, the `insert` operation is often utilized in scenarios where dynamic data manipulation is necessary. For instance, you might want to maintain a sorted list of numbers. By inserting elements in the correct order using `insert`, you can keep the vector sorted after every addition.
Performance Considerations
When it comes to time complexity, the `insert` function can be relatively expensive, especially if the insertion point is near the beginning of the vector. The time complexity can be O(n) in the worst case because it may involve shifting many elements. Therefore, it is essential to consider your use case and the size of the vector when using insertions.
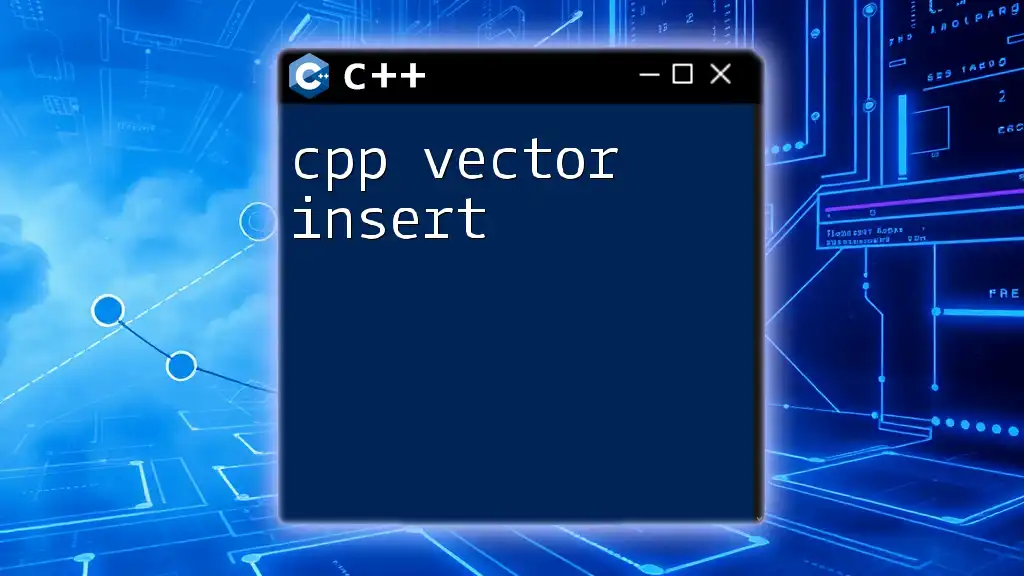
Best Practices
When to Use Insert vs Other Methods
Choosing between `insert()` and other methods like `push_back()` often depends on your specific needs. Use `insert()` when you need precise control over where elements are added, while `push_back()` is ideal for appending items to the end.
Ensuring Optimal Performance
To ensure better performance, consider preallocating space if you know the number of elements in advance. You can use `reserve(size)` to reserve memory, minimizing the need for frequent reallocations.
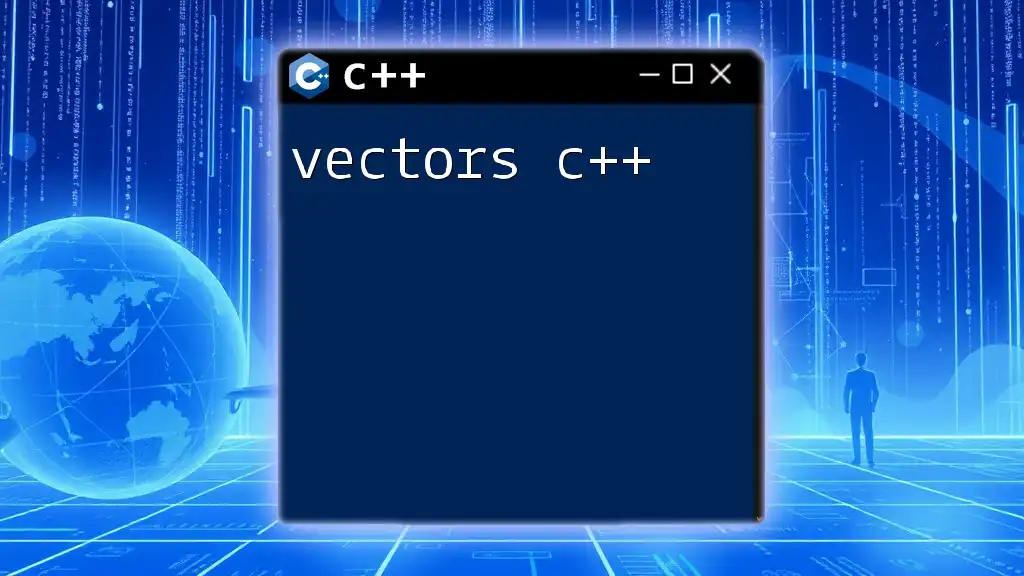
Conclusion
Understanding the `vector insert c++` functionality is essential for anyone working with C++ Standard Library containers. The `insert` function offers powerful capabilities for managing dynamic collections effectively. By practicing various insertion methods and factors affecting performance, developers can effectively leverage vectors for their data manipulation needs.
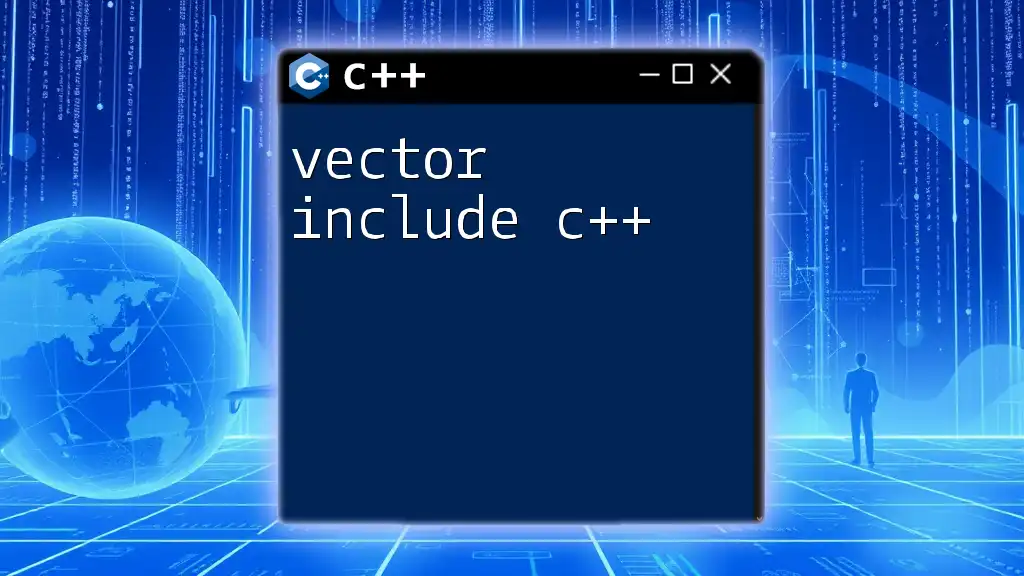
Additional Resources
For further exploration into vectors and more advanced data structures, consider checking out the official C++ documentation and numerous online resources available for in-depth learning. Learning through practical examples will enhance your command over C++ and help you become more proficient in using such powerful data structures.