In C++, a vector is a dynamic array that can grow in size, allowing for efficient storage and manipulation of a collection of elements.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Add an element at the end
for (int num : numbers) {
std::cout << num << " "; // Display each number
}
return 0;
}
What is a Vector?
In C++, a vector is a sequence container that can dynamically resize itself to accommodate more elements. Unlike traditional arrays, which have a fixed size, vectors can grow and shrink as needed, making them a versatile option for handling collections of data. Vectors are part of the Standard Template Library (STL) and provide several advantages over basic arrays, including built-in memory management and a variety of member functions that simplify complex tasks.
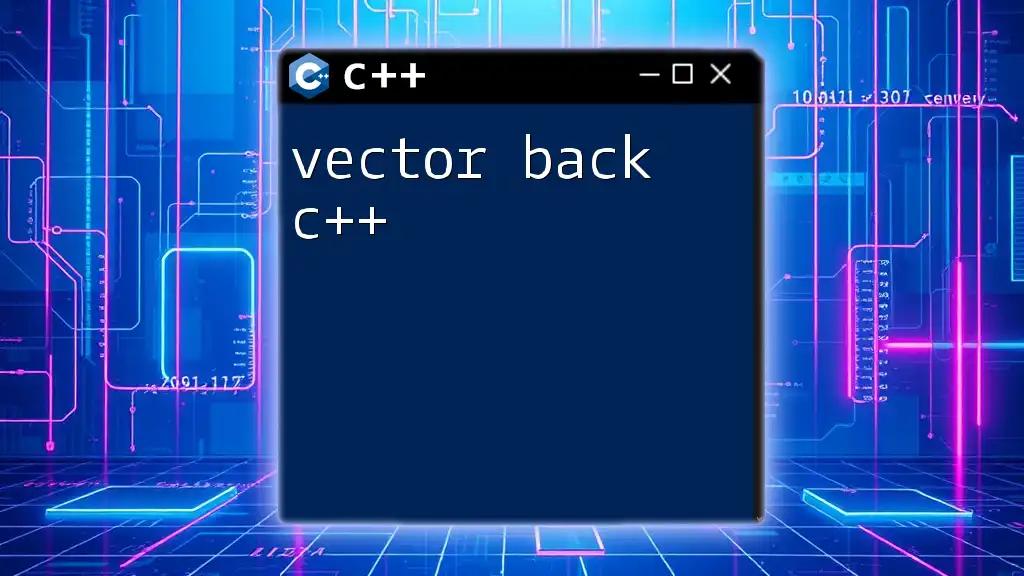
Why Use Vectors?
There are several reasons why vectors are often preferred in C++ programming:
- Dynamic Sizing: Vectors automatically adjust their size based on the number of elements they hold, eliminating the need for manual reallocation.
- Built-in Functionalities: C++ vectors come with a rich set of functions that simplify the management of collections, making coding easier and less error-prone.
- Memory Efficiency: Vectors make use of contiguous memory, improving performance during element access due to better cache locality compared to non-contiguous data structures.
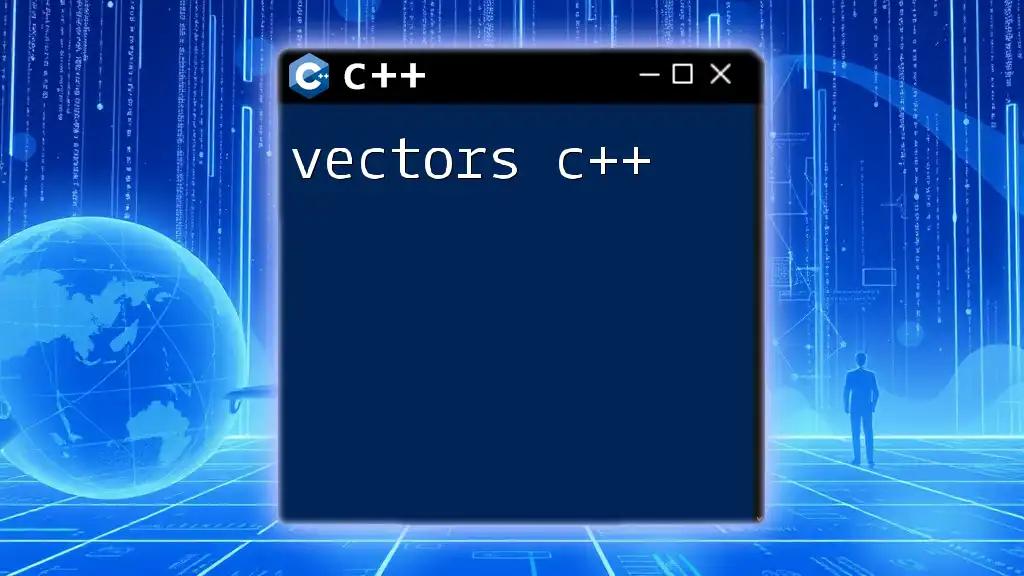
Understanding C++ Vector Data Structure
Overview of Vector Structure
Vectors manage their own memory internally. When the number of elements exceeds the current capacity, they allocate a larger block of memory and copy the existing elements to it. This process, while efficient, can temporarily affect performance during resizing. Understanding this behavior is crucial for optimizing your applications.
Key Properties of Vectors
Size and Capacity
- Size: The current number of elements in the vector, accessible via the `size()` member function.
- Capacity: Indicates the amount of allocated storage (approximates the maximum number of elements the vector can hold before needing to allocate more memory). Use `capacity()` to retrieve this value.
Understanding these properties helps manage performance, especially in large applications where memory usage can significantly impact execution time.
Element Access
Vectors support multiple methods for accessing elements, including:
- `at(index)`: Provides bounds-checked access to elements.
- `[]`: Faster access that doesn’t perform bounds checking.
- `front()`: Returns the first element.
- `back()`: Returns the last element.
Example of element access:
std::vector<int> numbers = {10, 20, 30};
std::cout << numbers.at(1); // Outputs 20
std::cout << numbers[2]; // Outputs 30

Basic Operations on Vectors
Creating a Vector
To create a vector, include the `<vector>` header and declare it like so:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
You can also create an empty vector and add elements later.
Adding Elements
To add an element to a vector, use the `push_back()` function, which appends an element to the end:
numbers.push_back(6); // Vector now contains {1, 2, 3, 4, 5, 6}
Removing Elements
To remove the last element, use `pop_back()`:
numbers.pop_back(); // Vector now contains {1, 2, 3, 4, 5}
For removing a specific element, use the `erase()` function along with an iterator:
numbers.erase(numbers.begin() + 1); // Removes the element at index 1
Iterating Through a Vector
One straightforward way to iterate through a vector is by using a range-based for loop:
for (const auto &num : numbers) {
std::cout << num << " "; // Outputs: 1 2 3 4 5
}
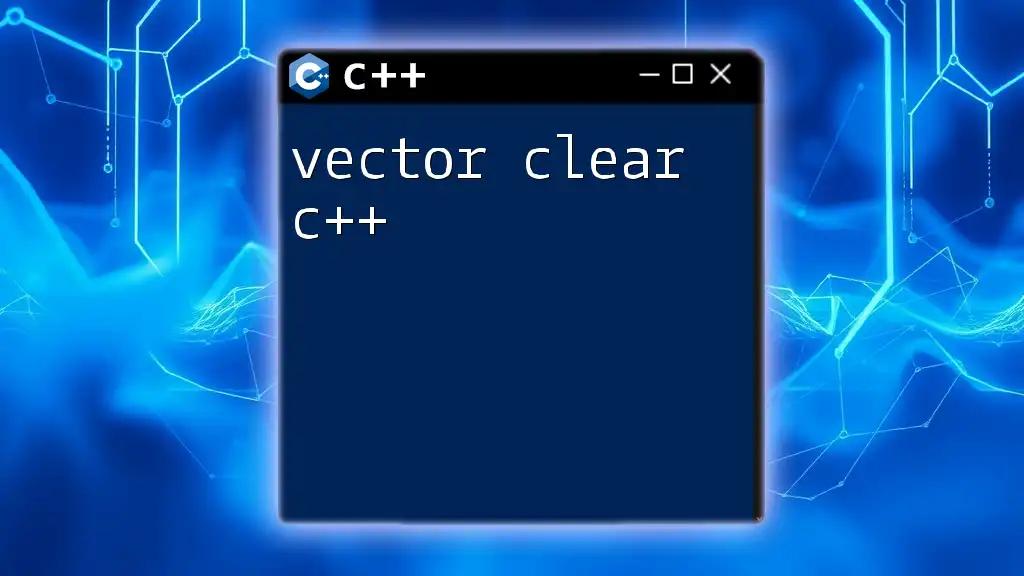
Advanced Vector Features
Resizing Vectors
You can change the size of a vector using the `resize()` function, which expands or reduces the size of the vector:
numbers.resize(10); // Expands the vector to hold 10 elements
Sorting Vectors
Sorting is easily accomplished using the `std::sort()` algorithm provided by the `<algorithm>` header:
#include <algorithm>
std::sort(numbers.begin(), numbers.end()); // Sorts the vector in ascending order
Searching in Vectors
To find an element in a vector, the `std::find()` function from the `<algorithm>` header can be utilized:
#include <algorithm>
auto it = std::find(numbers.begin(), numbers.end(), 3);
if (it != numbers.end()) {
std::cout << "Element found: " << *it << "\n"; // Outputs: Element found: 3
}
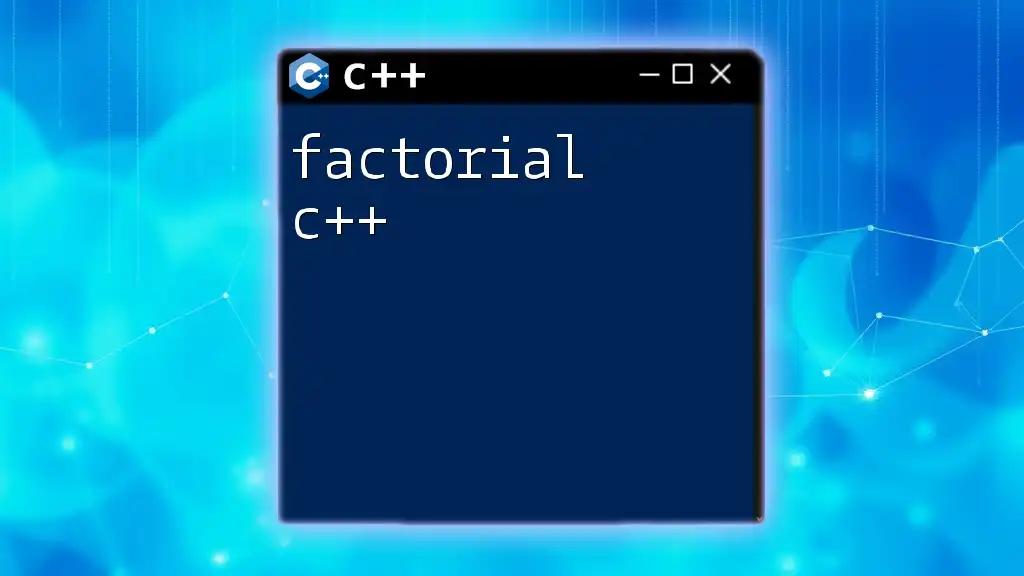
Vector Algorithms and Functions
Common Vector Functions
Vectors come with various member functions that serve different purposes, such as:
- `clear()`: Removes all elements from the vector, resulting in a size of zero.
- `empty()`: Checks if the vector contains no elements.
Example of checking if a vector is empty:
if (numbers.empty()) {
std::cout << "Numbers vector is empty.";
}
Using Vectors with Algorithms
Vectors can be used seamlessly with STL algorithms to perform complex operations. For instance, you can use `std::transform()` to apply a function to each element:
#include <algorithm>
std::transform(numbers.begin(), numbers.end(), numbers.begin(), [](int num) {
return num * 2; // Doubles each element in the vector
});
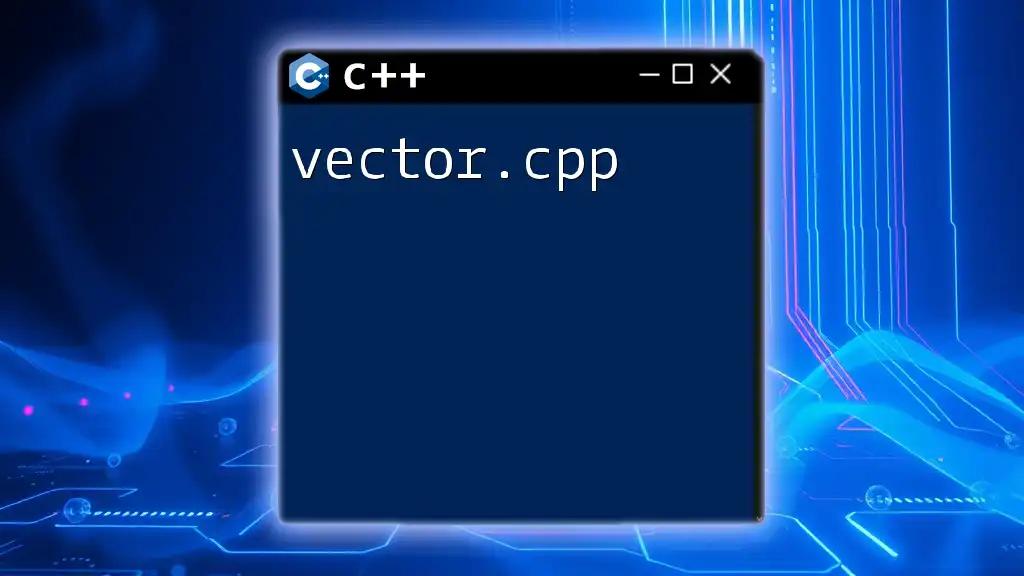
Real-World Applications of Vectors
Using Vectors in Practical Scenarios
Vectors are ideal for handling dynamic data. For example, consider a scenario where you need to store students' grades:
std::vector<double> grades = {88.5, 92.0, 76.5};
// Perform operations like adding or removing grades as needed
Advantages of Vectors in Complex Applications
Vectors play a critical role in complex applications, such as:
- Game Development: Managing dynamic game objects or player scores.
- User Configurations: Easily handling user input settings that may change during execution.
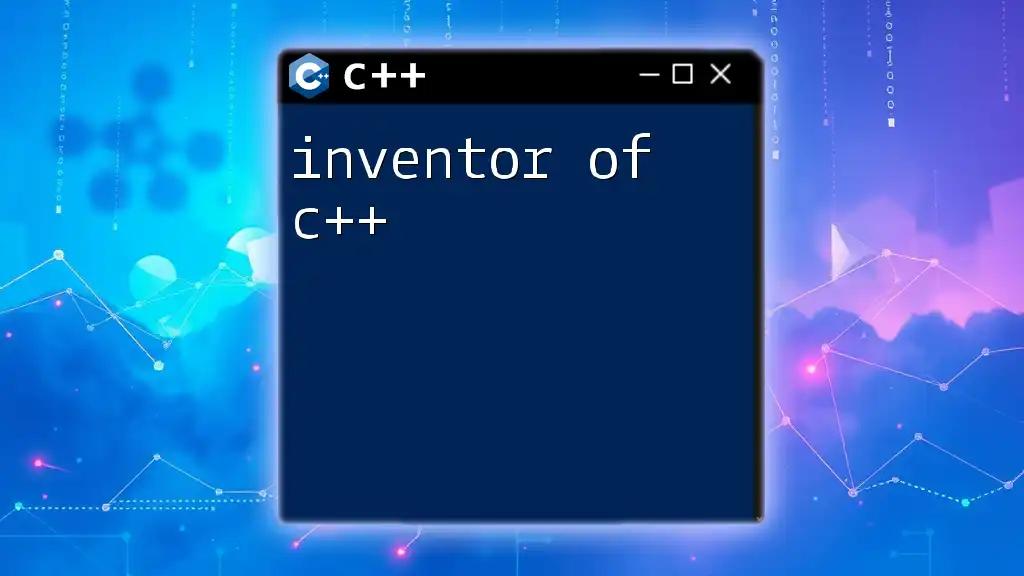
Best Practices for Using Vectors in C++
Memory Management Tips
When working with vectors, it's crucial to manage memory effectively to avoid overhead. Opt for `reserve()` to preallocate memory if you anticipate a large number of elements, minimizing reallocations:
numbers.reserve(100); // Reserving space for 100 elements
Choosing Vectors over Arrays
Use vectors instead of arrays when:
- You need dynamic resizing.
- You require built-in functionalities and simplified memory management.
- Performance during accessing elements is a priority due to contiguous memory.
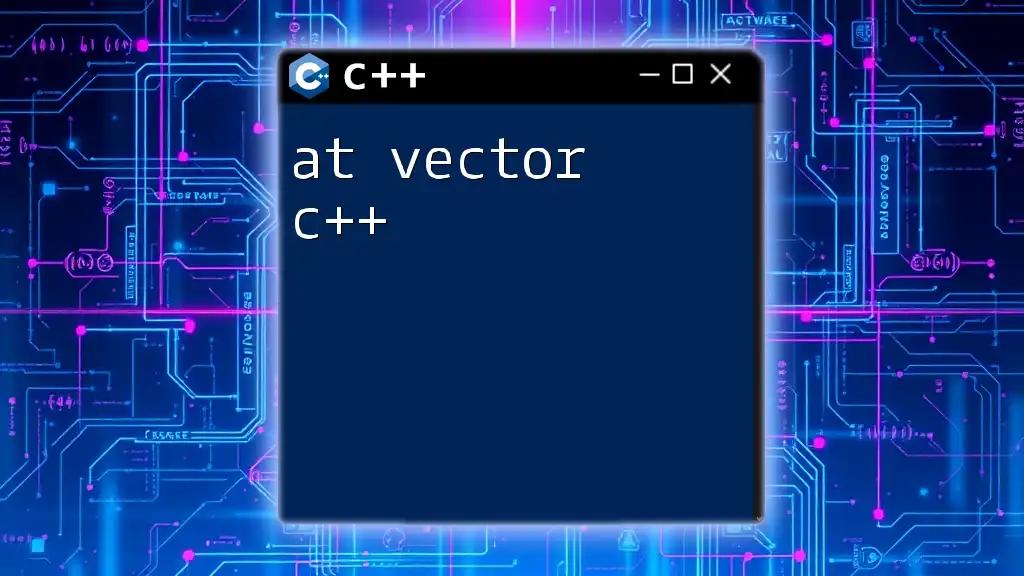
Conclusion
Mastering vector data in C++ is essential for any programmer. Vectors offer both flexibility and power, making them a critical tool in your programming arsenal. As you practice the examples provided and explore further, you'll find that vectors enable you to write cleaner, more efficient code.
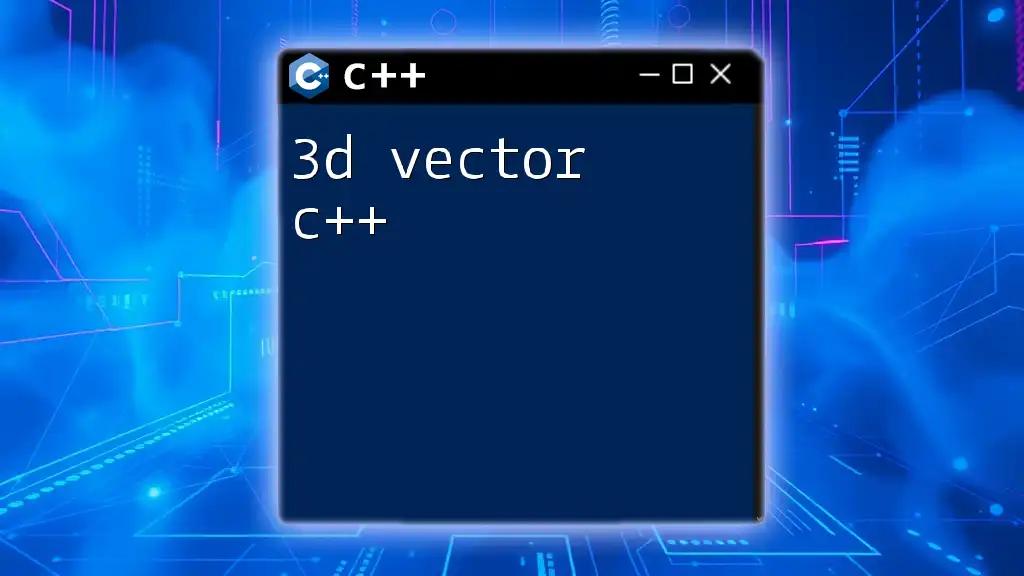
Additional Resources
To deepen your understanding of C++ vectors, consider exploring books such as "The C++ Programming Language" by Bjarne Stroustrup and joining online forums and communities focused on C++ development. Challenge yourself by taking on practice problems that involve manipulating vectors to solidify your knowledge.
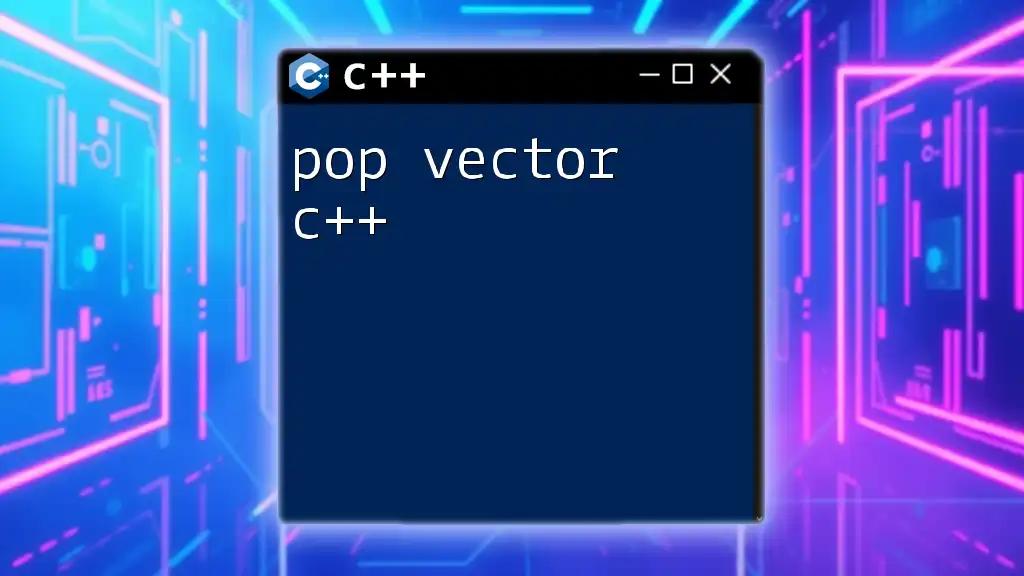
Call to Action
Begin experimenting with vectors in your projects today! Whether you're managing data in a game or handling user configurations, vectors will streamline your process. If you have questions or need further clarification, feel free to reach out for a deeper discussion on vector data in C++.