The `back()` function in C++ vectors is used to access the last element of the vector without removing it.
Here’s a simple example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::cout << "Last element: " << numbers.back() << std::endl;
return 0;
}
What is a C++ Vector?
Overview of Vectors
Vectors in C++ are a part of the Standard Template Library (STL) and represent a dynamic array. Unlike traditional arrays, which have a fixed size, vectors can grow or shrink as needed. This dynamic behavior makes them versatile and widely used in C++ programming.
Advantages of Using Vectors
Using vectors in C++ provides several advantages:
- Dynamic Sizing: Vectors can automatically adjust their size during runtime, making them ideal for scenarios where the number of elements is unknown until execution.
- Ease of Use: Vectors come with a variety of built-in functions that simplify manipulation and access to data.
- Automatic Memory Management: C++ vectors handle memory allocation and deallocation automatically, reducing the risk of memory leaks.
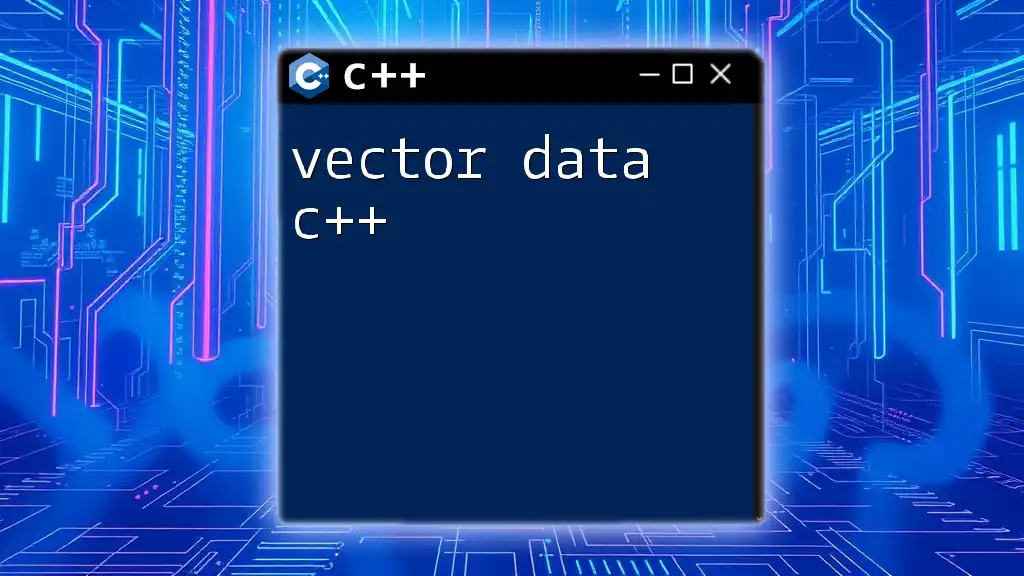
Understanding the `back()` Function
What Does `back()` Do?
The `back()` function is designed to provide direct access to the last element of a vector. It returns a reference to this element, enabling not just retrieval of its value, but also modification when necessary.
Syntax of `back()`
Here’s the basic syntax for using `back()`:
reference back();
const_reference back() const;
The first variant allows you to modify the last element, while the `const` version prevents any modifications. This distinction is crucial for maintaining data integrity in your applications.
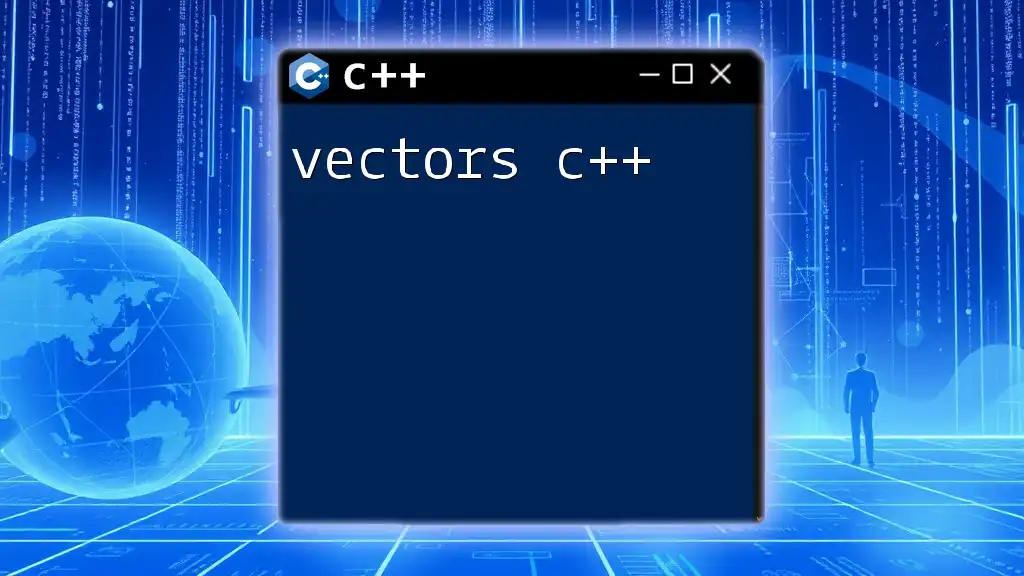
How to Use `back()` in C++
Basic Example of `back()`
To illustrate the use of `back()`, consider the following example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
std::cout << "The last element is: " << vec.back() << std::endl;
return 0;
}
In this example, the output would be 50, as it is the last element in the vector. This emphasizes how simple and efficient it is to retrieve the last element using `back()`.
Modifying the Last Element
Using `back()` to Change the Value
Another powerful feature of `back()` is its ability to modify the last element directly. For instance, if you wanted to change the last element to 60, you could do this:
vec.back() = 60;
This directly alters the last element in the vector, showcasing how `back()` not only helps retrieve data but also allows for updates in a clean, readable manner.
Importance of Checking Vector Size
Safe Use of `back()`
Using `back()` on an empty vector can lead to undefined behavior. Therefore, it is always good practice to check whether the vector has elements:
if (!vec.empty()) {
std::cout << "Last element: " << vec.back() << std::endl;
}
This check ensures you avoid runtime errors and maintain code safety, especially when working with vectors that can change sizes dynamically.
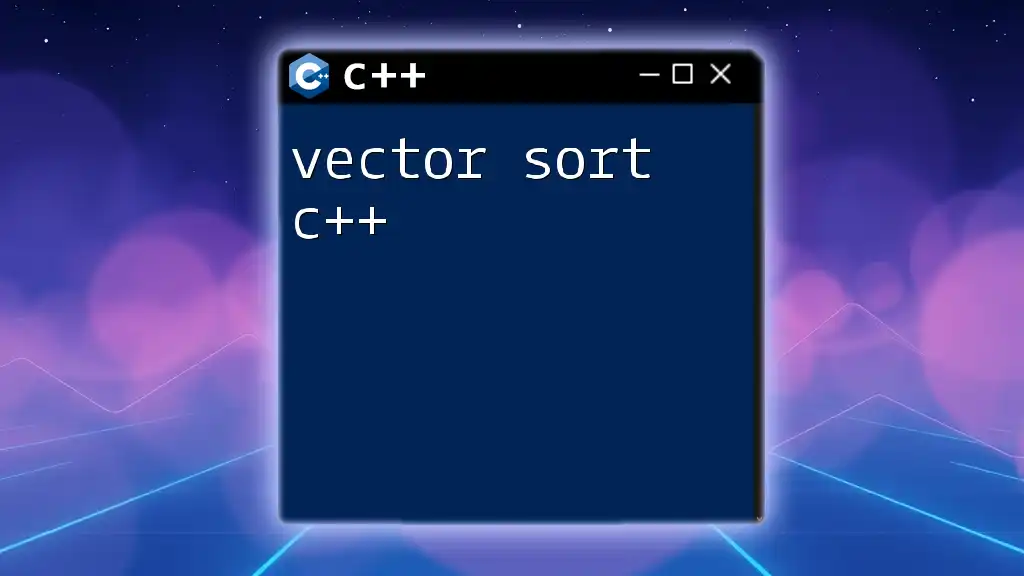
Common Use Cases for `back()`
Iteration and Accessing Last Elements
In many applications, you may frequently need to access the last element of your collection for various computations or checks. For example, in algorithms that require backtracking, the last element often represents the current state.
Using `back()` with Other Vector Operations
Combining with `pop_back()`
The `back()` function shines when used in combination with `pop_back()`, which removes the last element from the vector. This can be useful when you want to know what you are about to remove:
std::cout << "Removing the last element: " << vec.back() << std::endl;
vec.pop_back();
Here, you ensure the last element's value is known before it's removed, creating clearer code and preventing any accidental loss of data.
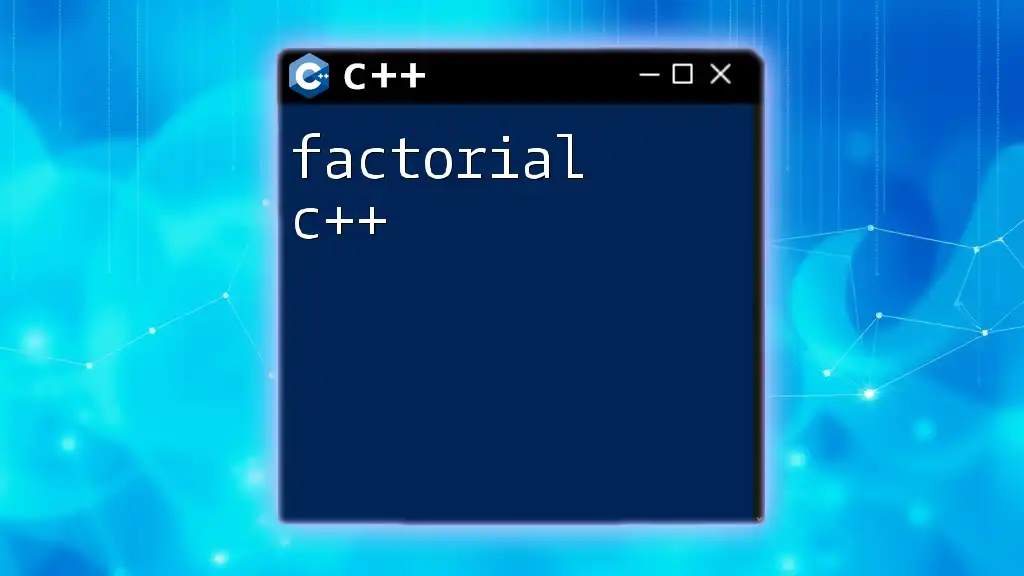
Performance Considerations
Complexity of Accessing Last Element
Accessing the last element using `back()` operates with a constant time complexity of O(1). This effectiveness is crucial when dealing with large datasets, allowing for efficient data retrieval without the overhead typically associated with traversing structures.
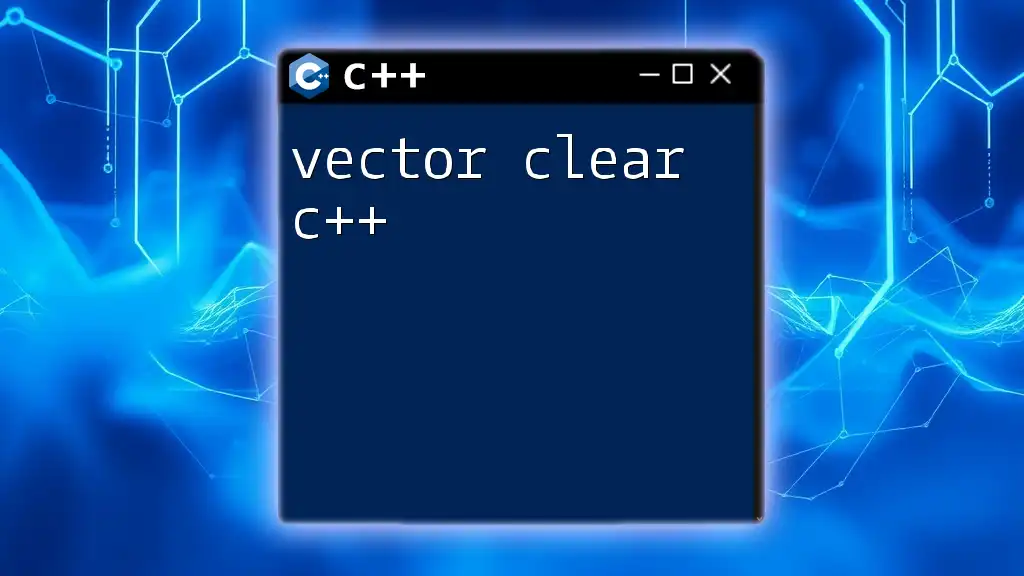
Conclusion
Understanding the `back()` function in the context of vectors is essential for any C++ programmer. This function not only simplifies code but also enhances its readability and maintainability. By mastering `back()`, you'll be able to handle vectors more effectively, paving the way for cleaner and more robust C++ applications.
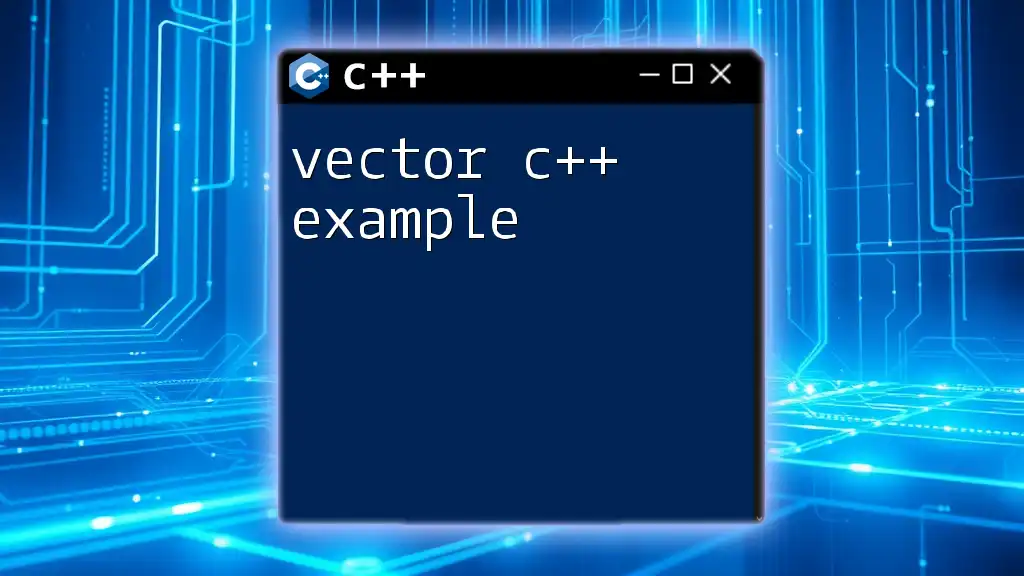
Additional Resources
Further Reading
For more extensive knowledge on the C++ Standard Library and vectors, consider exploring the official documentation. Familiarizing yourself with the principles of dynamic memory management will also aid in your journey to becoming a proficient C++ developer.
Recommended Tools
Choosing the right development environment can significantly improve your coding experience. IDEs like Visual Studio and Code::Blocks provide the necessary tools for developing and testing vector operations and other C++ functionalities.
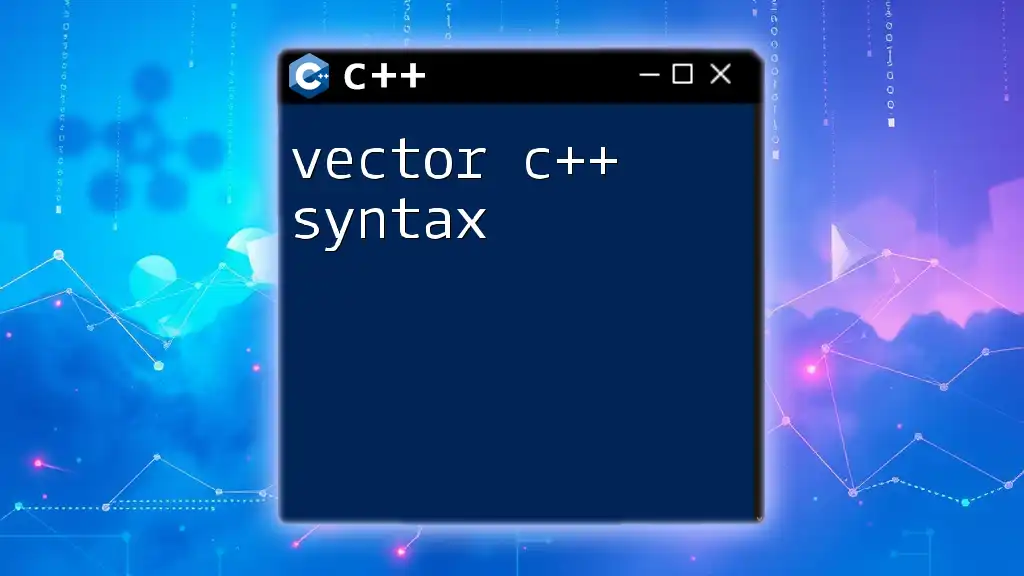
Call to Action
Share your experiences or questions regarding the `back()` function or any other aspects of C++ vectors in the comments below. Don’t forget to subscribe to our newsletter for concise tutorials and guides designed to make you proficient in C++.